ui.igGridSorting
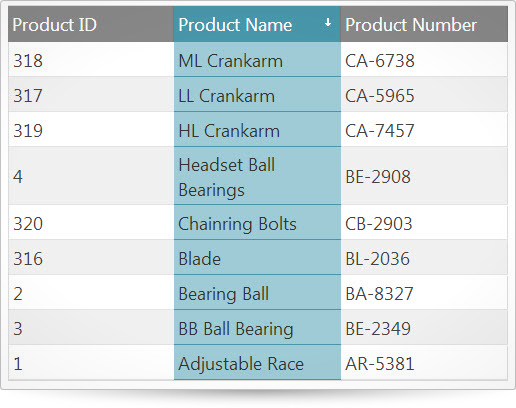
igGrid コントロールおよび igHierarchicalGrid コントロールはどちらも、列の並べ替え機能を備えています。この機能を使用すると、ユーザーは、指定された列の並べ替え順序に従ってグリッド内の行を順序付けできます。昇順および降順の並べ替え方向を両方とも使用可能です。この API のクラス、オプション、イベント、メソッド、およびテーマに関するさらに詳しい情報は上の関連するタブの下で入手可能です。
次のコード スニペットは、igGrid コントロールを初期化する方法を示します。
この API を使用した作業方法の詳細についてはここをクリックしてください。igGrid コントロールの必要なスクリプトおよびテーマを参照する方法については、 「Ignite UI で JavaScript リソースを使用する」および Ignite UI のスタイル設定とテーマを参照してください。コード サンプル
<!doctype html> <html> <head> <!-- Infragistics Combined CSS --> <link href="css/themes/infragistics/infragistics.theme.css" rel="stylesheet" type="text/css" /> <link href="css/structure/infragistics.css" rel="stylesheet" type="text/css" /> <!-- jQuery Core --> <script src="js/jquery.js" type="text/javascript"></script> <!-- jQuery UI --> <script src="js/jquery-ui.js" type="text/javascript"></script> <!-- Infragistics Combined Scripts --> <script src="js/infragistics.core.js" type="text/javascript"></script> <script src="js/infragistics.lob.js" type="text/javascript"></script> <script type="text/javascript"> var products = [ { "ProductID": 1, "Name": "Adjustable Race", "ProductNumber": "AR-5381" }, { "ProductID": 2, "Name": "Bearing Ball", "ProductNumber": "BA-8327" }, { "ProductID": 3, "Name": "BB Ball Bearing", "ProductNumber": "BE-2349" }, { "ProductID": 4, "Name": "Headset Ball Bearings", "ProductNumber": "BE-2908" }, { "ProductID": 316, "Name": "Blade", "ProductNumber": "BL-2036" }, { "ProductID": 317, "Name": "LL Crankarm", "ProductNumber": "CA-5965" }, { "ProductID": 318, "Name": "ML Crankarm", "ProductNumber": "CA-6738" }, { "ProductID": 319, "Name": "HL Crankarm", "ProductNumber": "CA-7457" }, { "ProductID": 320, "Name": "Chainring Bolts", "ProductNumber": "CB-2903" } ]; $(function () { $("#gridSorting").igGrid({ columns: [ { headerText: "Product ID", key: "ProductID", dataType: "number" }, { headerText: "Product Name", key: "Name", dataType: "string" }, { headerText: "Product Number", key: "ProductNumber", dataType: "string" } ], features: [ { name: "Sorting", type: "local" } ], width: "500px", dataSource: products }); }); </script> </head> <body> <table id="gridSorting"></table> </body> </html>
関連サンプル
関連トピック
依存関係
-
applySortedColumnCss
- タイプ:
- bool
- デフォルト:
- true
並べ替えた列の特別なスタイル設定を有効/無効にします。False の場合、並べ替えられた列のセルで並べ替えに関連する特別なスタイルは適用されません。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", applySortedColumnCss : false } ] }); // Get var sortCss = $(".selector").igGridSorting("option", "applySortedColumnCss"); // Set $(".selector").igGridSorting("option", "applySortedColumnCss", false);
-
caseSensitive
- タイプ:
- bool
- デフォルト:
- false
並べ替えの大文字と小文字の区別を有効または無効にします。ローカルの並べ替えでのみ操作します。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", caseSensitive : true } ] }); // Get var caseSensitive = $(".selector").igGridSorting("option", "caseSensitive"); // Set $(".selector").igGridSorting("option", "caseSensitive", true);
-
columnSettings
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
特定の列のカスタムの並べ替え設定 (並べ替えが有効か無効か、デフォルトの並べ替え方向、最初の並べ替え方向など) を指定するカスタム列設定のリスト。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", columnSettings : [ { columnIndex: 0, allowSorting: true, firstSortDirection: "ascending", currentSortDirection: "descending" } ] } ] }); // Get var colSettings = $(".selector").igGridSorting("option", "columnSettings");
-
allowSorting
- タイプ:
- bool
- デフォルト:
- true
指定された列での並べ替えを有効または無効にします。デフォルトでは、すべての列が並べ替え可能です。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", columnSettings : [ { columnIndex: 0, allowSorting: true, firstSortDirection: "ascending", currentSortDirection: "descending" } ] } ] });
-
columnIndex
- タイプ:
- number
- デフォルト:
- null
列インデックス。すべての列設定にキーまたはインデックスのどちらかを設定する必要があります。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", columnSettings : [ { columnIndex: 0, allowSorting: true, firstSortDirection: "ascending", currentSortDirection: "descending" } ] } ] }); // Get var colSettings = $(".selector").igGridSorting("option", "columnSettings"); var colIndex = colSettings[0].columnIndex;
-
columnKey
- タイプ:
- string
- デフォルト:
- null
列キー。すべての列設定にキーまたはインデックスのどちらかを設定する必要があります。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", columnSettings : [ { columnKey: "ProductID", allowSorting: true, firstSortDirection: "ascending", currentSortDirection: "descending" } ] } ] }); //Get var colSettings = $(".selector").igGridSorting("option", "columnSettings"); var colKey = colSettings[0].columnKey;
-
compareFunc
- タイプ:
- enumeration
- デフォルト:
- null
カスタム比較に使用される関数 (文字列または関数) への参照。 関数は以下の引数を受け取ります:
val1: 比較する最初の値
val2: 比較する 2 番目の値
recordsData (オプション) - 3 つのプロパティを持つオブジェクト: fieldName - 並べ替えたフィールドの名前; record1 - 比較する最初のレコード; record2 - 比較する 2 番目のレコード
関数は以下の数値を返します:
0 - 値が等しい場合
1 - val1 > val2 の場合
-1 - val1 < val2 の場合メンバー
- string
- タイプ:string
- グローバル ウィンドウ オブジェクトにある文字列としての関数名。
- function
- タイプ:function
- カスタム比較で使用される関数。
コード サンプル
var myCompareFunc = function (fields, schema, reverse, convertf) { return function (val1, val2) { if (val1.Price > val2.Price) { return 1; } if (val1.Price < val2.Price) { return -1; } return 0; } } // Initialize $(".selector").igGrid({ features : [ { name : "Sorting", columnSettings: [ { columnIndex: 0, allowSorting: true, firstSortDirection: "ascending", compareFunc: "myCompareFunc" } ] } ] });
-
currentSortDirection
- タイプ:
- enumeration
- デフォルト:
- null
現在の (またはデフォルトの) 並べ替え方向。この設定が指定されていない場合、このオプションに基づいて列が描画および並べ替えされます。
メンバー
- asc
- タイプ:string
- 列データの初期の並べ替えは昇順です。
- desc
- タイプ:string
- 列データの初期の並べ替えは降順です。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", columnSettings : [ { columnIndex: 0, allowSorting: true, firstSortDirection: "ascending", currentSortDirection: "descending" } ] } ] });
-
firstSortDirection
- タイプ:
- enumeration
- デフォルト:
- null
それまでその列で並べ替えをしていない場合、これは最初の並べ替え方向になります。
メンバー
- asc
- タイプ:string
- 列データの最初の並べ替えは昇順です。
- desc
- タイプ:string
- 列データの最初の並べ替えは降順です。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", columnSettings : [ { columnIndex: 0, allowSorting: true, firstSortDirection: "ascending", currentSortDirection: "descending" } ] } ] });
-
customSortFunction
- タイプ:
- function
- デフォルト:
- null
カスタム並べ替え関数 (または文字列として関数の名前)。3 つのパラメーターを受け付けます - 並べ替えるデータ、データ ソースのフィールド定義の配列、および並べ替える方向 (オプション)。関数は並べ替えられたデータ配列を返す必要があります。
コード サンプル
var myCustomFunc = function(data, fields, direction) { function myCompareFunc(obj1, obj2) { if (direction == "descending") { return obj2[fields[0].fieldName] - obj1[fields[0].fieldName]; } return obj1[fields[0].fieldName] - obj2[fields[0].fieldName]; } var result = data.sort(myCompareFunc); return result; } //Initialize $(".selector").igGrid({ features : [ { name : "Sorting", customSortFunction : myCustomFunc } ] }); //Get var sortFunc = $(".selector").igGridSorting("option", "customSortFunction"); //Set $(".selector").igGridSorting("option", "customSortFunction", myCustomFunc);
-
dialogWidget
- タイプ:
- string
- デフォルト:
- "igGridModalDialog"
使用するダイアログ ウィジェットの名前。 $.ui.igGridModalDialog から継承します。
コード サンプル
// Initialize $(".selector").igGrid({ features: [ { name : "Sorting", dialogWidget: "advancedModalDialog" } ] }); // Get var widgetName = $(".selector").igGridSorting("option", "dialogWidget");
-
featureChooserSortAsc
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
昇順で並べ替えるために機能セレクター項目でテキストを指定します (タッチ環境のみで表示されます)。locale.featureChooserSortAsc オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", featureChooserSortAsc : "Sort A to Z" } ] }); // Get var featureChooserSortAsc = $(".selector").igGridSorting("option", "featureChooserSortAsc");
-
featureChooserSortDesc
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
降順で並べ替えるために機能セレクター項目でテキストを指定します (タッチ環境のみで表示されます)。
locale.featureChooserSortDesc オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", featureChooserSortDesc : "Sort Z to A" } ] }); // Get var featureChooserSortDesc = $(".selector").igGridSorting("option", "featureChooserSortDesc");
-
featureChooserText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
機能セレクターのテキストを指定します。
locale.featureChooserText オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", featureChooserText : "Sorting" } ] }); // Get var text = $(".selector").igGridSorting("option", "featureChooserText"); // Set $(".selector").igGridSorting("option", "featureChooserText", "Sorting");
-
firstSortDirection
- タイプ:
- enumeration
- デフォルト:
- ascending
それまでその列で並べ替えをしていない場合、最初のクリックまたはキーの押下で使用する並べ替え方向を指定します。
メンバー
- ascending
- タイプ:string
- 列データの最初の並べ替えは昇順です。
- descending
- タイプ:string
- 列データの最初の並べ替えは降順です。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", firstSortDirection : "descending" } ] }); // Get var direction = $(".selector").igGridSorting("option", "firstSortDirection"); // Set $(".selector").igGridSorting("option", "firstSortDirection", "descending");
-
inherit
- タイプ:
- bool
- デフォルト:
- false
子レイアウトで機能継承を有効または無効にします。注: igHierarchicalGrid のみに適用します。
コード サンプル
// Initialize $(".selector").igHierarchicalGrid({ features: [ { name: "Sorting", inherit: true } ] }); // Get var inherit = $(".selector").igGridSorting("option", "inherit");
-
language
継承- タイプ:
- string
- デフォルト:
- "en"
ウィジェットのロケール言語設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igGridSorting({ language: "ja" }); // Get var language = $(".selector").igGridSorting("option", "language"); // Set $(".selector").igGridSorting("option", "language", "ja");
-
locale
- タイプ:
- object
- デフォルト:
- {}
-
ascending
- タイプ:
- string
- デフォルト:
- ""
ヘッダー タイトルに使用される昇順テキスト。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { ascending: "Ascending" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").ascending; // Set $(".selector").igGridSorting("option", "locale", { ascending: "Ascending" });
-
descending
- タイプ:
- string
- デフォルト:
- ""
ヘッダー タイトルに使用される降順テキスト。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { ascending: "Ascending" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").ascending; // Set $(".selector").igGridSorting("option", "locale", { ascending: "Ascending" });
-
featureChooserSortAsc
- タイプ:
- string
- デフォルト:
- ""
昇順で並べ替えるために機能セレクター項目でテキストを指定します (タッチ環境のみで表示されます)。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { featureChooserSortAsc: "Sort A to Z" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").featureChooserSortAsc; // Set $(".selector").igGridSorting("option", "locale", { featureChooserSortAsc: "Sort A to Z" });
-
featureChooserSortDesc
- タイプ:
- string
- デフォルト:
- ""
降順で並べ替えるために機能セレクター項目でテキストを指定します (タッチ環境のみで表示されます)。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { featureChooserSortDesc: "Sort Z to A" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").featureChooserSortDesc; // Set $(".selector").igGridSorting("option", "locale", { featureChooserSortDesc: "Sort Z to A" });
-
featureChooserText
- タイプ:
- string
- デフォルト:
- ""
機能セレクターの並べ替えボタンのテキストを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { featureChooserText: "Sorting" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").featureChooserText; // Set $(".selector").igGridSorting("option", "locale", { featureChooserText: "Sorting" });
-
modalDialogButtonApplyText
- タイプ:
- string
- デフォルト:
- ""
モーダル ダイアログで変更を適用するボタンのテキストを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { modalDialogButtonApplyText: "Apply" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").modalDialogButtonApplyText; // Set $(".selector").igGridSorting("option", "locale", { modalDialogButtonApplyText: "Apply" });
-
modalDialogButtonCancelText
- タイプ:
- string
- デフォルト:
- ""
詳細並べ替えのモーダル ダイアログで変更をキャンセルするボタンのテキストを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { modalDialogButtonCancelText: "Cancel" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").modalDialogButtonCancelText; // Set $(".selector").igGridSorting("option", "locale", { modalDialogButtonCancelText: "Cancel" });
-
modalDialogCaptionButtonAsc
- タイプ:
- string
- デフォルト:
- ""
複数の並べ替えダイアログで昇順に並べ替えられた各列のキャプションを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { modalDialogCaptionButtonAsc: "Ascending" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").modalDialogCaptionButtonAsc; // Set $(".selector").igGridSorting("option", "locale", { modalDialogCaptionButtonAsc: "Ascending" });
-
modalDialogCaptionButtonDesc
- タイプ:
- string
- デフォルト:
- ""
[複数並べ替え] ダイアログの降順に並べ替えられた項目ごとにキャプションを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { modalDialogCaptionButtonDesc: "Descending" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").modalDialogCaptionButtonDesc; // Set $(".selector").igGridSorting("option", "locale", { modalDialogCaptionButtonDesc: "Descending" });
-
modalDialogCaptionButtonUnsort
- タイプ:
- string
- デフォルト:
- ""
[複数並べ替え] ダイアログの [並べ替えなし]ボタンのキャプションを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { modalDialogCaptionButtonUnsort: "Unsort" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").modalDialogCaptionButtonUnsort; // Set $(".selector").igGridSorting("option", "locale", { modalDialogCaptionButtonUnsort: "Unsort" });
-
modalDialogCaptionText
- タイプ:
- string
- デフォルト:
- ""
[複数並べ替え] ダイアログのキャプションのテキストを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { modalDialogCaptionText: "Multiple Sorting" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").modalDialogCaptionText; // Set $(".selector").igGridSorting("option", "locale", { modalDialogCaptionText: "Multiple Sorting" });
-
modalDialogResetButton
- タイプ:
- string
- デフォルト:
- ""
モーダル ダイアログで [リセット] ボタンのテキストを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { modalDialogResetButton: "Reset" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").modalDialogResetButton; // Set $(".selector").igGridSorting("option", "locale", { modalDialogResetButton: "Reset" });
-
modalDialogSortByButtonText
- タイプ:
- string
- デフォルト:
- ""
複数の並べ替えダイアログで並べ替えが解除された列ごとに並べ替えボタンのテキストを指定します。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { modalDialogSortByButtonText: "Sort By" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").modalDialogSortByButtonText; // Set $(".selector").igGridSorting("option", "locale", { modalDialogSortByButtonText: "Sort By" });
-
sortedColumnTooltip
- タイプ:
- string
- デフォルト:
- ""
並べ替えられた列の igTemplating 形式のカスタム ツールチップ。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { sortedColumnTooltip: "Sorted ${direction}" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").sortedColumnTooltip; // Set $(".selector").igGridSorting("option", "locale", { sortedColumnTooltip: "Sorted ${direction}" });
-
unsortedColumnTooltip
- タイプ:
- string
- デフォルト:
- ""
並べ替えていない列のツールチップ。
コード サンプル
//Initialize $(".selector").igGrid({ features: [{ name: "Sorting", locale: { unsortedColumnTooltip: "Sort column" } }] }); // Get var text = $(".selector").igGridSorting("option", "locale").unsortedColumnTooltip; // Set $(".selector").igGridSorting("option", "locale", { unsortedColumnTooltip: "Sort column" });
-
modalDialogAnimationDuration
- タイプ:
- number
- デフォルト:
- 200
モーダル ダイアログを表示/非表示にするためにアニメーション期間のミリ秒の時間を指定します。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogAnimationDuration : 300 } ] }); // Get var duration = $(".selector").igGridSorting("option", "modalDialogAnimationDuration"); // Set $(".selector").igGridSorting("option", "modalDialogAnimationDuration", 300);
-
modalDialogButtonApplyText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
モーダル ダイアログで変更を適用するボタンのテキストを指定します。
locale.modalDialogButtonApplyText オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogButtonApplyText : "Apply" } ] }); // Get var text = $(".selector").igGridSorting("option", "modalDialogButtonApplyText"); // Set $(".selector").igGridSorting("option", "modalDialogButtonApplyText", "Apply");
-
modalDialogButtonCancelText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
モーダル ダイアログで変更をキャンセルするボタンのテキストを指定します。
locale.modalDialogButtonCancelText オプションを使用します。コード サンプル
//Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogButtonCancelText : "Cancel" } ] }); //Get var text = $(".selector").igGridSorting("option", "modalDialogButtonCancelText"); //Set $(".selector").igGridSorting("option", "modalDialogButtonCancelText", "Cancel");
-
modalDialogCaptionButtonAsc
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
複数の並べ替えダイアログで昇順に並べ替えられた各列のキャプションを指定します。
locale.modalDialogCaptionButtonAsc オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogCaptionButtonAsc : "Ascending" } ] }); // Get var caption = $(".selector").igGridSorting("option", "modalDialogCaptionButtonAsc"); // Set $(".selector").igGridSorting("option", "modalDialogCaptionButtonAsc", "Ascending");
-
modalDialogCaptionButtonDesc
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
[複数並べ替え] ダイアログの降順に並べ替えられた項目ごとにキャプションを指定します。
locale.modalDialogCaptionButtonDesc オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogCaptionButtonDesc : "Descending" } ] }); // Get var caption = $(".selector").igGridSorting("option", "modalDialogCaptionButtonDesc"); // Set $(".selector").igGridSorting("option", "modalDialogCaptionButtonDesc", "Descending");
-
modalDialogCaptionButtonUnsort
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
[複数並べ替え] ダイアログの [並べ替えなし]ボタンのキャプションを指定します。
locale.modalDialogCaptionButtonUnsort オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogCaptionButtonUnsort : "Unsort" } ] }); // Get var caption = $(".selector").igGridSorting("option", "modalDialogCaptionButtonUnsort"); // Set $(".selector").igGridSorting("option", "modalDialogCaptionButtonUnsort", "Unsort");
-
modalDialogCaptionText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
[複数並べ替え] ダイアログのキャプションのテキストを指定します。
locale.modalDialogCaptionText オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogCaptionText : "Multiple Sorting" } ] }); // Get var caption = $(".selector").igGridSorting("option", "modalDialogCaptionText"); // Set $(".selector").igGridSorting("option", "modalDialogCaptionText", "Multiple Sorting");
-
modalDialogHeight
- タイプ:
- enumeration
- デフォルト:
- ""
複数の並べ替えダイアログの高さを指定します。
メンバー
- string
- タイプ:string
- ウィジェットの高さはピクセル (px) およびパーセント (%) で設定できます (%)。
- number
- タイプ:number
- 幅の高さは数値 (25) として設定できます。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogHeight : 300 } ] }); // Get var height = $(".selector").igGridSorting("option", "modalDialogHeight"); // Set $(".selector").igGridSorting("option", "modalDialogHeight", 300);
-
modalDialogResetButtonLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
複数の並べ替えダイアログで並べ替えが解除された列ごとに並べ替えボタンのラベルを指定します。
locale.modalDialogResetButton オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogResetButtonLabel : "Reset" } ] }); // Get var label = $(".selector").igGridSorting("option", "modalDialogResetButtonLabel"); // Set $(".selector").igGridSorting("option", "modalDialogResetButtonLabel", "Reset");
-
modalDialogSortByButtonText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
複数の並べ替えダイアログで並べ替えが解除された列ごとに並べ替えボタンのテキストを指定します。
locale.modalDialogSortByButtonText オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogSortByButtonText : "Sort By" } ] }); // Get var text = $(".selector").igGridSorting("option", "modalDialogSortByButtonText"); // Set $(".selector").igGridSorting("option", "modalDialogSortByButtonText", "Sort By");
-
modalDialogSortOnClick
- タイプ:
- bool
- デフォルト:
- false
複数の並べ替えダイアログで列を並べ替える/並べ替えを解除するをクリックした時に直ちに並べ替えが適用されるかどうかを指定します。false の場合 [適用] ボタンが表示され、ボタンをクリックすると並べ替えが適用されます。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogSortOnClick : true } ] }); // Get var text = $(".selector").igGridSorting("option", "modalDialogSortOnClick"); // Set $(".selector").igGridSorting("option", "modalDialogSortOnClick", true);
-
modalDialogWidth
- タイプ:
- enumeration
- デフォルト:
- 350
複数の並べ替えダイアログの幅を指定します。
メンバー
- string
- タイプ:string
- 幅をピクセル単位で文字列として指定します ("300px")。
- number
- タイプ:number
- 幅をピクセル単位で数値として指定します (300)。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", modalDialogWidth : 400 } ] }); // Get var width = $(".selector").igGridSorting("option", "modalDialogWidth"); // Set $(".selector").igGridSorting("option", "modalDialogWidth", 300);
-
mode
- タイプ:
- enumeration
- デフォルト:
- single
単一列の並べ替えまたは複数列の並べ替えを定義します。
メンバー
- single
- タイプ:string
- 単一の列のみを並べ替えます。新しい列を並べ替えると、以前に並べ替えた列の並べ替えが保持されていません。
- multi
- タイプ:string
- 有効な場合、前に列の並べ替えを行った状態はクリアされません。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", mode : "multi" } ] }); // Get var mode = $(".selector").igGridSorting("option", "mode"); // Set $(".selector").igGridSorting("option", "mode", "multi");
-
persist
- タイプ:
- bool
- デフォルト:
- true
状態の間に並べ替えの永続化を有効/無効にします。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", persist : false } ] }); // Get var persist = $(".selector").igGridSorting("option", "persist"); // Set $(".selector").igGridSorting("option", "persist", true);
-
regional
継承- タイプ:
- enumeration
- デフォルト:
- defaults
ウィジェットの領域設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igGridSorting({ regional: "ja" }); // Get var regional = $(".selector").igGridSorting("option", "regional"); // Set $(".selector").igGridSorting("option", "regional", "ja");
-
sortingDialogContainment
- タイプ:
- string
- デフォルト:
- "owner"
複数並べ替えダイアログのコンテインメント動作を管理します。
owner 複数並べ替えダイアログはグリッド領域のみにドラッグ可能です。
window 複数並べ替えダイアログはウィンドウ領域の全体にドラッグ可能です。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", sortingDialogContainment : "window" } ] }); // Get var sortingDialogContainment = $(".selector").igGridSorting("option", "sortingDialogContainment");
-
sortUrlKey
- タイプ:
- string
- デフォルト:
- null
並べ替えの式を URL 内でエンコードする方法を指定する URL パラメーター名。OData conventions. ex: ?sort(col1)=asc を使用します。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", sortUrlKey: "myCustomSort" } ] }); // Get var sortKey = $(".selector").igGridSorting("option", "sortUrlKey"); // Set // Results in the following URL "?myCustomSort(col1)=asc" $(".selector").igGridSorting("option", "sortUrlKey", "myCustomSort");
-
sortUrlKeyAscValue
- タイプ:
- string
- デフォルト:
- null
昇順の並べ替えの URL パラメーター値。OData 規約を使用します。例: ?sort(col1)=asc。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", sortUrlKey: "myCustomSort", sortUrlKeyAscValue: "myAsc" } ] }); // Get var sortKeyAsc = $(".selector").igGridSorting("option", "sortUrlKeyAscValue"); // Set // Results in the following URL "?sort(col1)=myAsc" $(".selector").igGridSorting("option", "sortUrlKeyAscValue", "myAsc");
-
sortUrlKeyDescValue
- タイプ:
- string
- デフォルト:
- null
降順の並べ替えの URL パラメーター値。OData 規約を使用します。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", sortUrlKey: "myCustomSort", sortUrlKeyDescValue: "myDesc" } ] }); // Get var sortKeyDesc = $(".selector").igGridSorting("option", "sortUrlKeyDescValue"); // Set // Results in the following URL "?sort(col1)=myDesc" $(".selector").igGridSorting("option", "sortUrlKeyDescValue", "myDesc");
-
type
- タイプ:
- enumeration
- デフォルト:
- null
ローカルまたはリモート並べ替えを定義。
メンバー
- remote
- タイプ:string
- 並べ替えはサーバー側操作としてリモートで実行されます。
- local
- タイプ:string
- 並べ替えは $.ig.DataSource コンポーネントによってローカルで実行されます。
コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", type : "remote" } ] }); // Get var sortType = $(".selector").igGridSorting("option", "type"); // Set $(".selector").igGridSorting("option", "type", "remote");
-
unsortedColumnTooltip
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
並べ替えされていない列の igTemplating 形式のカスタム ツールチップ。
locale.unsortedColumnTooltip オプションを使用します。コード サンプル
// Initialize $(".selector").igGrid({ features : [ { name : "Sorting", unsortedColumnTooltip : "Unsorted" } ] }); // Get var tooltip = $(".selector").igGridSorting("option", "unsortedColumnTooltip"); // Set $(".selector").igGridSorting("option", "unsortedColumnTooltip", "Unsorted");
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
columnSorted
- キャンセル可能:
- false
列が並べ替えられ、データが再描画された後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingcolumnsorted", ".selector", function (evt, ui) { //return reference to igGridSorting object ui.owner; //return reference to igGrid object ui.owner.grid; //return column key ui.columnKey; //return sort direction ui.direction; //return sorting expressions from the datasource ui.expressions; }); //Initialize $(".selector").igGrid({ features: [{ name: "Sorting", type: "local", columnSorted: function (evt, ui) {...} }] });
-
columnSorting
- キャンセル可能:
- true
ある列に対して並べ替えを呼び出す前に発生するイベント。
行の並べ替えをキャンセルするには、false を返します。コード サンプル
//Bind after initialization $(document).on("iggridsortingcolumnsorting", ".selector", function (evt, ui) { //return reference to igGridSorting object ui.owner; //return reference to igGrid object ui.owner.grid; //return column key ui.columnKey; //return sort direction ui.direction; //return sorting expressions from the datasource ui.newExpressions; }); //Initialize $(".selector").igGrid({ features: [{ name: "Sorting", type: "local", columnSorting: function (evt, ui) {...} }] });
-
modalDialogButtonApplyClick
- キャンセル可能:
- true
モーダル ダイアログの [適用] ボタンをクリックしたときに発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogbuttonapplyclick", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; // Get array of columns which should be sorted - array of objects of sort order - Asc/Desc and column key ui.columnsToSort; }); //Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogButtonApplyClick: function (evt, ui) {...} }] });
-
modalDialogButtonResetClick
- キャンセル可能:
- true
並べ替えをリセットするボタンをクリックしたときに発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogbuttonresetclick", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; }); //Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogButtonResetClick: function (evt, ui) {...} }] });
-
modalDialogButtonUnsortClick
- キャンセル可能:
- true
列の並べ替えをしないボタンをモーダル ダイアログでクリックした場合に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogbuttonunsortclick", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; // Get column key. ui.columnKey; }); //Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogButtonUnsortClick: function (evt, ui) {...} }] });
-
modalDialogClosed
- キャンセル可能:
- false
モーダル ダイアログが閉じた後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogclosed", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogClosed: function (evt, ui) {...} }] });
-
modalDialogClosing
- キャンセル可能:
- true
モーダル ダイアログが閉じる前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogclosing", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogClosing: function (evt, ui) {...} }] });
-
modalDialogContentsRendered
- キャンセル可能:
- false
モーダル ダイアログのコンテンツが描画された後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogcontentsrendered", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogContentsRendered: function (evt, ui) {...} }] });
-
modalDialogContentsRendering
- キャンセル可能:
- true
モーダル ダイアログのコンテンツが描画される前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogcontentsrendering", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogContentsRendering: function (evt, ui) {...} }] });
-
modalDialogMoving
- キャンセル可能:
- true
モーダル ダイアログの位置が変更するたびに発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
igGridSorting への参照を取得します。
-
owner.gridタイプ: Object
igGrid への参照を取得します。
-
modalDialogElementタイプ: jQuery
モダル ダイアログ要素への参照を取得します。これは jQuery オブジェクトです。
-
originalPositionタイプ: Object
モーダル ダイアログ div の元の位置をページに相対して { top, left } オブジェクトとして取得します。
-
positionタイプ: Object
モーダル ダイアログ div の現在の位置をページに相対して { top, left } オブジェクトとして取得します。
-
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogmoving", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; // Get the original position of the modal dialog div as { top, left } object, relative to the page. ui.originalPosition; // Get the current position of the modal dialog div as { top, left } object, relative to the page. ui.position; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogMoving: function (evt, ui) {...} }] });
-
modalDialogOpened
- キャンセル可能:
- false
モーダル ダイアログがすでに開いた後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogopened", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogOpened: function (evt, ui) {...} }] });
-
modalDialogOpening
- キャンセル可能:
- true
モーダル ダイアログが開く前に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogopening", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogOpening: function (evt, ui) {...} }] });
-
modalDialogSortClick
- キャンセル可能:
- true
並べ替えられていない列をクリックして、モーダル ダイアログで並べ替える場合に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogsortclick", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; // Get the column key. ui.columnKey; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogSortClick: function (evt, ui) {...} }] });
-
modalDialogSortingChanged
- キャンセル可能:
- true
列の並べ替えがモーダル ダイアログで変更された場合に発生するイベント。列を並べ替える必要があります。
コード サンプル
//Bind after initialization $(document).on("iggridsortingmodaldialogsortingchanged", ".selector", function (evt, ui) { // Get reference to igGridSorting object ui.owner; // Get the reference to the igGrid widget. ui.owner.grid; // Get a reference to the modal dialog element. This is a jQuery object. ui.modalDialogElement; // Get the column key. ui.columnKey; // Get whether column should be ascending or not. If true it should be ascending ui.isAsc; }); // Initialize $(".selector").igGrid({ features: [{ name: "Sorting", modalDialogSortingChanged: function (evt, ui) {...} }] });
-
changeLocale
- .igGridSorting( "changeLocale" );
ウィジェット要素のすべてのロケールを options.language に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。コード サンプル
$(".selector").igGridSorting("changeLocale");
-
clearSorting
- .igGridSorting( "clearSorting" );
すべての並べ替えた列で現在の並べ替えを解除し、UI を更新します。
コード サンプル
$(".selector").igGridSorting("clearSorting");
-
closeMultipleSortingDialog
- .igGridSorting( "closeMultipleSortingDialog" );
複数並べ替えダイアログを閉じます。
コード サンプル
$(".selector").igGridSorting("closeMultipleSortingDialog");
-
destroy
- .igGridSorting( "destroy" );
イベントのバインディングを解除し、追加された並べ替え要素を削除するなど。
コード サンプル
$(".selector").igGridSorting("destroy");
-
openMultipleSortingDialog
- .igGridSorting( "openMultipleSortingDialog" );
複数並べ替えダイアログを開きます。
コード サンプル
$(".selector").igGridSorting("openMultipleSortingDialog");
-
removeDialogClearButton
- .igGridSorting( "removeDialogClearButton" );
[複数並べ替え] ダイアログの [クリア] ボタンを削除します。
コード サンプル
$(".selector").igGridSorting("removeDialogClearButton");
-
renderMultipleSortingDialogContent
- .igGridSorting( "renderMultipleSortingDialogContent", isToCallEvents:object );
並べ替えられた項目および並べ替えられていない項目の [複数並べ替え] ダイアログの内容を描画します。
- isToCallEvents
- タイプ:object
コード サンプル
$(".selector").igGridSorting("renderMultipleSortingDialogContent", true);
-
sortColumn
- .igGridSorting( "sortColumn", index:object, direction:object );
グリッド列を並べ替え、UI を更新します。
- index
- タイプ:object
- 列キー (文字列) またはインデックス (数値) - 複数行グリッドのみ列キーが使用できます。並べ替える列を指定します。モードが複数の場合、前の並べ替え状態がクリアされません。
- direction
- タイプ:object
- 並べ替え方向 (昇順または降順) を指定します。
コード サンプル
$(".selector").igGridSorting("sortColumn", 1, "descending");
-
sortMultiple
- .igGridSorting( "sortMultiple", [exprs:array] );
グリッド列を並べ替え、UI を更新します。並べ替え式の配列であるオプションの引数を受け付けます。渡された場合、データを並べ替え、データ ソースの並べ替え式を設定します。渡されない場合、データ ソースの現在並べ替え式を使用します。
- exprs
- タイプ:array
- オプション
- 並べ替え式の配列。設定されない場合、メソッドはデータ ソースの並べ替え設定に定義される式を使用します
コード サンプル
$(".selector").igGridSorting("sortMultiple");
-
unsortColumn
- .igGridSorting( "unsortColumn", index:object );
指定した columnKey/columnIndex を持つグリッド列で並べ替えを解除し、UI を更新します。
- index
- タイプ:object
- 列キー (文字列) またはインデックス (数値) - 複数行グリッドのみ列キーが使用できます。並べ替えを解除する列を指定します。モードが複数の場合、前の並べ替え状態がクリアされません。
コード サンプル
$(".selector").igGridSorting("unsortColumn", "ProductName");
-
ui-iggrid-colasc ui-state-highlight
- 昇順に並べ替えられるある列のセルに適用されるクラス。
-
ui-iggrid-colheaderasc
- 昇順に並べ替えられる列ヘッダーに適用されるクラス。
-
ui-iggrid-coldesc ui-state-highlight
- 降順に並べ替えられるある列のセルに適用されるクラス。
-
ui-iggrid-colheaderdesc
- 降順に並べ替えられる列ヘッダーに適用されるクラス。
-
ui-iggrid-sorting-dialog-ascdescbutton
- 複数並べ替えダイアログで昇順/降順ボタンに適用されるクラス。
-
ui-button ui-corner-all ui-button-icon-only ig-sorting-indicator
- 複数並べ替えダイアログで並べ替えた列の昇順ボタンに適用されるクラス。
-
ui-button-icon-primary ui-icon ui-icon-arrowthick-1-n
- 複数並べ替えダイアログで並べ替えた列の昇順ボタン アイコンに適用されるクラス。
-
ui-button ui-corner-all ui-button-icon-only ig-sorting-indicator
- 複数並べ替えダイアログで並べ替えた列の降順ボタン アイコンに適用されるクラス。
-
ui-button-icon-primary ui-icon ui-icon-arrowthick-1-s
- 複数並べ替えダイアログで並べ替えた列の降順ボタン アイコンに適用されるクラス。
-
ui-state-hover
- 複数並べ替えダイアログの並べ替え解除ボタンなどのホバーされたボタンに適用されるクラス。
-
ui-iggrid-sorting-dialog-sortbybuttons ui-button ui-widget ui-state-default ui-corner-all ui-button-icon-only ui-igbutton ui-widget-content ui-igbutton-remove
- 複数並べ替えダイアログで並べ替えた列の並べ替え解除ボタンに適用されるクラス。
-
ui-button-icon-primary ui-icon ui-icon-circle-close
- 複数並べ替えダイアログで並べ替えた列の並べ替え解除ボタンに適用されるクラス。
-
ui-iggrid-dialog-text
- 複数並べ替えダイアログで並べ替えていない列名を表示するコンテナーに適用されるクラス。
-
ui-iggrid-sorting-dialog-sortedcolumns
- 複数並べ替えダイアログで並べ替えた列のコンテナーに適用されるクラス。
-
ui-widget-content
- 複数並べ替えダイアログで各並べ替えた列のリスト項目に適用されるクラス。
-
ui-iggrid-dialog-text
- 複数並べ替えダイアログで並べ替えた列名を表示するコンテナーに適用されるクラス。
-
ui-iggrid-sorting-dialog-unsortedcolumns
- 複数並べ替えダイアログで並べ替えていない列のコンテナーに適用されるクラス。
-
ui-widget-content
- 複数並べ替えダイアログで並べ替えていない各列のリスト項目に適用されるクラス。
-
ui-iggrid-sorting-dialog-unsortedcolumns-sortbybutton
- 複数並べ替えダイアログで各列の並べ替えボタンに適用されるクラス。
-
ui-iggrid-featurechooser-li-iconcontainer ui-icon ui-iggrid-icon-sort-a-z
- 機能セレクターで昇順並べ替えインジケーターに適用されるクラス。
-
ui-iggrid-featurechooser-li-iconcontainer ui-icon ui-iggrid-icon-sort-z-a
- 機能セレクターで降順並べ替えインジケーターに適用されるクラス。
-
ui-icon ui-iggrid-icon-multiple-sorting
- 複数並べ替えダイアログを表示する昨日セレクター アイコンに適用されるクラス。
-
ui-iggrid-sorting-dialog-sortbybutton
- 複数並べ替えダイアログで並べ替え条件ボタンに適用されるクラス。
-
ui-iggrid-sortableheader ui-state-default
- 並べ替え可能な列ヘッダーに適用されるクラス。
-
ui-iggrid-sortableheaderactive ui-state-active
- アクティブな (キーボードでのナビゲートやクリックのできる) 並べ替え可能なヘッダーに適用されるクラス。
-
ui-iggrid-sortableheaderfocus ui-state-focus
- フォーカスがある並べ替え可能な列ヘッダーに適用されるクラス。
-
ui-iggrid-sortableheaderhover ui-state-hover
- ホバー状態の並べ替え可能な列ヘッダーに適用されるクラス。
-
ui-iggrid-colindicator
- 列ヘッダー内に描画される並べ替えインジケーター SPAN に適用されるクラス。
-
ui-iggrid-colindicator-asc ui-icon ui-icon-arrowthick-1-n
- 昇順状態の並べ替えインジケーター スパンに適用されるクラス。
-
ui-iggrid-colindicator-desc ui-icon ui-icon-arrowthick-1-s
- 降順状態の並べ替えインジケーター スパンに適用されるクラス。