ui.igTreeGridHiding
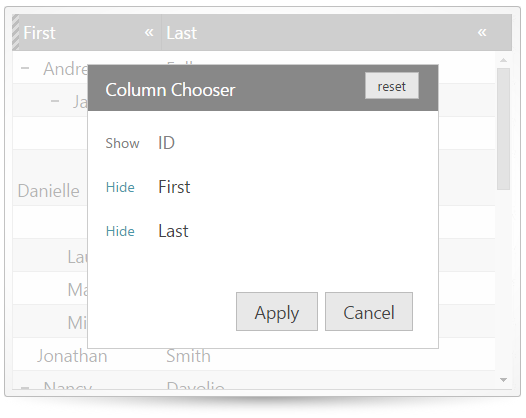
igTreeGrid の列の非表示機能を使用すると、ユーザーは、グリッドの表示レイアウトから列を削除および復元できます。この API のクラス、オプション、イベント、メソッドおよびテーマに関する詳細は、上記の関連するタブを参照してください。
この API を使用して作業を開始するための情報はここをクリックしてください。igGrid コントロールの必要なスクリプトおよびテーマを参照する方法については、 「Ignite UI で JavaScript リソースを使用する」および「Ignite UI のスタイル設定とテーマ」を参照してください。
コード サンプル
<!DOCTYPE html> <html> <head> <!-- Infragistics Combined CSS --> <link href="css/themes/infragistics/infragistics.theme.css" rel="stylesheet" type="text/css" /> <link href="css/structure/infragistics.css" rel="stylesheet" type="text/css" /> <!-- jQuery Core --> <script src="js/jquery.js" type="text/javascript"></script> <!-- jQuery UI --> <script src="js/jquery-ui.js" type="text/javascript"></script> <!-- Infragistics Combined Scripts --> <script src="js/infragistics.core.js" type="text/javascript"></script> <script src="js/infragistics.lob.js" type="text/javascript"></script> <script type="text/javascript"> var employees = [ { "employeeId": 0, "supervisorId": -1, "firstName": "Andrew", "lastName": "Fuller" }, { "employeeId": 1, "supervisorId": -1, "firstName": "Jonathan", "lastName": "Smith" }, { "employeeId": 2, "supervisorId": -1, "firstName": "Nancy", "lastName": "Davolio" }, { "employeeId": 3, "supervisorId": -1, "firstName": "Steven", "lastName": "Buchanan" }, // Andrew Fuller's direct reports { "employeeId": 4, "supervisorId": 0, "firstName": "Janet", "lastName": "Leverling" }, { "employeeId": 5, "supervisorId": 0, "firstName": "Laura", "lastName": "Callahan" }, { "employeeId": 6, "supervisorId": 0, "firstName": "Margaret", "lastName": "Peacock" }, { "employeeId": 7, "supervisorId": 0, "firstName": "Michael", "lastName": "Suyama" }, // Janet Leverling's direct reports { "employeeId": 8, "supervisorId": 4, "firstName": "Anne", "lastName": "Dodsworth" }, { "employeeId": 9, "supervisorId": 4, "firstName": "Danielle", "lastName": "Davis" }, { "employeeId": 10, "supervisorId": 4, "firstName": "Robert", "lastName": "King" }, // Nancy Davolio's direct reports { "employeeId": 11, "supervisorId": 2, "firstName": "Peter", "lastName": "Lewis" }, { "employeeId": 12, "supervisorId": 2, "firstName": "Ryder", "lastName": "Zenaida" }, { "employeeId": 13, "supervisorId": 2, "firstName": "Wang", "lastName": "Mercedes" }, // Steve Buchanan's direct reports { "employeeId": 14, "supervisorId": 3, "firstName": "Theodore", "lastName": "Zia" }, { "employeeId": 15, "supervisorId": 3, "firstName": "Lacota", "lastName": "Mufutau" }, // Lacota Mufutau's direct reports { "employeeId": 16, "supervisorId": 15, "firstName": "Jin", "lastName": "Elliott" }, { "employeeId": 17, "supervisorId": 15, "firstName": "Armand", "lastName": "Ross" }, { "employeeId": 18, "supervisorId": 15, "firstName": "Dane", "lastName": "Rodriquez" }, // Dane Rodriquez's direct reports { "employeeId": 19, "supervisorId": 18, "firstName": "Declan", "lastName": "Lester" }, { "employeeId": 20, "supervisorId": 18, "firstName": "Bernard", "lastName": "Jarvis" }, // Bernard Jarvis' direct report { "employeeId": 21, "supervisorId": 20, "firstName": "Jeremy", "lastName": "Donaldson" } ]; $(function () { $("#treegrid").igTreeGrid({ dataSource: employees, primaryKey: "employeeId", foreignKey: "supervisorId", autoGenerateColumns: false, columns: [ { headerText: "ID", key: "employeeId", width: "150px", dataType: "number" }, { headerText: "First", key: "firstName", width: "150px", dataType: "string" }, { headerText: "Last", key: "lastName", width: "150px", dataType: "string" } ], features: [ { name: "Hiding", columnSettings: [ { columnKey: "employeeId", allowHiding: true, hidden: true }, { columnKey: "lastName", allowHiding: false } ] } ] }); }); </script> </head> <body> <table id="treegrid"></table> </body> </html>
関連トピック
依存関係
-
columnChooserAnimationDuration
継承- タイプ:
- number
- デフォルト:
- 200
モーダル ダイアログを表示/非表示にするためにアニメーション期間のミリ秒の時間を指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserAnimationDuration: 300 } ] }); //Get var duration = $(".selector").igTreeGridHiding("option", "columnChooserAnimationDuration");
-
columnChooserButtonApplyText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
モーダル ダイアログで変更を適用するボタンのテキストを指定します。locale.columnChooserButtonApplyText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserButtonApplyText: "Apply" } ] }); //Get var text = $(".selector").igTreeGridHiding("option", "columnChooserButtonApplyText");
-
columnChooserButtonCancelText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
モーダル ダイアログで変更をキャンセルするボタンのテキストを指定します。locale.columnChooserButtonCancelText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserButtonCancelText: "Cancel" } ] }); //Get var text = $(".selector").igTreeGridHiding("option", "columnChooserButtonCancelText");
-
columnChooserCaptionText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
列チューザー ダイアログのキャプション。locale.columnChooserCaptionText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserCaptionText: "New Caption" } ] }); //Get var columnChooserCaptionText = $(".selector").igTreeGridHiding("option", "columnChooserCaptionText");
-
columnChooserContainment
継承- タイプ:
- string
- デフォルト:
- "owner"
コンテインメント動作を制御します。
owner 列選択ダイアログはグリッド領域のみにドラッグ可能です。
window 列選択ダイアログはウィンドウ領域の全体にドラッグ可能です。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserContainment: "window" } ] }); //Get var columnChooserContainment = $(".selector").igTreeGridHiding("option", "columnChooserContainment");
-
columnChooserDisplayText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
列チューザー ダイアログを開始するために、ドロップ ダウンのツール メニュー (機能選択) で使用されるテキスト。locale.columnChooserDisplayText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserDisplayText: "New Text" } ] }); //Get var columnChooserDisplayText = $(".selector").igTreeGridHiding("option", "columnChooserDisplayText");
-
columnChooserHeight
継承- タイプ:
- string
- デフォルト:
- ""
デフォルトの列セレクターの高さ。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserHeight: 100 } ] }); //Get var columnChooserHeight = $(".selector").igTreeGridHiding("option", "columnChooserHeight");
-
columnChooserHideOnClick
継承- タイプ:
- bool
- デフォルト:
- false
列を表示/非表示にするためにクリックして直接表示するか、それとも非表示にするかを指定します。columnChooserHideOnClick が false の場合、適用ボタンとキャンセルボタンがモーダル ダイアログの下側に表示されます。[適用] ボタンがクリックされた後で列が表示/非表示になります。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserHideOnClick: true } ] }); //Get var onClick = $(".selector").igTreeGridHiding("option", "columnChooserHideOnClick");
-
columnChooserHideText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
列を非表示にするために列選択で使用されるテキスト。locale.columnChooserHideText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserHideText: "Hide column" } ] }); //Get var text = $(".selector").igTreeGridHiding("option", "columnChooserHideText");
-
columnChooserResetButtonLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
リセット ボタン用のテキストラベル。locale.columnChooserResetButtonLabel オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserResetButtonLabel: "Reset" } ] }); //Get var text = $(".selector").igTreeGridHiding("option", "columnChooserResetButtonLabel");
-
columnChooserShowText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
列を表示するために列選択で使用されるテキスト。locale.columnChooserShowText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserShowText: "Show Column" } ] }); //Get var text = $(".selector").igTreeGridHiding("option", "columnChooserShowText");
-
columnChooserWidth
継承- タイプ:
- string
- デフォルト:
- "350"
デフォルトの列セレクターの幅。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserWidth: 100 } ] }); //Get var columnChooserWidth = $(".selector").igTreeGridHiding("option", "columnChooserWidth");
-
columnHideText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
列を非表示にするために、ドロップ ダウンのツール メニュー (機能選択) で使用されるテキスト。locale.columnHideText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnHideText: "Functionallity to hide the column" } ] }); //Get var columnHideText = $(".selector").igTreeGridHiding("option", "columnHideText");
-
columnSettings
継承- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
非表示オプションを列ごとに指定する列設定のリスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnSettings: [ { columnKey: "ProductID", allowHiding: true }, ] } ] }); //Get var columnSettings = $(".selector").igTreeGridHiding("option", "columnSettings");
-
allowHiding
- タイプ:
- bool
- デフォルト:
- true
列の非表示を許可します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name: "Hiding", columnSettings: [ { columnIndex: 0, allowHiding: true }, ] } ] }); //Get var columnSettings = $(".selector").igTreeGridHiding("option", "columnSettings"); var allowHiding = columnSettings[0].allowHiding;
-
columnIndex
- タイプ:
- number
- デフォルト:
- null
列インデックス。列キーの代わりに使用できます。列設定の生成には列を常に識別子として使用することを推奨します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name: "Hiding", columnSettings: [ { columnIndex: 0, allowHiding: true }, ] } ] });
-
columnKey
- タイプ:
- string
- デフォルト:
- null
列キー。これは、columnIndex が設定されていない場合に各列設定で必要なプロパティです。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name: "Hiding", columnSettings: [ { columnKey: "ProductID", allowHiding: true }, ] } ] });
-
hidden
- タイプ:
- bool
- デフォルト:
- false
列の初期表示状態 (表示/非表示) を設定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name: "Hiding", columnSettings: [ { columnKey: "ProductID", hidden: true }, ] } ] });
-
dialogWidget
継承- タイプ:
- string
- デフォルト:
- "igGridModalDialog"
使用するダイアログ ウィジェットの名前。$.ui.igGridModalDialog から継承します。
コード サンプル
//create dialog widget that inherits from $.ui.igGridModalDialog $.widget("ui.CustomDialog", $.ui.igGridModalDialog, {}); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", dialogWidget: "CustomDialog" } ] }); //Get var dialogWidget = $(".selector").igTreeGridHiding("option", "dialogWidget");
-
dropDownAnimationDuration
継承- タイプ:
- number
- デフォルト:
- 500
ドロップダウン アニメーションの時間 (ミリ秒)。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", dropDownAnimationDuration: 500 } ] }); //Get var dropDownAnimationDuration = $(".selector").igTreeGridHiding("option", "dropDownAnimationDuration");
-
hiddenColumnIndicatorHeaderWidth
継承- タイプ:
- number
- デフォルト:
- 7
ヘッダー内の非表示の列インジケーターの幅 (ピクセル)。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", hiddenColumnIndicatorHeaderWidth: 15 } ] }); //Get var hiddenColumnIndicatorHeaderWidth = $(".selector").igTreeGridHiding("option", "hiddenColumnIndicatorHeaderWidth");
-
hiddenColumnIndicatorTooltipText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
非表示の列インジケーターのツールチップに表示されるテキスト。locale.hiddenColumnIndicatorTooltipText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", hiddenColumnIndicatorTooltipText: "New Tooltip Text" } ] }); //Get var hiddenColumnIndicatorTooltipText = $(".selector").igTreeGridHiding("option", "hiddenColumnIndicatorTooltipText");
-
locale
継承- タイプ:
- object
- デフォルト:
- {}
-
columnChooserButtonApplyText
- タイプ:
- string
- デフォルト:
- ""
モーダル ダイアログで変更を適用するボタンのテキストを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnChooserButtonApplyText: "Apply" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnChooserButtonApplyText; // Set $(".selector").igTreeGridHiding("option", "locale", { columnChooserButtonApplyText: "Apply" });
-
columnChooserButtonCancelText
- タイプ:
- string
- デフォルト:
- ""
モーダル ダイアログで変更を取り消すボタンのテキストを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnChooserButtonCancelText: "Cancel" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnChooserButtonCancelText; // Set $(".selector").igTreeGridHiding("option", "locale", { columnChooserButtonCancelText: "Cancel" });
-
columnChooserCaptionLabel
- タイプ:
- string
- デフォルト:
- ""
列チューザー ダイアログのキャプション。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnChooserCaptionLabel: "New Caption" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnChooserCaptionLabel; // Set $(".selector").igTreeGridHiding("option", "locale", { columnChooserCaptionLabel: "New Caption" });
-
columnChooserCloseButtonTooltip
- タイプ:
- string
- デフォルト:
- ""
列チューザー ダイアログの [閉じる] ボタンのツールチップ。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnChooserCloseButtonTooltip: "Close" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnChooserCloseButtonTooltip; // Set $(".selector").igTreeGridHiding("option", "locale", { columnChooserCloseButtonTooltip: "Close" });
-
columnChooserDisplayText
- タイプ:
- string
- デフォルト:
- ""
列チューザー ダイアログを開始するために、ドロップ ダウンのツール メニュー (機能選択) で使用されるテキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnChooserDisplayText: "New Text" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnChooserDisplayText; // Set $(".selector").igTreeGridHiding("option", "locale", { columnChooserDisplayText: "New Text" });
-
columnChooserHideText
- タイプ:
- string
- デフォルト:
- ""
列を非表示にするために列選択で使用されるテキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnChooserHideText: "Hide Column" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnChooserHideText; // Set $(".selector").igTreeGridHiding("option", "locale", { columnChooserHideText: "Hide Column" });
-
columnChooserResetButtonLabel
- タイプ:
- string
- デフォルト:
- ""
リセット ボタン用のテキストラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnChooserResetButtonLabel: "Reset" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnChooserResetButtonLabel; // Set $(".selector").igTreeGridHiding("option", "locale", { columnChooserResetButtonLabel: "Reset" });
-
columnChooserShowText
- タイプ:
- string
- デフォルト:
- ""
列を表示するために列選択で使用されるテキスト
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnChooserShowText: "Show Column" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnChooserShowText; // Set $(".selector").igTreeGridHiding("option", "locale", { columnChooserShowText: "Show Column" });
-
columnHideText
- タイプ:
- string
- デフォルト:
- ""
列を非表示にするために、ドロップ ダウンのツール メニュー (機能選択) で使用されるテキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { columnHideText: "New Tooltip Text" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").columnHideText; // Set $(".selector").igTreeGridHiding("option", "locale", { columnHideText: "New Tooltip Text" });
-
hiddenColumnIndicatorTooltipText
- タイプ:
- string
- デフォルト:
- ""
非表示の列インジケーターのツールチップに表示されるテキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { hiddenColumnIndicatorTooltipText: "New Tooltip Text" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").hiddenColumnIndicatorTooltipText; // Set $(".selector").igTreeGridHiding("option", "locale", { hiddenColumnIndicatorTooltipText: "New Tooltip Text" });
-
hideColumnIconTooltip
- タイプ:
- string
- デフォルト:
- ""
非表示列アイコンのツールチップを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [{ name: "Hiding", locale: { hideColumnIconTooltip: "Hide" } }] }); // Get var text = $(".selector").igTreeGridHiding("option", "locale").hideColumnIconTooltip; // Set $(".selector").igTreeGridHiding("option", "locale", { hideColumnIconTooltip: "Hide" });
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
columnChooserButtonApplyClick
継承- キャンセル可能:
- false
列選択の [適用] ボタンがクリックされたときに発生するイベント。
コード サンプル
//Bind after initialization $(document).delegate(".selector", "igtreegridhidingcolumnchooserbuttonapplyclick", function (evt, ui) { //return the triggered event evt; // reference to the igTreeGridHiding widget. ui.owner; // reference to the grid widget. ui.owner.grid; // reference to the Column Chooser jQuery element. ui.columnChooserElement; // reference to array of columns identifiers which should be shown. ui.columnsToShow; // reference to array of columns identifiers which should be hidden. ui.columnsToHide; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserButtonApplyClick: function(evt, ui){ ... } } ] });
-
columnChooserButtonResetClick
継承- キャンセル可能:
- false
列セレクターの Reset ボタンをクリックすると、イベントが開始します。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnchooserbuttonresetclick", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the igTreeGridHiding widget. ui.owner; // reference to the grid widget. ui.owner.grid; // reference to the Column Chooser jQuery element. ui.columnChooserElement; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserButtonResetClick: function(evt, ui){ ... } } ] });
-
columnChooserClosed
継承- キャンセル可能:
- false
列選択が閉じた後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnchooserclosed", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the Column Chooser jQuery element. ui.columnChooserElement; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserClosed: function(evt, ui){ ... } } ] });
-
columnChooserClosing
継承- キャンセル可能:
- true
列選択が閉じる前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnchooserclosing", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the Column Chooser jQuery element. ui.columnChooserElement; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserClosing: function(evt, ui){ ... } } ] });
-
columnChooserContentsRendered
継承- キャンセル可能:
- false
列選択のコンテンツが描画された後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnchoosercontentsrendered", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the Column Chooser jQuery element. ui.columnChooserElement; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserContentsRendered: function(evt, ui){ ... } } ] });
-
columnChooserContentsRendering
継承- キャンセル可能:
- true
列選択のコンテンツが描画される前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnchoosercontentsrendering", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the Column Chooser jQuery element. ui.columnChooserElement; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserContentsRendering: function(evt, ui){ ... } } ] });
-
columnChooserMoving
継承- キャンセル可能:
- true
列選択の位置が変わるたびに発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
GridHiding ウィジェットへの参照を取得します。
-
owner.gridタイプ: Object
グリッド ウィジェットへの参照を取得します。
-
columnChooserElementタイプ: jQuery
列の選択要素への参照を取得します。これは jQuery オブジェクトです。
-
originalPositionタイプ: Object
ページを基準とした列の選択 DIV の元の位置を { top, left } として取得します。
-
positionタイプ: Object
ページを基準とした列の選択 DIV の現在の位置を { top, left } として取得します。
-
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnchoosermoving", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the Column Chooser jQuery element. ui.columnChooserElement; // reference to the igTreeGridHiding widget ui.owner; // current postion ui.position; // previous position ui.originalPosition; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserMoving: function(evt, ui){ ... } } ] });
-
columnChooserOpened
継承- キャンセル可能:
- false
列選択が既に開いた後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnchooseropened", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the Column Chooser jQuery element. ui.columnChooserElement; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserOpened: function(evt, ui){ ... } } ] });
-
columnChooserOpening
継承- キャンセル可能:
- true
列選択が開く前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnchooseropening", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the Column Chooser jQuery element. ui.columnChooserElement; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnChooserOpening: function(evt, ui){ ... } } ] });
-
columnHidden
継承- キャンセル可能:
- false
非表示が実行され結果が描画された後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnhidden", ".selector", function (evt, ui) { //return the triggered event evt; // the index of the column, which is hidden ui.columnIndex; // the key of the column, which is hidden ui.columnKey; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnHidden: function(evt, ui){ ... } } ] });
-
columnHiding
継承- キャンセル可能:
- true
非表示操作が実行される前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnhiding", ".selector", function (evt, ui) { //return the triggered event evt; // the index of the column, which is going to be hidden ui.columnIndex; // the key of the column, which is going to be hidden ui.columnKey; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnHiding: function(evt, ui){ ... } } ] });
-
columnHidingRefused
継承- キャンセル可能:
- false
固定または固定解除領域ですべての列を非表示するときに発生されるイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnhidingrefused", ".selector", function (evt, ui) { //return the triggered event evt; // array of column keys, which are refused to hide ui.columnKeys; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnHidingRefused: function(evt, ui){ ... } }, { name: "ColumnFixing" } ] });
-
columnShowing
継承- キャンセル可能:
- true
表示操作が実行される前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnshowing", ".selector", function (evt, ui) { //return the triggered event evt; // the index of the column, which is going to be shown ui.columnIndex; // the key of the column, which is going to be shown ui.columnKey; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnShowing: function(evt, ui){ ... } } ] });
-
columnShowingRefused
継承- キャンセル可能:
- false
固定領域に列を表示し、固定領域の幅はグリッドの幅より大きい場合に発生されるイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnshowingrefused", ".selector", function (evt, ui) { //return the triggered event evt; // array of column keys, which are refused to show ui.columnKeys; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnShowingRefused: function(evt, ui){ ... } }, { name: "ColumnFixing" } ] });
-
columnShown
継承- キャンセル可能:
- false
表示が実行され結果が描画された後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingcolumnshown", ".selector", function (evt, ui) { //return the triggered event evt; // the index of the column, which is shown ui.columnIndex; // the key of the column, which is shown ui.columnKey; // reference to the igTreeGridHiding widget ui.owner; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", columnShown: function(evt, ui){ ... } } ] });
-
multiColumnHiding
継承- キャンセル可能:
- true
非表示操作が実行される前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridhidingmulticolumnhiding", ".selector", function (evt, ui) { //return the triggered event evt; // reference to the igTreeGridHiding widget ui.owner; // reference to the igTreeGrid widget ui.owner.grid; // array of the hidden column keys ui.columnKeys; }); //Initialize $(".selector").igTreeGrid({ features : [ { name : "Hiding", multiColumnHiding: function(evt, ui){ ... } } ] });
-
changeLocale
継承- .igTreeGridHiding( "changeLocale" );
ウィジェット要素のすべてのロケールを options.language に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。コード サンプル
$(".selector").igTreeGridHiding("changeLocale");
-
destroy
- .igTreeGridHiding( "destroy" );
非表示ウィジェットを破棄します。
コード サンプル
$(".selector").igTreeGridHiding("destroy");
-
hideColumn
継承- .igTreeGridHiding( "hideColumn", column:object, [callback:function] );
表示される列を非表示にします。列が非表示の場合、このメソッドは何もしません。
注: このメソッドは非同期です。ただちに返し、以後のコードは並行して実行されます。ランタイム エラーを発生する場合があります。エラーを回避するには、メソッドによって提供されるコールバック パラメーターに以後のコードを挿入します。- column
- タイプ:object
- 列の識別子。数が提供した場合、列インデックスとして使用され、文字列が提供した場合、列キーとして使用されます。
- callback
- タイプ:function
- オプション
- 列を非表示したときに呼び出すカスタム関数を指定します (オプション)。
コード サンプル
//Hide the column with columnIndex 0 $(".selector").igTreeGridHiding("hideColumn", 0); //Hide the column with a key 'Name' $(".selector").igTreeGridHiding("hideColumn", "Name"); //Hide the column with a key 'Name', with a callback function which executes after the column is hidden (hiding column operation is asynchronous) $(".selector").igTreeGridHiding("hideColumn", "Name", function () { // Custom code which executes after the hide column operation is complete. });
-
hideColumnChooser
継承- .igTreeGridHiding( "hideColumnChooser" );
列選択ダイアログを非表示にします。非表示の場合、メソッドは何も行いません。
コード サンプル
$(".selector").igTreeGridHiding("hideColumnChooser");
-
hideMultiColumns
継承- .igTreeGridHiding( "hideMultiColumns", columns:array, [callback:function] );
配列によって指定される表示列を非表示にします。列が非表示の場合、このメソッドは何もしません。
注: このメソッドは非同期です。ただちに返し、以後のコードは並行して実行されます。ランタイム エラーを発生する場合があります。エラーを回避するには、メソッドによって提供されるコールバック パラメーターに以後のコードを挿入します。- columns
- タイプ:array
- 列の識別子の配列。数が提供した場合、列インデックスとして使用され、文字列が提供した場合、列キーとして使用されます。
- callback
- タイプ:function
- オプション
- すべての列が非表示したときに呼び出すカスタム関数を指定します (オプション)。
コード サンプル
// the parameter is an array of the column keys $(".selector").igTreeGridHiding("hideMultiColumns", ['FirstName', 'Address', 'PhoneNumber'] ); // the parameter is an array of the column index $(".selector").igTreeGridHiding("hideMultiColumns", [1, 3, 4] ); // the parameters are an array of column keys and a callback function which executes after the columns are hidden $(".selector").igTreeGridHiding("hideMultiColumns", ['FirstName', 'Address', 'PhoneNumber'], function () { // Custom code which executes after the hide columns operation is complete. });
-
isToRenderButtonReset
継承- .igTreeGridHiding( "isToRenderButtonReset" );
列チューザー ダイアログのリセット ボタンが描画されるかどうかを取得します。
コード サンプル
$(".selector").igTreeGridHiding("isToRenderButtonReset");
-
removeColumnChooserResetButton
継承- .igTreeGridHiding( "removeColumnChooserResetButton" );
列選択のモーダル ダイアログから [リセット] ボタンを削除します
コード サンプル
$(".selector").igTreeGridHiding("removeColumnChooserResetButton");
-
renderColumnChooserResetButton
継承- .igTreeGridHiding( "renderColumnChooserResetButton" );
列チューザー ダイアログのリセット ボタンを描画します。
コード サンプル
$(".selector").igTreeGridHiding("renderColumnChooserResetButton");
-
resetHidingColumnChooser
継承- .igTreeGridHiding( "resetHidingColumnChooser" );
非表示 / 表示した列を、ダイアログの初期状態 (開いた状態) にリセットします 。
コード サンプル
$(".selector").igTreeGridHiding("resetHidingColumnChooser");
-
showColumn
継承- .igTreeGridHiding( "showColumn", column:object, [callback:function] );
非表示の列を表示します。列が非表示でない場合、このメソッドは何もしません。
注: このメソッドは非同期です。ただちに返し、以後のコードは並行して実行されます。ランタイム エラーを発生する場合があります。エラーを回避するには、メソッドによって提供されるコールバック パラメーターに以後のコードを挿入します。- column
- タイプ:object
- 列の識別子。数が提供した場合、列インデックスとして使用され、文字列が提供した場合、列キーとして使用されます。
- callback
- タイプ:function
- オプション
- 列が表示したときに呼び出すカスタム関数を指定します (オプション)。
コード サンプル
//Show the column with columnIndex 0 $(".selector").igTreeGridHiding("showColumn", 0); //Show the column with a key 'Name' $(".selector").igTreeGridHiding("showColumn", "Name"); //Show the column with a key 'Name', with a callback function which executes after the column is shown (showing column operation is asynchronous) $(".selector").igTreeGridHiding("showColumn", "Name", function () { // Custom code which executes after the show column operation is complete. });
-
showColumnChooser
継承- .igTreeGridHiding( "showColumnChooser" );
列選択ダイアログを表示します。表示されている場合、メソッドは何も行いません。
コード サンプル
$(".selector").igTreeGridHiding("showColumnChooser");
-
showMultiColumns
継承- .igTreeGridHiding( "showMultiColumns", columns:array, [callback:function] );
配列によって指定される表示列を表示にします。列が表示される場合、このメソッドは何もしません。
注: このメソッドは非同期です。ただちに返し、以後のコードは並行して実行されます。ランタイム エラーを発生する場合があります。エラーを回避するには、メソッドによって提供されるコールバック パラメーターに以後のコードを挿入します。- columns
- タイプ:array
- 列の識別子の配列。数が提供した場合、列インデックスとして使用され、文字列が提供した場合、列キーとして使用されます。
- callback
- タイプ:function
- オプション
- すべての列が表示したときに呼び出すカスタム関数を指定します (オプション)。
コード サンプル
// the parameter is an array of the column keys $(".selector").igTreeGridHiding("showMultiColumns", ['FirstName', 'Address', 'PhoneNumber'] ); // the parameter is an array of the column index $(".selector").igTreeGridHiding("showMultiColumns", [1, 3, 4] ); // the parameters are an array of column keys and a callback function which executes after the columns are shown $(".selector").igTreeGridHiding("showMultiColumns", ['FirstName', 'Address', 'PhoneNumber'], function () { // Custom code which executes after the show columns operation is complete. });
-
ui-dialog ui-draggable ui-resizable ui-iggrid-dialog ui-widget ui-widget-content ui-corner-all
- 列選択要素に適用されるクラス。
-
ui-dialog-content ui-iggrid-columnchooser-content
- 列選択のダイアログ コンテンツに適用されるクラス。
-
ui-iggrid-columnchooser-handlebar
- 下部にある列チューザーのハンドルバーに適用されるクラス。
-
ui-dialog-titlebar ui-iggrid-columnchooser-caption ui-widget-header ui-corner-top ui-helper-reset ui-helper-clearfix
- 列選択ダイアログのヘッダー キャプション領域に適用されるクラス。
-
ui-dialog-title ui-iggrid-columnchooser-caption-title
- 列選択ダイアログのヘッダー キャプション タイトルに適用されるクラス。
-
ui-iggrid-columnchooser-hidebutton
- 列選択の (単一の列を表示する) リスト項目の [非表示] ボタンに適用されるクラス。
-
ui-iggrid-columnchooser-item ui-widget-content
- 列選択の (単一の列を表示する) リスト項目に適用されるクラス。
-
ui-iggrid-columnchooser-itemhidden
- 列が非表示される場合、列選択の (単一の列を表示する) リスト項目に適用されるクラス。
-
ui-iggrid-dialog-text
- 列選択の (単一の列を表示する) リスト項目の [表示] ボタンに適用されるクラス。
-
ui-iggrid-columnchooser-listitems
- 列選択の (すべての列を表示する) リストに適用されるクラス。
-
ui-icon ui-iggrid-icon-hide
- 非表示機能の機能選択アイコンに適用されるクラス。
-
ui-icon ui-iggrid-icon-column-chooser
- 列選択機能の機能選択アイコンに適用されるクラス。
-
ui-iggrid-hiding-hiddencolumnindicator
- ヘッダーの非表示の列インジケーターに適用されるクラス。
-
ui-iggrid-hiding-indicator-mouseover
- マウスがその上にある時に、ヘッダーの非表示の列インジケーターに適用されるクラス。
-
ui-iggrid-hiding-indicator-selected
- 選択された (ユーザーがクリックし、ドロップダウンが表示されている) ときに、ヘッダーの非表示の列インジケーターに適用されるクラス。
-
ui-iggrid-hiding-dropdown-dialog ui-widget ui-widget-content ui-corner-all
- 非表示の列のドロップダウン div に適用されるクラス。
-
ui-iggrid-hiding-dropdown-ddlistitemicons ui-state-default
- 非表示の列のドロップダウン li に適用されるクラス。
-
ui-iggrid-hiding-dropdown-listitem-hover ui-state-active ui-state-hover
- ホバー状態のときに、非表示の列のドロップダウン li に適用されるクラス。
-
ui-iggrid-hiding-dropdown-ddlistitemtext
- li 内の非表示の列のドロップダウン スパンに適用されるクラス。
-
ui-iggrid-hiding-dropdown-list ui-menu
- 非表示の列のドロップダウン ul に適用されるクラス。
-
ui-iggrid-hiding-indicator
- 非表示のヘッダー アイコンに適用されるクラス。