ui.igGrid
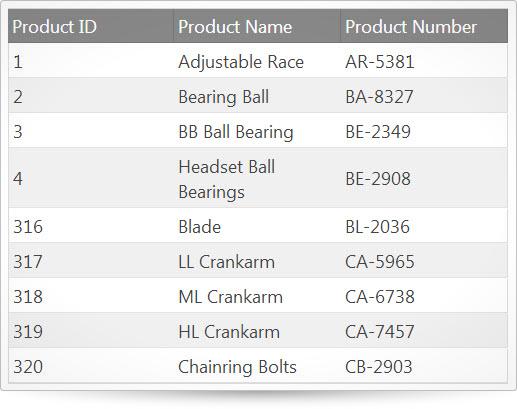
igGrid コントロールは、以下のようなユーザーインタラクションを含む jQuery グリッドです。 フィルタリング、 グループ化、 列の非表示 および サイズ変更、 ページング、 行 および セル選択、 並べ替え、 集計、 ツールチップ、および データ編集機能。
注: igGrid はスタンドアロン コントロールで、igHierarchicalGrid コントロールのベースです。igGrid に適用する API は igHierarchicalGrid コントロールにも適用します。
以下のコード スニペットは、igGrid コントロールを初期化する方法を示します。
この API を使用して作業を開始するための情報はここをクリックしてください。igGrid コントロールに必要なスクリプトとテーマを参照する方法については、Ignite UI の JavaScript リソースの使用 および Ignite UI のスタイリングとテーマ。コード サンプル
<!doctype html> <html> <head> <!-- Infragistics Combined CSS --> <link href= "css/themes/infragistics/infragistics.theme.css" rel= "stylesheet" type= "text/css" /> <link href= "css/structure/infragistics.css" rel= "stylesheet" type= "text/css" /> <!-- jQuery Core --> <script src= "js/jquery.js" type= "text/javascript" ></script> <!-- jQuery UI --> <script src= "js/jquery-ui.js" type= "text/javascript" ></script> <!-- Infragistics Combined Scripts --> <script src= "js/infragistics.core.js" type= "text/javascript" ></script> <script src= "js/infragistics.lob.js" type= "text/javascript" ></script> <script type= "text/javascript" > var products = [ { "ProductID" : 1, "Name" : "Adjustable Race" , "ProductNumber" : "AR-5381" , "Category" : { "ID" : 0, "Name" : "Food" , "Active" : true , "Date" : "\/Date(1059660800000)\/" } }, { "ProductID" : 2, "Name" : "Bearing Ball" , "ProductNumber" : "BA-8327" , "Category" : { "ID" : 1, "Name" : "Beverages" , "Active" : true , "Date" : "\/Date(1159660800000)\/" } }, { "ProductID" : 3, "Name" : "BB Ball Bearing" , "ProductNumber" : "BE-2349" , "Category" : { "ID" : 1, "Name" : "Beverages" , "Active" : true , "Date" : "\/Date(1259660800000)\/" } }, { "ProductID" : 4, "Name" : "Headset Ball Bearings" , "ProductNumber" : "BE-2908" , "Category" : { "ID" : 1, "Name" : "Beverages" , "Active" : true , "Date" : "\/Date(1359660800000)\/" } }, { "ProductID" : 316, "Name" : "Blade" , "ProductNumber" : "BL-2036" , "Category" : { "ID" : 1, "Name" : "Beverages" , "Active" : false , "Date" : "\/Date(1459660800000)\/" } }, { "ProductID" : 317, "Name" : "LL Crankarm" , "ProductNumber" : "CA-5965" , "Category" : { "ID" : 1, "Name" : "Beverages" , "Active" : false , "Date" : "\/Date(1559660800000)\/" } }, { "ProductID" : 318, "Name" : "ML Crankarm" , "ProductNumber" : "CA-6738" , "Category" : { "ID" : 1, "Name" : "Beverages" , "Active" : false , "Date" : "\/Date(1659660800000)\/" } }, { "ProductID" : 319, "Name" : "HL Crankarm" , "ProductNumber" : "CA-7457" , "Category" : { "ID" : 2, "Name" : "Electronics" , "Active" : false , "Date" : "\/Date(1759660800000)\/" } }, { "ProductID" : 320, "Name" : "Chainring Bolts" , "ProductNumber" : "CB-2903" , "Category" : { "ID" : 2, "Name" : "Electronics" , "Active" : false , "Date" : "\/Date(1859660800000)\/" } } ]; $( function () { $( "#grid" ).igGrid({ columns: [ { headerText: "Product ID" , key: "ProductID" , dataType: "number" }, { headerText: "Product Name" , key: "Name" , dataType: "string" }, { headerText: "Product Number" , key: "ProductNumber" , dataType: "string" } ], width: "500px" , dataSource: products }); }); </script> </head> <body> <table id= "grid" ></table> </body> </html> |
関連サンプル
関連トピック
依存関係
-
adjustVirtualHeights
- タイプ:
- bool
- デフォルト:
- false
このオプションが true に設定されている場合、グリッド行の高さは、avgRowHeight と表示バーチャル レコードに基づいて自動的に計算されます。 avgRowHeight が指定されていない場合、ランタイムで自動的に計算されます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
adjustVirtualHeights :
true
});
// Get
var
virtualHeights = $(
".selector"
).igGrid(
"option"
,
"adjustVirtualHeights"
);
-
aggregateTransactions
- タイプ:
- bool
- デフォルト:
- false
true に設定した場合、次の動作を実行します:
新しい行が追加された後に削除された場合、トランザクションはログに追加されません。
新しい行を追加し、編集した後に削除された場合、トランザクションはログに追加されません。
行または特定のセルに複数の編集を実行すると、単一のトランザクションになります。
注: このオプションは、 autoCommit を false に設定した場合のみに適用されます。コード サンプル
//Initialize
$(
".selector"
).igGrid({
aggregateTransactions :
true
});
//Get
var
aggregateTransactions = $(
".selector"
).igGrid(
"option"
,
"aggregateTransactions"
);
-
alternateRowStyles
- タイプ:
- bool
- デフォルト:
- true
交互行スタイル (奇数行と偶数行で異なるスタイルになる) のレンダリングを有効または無効にします。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
alternateRowStyles :
true
;
});
//Get
var
rowAltStyles = $(
".selector"
).igGrid(
"option"
,
"alternateRowStyles"
);
//Set
$(
".selector"
).igGrid(
"option"
,
"alternateRowStyles"
,
true
);
-
autoAdjustHeight
- タイプ:
- bool
- デフォルト:
- true
autoAdjustHeight を false に設定した場合、height はスクロール コンテナーのみに設定されます。ページング フッター、フィルター行、ヘッダーなどのすべての他の UI 要素は高さを追加します。グリッドの合計の高さはこの値より大きくなります。スクロール コンテナー (コンテンツ領域) の高さは動的に計算されません。このオプションを false に設定するとサイズの大きなデータ セット (1000 行を一度に描画、virtualizationは無効) の初期描画パフォーマンスが向上します。これは offsetHeight などの DOM プロパティにアクセスするときにリフローがブラウザーで行われないためです。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoAdjustHeight :
false
});
// Get
var
adjustHeight = $(
".selector"
).igGrid(
"option"
,
"autoAdjustHeight"
);
-
autoCommit
- タイプ:
- bool
- デフォルト:
- false
行またはセルを編集するとトランザクションをクライアント データソースに自動的にコミットします。その場合も、トランザクションをサーバー側のデータソースにコミットするために、saveChanges 呼び出しを実行する必要があります。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autoCommit :
true
});
//Get
var
commit = $(
".selector"
).igGrid(
"option"
,
"autoCommit"
);
-
autofitLastColumn
- タイプ:
- bool
- デフォルト:
- true
true およびすべての列の幅を指定し、合計幅がグリッド幅より小さい場合、最後の列幅がグリッドにフィットするよう自動的に調整されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autofitLastColumn :
true
});
//Get
var
autoFit = $(
".selector"
).igGrid(
"option"
,
"autofitLastColumn"
);
-
autoFormat
- タイプ:
- enumeration
- デフォルト:
- date
数値列、日付列、および時間列のセルの自動書式設定テキストを取得または設定します。数値および日付の書式パターンおよびルールは $.ig.regional.defaults オブジェクトで定義されます。 列形式の詳細です。
メンバー
- date
- タイプ:string
- 日付列のみ書式設定します。
- time
- タイプ:string
- 時間列のみ書式設定します。
- number
- タイプ:string
- 数値列のみ書式設定します。
- dateandnumber
- タイプ:string
- 日付列および数値列を書式設定します。
- true
- タイプ:bool
- 日付列および数値列を書式設定します。
- false
- タイプ:bool
- 自動書式設定を無効にします。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autoFormat :
"number"
});
//Get
var
autoFormat = $(
".selector"
).igGrid(
"option"
,
"autoFormat"
);
-
autoGenerateColumns
- タイプ:
- bool
- デフォルト:
- true
columns コレクションが定義されておらず、autoGenerateColumns が true に設定された場合、columns は dataRendering イベントが発生される前にデータソースから暗示されます。暗示された columns コレクションは dataRendering で変更できます。autoGenerateColumns が明示的に設定されておらず、columns に少なくとも 1 つの列が定義されている場合、autoGenerateColumns は自動的に false に設定されます。
autoGenerateColumns が true で列が自動生成列に定義された列がある場合、明示的に定義した後に描画されます。
自動生成列に幅が定義されないため、defaultColumnWidth の設定も検討してください。コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns :
false
});
// Get
var
autoCols = $(
".selector"
).igGrid(
"option"
,
"autoGenerateColumns"
);
-
avgColumnWidth
- タイプ:
- enumeration
- デフォルト:
- null
fixed モードでcolumnVirtualizationに使用されます。これは列幅の平均値 (ピクセル単位) です。
メンバー
- string
- タイプ:string
- 平均列幅はピクセル (25px) で設定できます。
- number
- タイプ:number
- 平均列幅は数値 (25) として設定できます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
avgColumnWidth :
"100px"
});
// Get
var
width = $(
".selector"
).igGrid(
"option"
,
"avgColumnWidth"
);
-
avgRowHeight
- タイプ:
- enumeration
- デフォルト:
- 25
rowVirtualization - fixed モードで使用されます。これは、エンド ユーザーがスクロールするときに描画する行の数を計算するために使用されるピクセル単位 (デフォルト) の平均値です。また、すべての行の高さが自動的にこの値に設定されます。
メンバー
- string
- タイプ:string
- 行の平均の高さはピクセル (25px) で設定できます。
- number
- タイプ:number
- 行の平均幅は数値 (25) として設定できます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
avgRowHeight :
"35px"
});
// Get
var
height = $(
".selector"
).igGrid(
"option"
,
"avgRowHeight"
);
-
caption
- タイプ:
- string
- デフォルト:
- null
グリッド ヘッダーの上に表示されるキャプション テキスト。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
caption :
"Sales Data"
});
//Get
var
caption = $(
".selector"
).igGrid(
"option"
,
"caption"
);
//Set
$(
".selector"
).igGrid(
"option"
,
"caption"
,
"Sales Data"
);
-
columns
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
列オブジェクトの配列。列配列の構成の詳細については、列とレイアウト トピックを参照してください。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
},
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
},
{ headerText:
"ProductNumber"
, key:
"ProductNumber"
, dataType:
"string"
},
{ headerText:
"Color"
, key:
"Color"
, dataType:
"string"
},
{ headerText:
"StandardCost"
, key:
"StandardCost"
, dataType:
"number"
},
]
});
// Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
// Set
var
newColumn = { headerText:
"New Column"
, key:
"NewCol"
};
cols.push(newColumn);
$(
".selector"
).igGrid(
"option"
,
"columns"
, cols);
-
colSpan
- タイプ:
- number
- デフォルト:
- 1
複数行レイアウト構成でセルの colSpan を指定します。colSpan 0 はサポートされていないため、グリッドにより 1 に変更されます。詳細については、複数行レイアウトを参照してください。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
},
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, colSpan: 2 },
{ headerText:
"ProductNumber"
, key:
"ProductNumber"
, dataType:
"string"
},
{ headerText:
"Color"
, key:
"Color"
, dataType:
"string"
},
{ headerText:
"StandardCost"
, key:
"StandardCost"
, dataType:
"number"
, colSpan: 2 },
]
});
//Get
var
colSpan = $(
".selector"
).igGrid(
"option"
,
"columns"
)[0].colSpan;
-
columnCssClass
- タイプ:
- string
- デフォルト:
- null
この列のデータ セルに適用される CSS クラスのスペースで分割されたリスト。このクラスは、テンプレートに完全な <td> 定義を含む列 template が定義されている列がある場合、適用されません。
コード サンプル
<style>
.colStyle {
background-color: red;
}
</style>
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
, columnCssClass:
"colStyle"
},
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
},
{ headerText:
"ProductNumber"
, key:
"ProductNumber"
, dataType:
"string"
},
{ headerText:
"Color"
, key:
"Color"
, dataType:
"string"
},
{ headerText:
"StandardCost"
, key:
"StandardCost"
, dataType:
"number"
}
]
});
//Get
var
colCssClass = $(
".selector"
).igGrid(
"option"
,
"columns"
)[0].columnCssClass;
-
columnIndex
- タイプ:
- number
- デフォルト:
- null
複数行レイアウト構成でセルの列インデックスを指定します。複数行レイアウト モードを有効にするには、すべての列にこのプロパティを設定する必要があります。 詳細については、複数行レイアウト機能を参照してください。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
, rowIndex: 0, columnIndex: 0 },
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, rowIndex: 0, columnIndex: 1, colSpan: 3 },
{ headerText:
"ProductNumber"
, key:
"ProductNumber"
, dataType:
"string"
, rowIndex: 1, columnIndex: 0 },
{ headerText:
"Color"
, key:
"Color"
, dataType:
"string"
, rowIndex: 1, columnIndex: 1 },
{ headerText:
"StandardCost"
, key:
"StandardCost"
, dataType:
"number"
, rowIndex: 1, columnIndex: 2, colSpan: 2 },
]
});
//Get
var
colIndex = $(
".selector"
).igGrid(
"option"
,
"columns"
)[0].columnIndex;
-
dataType
- タイプ:
- enumeration
- デフォルト:
- string
列セル値のデータ型。string、number、bool、date、time、または object。
メンバー
- string
- タイプ:string
- 列のデータが string 型の場合に使用されます。
- number
- タイプ:string
- 列のデータが number 型の場合に使用されます。
- boolean
- タイプ:string
- 列のデータが boolean 型の場合に使用されます。
- date
- タイプ:string
- 列のデータが date 型の場合に使用されます。
- time
- タイプ:string
- 列のデータは日付型ですが、時間部分のみが必要な場合に使用されます。
- object
- タイプ:string
- 列のデータが object 型の場合に使用されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
},
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
},
{ headerText:
"Production Date"
, key:
"ProductionDate"
, dataType:
"date"
},
]
});
//Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
productIdDataType = cols[0].dataType;
-
dateDisplayType
- タイプ:
- enumeration
- デフォルト:
- local
グリッドのこの列で日付が表示される方法を決定します。
メンバー
- local
- タイプ:string
- この列の日付がクライアントのローカル タイムゾーンで描画されます。
- utc
- タイプ:string
- この列の日付が UTC 表記で描画されます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
columns: [
{ headerText:
"Departure Date"
, key:
"DepartureDate"
, dataType:
"date"
, dateDisplayType:
"local"
},
{ headerText:
"Arrival Date"
, key:
"ArrivalDate"
, dataType:
"date"
, dateDisplayType:
"utc"
}
]
});
// Get
var
cols = $(
'.selector'
).igGrid(
'option'
,
'columns'
);
var
departureDateDisplayType = cols[0].dateDisplayType;
-
format
- タイプ:
- string
- デフォルト:
- null
列のセルの書式タイプを取得または設定します。デフォルト値は null です。書式指定子の詳細については、日付、数値、および文字列の書式設定 を参照してください。
dataType が "date" の場合、次の書式がサポートされます。"date", "dateLong", "dateTime", "time", "timeLong", "MM/dd/yyyy", "MMM-d, yy, h:mm:ss tt", "dddd d MMM" など。
dataType が "time" の場合、次の書式がサポートされます。"date", "dateLong", "dateTime", "time", "timeLong", "MMM-d, yy, h:mm:ss tt" など。
dataType が "number" の場合、次の書式がサポートされます。 "number", "currency", "percent", "int", "double", "0.00", "#.0####", "0", "#.#######" など。
"double" の値は "number" と同様ですが、小数点以下の桁数に制限はありません。
数値および日付の書式パターンおよびルールは $.ig.regional.defaults オブジェクトで定義されます。
dataType が "string" または設定されていない場合、可能性のある書式は "{0}" フラグの代用で描画されます。たとえば、書式が Name: {0} に設定されていて、セル値が Bob の場合、値は Name: Bob として表示されます。
値が checkbox に設定される場合、グリッドの renderCheckboxes オプションを無視し、チェックボックスが常に使用されます。列の dataType オプションが bool に設定される場合のみに影響します。コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
, format:
"number"
},
{ headerText:
"Production Date"
, key:
"ProductionDate"
, dataType:
"date"
, format:
"ddd, MMM-d-yy HH:mm"
},
]
});
// Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
productIdFormat = cols[0].format;
-
formatter
- タイプ:
- enumeration
- デフォルト:
- null
セル値を書式設定するために使用される関数 (文字列または関数) への参照。関数は値を取得して、新しく書式設定された値を返します。 詳細については、列の書式設定を参照してください。
メンバー
- string
- タイプ:string
- セル値を書式設定するために使用される関数の名前。
- function
- タイプ:function
- セル値を書式設定するために使用される関数。関数は値を取得して、新しく書式設定された値を返します。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"In production"
, key:
"InProduction"
, formatter:
function
(val, record) {
return
(val === 1)?
"Yes"
:
"No"
;} }
]
});
// Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
inProductionFormatter = cols[0].formatter;
-
formula
- タイプ:
- enumeration
- デフォルト:
- null
同じ行の他のセル値に基づいて現在のセルの値を計算するJavaScript関数への参照または名前。unbound columns で使用されます。
メンバー
- string
- タイプ:string
- JavaScript 関数の名前。
- function
- タイプ:function
- JavaScript 関数への参照。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Total"
, key:
"TotalUC"
, unbound:
true
, formula:
function
(data, grid)
{
return
data[
"UnitPrice"
] * data[
"UnitsInStock"
];
}
}
]
});
// Get
var
totalUC = $(
'.selector'
).igGrid(
'getUnboundColumnByKey'
,
'TotalUC'
);
var
totalFormula = totalUC.formula;
-
group
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
子列定義の配列。列がプロパティ グループを持つ場合、グリッドは複数列ヘッダーを含みます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
columns: [
{ headerText:
"Product Data"
, key:
"ProductData"
, group: [
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
},
{ headerText:
"Product Number"
, key:
"ProductNumber"
, dataType:
"string"
}
]}
],
features:[
{
name:
"MultiColumnHeaders"
}
],
width:
"500px"
});
// Get
// getMultiColumnHeaders method returns multicolumn headers array. if there aren't multicolumn headers returns undefined.
var
columns = $(
".selector"
).igGridMultiColumnHeaders(
"getMultiColumnHeaders"
);
var
group = columns[0].group;
-
groupOptions
- タイプ:
- object
- デフォルト:
- {}
縮小可能な列 groups の構成に使用されるオプション。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
columns: [
{
headerText:
"Company Information"
,
group: [
{
headerText:
"Company Name"
, key:
"CompanyName"
, dataType:
"string"
, width:
"150px"
,
groupOptions: {
hidden:
"parentcollapsed"
}
},
{
headerText:
"Contact Name"
, key:
"ContactName"
, dataType:
"string"
, width:
"150px"
,
groupOptions: {
hidden:
"parentcollapsed"
}
},
{ headerText:
"Contact Title"
, key:
"ContactTitle"
, dataType:
"string"
, width:
"150px"
}
],
groupOptions: {
expanded:
false
,
allowGroupCollapsing:
true
},
key:
"companyInfo"
}
],
features: [
{
name:
"MultiColumnHeaders"
}
],
width:
"500px"
});
//Get
var
columns = $(
".selector"
).igGridMultiColumnHeaders(
"getMultiColumnHeaders"
);
var
groupOptions = columns[0].groupOptions;
-
allowGroupCollapsing
- タイプ:
- bool
- デフォルト:
- false
列ヘッダーに展開インジケーターを表示するかどうかを設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
columns: [
{
headerText:
"Company Information"
,
group: [
{
headerText:
"Company Name"
, key:
"CompanyName"
, dataType:
"string"
, width:
"150px"
,
groupOptions: {
hidden:
"parentcollapsed"
}
},
{
headerText:
"Contact Name"
, key:
"ContactName"
, dataType:
"string"
, width:
"150px"
,
groupOptions: {
hidden:
"parentcollapsed"
}
},
{ headerText:
"Contact Title"
, key:
"ContactTitle"
, dataType:
"string"
, width:
"150px"
}
],
groupOptions: {
expanded:
false
,
allowGroupCollapsing:
true
},
key:
"companyInfo"
}
],
features: [
{
name:
"MultiColumnHeaders"
}
],
width:
"500px"
});
//Get
var
columns = $(
".selector"
).igGridMultiColumnHeaders(
"getMultiColumnHeaders"
);
var
groupOptions = columns[0].groupOptions;
var
allowGroupCollapsing = groupOptions.allowGroupCollapsing;
-
expanded
- タイプ:
- bool
- デフォルト:
- true
グループが展開または縮小されるかどうかを設定します。allowGroupCollapsing が true に設定された場合のみ適用します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
columns: [
{
headerText:
"Company Information"
,
group: [
{
headerText:
"Company Name"
, key:
"CompanyName"
, dataType:
"string"
, width:
"150px"
,
groupOptions: {
hidden:
"parentcollapsed"
}
},
{
headerText:
"Contact Name"
, key:
"ContactName"
, dataType:
"string"
, width:
"150px"
,
groupOptions: {
hidden:
"parentcollapsed"
}
},
{ headerText:
"Contact Title"
, key:
"ContactTitle"
, dataType:
"string"
, width:
"150px"
}
],
groupOptions: {
expanded:
false
,
allowGroupCollapsing:
true
},
key:
"companyInfo"
}
],
features: [
{
name:
"MultiColumnHeaders"
}
],
width:
"500px"
});
//Get
var
columns = $(
".selector"
).igGridMultiColumnHeaders(
"getMultiColumnHeaders"
);
var
groupOptions = columns[0].groupOptions;
var
expanded = groupOptions.expanded;
-
hidden
- タイプ:
- enumeration
- デフォルト:
- never
グループが非表示にされる条件を設定します。allowGroupCollapsing が true に設定された場合のみ適用します。
メンバー
- never
- タイプ:string
- グループを非表示にしません。
- always
- タイプ:string
- グループを常に非表示にします。
- parentcollapsed
- タイプ:string
- 親グループが縮小されるときにグループを非表示にします。
- parentexpanded
- タイプ:string
- 親グループが展開されるときにグループを非表示にします。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
columns: [
{
headerText:
"Company Information"
,
group: [
{
headerText:
"Company Name"
, key:
"CompanyName"
, dataType:
"string"
, width:
"150px"
,
groupOptions: {
hidden:
"parentcollapsed"
}
},
{
headerText:
"Contact Name"
, key:
"ContactName"
, dataType:
"string"
, width:
"150px"
,
groupOptions: {
hidden:
"parentcollapsed"
}
},
{ headerText:
"Contact Title"
, key:
"ContactTitle"
, dataType:
"string"
, width:
"150px"
}
],
groupOptions: {
expanded:
false
,
allowGroupCollapsing:
true
},
key:
"companyInfo"
}
],
features: [
{
name:
"MultiColumnHeaders"
}
],
width:
"500px"
});
//Get
var
columns = $(
".selector"
).igGridMultiColumnHeaders(
"getMultiColumnHeaders"
);
var
groupOptions = columns[0].group[0].groupOptions;
var
hidden = groupOptions.hidden;
-
headerCssClass
- タイプ:
- string
- デフォルト:
- null
この列のヘッダー セルに適用される CSS クラスのスペースで分割されたリスト。
コード サンプル
<style>
.headerCss {
background-color: red;
}
</style>
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, headerCssClass:
"headerCss"
}
]
});
// Get
var
headerCss = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
headerCssClass = cols[0].headerCssClass;
-
headerText
- タイプ:
- string
- デフォルト:
- null
指定した列のヘッダー テキスト。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
},
]
});
// Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
productNameHeaderText = cols[0].headerText;
-
hidden
- タイプ:
- bool
- デフォルト:
- false
列の初期表示状態 (表示/非表示)。非表示機能が有効ではなくても列を非表示にすることができますが、表示にするための UI はありません。非表示機能のオプションで列を非表示として定義することもでき、これらの定義が優先されます。
コード サンプル
// Initialize
$(
'.selector'
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, hidden:
true
},
]
});
// Get
var
cols = $(
'.selector'
).igGrid(
'option'
,
'columns'
);
var
productNameHidden = cols[0].hidden;
-
key
- タイプ:
- string
- デフォルト:
- null
列がバインドされるデータ ソースのプロパティ。また、columnByKey などの API メソッドを使用して列を識別し、特定の列を検索するために使用されます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
},
]
});
// Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
productNameKey = cols[0].key;
-
mapper
- タイプ:
- enumeration
- デフォルト:
- null
このオプションは、dataType オブジェクトのある列のみ適用されます。データレコードからの複雑なデータ抽出に使用される関数への参照、または関数の名前。戻り値はこの列に関連付けられたすべてのデータ操作に使用され、セル値として表示されます。詳細については、列マッパーを設定する方法の例を参照してください。
メンバー
- string
- タイプ:string
- マッパー関数の名前。
- function
- タイプ:function
- マッパー関数への参照。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
},
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
},
{ headerText:
"Product Number"
, key:
"ProductNumber"
, dataType:
"string"
},
{
headerText:
"Category"
,
key:
"Category"
,
dataType:
"object"
,
mapper:
function
(record) {
return
record.Category.Name;
}
}
]
});
//Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
categoryMapper = cols[3].mapper;
-
navigationIndex
- タイプ:
- number
- デフォルト:
- null
複数行レイアウトを持つグリッドでセルが編集モードにある場合、TAB シーケンスでセルのナビゲーション インデックスを指定します。それ以外の場合に結果がありません。 グリッドの複数行レイアウトを参照してください。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
, rowIndex: 0, columnIndex: 0 },
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, rowIndex: 0, columnIndex: 1, colSpan: 3, navigationIndex: 1 },
{ headerText:
"ProductNumber"
, key:
"ProductNumber"
, dataType:
"string"
, rowIndex: 1, columnIndex: 0, navigationIndex: 2 },
{ headerText:
"Color"
, key:
"Color"
, dataType:
"string"
, rowIndex: 1, columnIndex: 1, navigationIndex: 4 },
{ headerText:
"StandardCost"
, key:
"StandardCost"
, dataType:
"number"
, rowIndex: 1, columnIndex: 2, colSpan: 2, navigationIndex: 3 }
]
});
//Get
var
colIndex = $(
".selector"
).igGrid(
"option"
,
"columns"
)[1].navigationIndex;
-
rowIndex
- タイプ:
- number
- デフォルト:
- null
複数行レイアウト構成でセルの行インデックスを指定します。複数行レイアウト モードを有効にするには、すべての列にこのプロパティを設定する必要があります。 詳細については、グリッドの複数行レイアウトを参照してください。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
, rowIndex: 0, columnIndex: 0 },
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, rowIndex: 0, columnIndex: 1, colSpan: 3 },
{ headerText:
"ProductNumber"
, key:
"ProductNumber"
, dataType:
"string"
, rowIndex: 1, columnIndex: 0 },
{ headerText:
"Color"
, key:
"Color"
, dataType:
"string"
, rowIndex: 1, columnIndex: 1 },
{ headerText:
"StandardCost"
, key:
"StandardCost"
, dataType:
"number"
, rowIndex: 1, columnIndex: 2, colSpan: 2 },
]
});
//Get
var
rowIndex = $(
".selector"
).igGrid(
"option"
,
"columns"
)[0].rowIndex;
-
rowspan
非推奨- タイプ:
- number
- デフォルト:
- 0
このオプションは 2016 年 6 月のサービス リリースより非推奨となります。
複数列ヘッダー セルのスパンを変更します。代わりに rowSpan オプションを使用します。 -
rowSpan
- タイプ:
- number
- デフォルト:
- 1
複数行レイアウト構成でセルの rowSpan を指定します。rowSpan 0 はサポートされていないため、グリッドにより 1 に変更されます。 詳細については、複数行レイアウト機能を参照してください。複数行レイアウトが使用されていないが複数列ヘッダーを設定する場合、このオプションはヘッダー セルのスパンを変更するために使用されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
, rowIndex: 0, columnIndex: 0, rowSpan: 2 },
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, rowIndex: 0, columnIndex: 1, colSpan: 3 },
{ headerText:
"ProductNumber"
, key:
"ProductNumber"
, dataType:
"string"
, rowIndex: 1, columnIndex: 1 },
{ headerText:
"Color"
, key:
"Color"
, dataType:
"string"
, rowIndex: 1, columnIndex: 2 },
{ headerText:
"StandardCost"
, key:
"StandardCost"
, dataType:
"number"
, rowIndex: 1, columnIndex: 3 },
]
});
//Get
var
rowSpan = $(
".selector"
).igGrid(
"option"
,
"columns"
)[0].rowSpan;
-
template
- タイプ:
- string
- デフォルト:
- null
個々の列のテンプレートを設定します。テンプレートのコンテンツは、テーブル セル内に入る HTML マークアップまたはテーブル セル マークアップ全体でなければなりません。 基本的な列テンプレート作成の例を参照してください。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, template:
"Product: ${Name}"
},
]
});
// Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
template = cols[0].template;
-
unbound
- タイプ:
- bool
- デフォルト:
- false
列データーがデータ ソースにバインドされるかどうかを設定します。true の場合、この列のセルはデータソースにバインドされません。 この列のデータは、数式、unboundValues、または setUnboundValues API メソッドで生成されます。 非バインド列機能の概要を参照してください。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{
headerText:
"Promotion Expired Date"
,
key:
"PromExpDate"
,
dataType:
"date"
,
unbound:
true
,
unboundValues: [
new
Date(
'4/24/2016'
),
new
Date(
'8/24/2016'
),
new
Date(
'6/24/2016'
),
new
Date(
'10/24/2016'
),
new
Date(
'11/24/2016'
)
]
}
]
});
// Get
var
unboundColumn = $(
".selector"
).igGrid(
'getUnboundColumnByKey'
,
'PromExpDate'
);
var
isUnbound = unboundColumn.unbound;
-
unboundValues
- タイプ:
- array
- デフォルト:
- null
- 要素タイプ:
列が非バインドの場合、列セルの初期化で生成される値の配列。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{
headerText:
"Promotion Expired Date"
,
key:
"PromotionExpDate"
,
dataType:
"date"
,
unbound:
true
,
unboundValues: [
new
Date(
'4/24/2016'
),
new
Date(
'8/24/2016'
),
new
Date(
'6/24/2016'
),
new
Date(
'10/24/2016'
),
new
Date(
'11/24/2016'
)
]
}
]
});
-
width
- タイプ:
- enumeration
- デフォルト:
- null
列幅 (ピクセルまたはパーセント)。列セルのコンテンツ (ヘッダー テキストを含む) に基づいた幅自動サイズ調整の場合、'*' として設定もできます。 幅を定義せずに defaultColumnWidth を設定した場合、すべての列に設定されます。
メンバー
- string
- タイプ:string
- 列幅は、ピクセル (px) およびパーセンテージ (%) で設定します。セルとヘッダーのコンテンツに基づいて自動サイズ調整するには、'*' を設定します。
- number
- タイプ:number
- 列幅は数値として設定できます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product Name"
, key:
"Name"
, width:
"100px"
, dataType:
"string"
},
]
});
// Get
var
cols = $(
".selector"
).igGrid(
"option"
,
"columns"
);
var
productNameWidth = cols[0].width;
-
columnVirtualization
- タイプ:
- bool
- デフォルト:
- false
列のみの可視化を有効にします。列の仮想化は、固定行仮想化でのみで有効です。columnVirtualization を true に設定するとvirtualization が自動的に true に設定され virtualizationMode は fixed に設定されます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
columnVirtualization :
true
});
// Get
var
columnVirtualization = $(
".selector"
).igGrid(
"option"
,
"columnVirtualization"
);
-
dataSource
- タイプ:
- enumeration
- デフォルト:
- null
$.ig.DataSource が受け取る任意の有効なデータソース、または $.ig.DataSource 自体のインスタンスにすることができます。
メンバー
- array
- タイプ:array
- 配列としての dataSource。
- object
- タイプ:object
- オブジェクトとしての dataSource。
- string
- タイプ:string
- 文字列としての dataSource。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
dataSource : ds
});
//Get
var
ds = $(
".selector"
).igGrid(
"option"
,
"dataSource"
);
Note:
This code will
return
the original data source used when initializing the grid.
To get the igDataSource instance use the following syntax:
var
igDs = $(
".selector"
).data(
"igGrid"
).dataSource;
//Set
$(
".selector"
).igGrid(
"option"
,
"dataSource"
, ds);
-
dataSourceType
- タイプ:
- string
- デフォルト:
- null
データ ソースのタイプ ("json" など) を明示的に設定します。$.ig.DataSource タイプを参照してください。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
dataSourceType :
"xml"
});
//Get
var
dsType = $(
".selector"
).igGrid(
"option"
,
"dataSourceType"
);
-
dataSourceUrl
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource を使用してデータが取得されるデータソースとしてリモート URL を指定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
dataSourceUrl : urlString
});
//Get
var
urlString = $(
".selector"
).igGrid(
"option"
,
"dataSourceUrl"
);
-
defaultColumnWidth
- タイプ:
- enumeration
- デフォルト:
- null
列幅が設定されていないすべての列に設定されるデフォルトの列幅。列セルのコンテンツ (ヘッダー テキストを含む) に基づいた幅自動サイズ調整の場合、'*' として設定もできます。
メンバー
- string
- タイプ:string
- デフォルトの列幅は、ピクセル ("100px") で設定、またはセルとヘッダーのコンテンツに基づいて自動サイズ調整するには、'*' を設定します。
- number
- タイプ:number
- デフォルトの列幅は数値 (100) としてピクセルで設定できます。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
defaultColumnWidth : 100
});
// Get
var
width = $(
".selector"
).igGrid(
"option"
,
"defaultColumnWidth"
);
-
enableHoverStyles
- タイプ:
- bool
- デフォルト:
- true
マウスがレコードの上にある場合にホバー スタイルの描画を有効/無効にします。テンプレートされたコンテンツにホバー スタイル設定を適用しないテンプレートなどの場合に便利です。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
enableHoverStyles :
false
});
//Get
var
enableHoverStyles = $(
".selector"
).igGrid(
"option"
,
"enableHoverStyles"
);
-
enableResizeContainerCheck
- タイプ:
- bool
- デフォルト:
- true
幅および/または高さをパーセント (%) で設定した際にグリッドの親 DOM コンテナーがサイズ変更されるときのグリッドのディメンションの調整を有効または無効にします。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
enableResizeContainerCheck :
false
});
//Get
var
enableResizeContainerCheck = $(
".selector"
).igGrid(
"option"
,
"enableResizeContainerCheck"
);
//Set
$(
".selector"
).igGrid(
"option"
,
"enableResizeContainerCheck"
,
false
);
-
enableUTCDates
- タイプ:
- bool
- デフォルト:
- false
ローカル時間およびゾーン値の代わりにクライアント側の日付を UTC ISO 8061 文字列としてのシリアル化を有効/無効にします。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
enableUTCDates :
true
});
//Get
var
enableUTCDates = $(
".selector"
).igGrid(
"option"
,
"enableUTCDates"
);
//Set
$(
".selector"
).igGrid(
"option"
,
"enableUTCDates"
,
true
);
-
featureChooserIconDisplay
- タイプ:
- enumeration
- デフォルト:
- desktopOnly
機能セレクター アイコンをヘッダー セルに表示する方法を構成します (ギアアイコンの表示など)。
メンバー
- none
- タイプ:string
- 機能セレクター アイコンを常に非表示します。機能セレクターは列ヘッダーをクリック/タップするときに表示されます。
- desktopOnly
- タイプ:string
- デスクトップでアイコンを常に表示しますが、タッチ デバイスで非表示します。
- always
- タイプ:string
- 環境に関係なく常に表示します。機能セレクターは、ギア アイコンまたは列ヘッダーをタップすると表示します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
featureChooserIconDisplay :
"always"
});
//Get
var
featureChooserIconDisplay = $(
".selector"
).igGrid(
"option"
,
"featureChooserIconDisplay"
);
-
features
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
並べ替え、ページングなどのグリッド機能の定義のリスト。各機能はそれぞれ別のオプションを使用します。オプションについては機能ごとに文書化されています。 詳細については、 igGrid 機能の詳細を参照してください。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Paging"
,
type:
"local"
,
pageSize: 13
},
{
name:
"Sorting"
,
type:
"local"
},
{
name:
"Selection"
},
{
name:
"Filtering"
,
type:
"local"
,
filterDropDownItemIcons:
false
,
filterDropDownWidth: 200
}
]
});
//Get
var
features = $(
".selector"
).igGrid(
"option"
,
"features"
);
-
name
- タイプ:
- string
- デフォルト:
- null
有効にする機能の名前。
-
fixedFooters
- タイプ:
- bool
- デフォルト:
- true
このオプションが true の場合はフッターが固定されるため、グリッド データのみがスクロール可能になります。もし 仮想化が有効な場合、fixedFooters は、どの値が設定されていても常に true として機能します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
fixedFooters :
false
});
//Get
var
fixed = $(
".selector"
).igGrid(
"option"
,
"fixedFooters"
);
//Set
$(
".selector"
).igGrid(
"option"
,
"fixedFooters"
,
false
);
-
fixedHeaders
- タイプ:
- bool
- デフォルト:
- true
このオプションが True の場合、ヘッダーが固定されるので、グリッド データのみがスクロール可能です。もし 仮想化 が有効な場合、fixedHeaders は、どの値が設定されていても常に True として機能します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
fixedHeaders :
false
});
//Get
var
fixedHeaders = $(
".selector"
).igGrid(
"option"
,
"fixedHeaders"
);
-
height
- タイプ:
- enumeration
- デフォルト:
- null
これは、データ行のあるスクロール コンテナー、ヘッダー、フッター、フィルター行など (もしあれば)、すべての UI 要素を含むグリッドの合計の高さです。 詳細については、igGrid の高さの設定を参照してください。
メンバー
- string
- タイプ:string
- ウィジェットの高さはピクセル (px) およびパーセント (%) で設定できます。
- number
- タイプ:number
- ウィジェットの高さは数値として設定できます。
- null
- タイプ:object
- データに合わせて垂直に拡張します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
height :
"850px"
});
// Get
var
height = $(
".selector"
).igGrid(
"option"
,
"height"
);
// Set
$(
".selector"
).igGrid(
"option"
,
"height"
,
"800px"
);
-
jsonpRequest
- タイプ:
- bool
- デフォルト:
- false
dataSource がリモート URL の場合、リモート データ ソースのタイプを JSONP 設定するかどうかを定義します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
jsonpRequest :
true
});
//Get
var
jsonpRequest = $(
".selector"
).igGrid(
"option"
,
"jsonpRequest"
);
-
language
継承- タイプ:
- string
- デフォルト:
- "en"
ウィジェットのロケール言語設定を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
language:
"ja"
});
// Get
var
language = $(
".selector"
).igGrid(
"option"
,
"language"
);
// Set
$(
".selector"
).igGrid(
"option"
,
"language"
,
"ja"
);
-
locale
- タイプ:
- object
- デフォルト:
- null
ウィジェットのロケール設定を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
locale: { optionChangeNotSupported:
"Changing {optionName} is not supported"
}
});
// Get
var
locale = $(
".selector"
).igGrid(
"option"
,
"locale"
);
// Set
$(
".selector"
).igGrid(
"option"
,
"locale"
, { optionChangeNotSupported:
"Changing {optionName} is not supported"
});
-
localSchemaTransform
- タイプ:
- bool
- デフォルト:
- true
-
mergeUnboundColumns
- タイプ:
- bool
- デフォルト:
- false
データ ソースがリモートの場合、データソース内に非バインド列を結合します。True の場合、非バインド列はランタイムでサーバーのデータソースに結合されます。注: データ ソースは新しいデータで拡張されるため、データが大きい場合はパフォーマンスに影響がある場合があります。mergeUnboundColumns が false の場合、非バインド データが送信されてクライアントで結合されます。この要素は igGrid MVC ヘルパー によって使用されます。
詳細については非バインド列をリモートに生成 (igGrid)を参照してください。コード サンプル
//Initialize
$(
".selector"
).igGrid({
mergeUnboundColumns :
true
,
dataSource : REMOTE_URL
});
//Get
var
mergeUC = $(
".selector"
).igGrid(
"option"
,
"mergeUnboundColumns"
);
-
primaryKey
- タイプ:
- string
- デフォルト:
- null
データ レコードの一意識別子を含む列キー。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
primaryKey :
"CustomerID"
});
//Get
var
key = $(
".selector"
).igGrid(
"option"
,
"primaryKey"
);
-
regional
継承- タイプ:
- enumeration
- デフォルト:
- defaults
ウィジェットの領域設定を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
regional:
"ja"
});
// Get
var
regional = $(
".selector"
).igGrid(
"option"
,
"regional"
);
// Set
$(
".selector"
).igGrid(
"option"
,
"regional"
,
"ja"
);
-
renderCheckboxes
- タイプ:
- bool
- デフォルト:
- false
列の dataType が bool の場合、チェックボックスを描画してチェックボックス エディターを使用する機能を取得または設定します。 チェックボックスは、列テンプレートのある列のブール値で描画されません。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
renderCheckboxes :
true
});
//Get
var
renderCheckboxes = $(
".selector"
).igGrid(
"option"
,
"renderCheckboxes"
);
//Set
$(
".selector"
).igGrid(
"option"
,
"renderCheckboxes"
,
true
);
-
requestType
- タイプ:
- string
- デフォルト:
- "GET"
リモートデータソースに要求を送信するために使用される HTTP 動詞を指定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
requestType :
"POST"
});
//Get
var
requestType = $(
".selector"
).igGrid(
"option"
,
"requestType"
);
-
responseContentType
- タイプ:
- string
- デフォルト:
- "application/json; charset=utf-8"
応答のコンテンツ タイプ。http://api.jquery.com/jQuery.ajax/で contentType セクションを参照してください。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
responseContentType :
"application/json; charset=utf-8"
});
//Get
var
responseContentType = $(
".selector"
).igGrid(
"option"
,
"responseContentType"
);
-
responseDataKey
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource responseDataKeyを参照してください。これは、応答がラップした場合にデータ レコードが保持される応答内のプロパティです。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
responseDataKey :
"records"
});
//Get
var
responseKey = $(
".selector"
).igGrid(
"option"
,
"responseDataKey"
);
-
responseTotalRecCountKey
非推奨- タイプ:
- string
- デフォルト:
- null
このオプションは非推奨です。 $.ig.DataSource responseTotalRecCountKeyを参照してください。サーバー上のレコード総数を指定する応答内のプロパティです。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
responseTotalRecCountKey :
"totalRecords"
});
//Get
var
recordsCount = $(
".selector"
).igGrid(
"option"
,
"responseTotalRecCountKey"
);
-
restSettings
- タイプ:
- object
- デフォルト:
- {}
REST コンプライアント更新ルーチンに関連する設定。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
create: {
url:
"/api/customers/"
,
batch:
true
},
update: {
url:
"/api/customers/"
,
batch:
true
},
remove: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
-
contentSerializer
- タイプ:
- function
- デフォルト:
- null
サーバーに送信するコンテンツをシリアル化するカスタム関数を指定します。単一のオブジェクトまたはオブジェクトの配列を受け入れ文字列を返します。指定されていない場合、JSON.stringify() が使用されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
contentSerializer :
"customSerializeFunction"
;
// the name of the function, it can be also a reference to a function
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
contentSerializerSetting = restSettings.contentSerializer;
-
contentType
- タイプ:
- string
- デフォルト:
- "application/json; charset=utf-8"
要求のコンテンツ タイプを指定します。http://api.jquery.com/jQuery.ajax/ => contentType。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
contentType :
"application/json; charset=utf-8"
;
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
contentTypeSetting = restSettings.contentType;
-
create
- タイプ:
- object
- デフォルト:
- {}
作成要求の設定。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
create: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
createRestSettings = restSettings.create;
-
batch
- タイプ:
- bool
- デフォルト:
- false
作成要求がバッチで送信されるどうかを指定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
create: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
batch = restSettings.create.batch;
-
template
- タイプ:
- string
- デフォルト:
- null
リモート URL テンプレートを指定します。リソース ID の代わりに ${id} を使用します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
update: {
template:
"/api/customers/${id}"
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
template = restSettings.update.template;
-
url
- タイプ:
- string
- デフォルト:
- null
作成要求を送信するリモート URL を指定します。バッチおよび非バッチの両方で使用されますが、テンプレートも設定されている場合、この URL はバッチ要求にのみ使用されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
update: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
url = restSettings.update.url;
-
encodeRemoveInRequestUri
- タイプ:
- bool
- デフォルト:
- true
削除されたリソースの id が要求 URI を介して送信されるかどうかを指定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
encodeRemoveInRequestUri:
true
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
encodeRemoveInRequestUri = restSettings.encodeRemoveInRequestUri;
-
remove
- タイプ:
- object
- デフォルト:
- {}
削除要求の設定。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
remove: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
removeRestSettings = restSettings.remove;
-
batch
- タイプ:
- bool
- デフォルト:
- false
更新要求がバッチで送信されるかどうかを指定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
remove: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
batch = restSettings.remove.batch;
-
template
- タイプ:
- string
- デフォルト:
- null
リモート URL テンプレートを指定します。リソース ID の代わりに ${id} を使用します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
remove: {
template:
"/api/customers/${id}"
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
template = restSettings.remove.template;
-
url
- タイプ:
- string
- デフォルト:
- null
削除要求を送信するリモート URL を指定します。バッチおよび非バッチのために使用されますが、テンプレートも設定される場合、この URL はバッチ要求のみに使用されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
remove: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
url = restSettings.remove.url;
-
update
- タイプ:
- object
- デフォルト:
- {}
更新要求の設定。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
update: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
updateRestSettings = restSettings.update;
-
batch
- タイプ:
- bool
- デフォルト:
- false
更新要求がバッチで送信されるかどうかを指定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
update: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
batch = restSettings.update.batch;
-
template
- タイプ:
- string
- デフォルト:
- null
リモート URL テンプレートを指定します。リソース ID の代わりに ${id} を使用します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
update: {
template:
"/api/customers/${id}"
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
template = restSettings.update.template;
-
url
- タイプ:
- string
- デフォルト:
- null
削除要求を送信するリモート URL を指定します。バッチおよび非バッチの両方で使用されますが、テンプレートも設定されている場合、この URL はバッチ要求にのみ使用されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
restSettings : {
update: {
url:
"/api/customers/"
,
batch:
true
}
}
});
//Get
var
restSettings = $(
".selector"
).igGrid(
"option"
,
"restSettings"
);
var
url = restSettings.update.url;
-
rowVirtualization
- タイプ:
- bool
- デフォルト:
- false
行のみの仮想化を有効にします。 igGrid の行仮想化の設定を参照してください。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
rowVirtualization :
true
});
// Get
var
virtualization = $(
".selector"
).igGrid(
"option"
,
"rowVirtualization"
);
-
scrollSettings
- タイプ:
- object
- デフォルト:
- {}
コンテンツのスクロールに関連する設定。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
scrollTop: 100,
smoothing:
true
,
smoothingStep: 2.5
}
});
//Get
var
scrollSettings = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
);
//Set
var
newSettings = {
scrollTop: 100,
smoothing:
true
,
smoothingStep: 2.5
}
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, newSettings);
-
inertiaDuration
- タイプ:
- number
- デフォルト:
- 1
タッチ デバイスで慣性が持続する時間の修飾子を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
inertiaDuration: 0.75
}
});
//Get
var
value = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
).inertiaDuration;
//Set
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, { inertiaDuration: 0.75 });
-
inertiaStep
- タイプ:
- number
- デフォルト:
- 1
タッチ デバイスで慣性がスクロールする量の修飾子を取得または設定します。注: 値を 0 に設定するとタッチ動作を無効にします。値を -1 に設定すると反転します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
inertiaStep: 2
}
});
//Get
var
value = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
).inertiaStep;
//Set
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, { inertiaStep: 2 });
-
scrollLeft
- タイプ:
- number
- デフォルト:
- 0
現在の水平位置を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
scrollLeft: 200
}
});
//Get
var
value = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
).scrollLeft;
//Set
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, { scrollLeft: 300 });
-
scrollTop
- タイプ:
- number
- デフォルト:
- 0
現在の垂直位置を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
scrollTop: 500
}
});
//Get
var
value = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
).scrollTop;
//Set
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, { scrollTop: 600 });
-
smoothing
- タイプ:
- bool
- デフォルト:
- false
マウス ホイールを使用する場合、小さい慣性を持つスムーズ スクロールを使用するかどうかを取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
smoothing:
true
}
});
//Get
var
value = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
).smoothing;
//Set
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, { smoothing:
true
});
-
smoothingDuration
- タイプ:
- number
- デフォルト:
- 1
マウス ホイールを一度使用した場合にスクロールのアニメーションが持続する時間の修飾子を取得または設定します。これはスムーズ スクロール動作でのみ使用されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
smoothingDuration: 1.25
}
});
//Get
var
value = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
).smoothingDuration;
//Set
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, { smoothingDuration: 1.25 });
-
smoothingStep
- タイプ:
- number
- デフォルト:
- 1
マウス ホイールを一度使用した場合にスクロールするピクセルを決定する修飾子を取得または設定します。スムーズ スクロール動作のみで使用されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
smoothingStep: 2.5
}
});
//Get
var
value = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
).smoothingStep;
//Set
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, { smoothingStep: 2.5 });
-
wheelStep
- タイプ:
- number
- デフォルト:
- 50
マウス ホイールを使用する場合、デフォルトのスクロール動作のステップを取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
scrollSettings: {
wheelStep: 100
}
});
//Get
var
value = $(
".selector"
).igGrid(
"option"
,
"scrollSettings"
).wheelStep;
//Set
$(
".selector"
).igGrid(
"option"
,
"scrollSettings"
, { wheelStep: 50 });
-
serializeTransactionLog
- タイプ:
- bool
- デフォルト:
- true
True の場合、トランザクション ログがデータ ソースによってリモート データの要求に常に送られます。また、ログに値がある場合、GET ではなく POST が実行されることを意味します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
serializeTransactionLog :
false
});
//Get
var
log = $(
".selector"
).igGrid(
"option"
,
"serializeTransactionLog"
);
-
showFooter
- タイプ:
- bool
- デフォルト:
- true
グリッドのフッターの可視性を制御します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
showFooter :
false
});
//Get
var
showFooter = $(
".selector"
).igGrid(
"option"
,
"showFooter"
);
//Set
$(
".selector"
).igGrid(
"option"
,
"showFooter"
,
false
);
-
showHeader
- タイプ:
- bool
- デフォルト:
- true
グリッドのヘッダーの可視性を制御します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
showHeader :
false
});
//Get
var
showHeader = $(
".selector"
).igGrid(
"option"
,
"showHeader"
);
//Set
$(
".selector"
).igGrid(
"option"
,
"showHeader"
,
false
);
-
tabIndex
- タイプ:
- number
- デフォルト:
- 0
すべてのフォーカス可能な要素に設定される初期 tabIndex 属性。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
tabIndex : 3
});
//Get
var
tabIndex = $(
".selector"
).igGrid(
"option"
,
"tabIndex"
);
-
templatingEngine
- タイプ:
- enumeration
- デフォルト:
- infragistics
グリッド列 templateを描画するために使用されるテンプレート エンジン。igTemplating と jsRender 以外のテンプレート エンジンの使用方法は、ここを参照してください。
メンバー
- infragistics
- タイプ:string
- グリッドは Infragistics テンプレート エンジンを使用して列 templateおよび UI の特定部分を描画します。
- jsRender
- タイプ:string
- グリッドは jsRender を使用して、列template および特定の UI パーツを使用して描画します。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
templatingEngine :
"jsRender"
});
// Get
var
templatingEngine = $(
".selector"
).igGrid(
"option"
,
"templatingEngine"
);
-
updateUrl
- タイプ:
- string
- デフォルト:
- null
更新要求先の URL。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
updateUrl :
"http://mydomain.com/UpdateCustomer"
});
//Get
var
updateUrl = $(
".selector"
).igGrid(
"option"
,
"updateUrl"
);
-
virtualization
- タイプ:
- bool
- デフォルト:
- false
列および行の仮想化を同時に有効または無効にします。仮想化が描画のパフォーマンスを向上します。有効な場合、描画される行 (DOM 要素) の数は定数で、グリッドの表示可能なビューポートに関連します。エンド ユーザーがスクロールすると、新しいデータを描画するために DOM 要素を動的に更新します。igGrid を使用する際のパフォーマンス ガイドを参照してください。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
virtualization :
true
});
// Get
var
virtualization = $(
".selector"
).igGrid(
"option"
,
"virtualization"
);
-
virtualizationMode
- タイプ:
- enumeration
- デフォルト:
- fixed
行仮想化モードを決定します。
メンバー
- fixed
- タイプ:string
- 表示される行/列のみグリッドに描画されます。スクロールすると、同じ行/列がデータ ソースからの新しいデータで更新されます。固定仮想化のみ列仮想化と同時に動作します。固定仮想化がサポートされないグリッド機能 (サイズ変更、グループ化、レスポンシブ) があります。
- continuous
- タイプ:string
- 定義済みの行数がグリッドに描画されます。スクロールすると、連続仮想化は行の他のグループを読み込み、現在の行を破棄します。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
virtualizationMode :
"continuous"
});
// Get
var
virtualizationMode = $(
".selector"
).igGrid(
"option"
,
"virtualizationMode"
);
// Set
$(
".selector"
).igGrid(
"option"
,
"virtualizationMode"
,
"continuous"
);
-
virtualizationMouseWheelStep
- タイプ:
- number
- デフォルト:
- null
仮想化が有効で、仮想グリッド領域上でマウス ホイールのスクロールを実行するときにグリッドの移動するピクセル数。 Null の場合、avgRowHeight と等しいです。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
virtualizationMouseWheelStep : 50
});
// Get
var
virtualizationStep = $(
".selector"
).igGrid(
"option"
,
"virtualizationMouseWheelStep"
);
// Set
$(
".selector"
).igGrid(
"option"
,
"virtualizationMouseWheelStep"
, 50);
-
width
- タイプ:
- enumeration
- デフォルト:
- null
グリッドの幅をピクセル単位またはパーセントで定義します。 詳細については、igGrid の幅設定を参照してください。
メンバー
- string
- タイプ:string
- ウィジェット幅をピクセル (px) またはパーセンテージ (%) に設定できます。値の例: "800px"、"800" (デフォルト単位はピクセル)、"100%"。
- number
- タイプ:number
- ウィジェット幅はピクセルの数値で設定できます。値の例: 800、700。
- null
- タイプ:object
- 列幅の合計に合うように拡張します。
コード サンプル
// Initialize
$(
".selector"
).igGrid({
width :
"800px"
});
// Get
var
width = $(
".selector"
).igGrid(
"option"
,
"width"
);
// Set
$(
".selector"
).igGrid(
"option"
,
"width"
,
"850px"
);
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
captionRendered
- キャンセル可能:
- false
キャプションが描画された後に発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
グリッドへの参照を取得します。
-
captionContainerタイプ: domElement
キャプション コンテナーへの参照を取得します。
-
コード サンプル
//Initialize
$(
".selector"
).igGrid({
captionRendered:
function
(evt, ui) {
//return reference to igGrid
ui.owner;
//return caption container DOM element
ui.captionContainer;
}
});
-
captionRendering
- キャンセル可能:
- true
キャプションが描画を開始する前に発生するイベント。
キャプションの描画をキャンセルするには、false を返します。コード サンプル
//Initialize
$(
".selector"
).igGrid({
captionRendering:
function
(evt, ui) {
//return reference to igGrid
ui.owner;
}
});
-
cellClick
- キャンセル可能:
- false
セルをクリックしたときに発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridcellclick"
,
".selector"
,
function
(evt, ui) {
//return cell html element in the DOM
ui.cellElement;
//return row index
ui.rowIndex;
//return row key
ui.rowKey;
//return col index of the DOM element
ui.colIndex;
//return col key
ui.colKey;
//return reference to igGrid
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
cellClick:
function
(evt, ui) {...}
});
-
cellRightClick
- キャンセル可能:
- false
セルを右クリックしたときに発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
cellElementタイプ: domElement
セルの DOM 要素への参照を取得します。
-
rowIndexタイプ: Number
行のインデックスを取得します。
-
rowKeyタイプ: Object
行キーを取得します。
-
colIndexタイプ: Number
DOM 要素の列インデックスを取得します。
-
colKeyタイプ: String
列キーを取得します。
-
rowタイプ: domElement
行 DOM 要素への参照を取得します。
-
ownerタイプ: Object
グリッドへの参照を取得します。
-
コード サンプル
$(document).on(
"iggridcellrightclick"
,
".selector"
,
function
(evt, ui) {
//return cell html element in the DOM
ui.cellElement;
//return row index
ui.rowIndex;
//return row key
ui.rowKey;
//return col index of the DOM element
ui.colIndex;
//return col key
ui.colKey;
//return reference to igGrid
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
cellRightClick:
function
(evt, ui) {...}
});
-
columnsCollectionModified
- キャンセル可能:
- false
列コレクションを修正した後に発生するイベント (列を非表示にするなど)。
コード サンプル
//Bind after initialization
$(document).on(
"iggridcolumnscollectionmodified"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
columnsCollectionModified:
function
(evt, ui) {...}
});
-
created
- キャンセル可能:
- false
グリッドを作成して最初の構造が描画されるときに発生されます。データ ソースがリモートの場合、データがない可能性があります。
コード サンプル
//Bind before the igGrid initialization code
$(document).on(
"igcontrolcreated"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
});
-
dataBinding
- キャンセル可能:
- true
データ バインドの実行前に発生するイベント。データ バインディングをキャンセルするには、false を返します。
コード サンプル
//Bind after initialization
$(document).on(
"iggriddatabinding"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
dataBinding:
function
(evt, ui) {...}
});
-
dataBound
- キャンセル可能:
- false
データ バインドが完了した後に発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggriddatabound"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
dataBound:
function
(evt, ui) {...}
});
-
dataRendered
- キャンセル可能:
- false
グリッド テーブル本体内のすべてのデータ レコードが描画された後に発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggriddatarendered"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
dataRendered:
function
(evt, ui) {...}
});
-
dataRendering
- キャンセル可能:
- true
データ レコードを保持する TBODY が描画を開始する前に発生するイベント。データ レコードの再バインディングをキャンセルするには、false を返します。
コード サンプル
//Bind after initialization
$(document).on(
"iggriddatarendering"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
//return grid's table body DOM element
ui.tbody;
});
//Initialize
$(
".selector"
).igGrid({
dataRendering:
function
(evt, ui) {...}
});
-
destroyed
- キャンセル可能:
- false
グリッドが破棄されたときに発生します。
コード サンプル
//Bind after initialization
$(document).on(
"igcontroldestroyed"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
});
-
footerRendered
- キャンセル可能:
- false
フッターが描画された後に発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
グリッドへの参照を取得します。
-
tableタイプ: domElement
フッター テーブルの DOM 要素への参照を取得します。
-
コード サンプル
//Initialize
$(
".selector"
).igGrid({
footerRendered:
function
(evt, ui) {
//return reference to igGrid
ui.owner;
//return footer html element in the DOM
ui.table;
}
});
-
footerRendering
- キャンセル可能:
- true
フッターが描画を開始する前に発生するイベント。フッターの描画をキャンセルするには、false を返します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
footerRendering:
function
(evt, ui) {
//return reference to igGrid
ui.owner;
}
});
-
headerCellRendered
- キャンセル可能:
- false
グリッド ヘッダー内の各 TH が描画された後に発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
グリッドへの参照を取得します。
-
columnKeyタイプ: String
列のキーを取得します。
-
thタイプ: domElement
ヘッダー セルの DOM 要素への参照を取得します。
-
コード サンプル
//Initialize
$(
".selector"
).igGrid({
headerCellRendered:
function
(evt, ui) {
//return reference to igGrid
ui.owner;
//return column key
ui.columnKey;
//return header cell DOM element
ui.th;
}
});
-
headerRendered
- キャンセル可能:
- false
ヘッダーが描画された後に発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
グリッドへの参照を取得します。
-
tableタイプ: domElement
ヘッダー テーブルの DOM 要素への参照を取得します。
-
コード サンプル
//Initialize
$(
".selector"
).igGrid({
headerRendered:
function
(evt, ui) {
//return reference to igGrid
ui.owner;
//return headers table DOM element
ui.table;
}
});
-
headerRendering
- キャンセル可能:
- true
ヘッダーの描画を開始する前に発生するイベント。ヘッダーの描画をキャンセルするには、false を返します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
headerRendering:
function
(evt, ui) {
//return reference to igGrid
ui.owner;
}
});
-
rendered
- キャンセル可能:
- false
グリッド ウィジェット全体 (ヘッダー、フッターなどを含む) が描画された後に発生するイベント。このイベントは、グリッドが初期化しているときのみに発生します。グリッドがデータに再バインドされる場合に発生しません。たとえば、dataBind() API メソッドを呼び出すか、ページングが有効な場合にページ サイズを変更する場合など。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
rendered:
function
(evt, ui) {
//return reference to igGrid
ui.owner;
}
});
-
rendering
- キャンセル可能:
- true
グリッドが (すべてのコンテンツの) 描画を開始する前に発生するイベント。このイベントは、グリッドが初期化しているときのみに発生します。グリッドがデータに再バインドされる場合に発生しません。たとえば、dataBind() API メソッドを呼び出すか、ページングが有効な場合にページ サイズを変更する場合など。グリッドの描画をキャンセルするには、false を返します。
コード サンプル
//Bind after initialization
$(document).on(
"iggridrendering"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
rendering:
function
(evt, ui) {...}
});
-
requestError
- キャンセル可能:
- false
グリッドがデータバインド、ページング、並べ替えなどのリモート操作を行っているときに、要求にエラーがある場合イベントが発生します。
コード サンプル
//Bind after initialization
$(document).on(
"iggridrequesterror"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner
//return error message coming from the server
ui.message
});
//Initialize
$(
".selector"
).igGrid({
requestError:
function
(evt, ui) {...}
});
-
rowsRendered
- キャンセル可能:
- false
データ行が描画された後に発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
グリッドへの参照を取得します。
-
tbodyタイプ: domElement
グリッドのテーブル本体への参照を取得します。
-
コード サンプル
//Bind after initialization
$(document).on(
"iggridrowsrendered"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
//return grid's table body DOM element
ui.tbody;
});
//Initialize
$(
".selector"
).igGrid({
rowsRendered:
function
(evt, ui) {...}
});
-
rowsRendering
- キャンセル可能:
- true
実際のデータ行 (TRs) が描画される前に発生するイベント。行の描画をキャンセルするには、false を返します。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
グリッドへの参照を取得します。
-
tbodyタイプ: domElement
グリッドのテーブル本体への参照を取得します。
-
コード サンプル
//Bind after initialization
$(document).on(
"iggridrowsrendering"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
//return grid's table body
ui.tbody;
});
//Initialize
$(
".selector"
).igGrid({
rowsRendering:
function
(evt, ui) {...}
});
-
schemaGenerated
- キャンセル可能:
- false
修正する必要がある場合に、$.ig.DataSource スキーマが生成された後に発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridschemagenerated"
,
".selector"
,
function
(evt, ui) {
//return reference to igGrid
ui.owner;
//return data source schema
ui.schema;
//return reference to data source
ui.dataSource;
});
//Initialize
$(
".selector"
).igGrid({
schemaGenerated:
function
(evt, ui) {...}
});
-
activeCell
- .igGrid( "activeCell" );
- 返却型:
- object
{element: , row: , index: , rowIndex: , columnKey: } の書式設定がある現在アクティブな (フォーカスを持つ) セルがある場合、それを返します。
コード サンプル
var
cell = $(
".selector"
).igGrid(
"activeCell"
);
-
activeRow
- .igGrid( "activeRow" );
- 返却型:
- object
{element: , index: } の書式設定がある現在アクティブな (フォーカスを持つ) 行がある場合、それを返します。
コード サンプル
var
row = $(
".selector"
).igGrid(
"activeRow"
);
-
allFixedRows
- .igGrid( "allFixedRows" );
- 返却型:
- array
ColumnFixing シナリオで、固定列が 1 つ以上の場合、即座に必要なものだけでなく、すべての固定データ行を再帰的に返します。
コード サンプル
var
fixedRows = $(
".selector"
).igGrid(
"allFixedRows"
);
-
allRows
- .igGrid( "allRows" );
- 返却型:
- array
即座に必要なものだけではなく、すべてのデータ行を再帰的に返します。固定列が 1 つ以上の場合、固定されていない表の行のみを返します。
コード サンプル
var
rows = $(
".selector"
).igGrid(
"allRows"
);
-
allTransactions
- .igGrid( "allTransactions" );
- 返却型:
- array
保留されているまたはデータ ソースにコミットされたすべてのトランザクション オブジェクトのリストを返します。
それは、this.dataSource.allTransactions() のラッパーです。コード サンプル
var
transactions = $(
".selector"
).igGrid(
"allTransactions"
);
-
autoSizeColumns
- .igGrid( "autoSizeColumns" );
コンテンツを自動調整してスペースに合わせて表示するために width プロパティが「*」に設定されている列を自動サイズ変更します。自動サイズ変更は表示列のみに適用されます。
コード サンプル
$(
".selector"
).igGrid(
"autoSizeColumns"
);
-
calculateAutoFitColumnWidth
- .igGrid( "calculateAutoFitColumnWidth", columnIndex:number );
- 返却型:
- number
- 返却型の説明:
- 計算された適切な幅 (指定した columnIndex に表示可能な列がない場合は -1)。
コンテンツがデータの幅に合わせてサイズ変更するために列の幅を計算します。コンテンツが縮小、クリップされません。
- columnIndex
- タイプ:number
- 表示可能な列インデックス。
コード サンプル
var
autoFitColumnWidth = $(
".selector"
).igGrid(
"calculateAutoFitColumnWidth"
, 3);
-
cellAt
- .igGrid( "cellAt", x:number, y:number, isFixed:bool );
- 返却型:
- domelement
- 返却型の説明:
- (x, y) にあるセル。
指定した位置にあるセル TD 要素を返します。
- x
- タイプ:number
- 列インデックス。
- y
- タイプ:number
- 行インデックス。
- isFixed
- タイプ:bool
- オプション パラメーター - True の場合、固定テーブルの指定した位置にセル TD を取得します。
コード サンプル
var
cell = $(
".selector"
).igGrid(
"cellAt"
, 3, 4);
-
cellById
- .igGrid( "cellById", rowId:object, columnKey:string );
- 返却型:
- domelement
- 返却型の説明:
- (rowId, columnKey) のセル。
行 ID および列キーによってセルの TD 要素を返します。
- rowId
- タイプ:object
- 行の ID。
- columnKey
- タイプ:string
- 列キー。
コード サンプル
var
cell = $(
".selector"
).igGrid(
"cellById"
, 3,
"ProductName"
);
-
changeGlobalLanguage
継承- .igGrid( "changeGlobalLanguage" );
ウィジェットの言語をグローバルの言語に変更します。グローバルの言語は $.ig.util.language の値です。
コード サンプル
$(
".selector"
).igGrid(
"changeGlobalLanguage"
);
-
changeGlobalRegional
継承- .igGrid( "changeGlobalRegional" );
ウィジェットの地域設定をグローバルの地域設定に変更します。グローバルの地域設定は $.ig.util.regional にあります。
コード サンプル
$(
".selector"
).igGrid(
"changeGlobalRegional"
);
-
changeLocale
継承- .igGrid( "changeLocale", $container:object );
指定したコンテナーに含まれるすべてのロケールを options.language で指定した言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。- $container
- タイプ:object
- オプションのパラメーター: 設定しない場合、ウィジェットの要素を $container として使用します。
コード サンプル
$(
".selector"
).igGrid(
"changeLocale"
);
-
changeRegional
- .igGrid( "changeRegional" );
ウィジェット要素の地域設定を options.regional に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。regional オプションのセッターを使用してください。コード サンプル
$(
".selector"
).igGrid(
"changeRegional"
);
-
children
- .igGrid( "children" );
- 返却型:
- array
現在のグリッドのすべての子要素を再帰的に取得します。
コード サンプル
var
allChildren = $(
".selector"
).igGrid(
"children"
);
-
childrenWidgets
- .igGrid( "childrenWidgets" );
- 返却型:
- array
現在のグリッドのすべての子を再帰的に取得します。
コード サンプル
var
allChildrenWidgets = $(
".selector"
).igGrid(
"childrenWidgets"
);
-
columnByKey
- .igGrid( "columnByKey", key:string );
- 返却型:
- object
- 返却型の説明:
- 列定義。
指定された列キーによって列オブジェクトを返します。
- key
- タイプ:string
- 列キー。
コード サンプル
var
col = $(
".selector"
).igGrid(
"columnByKey"
,
"ProductName"
);
-
columnByText
- .igGrid( "columnByText", text:string );
- 返却型:
- object
- 返却型の説明:
- 列定義。
指定されたヘッダー テキストによって列オブジェクトを返します。複数の一致がある場合、最初の一致を返します。
- text
- タイプ:string
- 列ヘッダー テキスト。
コード サンプル
var
col = $(
".selector"
).igGrid(
"columnByText"
,
"Product Name"
);
-
commit
- .igGrid( "commit", [rowId:object] );
クライアント データ ソースに対するすべての保留トランザクションをコミットします。すぐに更新されるため、UI でコミットするトランザクションがありません。実際の UI をロールバックするには、dataBind() への呼び出しが必要です。
- rowId
- タイプ:object
- オプション
- 指定した場合、指定したインデックスまたはキーに対応するトランザクションのみをコミットします。
コード サンプル
$(
".selector"
).igGrid(
"commit"
);
-
container
- .igGrid( "container" );
- 返却型:
- domelement
グリッド ウィジェットの最上位コンテナーである DIV を返します。
コード サンプル
var
containerDiv = $(
".selector"
).igGrid(
"container"
);
-
dataBind
- .igGrid( "dataBind" );
これによって、グリッドが (ローカルまたはリモートの) データ ソースにデータ バインドされ、すべてのデータが再描画されます。
コード サンプル
$(
".selector"
).igGrid(
"dataBind"
);
-
dataSourceObject
- .igGrid( "dataSourceObject", dataSource:object );
データ ソースがローカルの JSON データ配列を指し、実行時にそれをリセットする必要がある場合、オプション (options.dataSource) ではなくこの API メンバーを使ってそれを実行する必要があります。
- dataSource
- タイプ:object
- 新しいデータ ソース オブジェクト。
コード サンプル
$(
".selector"
).igGrid(
"dataSourceObject"
, jsonData);
-
destroy
- .igGrid( "destroy", notToCallDestroy:object );
破棄は jQuery UI ウィジェット API の一部であり、以下を行います。
1.追加されたカスタム CSS クラスを削除します。
2.スクロール div または他のコンテナーなどのラッピング要素をアンラップします。
3.バインドされたイベントを解除します。- notToCallDestroy
- タイプ:object
コード サンプル
$(
".selector"
).igGrid(
"destroy"
);
-
findRecordByKey
- .igGrid( "findRecordByKey", key:object );
- 返却型:
- object
- 返却型の説明:
- 検索したレコードを指定する JavaScript オブジェクト。レコードが検索されていない場合は null。
指定されたキーによってレコードを返します (設定の中で primaryKey が設定されている必要があります)。
それは、this.dataSource.findRecordByKey(key) のラッパーです。- key
- タイプ:object
- レコードのプライマリ キー。
コード サンプル
var
record = $(
".selector"
).igGrid(
"findRecordByKey"
,
"AR-5381"
);
-
fixedBodyContainer
- .igGrid( "fixedBodyContainer" );
- 返却型:
- domelement
固定ボディ グリッドの最上位のコンテナーである DIV を返します。ColumnFixing シナリオで、固定列を含みます。
コード サンプル
var
fixedBodyContainer = $(
".selector"
).igGrid(
"fixedBodyContainer"
);
-
fixedContainer
- .igGrid( "fixedContainer" );
- 返却型:
- domelement
固定グリッドの最上位のコンテナーである DIV を返します。ColumnFixing シナリオで、固定列を含みます。
コード サンプル
var
fixedContainer = $(
".selector"
).igGrid(
"fixedContainer"
);
-
fixedFooterContainer
- .igGrid( "fixedFooterContainer" );
- 返却型:
- object
- 返却型の説明:
- 固定テーブルの jQuery 要素。
固定フッターを含むコンテナー (jQuery 要素) を返します。ColumnFixing シナリオで、固定列を含みます。
コード サンプル
var
fixedFooterContainer = $(
".selector"
).igGrid(
"fixedFooterContainer"
);
-
fixedFootersTable
- .igGrid( "fixedFootersTable" );
- 返却型:
- domelement
フッター セルを含むテーブルを返します。ColumnFixing シナリオで、固定列を含みます。
コード サンプル
var
fixedFootersTable = $(
".selector"
).igGrid(
"fixedFootersTable"
);
-
fixedHeaderContainer
- .igGrid( "fixedHeaderContainer" );
- 返却型:
- object
- 返却型の説明:
- 固定テーブルの jQuery 要素。
固定ヘッダーを含むコンテナー (jQuery 要素) を返します。ColumnFixing シナリオで、固定列を含みます。
コード サンプル
var
fixedHeaderContainer = $(
".selector"
).igGrid(
"fixedHeaderContainer"
);
-
fixedHeadersTable
- .igGrid( "fixedHeadersTable" );
- 返却型:
- domelement
固定ヘッダー セルを含むテーブルを返します。ColumnFixing シナリオで、固定列を含みます。
コード サンプル
var
fixedHeadersTable = $(
".selector"
).igGrid(
"fixedHeadersTable"
);
-
fixedRowAt
- .igGrid( "fixedRowAt", i:number );
- 返却型:
- domelement
- 返却型の説明:
- 指定されたインデックスにある行。
指定したインデックスにある固定行 (TR 要素) を返します。ColumnFixing シナリオで、1 つ以上の固定列がある場合、パフォーマンスの理由により、jQuery セレクターは使用しません。
- i
- タイプ:number
- 行インデックス。
コード サンプル
var
fixedRow = $(
".selector"
).igGrid(
"fixedRowAt"
, 10);
-
fixedRows
- .igGrid( "fixedRows" );
- 返却型:
- array
ColumnFixing シナリオで、1 つ以上の固定列がある場合、グリッド内のデータを保持しているすべての固定 TR 要素のリストを返します。
コード サンプル
var
fixedRows = $(
".selector"
).igGrid(
"fixedRows"
);
-
fixedTable
- .igGrid( "fixedTable" );
- 返却型:
- object
- 返却型の説明:
- 固定テーブルの jQuery 要素。
固定テーブルを返します。ColumnFixing シナリオで、固定列を含みます。固定列がない場合、グリッド テーブルを返します。
コード サンプル
var
fixedTable = $(
".selector"
).igGrid(
"fixedTable"
);
-
fixingDirection
- .igGrid( "fixingDirection" );
- 返却型:
- enumeration
- 返却型の説明:
- Can return 'left|right'.
現在の固定方向を返します。注: ColumnFixing 機能が有効な場合にも使用してください。
コード サンプル
var
fixingDirection = $(
".selector"
).igGrid(
"fixingDirection"
);
-
footersTable
- .igGrid( "footersTable" );
- 返却型:
- domelement
フッター セルを含むテーブルを返します。
コード サンプル
var
footer = $(
".selector"
).igGrid(
"footersTable"
);
-
getCellText
- .igGrid( "getCellText", rowId:object, colKey:string );
- 返却型:
- string
- 返却型の説明:
- 相対するセルのセル テキスト。
セル テキストを返します。colKey が数値の場合、列のインデックスが使用され (列幅の代わりに)、複数行レイアウト グリッドを使用する場合は適用されません。
これは、セルのコンテンツの実際のテキスト (または HTML 文字列) です。- rowId
- タイプ:object
- 行インデックスおよび行データ キー (プライマリ キー)。
- colKey
- タイプ:string
- 列キー。
コード サンプル
var
text = $(
".selector"
).igGrid(
"getCellText"
, 3,
"ProductName"
);
-
getCellValue
- .igGrid( "getCellValue", rowId:object, colKey:string );
- 返却型:
- object
- 返却型の説明:
- 相対するセル値。
行インデックスおよび列キーを使用してセル値を取得します。primaryKey が定義される場合、rowId は (インデックスではない) 行キーです。
プライマリ キーを定義しない場合、rowId は数に変換され、行インデックスとして使用されます。- rowId
- タイプ:object
- 行インデックスまたは行キー (プライマリ キー)。
- colKey
- タイプ:string
- 列キー。
コード サンプル
var
date = $(
".selector"
).igGrid(
"getCellValue"
, 3,
"ShipDate"
);
-
getColumnByTD
- .igGrid( "getColumnByTD", $td:object );
- 返却型:
- object
- 返却型の説明:
- 列オブジェクトおよびセルが属す表示インデックスを含むオブジェクト。
列オブジェクトと引数として渡された表のセル インデックスを返します。
- $td
- タイプ:object
- cell(TD) - DOM TD 要素または jQuery オブジェクト。
コード サンプル
var
columnObject = $(
".selector"
).igGrid(
"getColumnByTD"
, $(
".cellSelector"
));
-
getDetachedRecord
- .igGrid( "getDetachedRecord", t:object );
- 返却型:
- object
- 返却型の説明:
- データ ソースからのレコードのコピー。
データ ソースからデタッチされるコミットしたトランザクションを表すスタンドアロン オブジェクト (コピー) を返します。
それは、this.dataSource.getDetachedRecord(t) のラッパーです。- t
- タイプ:object
- トランザクション オブジェクト。
コード サンプル
var
ds = $(
".selector"
).igGrid(
"option"
,
"dataSource"
);
var
transactionObject = ds.addRow(123, {
Name:
"CD Player"
,
Price:
"40"
,
Rating:
"4"
},
true
);
var
record = $(
".selector"
).igGrid(
"getDetachedRecord"
, transactionObject);
-
getElementInfo
- .igGrid( "getElementInfo", elem:domelement );
- 返却型:
- object
- 返却型の説明:
- 渡した DOM 要素で以下の情報を含むオブジェクト。
渡された Dom 要素の情報を含むオブジェクトを返します。
rowId - 要素と関連付けされるレコードの id - primaryKey を設定しない場合、null になります。
rowIndex - 要素に関連付けされた行のインデックス (DOM 内)。
recordIndex - 現在の dataView でこの要素に関連付けされたデータ レコードのインデックス。
columnObject - この要素に関連付けされた列オブジェクト (要素が tr の場合、これは null になります)。- elem
- タイプ:domelement
- Dom 要素またはグリッドの TD または TR 要素になる jQuery オブジェクト。
コード サンプル
var
info = $(
".selector"
).igGrid(
"getElementInfo"
, $(
".elementSelector"
));
-
getUnboundColumnByKey
- .igGrid( "getUnboundColumnByKey", key:string );
- 返却型:
- object
- 返却型の説明:
- 列定義。
指定したキーを持つ非バインド列を返します。見つからない場合は null を返します。
- key
- タイプ:string
- 列キー。
コード サンプル
// returns the unbound column with key "Total"
var
totalUC = $(
'.selector'
).igGrid(
'getUnboundColumnByKey'
,
'Total'
);
-
getUnboundValues
- .igGrid( "getUnboundValues", key:string );
- 返却型:
- object
- 返却型の説明:
- 非バインド値。
指定された列キーの非バインド値を取得します。キーが指定されない場合、すべて非バインド値を返します。
- key
- タイプ:string
- 列キー。
コード サンプル
// returns the array of unbound values for the unbound column with key = "Total"
var
unboundValues = $(
'.selector'
).igGrid(
'getUnboundValues'
,
'Total'
);
// returns all unbound values in the igGrid
var
allUnboundValues = $(
'.selector'
).igGrid(
'getUnboundValues'
);
// returns null if BoundColumnKey is a key of a bound column
var
boundColumnValues = $(
'.selector'
).igGrid(
'getUnboundValues'
,
'BoundColumnKey'
);
-
getVisibleIndexByKey
- .igGrid( "getVisibleIndexByKey", columnKey:string, includeDataSkip:bool );
- 返却型:
- number
- 返却型の説明:
- 表示可能なインデックスを返します。列が見つからないか、列が非表示の場合、-1 を返します。
指定した列キーによって表示可能なインデックスを取得します。列が見つからないか、非表示にされる場合、-1 を返します。
注: メソッドは列グループ (複数列ヘッダー) をカウントしません。- columnKey
- タイプ:string
- columnKey。
- includeDataSkip
- タイプ:bool
- オプション パラメーター - true に設定される場合、データ以外の列 (エクスパンダー列、行セレクター列など) を計算に含みます。
コード サンプル
var
visibleIndexByKey = $(
".selector"
).igGrid(
"getVisibleIndexByKey"
,
"ProductName"
);
-
hasFixedColumns
- .igGrid( "hasFixedColumns" );
- 返却型:
- bool
グリッドに固定列が少なくとも 1 列あるかどうかを返します (行セレクター列などのデータを含まない列の場合も)。
コード サンプル
var
hasFixedColumns = $(
".selector"
).igGrid(
"hasFixedColumns"
);
-
hasFixedDataSkippedColumns
- .igGrid( "hasFixedDataSkippedColumns" );
- 返却型:
- bool
グリッドにデータ以外の固定列 (行セレクター列など) があるかどうかを返します。
コード サンプル
var
hasFixedDataSkippedColumns = $(
".selector"
).igGrid(
"hasFixedDataSkippedColumns"
);
-
hasVerticalScrollbar
- .igGrid( "hasVerticalScrollbar" );
垂直スクロールバーがあるかどうかを返します。Internet Explorer 8 または 9 などの古いバージョンにおいて、高さが設定されていない場合にスクロールバーないが行仮想化が有効な場合は垂直スクロールバーがあるというパフォーマンスの問題があります。
コード サンプル
var
hasVerticalScrollbar = $(
".selector"
).igGrid(
"hasVerticalScrollbar"
);
-
headersTable
- .igGrid( "headersTable" );
- 返却型:
- domelement
ヘッダー セルを含むテーブルを返します。
コード サンプル
var
headers = $(
".selector"
).igGrid(
"headersTable"
);
-
hideColumn
- .igGrid( "hideColumn", column:object, callback:function );
表示される列を非表示にします。列が非表示の場合、このメソッドは何もしません。
注: このメソッドは非同期です。ただちに返し、以後のコードは並行して実行されます。ランタイム エラーを発生する場合があります。エラーを回避するには、メソッドによって提供されるコールバック パラメーターに以後のコードを挿入します。- column
- タイプ:object
- 列の識別子。数字が提供される場合、列インデックスとして使用されます。文字列が提供される場合、列キーとして使用されます。
- callback
- タイプ:function
- 列を非表示にしたときに呼び出すカスタム関数を指定します (オプション)。
コード サンプル
$(
".selector"
).igGrid(
"hideColumn"
, 1);
$(
".selector"
).igGrid(
"hideColumn"
,
"ProductID"
);
-
id
- .igGrid( "id" );
- 返却型:
- string
データ レコードが描画される TABLE 要素の ID を返します。
コード サンプル
var
id = $(
".selector"
).igGrid(
"id"
);
-
immediateChildren
- .igGrid( "immediateChildren" );
- 返却型:
- array
現在のグリッドのすぐ下にある子の要素をすべて取得します。
コード サンプル
var
children = $(
".selector"
).igGrid(
"immediateChildren"
);
-
immediateChildrenWidgets
- .igGrid( "immediateChildrenWidgets" );
- 返却型:
- array
現在のグリッドのすぐ下にある子をすべて取得します。
コード サンプル
var
childrenWidgets = $(
".selector"
).igGrid(
"immediateChildrenWidgets"
);
-
isFixedColumn
- .igGrid( "isFixedColumn", colKey:object );
- 返却型:
- bool
colKey の識別子を持つ列が固定されるかどうかを返します。
- colKey
- タイプ:object
- 確認する列の識別子。キーまたは表示可能なインデックスです。
コード サンプル
var
isFixedColumn = $(
".selector"
).igGrid(
"isFixedColumn"
,
"Name"
);
-
isGroupHeader
- .igGrid( "isGroupHeader", colKey:string );
colKey によって識別されるヘッダーが複数列ヘッダーかどうかを返します。
- colKey
- タイプ:string
- 列キーの値。
コード サンプル
var
isGroupHeader = $(
".selector"
).igGrid(
"isGroupHeader"
,
"Name"
)
-
moveColumn
- .igGrid( "moveColumn", column:object, target:object, [after:bool], [inDom:bool], [callback:function] );
指定した位置にある表示列を、対象列の前または後に移動するか、対象のインデックスに移動します。
注: このメソッドは非同期です。ただちに返し、以後のコードは並行して実行されます。ランタイム エラーを発生する場合があります。エラーを回避するには、メソッドによって提供されるコールバック パラメーターに以後のコードを挿入します。- column
- タイプ:object
- 移動される列の ID。キー、複数列ヘッダーの ID、または数値書式のインデックスです。グリッドが複数列ヘッダーを含む場合、数値書式のインデックスはサポートされません。
- target
- タイプ:object
- 列に移動する列の識別子、または列に移動するインデックス。列の識別子の場合、デフォルトでその列の後に移動されます。
- after
- タイプ:bool
- オプション
- 列が対象の列の前または後に移動するかどうかを指定します。指定したターゲット列がなくて、ターゲット インデックスが使用される場合、このパラメーターは使用されません。
- inDom
- タイプ:bool
- オプション
- 列は DOM 操作またはグリッドの描画によって移動されるかどうかを指定します。
- callback
- タイプ:function
- オプション
- 列を移動したときに呼び出すカスタム関数を指定します。
コード サンプル
$(
".selector"
).igGrid(
"moveColumn"
,
"CustomerID"
,
"Address"
,
false
,
true
);
// OR
$(
".selector"
).igGrid(
"moveColumn"
,
"CustomerID"
,
"Address"
,
false
,
true
,
function
() {
// Custom code executed after the move operation finishes
});
-
pendingTransactions
- .igGrid( "pendingTransactions" );
- 返却型:
- array
データ ソースへのコミットまたはロール バックが保留されているすべてのトランザクション オブジェクトのリストを返します。
それは、this.dataSource.pendingTransactions() のラッパーです。コード サンプル
var
pendingTrans = $(
".selector"
).igGrid(
"pendingTransactions"
);
-
renderMultiColumnHeader
- .igGrid( "renderMultiColumnHeader", cols:array );
呼び出されるときに、このメソッドはグリッドの全体を再描画し、データ ソースにバインドして、cols オブジェクトを描画します。
- cols
- タイプ:array
- 列オブジェクトの配列。
コード サンプル
var
columns = [
{ headerText:
"Customer ID"
, key:
"CustomerID"
, dataType:
"string"
, width:
"100px"
},
{ headerText:
"Company Information"
,
group: [
{ headerText:
"Company Name"
, key:
"CompanyName"
, dataType:
"string"
, width:
"150px"
},
{ headerText:
"Contact Name"
, key:
"ContactName"
, dataType:
"string"
, width:
"150px"
},
{ headerText:
"Contact Title"
, key:
"ContactTitle"
, dataType:
"string"
, width:
"150px"
}
]
}
];
$(
".selector"
).igGrid(
"renderMultiColumnHeader"
, columns);
-
renderNewRow
- .igGrid( "renderNewRow", [rec:string] );
データ行オブジェクトを取得して、グリッドに新しい行 (TR) を追加します。レコードはプライマリ キーを持つ必要があります。
- rec
- タイプ:string
- オプション
- 行の識別子/キー。ない場合、グリッドの行数が使用されます。
コード サンプル
$(
".selector"
).igGrid(
"renderNewRow"
, {
ProductID: 2,
Name:
"CD Player"
,
ProductNumber:
"test"
,
Color:
"test"
,
StandardCost: 40,
});
-
resizeContainer
- .igGrid( "resizeContainer" );
グリッド コンテナーがサイズ変更されたかどうかを検出するために呼び出されます。autoAdjustHeight が true で、グリッドの高さが変更された場合、グリッドの高さはリセットされます。
コード サンプル
$(
".selector"
).igGrid(
"resizeContainer"
);
-
rollback
- .igGrid( "rollback", [rowId:object], [updateUI:bool] );
- 返却型:
- array
- 返却型の説明:
- ロールバックされたトランザクション。
トランザクション ログをクリアします (igDataSource のデリゲート)。これは UI を更新しないことに注意してください。UI を更新する場合、第 2 のパラメーター (updateUI) を true に設定すると、dataBind() への呼び出しをトリガーして、コンテンツを再描画します。
- rowId
- タイプ:object
- オプション
- 指定した場合、その行 ID を持つトランザクションのみをロールバックします。
- updateUI
- タイプ:bool
- オプション
- UI を更新するかどうか。
コード サンプル
$(
".selector"
).igGrid(
"rollback"
, 5,
true
);
-
rowAt
- .igGrid( "rowAt", i:number );
- 返却型:
- domelement
- 返却型の説明:
- 指定されたインデックスにある行。
指定したインデックスにある行 (TR 要素) を返します。パフォーマンスの理由により、jQuery セレクターは使用しません。
- i
- タイプ:number
- 行インデックス。
コード サンプル
var
row = $(
".selector"
).igGrid(
"rowAt"
, 5);
-
rowById
- .igGrid( "rowById", rowId:object, [isFixed:bool] );
- 返却型:
- domelement
- 返却型の説明:
- (rowId) の行。
行 ID によって行 TR 要素を返します。
- rowId
- タイプ:object
- 行の ID。
- isFixed
- タイプ:bool
- オプション
- 固定コンテナーに検索を指定します。
コード サンプル
var
row = $(
".selector"
).igGrid(
"rowById"
, 1);
-
rows
- .igGrid( "rows" );
- 返却型:
- array
グリッド内のデータを保持しているすべての TR 要素のリストを返します。固定列が 1 つ以上の場合、固定されていない表の行のみを返します。
コード サンプル
var
rows = $(
".selector"
).igGrid(
"rows"
);
-
saveChanges
- .igGrid( "saveChanges", success:function, error:function );
(指定される場合) updateUrl オプションへの AJAX リクエストを起動し、シリアル化されるトランザクション ログ (シリアル化される JSON 文字列) を POST 要求の部分として渡されます。
- success
- タイプ:function
- updateUrl オプションへの AJAX リクエストに成功したときに呼び出すカスタム関数を指定します (オプション)。
- error
- タイプ:function
- updateUrl オプションへの AJAX リクエストに失敗したときに呼び出すカスタム関数を指定します (オプション)。
コード サンプル
// Example 1: Save changes without callbacks
$(
".selector"
).igGrid(
"saveChanges"
);
// Example 2: Save changes with success and error callbacks
$(
".selector"
).igGrid(
"saveChanges"
,
function
(data) {
$(
"#message"
).text(
"Changes were saved successfully"
).fadeIn(3000).fadeOut(5000);
},
function
(jqXHR, textStatus, errorThrown) {
$(
"#message"
).text(
"An error occurred while saving the changes. Error details: "
+ textStatus).fadeIn(3000).fadeOut(5000);
});
-
scrollContainer
- .igGrid( "scrollContainer" );
- 返却型:
- domelement
グリッド コンテンツのスクロール コンテナーとして使用する DIV を返します。
コード サンプル
var
container = $(
".selector"
).igGrid(
"scrollContainer"
);
-
selectedCell
- .igGrid( "selectedCell" );
- 返却型:
- object
{element: , row: , index: , rowIndex: , columnKey: } の書式設定がある現在選択されているセルある場合、それを返します。
複数選択が有効な場合、null を返します。コード サンプル
var
cell = $(
".selector"
).igGrid(
"selectedCell"
);
-
selectedCells
- .igGrid( "selectedCells" );
- 返却型:
- array
すべてのオブジェクトが {element: , row: , index: , rowIndex: , columnKey: } の書式を持つ選択セルの配列を順序不同に返します。
複数選択が無効な場合、null を返します。コード サンプル
var
cells = $(
".selector"
).igGrid(
"selectedCells"
);
-
selectedRow
- .igGrid( "selectedRow" );
- 返却型:
- object
{element: , index: } の書式設定がある現在選択されている行がある場合、それを返します。
複数選択が有効な場合、null を返します。コード サンプル
var
row = $(
".selector"
).igGrid(
"selectedRow"
);
-
selectedRows
- .igGrid( "selectedRows" );
- 返却型:
- array
すべてのオブジェクトが { element: , index: } の書式を持つ選択行の配列を順序不同で返します。
複数選択が無効な場合、null を返します。コード サンプル
var
rows = $(
".selector"
).igGrid(
"selectedRows"
);
-
setColumnTemplate
- .igGrid( "setColumnTemplate", col:object, tmpl:string, [render:bool] );
初期化の後に列に新しいテンプレートを設定し、明示的に無効に設定されていない場合にグリッドを描画します。このメソッドは、既存の設定した行テンプレートを置き換え、新しい行テンプレートを列テンプレートから作成します。
- col
- タイプ:object
- テンプレートを設定する列の識別子 (インデックスまたはキー)。
- tmpl
- タイプ:string
- 設定する列テンプレート。
- render
- タイプ:bool
- オプション
- テンプレートが設定した後にグリッドを再描画するかどうか。
コード サンプル
$(
".selector"
).igGrid(
"setColumnTemplate"
,
"Name"
,
"<img src='${ImageUrl}'/>"
,
true
);
-
setUnboundValueByPK
- .igGrid( "setUnboundValueByPK", col:string, rowId:string, val:object, notToRender:object );
指定した列キーおよび行プライマリ キーによって非バインド セルの非バインド値を設定します。
- col
- タイプ:string
- 非バインド列のキー。
- rowId
- タイプ:string
- 行のプライマリ キー値
- val
- タイプ:object
- 非バインドセルに設定する値。
- notToRender
- タイプ:object
- false の場合行を再描画します。
コード サンプル
//IsPromotion is the key of an unbound column and 2 is the id of the row being affected based on the primaryКey column. The new value for the cell would be true and notToRender - false will re-render the row
$(
".selector"
).igGrid(
"setUnboundValueByPK"
,
"IsPromotion"
, 2,
true
,
false
);
-
setUnboundValues
- .igGrid( "setUnboundValues", key:string, values:array, removeOldValues:object );
指定したキーの非バインド列の非バインド値を設定します。removeOldValues が true の場合、非バインド列の値は新しい値とリセットされます。
- key
- タイプ:string
- 非バインド列のキー。
- values
- タイプ:array
- 非バインド列に設定する値の配列。
- removeOldValues
- タイプ:object
- true の場合、指定した列の現在に非バインドされる値を削除し、パラメーター値に指定される新しい値を適用します。それ以外の場合、パラメーター値に指定される値と現在値を結合します。
コード サンプル
// PromotionExpDate is the key of an unbound column. The array of dates is used to set unbound values
$(
".selector"
).igGrid(
"setUnboundValues"
,
"PromotionExpDate"
, [
new
Date(
'4/24/2012'
),
new
Date(
'8/24/2012'
),
new
Date(
'6/24/2012'
),
new
Date(
'7/24/2012'
)]);
-
showColumn
- .igGrid( "showColumn", column:object, callback:function );
非表示の列を表示します。列が非表示でない場合、このメソッドは何もしません。
注: このメソッドは非同期です。ただちに返し、以後のコードは並行して実行されます。ランタイム エラーを発生する場合があります。エラーを回避するには、メソッドによって提供されるコールバック パラメーターに以後のコードを挿入します。- column
- タイプ:object
- 列の識別子。数字が提供される場合、列インデックスとして使用されます。文字列が提供される場合、列キーとして使用されます。
- callback
- タイプ:function
- 列を表示したときに呼び出すカスタム関数を指定します (オプション)。
コード サンプル
$(
".selector"
).igGrid(
"showColumn"
, 1);
$(
".selector"
).igGrid(
"showColumn"
,
"ProductID"
);
-
totalRecordsCount
- .igGrid( "totalRecordsCount" );
- 返却型:
- number
- 返却型の説明:
- バックエンド内のレコード数。
基本のバックエンドのレコードの合計数を返します。ページングまたはフィルタリングが有効な場合、クライアント側のデータ ソースのレコードの数と異なる可能性があります。
これを機能させるには、応答の JSON/XML にレコードの総数を指定するプロパティが含まれている必要があります。その名前は options.responseTotalRecCountKey で指定されます。
この機能は、データ ソース コントロールに完全にデリゲートされます。コード サンプル
var
count = $(
".selector"
).igGrid(
"totalRecordsCount"
);
-
transactionsAsString
- .igGrid( "transactionsAsString" );
- 返却型:
- string
蓄積されたトランザクション ログを文字列として返します。URL に渡す場合など有効に利用するのが目的です。
それは、this.dataSource.transactionsAsString() のラッパーです。コード サンプル
var
transactionsString = $(
".selector"
).igGrid(
"transactionsAsString"
);
-
virtualScrollTo
- .igGrid( "virtualScrollTo", scrollerPosition:object );
指定した行またはピクセル単位の指定した位置にスクロールします。
- scrollerPosition
- タイプ:object
- 垂直スクロール位置の識別子。文字列の場合、ピクセルとして操作されます。それ以外の場合、行番号です。
コード サンプル
$(
".selector"
).igGrid(
"virtualScrollTo"
, scrollContainerTop);
-
widget
- .igGrid( "widget" );
データ レコードを保持する要素を返します。
コード サンプル
var
grid = $(
".selector"
).igGrid(
"widget"
);
-
ui-widget ui-helper-clearfix ui-corner-all
- 上のコンテナー要素に適用されるクラス。
-
ui-widget-content
- グリッド内の様々なコンテンツ コンテナーに適用されるウィジェット コンテンツ クラス。
-
ui-widget-header
- グリッド ヘッダー要素に適用される JQuery UI クラス。
-
ui-iggrid-deletedrecord
- コミット前にグリッドの削除された行に適用されるクラス。
-
ui-iggrid
- 最上位のコンテナー要素に適用されるクラス。
-
ui-iggrid-footertable ui-widget-footer
- 固定フッターが有効な場合、このクラスは、フッター TD 要素を保持するテーブルに適用されます。
-
ui-iggrid-headercaption ui-widget-header ui-corner-top
- 記述を含むヘッダーの上にある要素に適用されるクラス。
-
ui-iggrid-headertable
- 固定ヘッダーが有効な場合、このクラスは、ヘッダー TH 要素を保持するテーブルに適用されます。
-
ui-iggrid-measurement-container
- 自動サイズ変更可能な列の測定で使用される非表示 DIV コンテナーに適用されるクラス。
-
iggrid-scrollbars-wrapper
- タッチが有効な環境で igScroll スクロールバーをラップする要素に適用されるクラス。
-
ui-iggrid-scrolldiv ui-widget-content
- 幅と高さが定義されていてスクロールバーが True のときにスクロール div コンテナーに適用されるクラス。
-
ui-iggrid-tablebody
- データ レコードを保持する TABLE の TBODY に適用されるクラス。
-
ui-iggrid-table ui-widget-content
- グリッド レコードを保持する TABLE に適用されるクラス。
-
ui-iggrid-virtualscrolldiv
- 仮想化が有効の場合、スクロール DIV コンテナーに適用されるクラス。
-
ui-iggrid-headercell-featureenabled
- 機能アイコンが描画される場合、グリッドのヘッダー セル要素に適用されるクラス。
-
ui-iggrid-header ui-widget-header
- グリッド ヘッダー要素に適用されるクラス。
-
ui-iggrid-headertext
- ヘッダー テキスト コンテナーに適用されるクラス。
-
ui-iggrid-modifiedrecord
- コミット前にグリッドの変更された行に適用されるクラス。
-
ui-iggrid-headertable-mrl-scrollable
-
ui-iggrid-headertable-mrl
- 複数行レイアウトが有効な場合、このクラスは、複数行レイアウト ヘッダーを持つテーブルに適用されます。
-
ui-iggrid-table-mrl ui-widget-content
- 複数行レイアウトが使用される場合にグリッドの TABLE 要素に適用されるクラス。
-
ui-ig-altrecord ui-iggrid-altrecord
- 代替レコードに適用されるクラス。
-
ui-ig-record ui-iggrid-record
- TBODY に適用され、レコードの CSS を介して継承されるクラス。
-
ui-iggrid-rtl
- RTL が有効な場合、グリッド要素に適用されるクラス。