mobile.igListViewFiltering
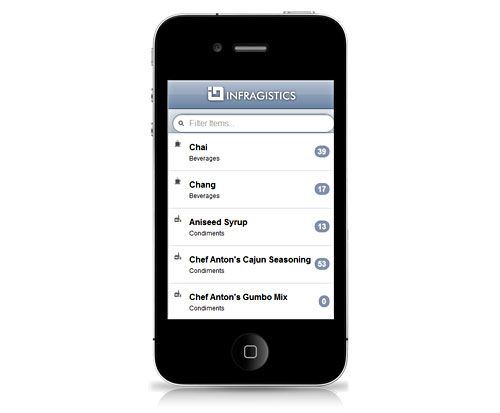
The igListView Filtering feature is intended to provide filtering functionality in the igListView control. Supported in the control are search box and filter presets to achieve filtering in the list. Filtering can be configured for remote or local filtering. The igListView control adheres to the jQuery Mobile approach of using markup-based initialization and configuration along with the standard jQuery initialization. For more information on the igListView control’s HTML data-* attributes see igListView Data Attribute Reference.
The following code snippet demonstrates how to initialize the igListView control with filtering feature enabled.
Click here for more information on how to get started using this API. For details on how to reference the required scripts and themes for the igList control read, Using JavaScript Resources in IgniteUI and Styling and Theming IgniteUI.Code Sample
<!doctype html> <html> <head> <!-- jQuery Mobile Styles --> <link rel="stylesheet" href="../content/jqm/jquery.mobile.structure.min.css" /> <!-- jQuery Core --> <script type="text/javascript" src="js/jquery.js"></script> <!-- jQuery Mobile Core --> <script type="text/javascript" src="js/jquery.mobile.js"><script> <!-- Infragistics mobile loader --> <script type="text/javascript" src="js/infragistics.mobile.loader.js"></script> <script type="text/javascript"> var northwindEmployees = [ { "ID": 1, "Name": "Davolio, Nancy", "Title": "Sales Representative", "ImageUrl": "../content/images/nw/employees/1.png", "Phone": "(206) 555-9857", "PhoneUrl": "tel:(206) 555-9857" }, { "ID": 2, "Name": "Fuller, Andrew", "Title": "Vice President, Sales", "ImageUrl": "../content/images/nw/employees/2.png", "Phone": "(206) 555-9482", "PhoneUrl": "tel:(206) 555-9482" }, { "ID": 3, "Name": "Leverling, Janet", "Title": "Sales Representative", "ImageUrl": "../content/images/nw/employees/3.png", "Phone": "(206) 555-3412", "PhoneUrl": "tel:(206) 555-3412" }, { "ID": 4, "Name": "Peacock, Margaret", "Title": "Sales Representative", "ImageUrl": "../content/images/nw/employees/4.png", "Phone": "(206) 555-8122", "PhoneUrl": "tel:(206) 555-8122" }, { "ID": 5, "Name": "Buchanan, Steven", "Title": "Sales Manager", "ImageUrl": "../content/images/nw/employees/5.png", "Phone": "(71) 555-4848", "PhoneUrl": "tel:(71) 555-4848" }, { "ID": 6, "Name": "Suyama, Michael", "Title": "Sales Representative", "ImageUrl": "../content/images/nw/employees/6.png", "Phone": "(71) 555-7773", "PhoneUrl": "tel:(71) 555-7773" }, { "ID": 7, "Name": "King, Robert", "Title": "Sales Representative", "ImageUrl": "../content/images/nw/employees/7.png", "Phone": "(71) 555-5598", "PhoneUrl": "tel:(71) 555-5598" }, { "ID": 8, "Name": "Callahan, Laura", "Title": "Inside Sales Coordinator", "ImageUrl": "../content/images/nw/employees/8.png", "Phone": "(206) 555-1189", "PhoneUrl": "tel:(206) 555-1189" }, { "ID": 9, "Name": "Dodsworth, Anne", "Title": "Sales Representative", "ImageUrl": "../content/images/nw/employees/9.png", "Phone": "(71) 555-4444", "PhoneUrl": "tel:(71) 555-4444" } ]; </script> <script type="text/javascript"> $.ig.loader({ scriptPath: "js", cssPath: "css", resources: "igmList.Filtering", theme: "ios" }); </script> </head> <body> <ul id="contactsListView" data-role="iglistview" data-icon-mode="thumbnail" data-data-source="northwindEmployees" data-bindings-header-key="Name" data-bindings-primary-key="ID" data-bindings-text-key="Phone" data-bindings-image-url-key="ImageUrl" data-filtering="true" data-filtering-search-bar-place-holder="Filter Contacts..."> </ul> </body> </html>
Related Samples
Related Topics
Dependencies
-
caseSensitive
- Type:
- enumeration
- Default:
- false
Enables/disables filtering case sensitivity.
Members
- true
- Type:bool
- Enables filtering case sensitivity.
- false
- Type:bool
- Disables filtering case sensitivity.
Code Sample
//Initialize $(".selector").igListView({ dataSource: northwindEmployees, features: [ { name: "Filtering", caseSensitive: true } ] }); //Get var caseSensitive = $(".selector").igListViewFiltering("option", "caseSensitive");
-
filteredFields
- Type:
- array
- Default:
- []
- Elements Type:
- object
A list of key/value pairs (fieldName, searchValue, condition, logic) representing the filtered fields.
Code Sample
//Initialize $(".selector").igListView({ dataSource: northwindEmployees, features: [ { name: "Filtering", filteredFields: [ { fieldName: "Name", searchValue: "Davolio", condition: "contains" } ] } ] }); //Get var filteredFields = $(".selector").igListViewFiltering("option", "filteredFields");
-
condition
- Type:
- enumeration
- Default:
- ""
The condition to use to filter the given field.
Members
- empty
- Type:string
- notEmpty
- Type:string
- null
- Type:string
- notNull
- Type:string
- equals
- Type:string
- doesNotEqual
- Type:string
- startsWith
- Type:string
- contains
- Type:string
- doesNotContain
- Type:string
- endsWith
- Type:string
- greaterThan
- Type:string
- lessThan
- Type:string
- greaterThanOrEqualTo
- Type:string
- lessThanOrEqualTo
- Type:string
- true
- Type:bool
- false
- Type:bool
- on
- Type:string
- notOn
- Type:string
- before
- Type:string
- after
- Type:string
- today
- Type:string
- yesterday
- Type:string
- thisMonth
- Type:string
- lastMonth
- Type:string
- nextMonth
- Type:string
- thisYear
- Type:string
- nextYear
- Type:string
- lastYear
- Type:string
Code Sample
//Initialize $(".selector").igListView({ dataSource: northwindEmployees, features: [ { name: "Filtering", filteredFields: [ { fieldName: "Name", searchValue: "Davolio", condition: "contains" } ] } ] }); //Get var filteredFields = $(".selector").igListViewFiltering("option", "filteredFields");
-
fieldName
- Type:
- string
- Default:
- ""
The name of the field to filter by.
Code Sample
//Initialize $(".selector").igListView({ dataSource: northwindEmployees, features: [ { name: "Filtering", filteredFields: [ { fieldName: "Name", searchValue: "Davolio", condition: "contains" } ] } ] }); //Get var filteredFields = $(".selector").igListViewFiltering("option", "filteredFields");
-
logic
- Type:
- enumeration
- Default:
- AND
Whether to use boolean AND or OR if there are multiple fields to filter by.
Members
- AND
- Type:string
- All conditions must be true for a record to be shown.
- OR
- Type:string
- Just one condition must be true for a record to be shown.
Code Sample
//Initialize $(".selector").igListView({ dataSource: northwindEmployees, features: [ { name: "Filtering", filteredFields: [ { fieldName: "Name", searchValue: "Davolio", condition: "contains" logic: "AND" }, { fieldName: "Title", searchValue: "Sales Representative", condition: "equals" } ] } ] }); //Get var filteredFields = $(".selector").igListViewFiltering("option", "filteredFields");
-
searchValue
- Type:
- string
- Default:
- ""
The value to use for this field's filter (if applicable).
Code Sample
//Initialize $(".selector").igListView({ dataSource: northwindEmployees, features: [ { name: "Filtering", filteredFields: [ { fieldName: "Name", searchValue: "Davolio", condition: "contains" } ] } ] }); //Get var filteredFields = $(".selector").igListViewFiltering("option", "filteredFields");
-
filterExprUrlKey
- Type:
- string
- Default:
- null
URL key name that specifies how the filtering expressions will be encoded for remote requests, e.g. &filter('col') = startsWith. Default is OData.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", filterExprUrlKey : "filter" } ] }); //Get var filterExpr = $(".selector").igListViewFiltering("option", "filterExprUrlKey");
-
filterPresetsLabel
- Type:
- string
- Default:
- null
The text that is displayed above the filter presets.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", filterPresetsLabel : "Filter presets:" } ] }); //Get var presetsLabel = $(".selector").igListViewFiltering("option", "filterPresetsLabel");
-
filterState
- Type:
- string
- Default:
- "default"
The index of the preset that should be used, 'default' for none.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", filterState : "default" } ] }); //Get var filterState = $(".selector").igListViewFiltering("option", "filterState");
-
presets
- Type:
- array
- Default:
- []
- Elements Type:
- object
A list of preset filtering options.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", presets : [ { text: "Sales", filteredFields: [ { fieldName: "Title", searchValue: "Sales Representative", condition: "equals" } ] } ] } ] }); //Get var presets = $(".selector").igListViewFiltering("option", "presets");
-
filteredFields
- Type:
- array
- Default:
- []
- Elements Type:
- object
A list of key/value pairs (fieldName, searchValue, condition, logic) representing the filtered fields.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", presets : [ { text: "Sales", filteredFields: [ { fieldName: "Title", searchValue: "Sales Representative", condition: "equals" } ] } ] } ] }); //Get var presets = $(".selector").igListViewFiltering("option", "presets");
-
condition
- Type:
- enumeration
- Default:
- ""
The condition to use to filter the given field in this preset.
Members
- empty
- Type:string
- notEmpty
- Type:string
- null
- Type:string
- notNull
- Type:string
- equals
- Type:string
- doesNotEqual
- Type:string
- startsWith
- Type:string
- contains
- Type:string
- doesNotContain
- Type:string
- endsWith
- Type:string
- greaterThan
- Type:string
- lessThan
- Type:string
- greaterThanOrEqualTo
- Type:string
- lessThanOrEqualTo
- Type:string
- true
- Type:bool
- false
- Type:bool
- on
- Type:string
- notOn
- Type:string
- before
- Type:string
- after
- Type:string
- today
- Type:string
- yesterday
- Type:string
- thisMonth
- Type:string
- lastMonth
- Type:string
- nextMonth
- Type:string
- thisYear
- Type:string
- nextYear
- Type:string
- lastYear
- Type:string
-
fieldName
- Type:
- string
- Default:
- ""
The name of the field to filter by.
-
logic
- Type:
- enumeration
- Default:
- AND
Whether to use boolean AND or OR if there are multiple fields to filter by.
Members
- AND
- Type:string
- All conditions must be true for a record to be shown.
- OR
- Type:string
- Just one condition must be true for a record to be shown.
-
searchValue
- Type:
- string
- Default:
- ""
The value to use for this field's filter (if applicable).
-
text
- Type:
- string
- Default:
- ""
The text to display in the filter preset.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", presets : [ { text: "Sales", filteredFields: [ { fieldName: "Title", searchValue: "Sales Representative", condition: "equals" } ] } ] } ] }); //Get var presets = $(".selector").igListViewFiltering("option", "presets");
-
searchBarAllFieldsCondition
- Type:
- enumeration
- Default:
- contains
The condition to use when switching keyword search back to all fields. Should be a string filter.
Members
- equals
- Type:string
- doesNotEqual
- Type:string
- startsWith
- Type:string
- contains
- Type:string
- doesNotContain
- Type:string
- endsWith
- Type:string
-
searchBarCondition
- Type:
- enumeration
- Default:
- contains
The condition to use for filtering done with the keyword search input.
Members
- empty
- Type:string
- notEmpty
- Type:string
- null
- Type:string
- notNull
- Type:string
- equals
- Type:string
- doesNotEqual
- Type:string
- startsWith
- Type:string
- contains
- Type:string
- doesNotContain
- Type:string
- endsWith
- Type:string
- greaterThan
- Type:string
- lessThan
- Type:string
- greaterThanOrEqualTo
- Type:string
- lessThanOrEqualTo
- Type:string
- true
- Type:bool
- false
- Type:bool
- on
- Type:string
- notOn
- Type:string
- before
- Type:string
- after
- Type:string
- today
- Type:string
- yesterday
- Type:string
- thisMonth
- Type:string
- lastMonth
- Type:string
- nextMonth
- Type:string
- thisYear
- Type:string
- nextYear
- Type:string
- lastYear
- Type:string
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", searchBarCondition: "contains" } ] }); //Get var searchBarCondition = $(".selector").igListViewFiltering("option", "searchBarCondition");
-
searchBarEnabled
- Type:
- bool
- Default:
- true
Whether to enable the search bar in the tray.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", searchBarEnabled: true } ] }); //Get var searchBarEnabled = $(".selector").igListViewFiltering("option", "searchBarEnabled");
-
searchBarFieldName
- Type:
- string
- Default:
- ""
The field to search through for keyword search. When empty, it will search all the fields.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", searchBarFieldName: "Name" } ] }); //Get var searchBarFieldName = $(".selector").igListViewFiltering("option", "searchBarFieldName");
-
searchBarFields
- Type:
- array
- Default:
- []
- Elements Type:
- object
A list of the fields in the source to allow end user keyword search configuration.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", searchBarFields: [ { text: "Name", fieldName: "Name", condition: "contains" } ] } ] }); //Get var searchBarFields = $(".selector").igListViewFiltering("option", "searchBarFields");
-
condition
- Type:
- enumeration
- Default:
- ""
The filtering condition to use when scoping the keyword search by this field.
Members
- empty
- Type:string
- notEmpty
- Type:string
- null
- Type:string
- notNull
- Type:string
- equals
- Type:string
- doesNotEqual
- Type:string
- startsWith
- Type:string
- contains
- Type:string
- doesNotContain
- Type:string
- endsWith
- Type:string
- greaterThan
- Type:string
- lessThan
- Type:string
- greaterThanOrEqualTo
- Type:string
- lessThanOrEqualTo
- Type:string
- true
- Type:bool
- false
- Type:bool
- on
- Type:string
- notOn
- Type:string
- before
- Type:string
- after
- Type:string
- today
- Type:string
- yesterday
- Type:string
- thisMonth
- Type:string
- lastMonth
- Type:string
- nextMonth
- Type:string
- thisYear
- Type:string
- nextYear
- Type:string
- lastYear
- Type:string
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", searchBarFields: [ { text: "Name", fieldName: "Name", condition: "contains" } ] } ] }); //Get var searchBarFields = $(".selector").igListViewFiltering("option", "searchBarFields");
-
fieldName
- Type:
- string
- Default:
- ""
The name of the field to filter by.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", searchBarFields: [ { text: "Name", fieldName: "Name", condition: "contains" } ] } ] }); //Get var searchBarFields = $(".selector").igListViewFiltering("option", "searchBarFields");
-
text
- Type:
- string
- Default:
- ""
The text to show in the scope option.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", searchBarFields: [ { text: "Name", fieldName: "Name", condition: "contains" } ] } ] }); //Get var searchBarFields = $(".selector").igListViewFiltering("option", "searchBarFields");
-
searchBarPlaceHolder
- Type:
- string
- Default:
- null
The placeholder to display in the search bar when it is empty.
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", searchBarPlaceHolder: "Search contacts..." } ] }); //Get var searchBarPlaceHolder = $(".selector").igListViewFiltering("option", "searchBarPlaceHolder");
-
type
- Type:
- enumeration
- Default:
- null
Defines local or remote filtering.
Members
- remote
- Type:string
- local
- Type:string
Code Sample
//Initialize $(".selector").igListView({ features: [ { name: "Filtering", type: "local" } ] }); //Get var filterType = $(".selector").igListViewFiltering("option", "type");
For more information on how to interact with the Ignite UI controls' events, refer to
Using Events in Ignite UI.
-
keywordChanged
- Cancellable:
- false
Event fired after the keyword in the search bar has changed.
Function takes arguments evt and ui.
Use ui.owner to get reference to igListViewFiltering.
Use ui.owner.list to get reference to igList.
Use ui.value to get new value of textbox.Code Sample
//Delegate $(document).delegate(".selector", "iglistviewfilteringkeywordchanged", function (evt, ui) { //return the new value of the search box ui.value; //return reference to igListViewFiltering ui.owner; //return reference to igList ui.owner.list; }); //Initialize $(".selector").igListView({ features: [ { name: "Filtering", keywordChanged: function(evt, ui) {...} } ] });
-
keywordChanging
- Cancellable:
- true
Event fired before the keyword in the search bar has changed.
Return false in order to cancel hanging of filter preset.
Function takes arguments evt and ui.
Use ui.owner to get reference to igListViewFiltering.
Use ui.owner.list to get reference to igList.
Use ui.value to get new value of textbox.Code Sample
//Delegate $(document).delegate(".selector", "iglistviewfilteringkeywordchanging", function (evt, ui) { //return the new value of the search box ui.value; //return reference to igListViewFiltering ui.owner; //return reference to igList ui.owner.list; }); //Initialize $(".selector").igListView({ features: [ { name: "Filtering", keywordChanging: function(evt, ui) {...} } ] });
-
presetChanged
- Cancellable:
- false
Event fired after the preset has been changed and the data rerendered.
Function takes arguments evt and ui.
Use ui.owner to get reference to igListViewFiltering.
Use ui.owner.list to get reference to igList.
Use ui.state to get new filterState.Code Sample
//Delegate $(document).delegate(".selector", "iglistviewfilteringpresetchanged", function (evt, ui) { //return the new value of filterState option ui.state; //return reference to igListViewFiltering ui.owner; //return reference to igList ui.owner.list; }); //Initialize $(".selector").igListView({ features: [ { name: "Filtering", presetChanged: function(evt, ui) {...} } ] });
-
presetChanging
- Cancellable:
- true
Event fired before sort preset is changed in the filter.
Return false in order to cancel changing of filter preset.
Function takes arguments evt and ui.
Use ui.owner to get reference to igListViewFiltering.
Use ui.owner.list to get reference to igList.
Use ui.state to get new filterState.Code Sample
//Delegate $(document).delegate(".selector", "iglistviewfilteringpresetchanging", function (evt, ui) { //return the new value of filterState option ui.state; //return reference to igListViewFiltering ui.owner; //return reference to igList ui.owner.list; }); //Initialize $(".selector").igListView({ features: [ { name: "Filtering", presetChanging: function(evt, ui) {...} } ] });
-
scopeChanged
- Cancellable:
- false
Event fired after the scope preset has been changed and the data (possibly) rerendered.
Function takes arguments evt and ui.
Use ui.owner to get reference to igListViewFiltering.
Use ui.owner.list to get reference to igList.
Use ui.scopeField to get new field name that keyword search will scope by.
Use ui.condition to get the new condition that the keyword search will filter by.Code Sample
//Delegate $(document).delegate(".selector", "iglistviewfilteringscopechanged", function (evt, ui) { //return the new field name that will scope keyword search ui.scopeField; //return the new condition that the keyword search will filter by ui.condition; //return reference to igListViewFiltering ui.owner; //return reference to igList ui.owner.list; }); //Initialize $(".selector").igListView({ features: [ { name: "Filtering", scopeChanged: function(evt, ui) {...} } ] });
-
scopeChanging
- Cancellable:
- true
Event fired before scope preset is changed in the filter.
Return false in order to cancel changing of filter preset.
Function takes arguments evt and ui.
Use ui.owner to get reference to igListViewFiltering.
Use ui.owner.list to get reference to igList.
Use ui.scopeField to get new field name that keyword search will scope by.
Use ui.condition to get/set the new condition that the keyword search will filter by.Code Sample
//Delegate $(document).delegate(".selector", "iglistviewfilteringscopechanging", function (evt, ui) { //return the new field name that will scope keyword search ui.scopeField; //return the new condition that the keyword search will filter by ui.condition; //return reference to igListViewFiltering ui.owner; //return reference to igList ui.owner.list; }); //Initialize $(".selector").igListView({ features: [ { name: "Filtering", scopeChanging: function(evt, ui) {...} } ] });
-
destroy
- .destroy( );
Destroys the igListViewFiltering feature by removing all elements in the tray.
Code Sample
$(".selector").igListViewFiltering("destroy");
-
filter
- .filter( fieldExpressions:array, trayText:string );
Filters the list with the custom list of expressions given. Should have the same properties as defined in filtered fields of presets.
- fieldExpressions
- Type:array
- An array of field expression definitions where each object is key/value pairs expecting keys "fieldName", "searchValue", "condition", and "logic" (optional).
- trayText
- Type:string
- The text to put in the search tray footer (if it exists).
Code Sample
$(".selector").igListViewFiltering("filter", [{fieldName:"CategoryName", searchValue: "Seafood", condition: "equals"}], "Filtering for category 'Seafood'");
-
ui-igelastic-block ui-iglist-button-cancel-container
- The class applied to the container that holds the keyword search text input.
-
ui-iglist-filter-key-word-area
- The classes applied to the element holding the keyword search input.
-
ui-iglist-filter-presets
- The classes applied to the element holding all of the sort preset options.
-
ui-iglist-keyword-scope-options
- The classes applied to the element holding all of the options to scope the keyword search.
-
ui-iglist-tray-footer-item ig-tray-keyword
- The classes applied to the element displaying the text in the keyword search input in the search tray footer.
-
ui-iglist-input-search-container
- The class applied to the container that holds the keyword search text input.
-
ui-igelastic-block
- The class applied to the container that holds the keyword search text input when there is a cancel button.
-
ui-iglist-search-scope-hidden
- The classes applied to the keyword search scope element when it is partially scrolled out of view horizontally.
-
ui-btn-inline ui-iglist-preset
- The classes applied to the element to select a preset in the search area.
-
ui-iglist-tray-footer-item ig-tray-filter-preset
- The classes applied to the element displaying the selected preset's text in the search tray footer.
-
ui-iglist-preset-hidden
- The classes applied to the preset element when it is partially scrolled out of view horizontally.
-
ui-iglist-preset-selected
- The classes applied to the preset element when it is selected and applied.
-
ui-iglist-tray-footer-sep
- The classes applied to the element in the search tray footer holding the bullet (between items) and the 'filtered by' label.