ui.igGridSummaries
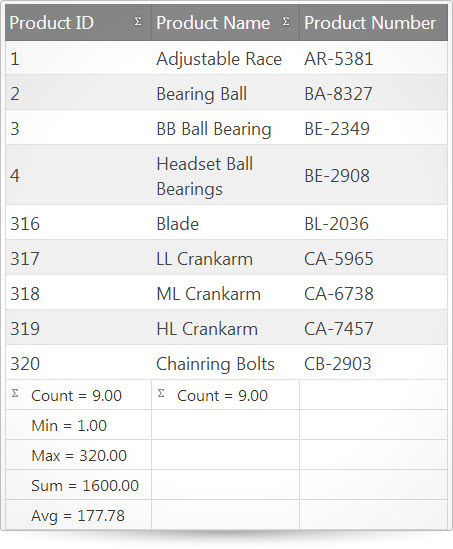
igGrid コントロールおよび igHierarchicalGrid コントロールはどちらも、グリッド内の列の集計機能を備えています。列の集計を 使用すると、最小値、最大値、平均値、合計値などの多数の集計を公開できます。この API のクラス、オプション、イベント、メソッド、およびテーマに関するさらに詳しい情報は上の関連するタブの下で入手可能です。
次のコード スニペットは、igGrid コントロールを初期化する方法を示します。
この API を使用した作業方法の詳細についてはここをクリックしてください。igGrid コントロールの必要なスクリプトおよびテーマを参照する方法については、 「Ignite UI で JavaScript リソースを使用する」および Ignite UI のスタイル設定とテーマを参照してください。コード サンプル
<!doctype html> <html> <head> <!-- Infragistics Combined CSS --> <link href= "css/themes/infragistics/infragistics.theme.css" rel= "stylesheet" type= "text/css" /> <link href= "css/structure/infragistics.css" rel= "stylesheet" type= "text/css" /> <!-- jQuery Core --> <script src= "js/jquery.js" type= "text/javascript" ></script> <!-- jQuery UI --> <script src= "js/jquery-ui.js" type= "text/javascript" ></script> <!-- Infragistics Combined Scripts --> <script src= "js/infragistics.core.js" type= "text/javascript" ></script> <script src= "js/infragistics.lob.js" type= "text/javascript" ></script> <script type= "text/javascript" > $( function () { var ds = [ { "ProductID" : 1, "Name" : "Adjustable Race" , "ProductNumber" : "AR-5381" }, { "ProductID" : 2, "Name" : "Bearing Ball" , "ProductNumber" : "BA-8327" }, { "ProductID" : 3, "Name" : "BB Ball Bearing" , "ProductNumber" : "BE-2349" }, { "ProductID" : 4, "Name" : "Headset Ball Bearings" , "ProductNumber" : "BE-2908" }, { "ProductID" : 316, "Name" : "Blade" , "ProductNumber" : "BL-2036" }, { "ProductID" : 317, "Name" : "LL Crankarm" , "ProductNumber" : "CA-5965" }, { "ProductID" : 318, "Name" : "ML Crankarm" , "ProductNumber" : "CA-6738" }, { "ProductID" : 319, "Name" : "HL Crankarm" , "ProductNumber" : "CA-7457" }, { "ProductID" : 320, "Name" : "Chainring Bolts" , "ProductNumber" : "CB-2903" } ]; $( "#gridSummaries" ).igGrid({ autoGenerateColumns: false , columns: [ { headerText: "Product ID" , key: "ProductID" , dataType: "number" }, { headerText: "Product Name" , key: "Name" , dataType: "string" }, { headerText: "Product Number" , key: "ProductNumber" , dataType: "string" } ], dataSource: ds, features: [ { name: "Summaries" , columnSettings: [ { columnKey: "ProductID" , allowSummaries: true }, { columnKey: "Name" , allowSummaries: true }, { columnKey: "ProductNumber" , allowSummaries: false } ] } ] }); }); </script> </head> <body> <table id= "gridSummaries" ></table> </body> </html> |
関連サンプル
関連トピック
依存関係
-
calculateRenderMode
- タイプ:
- enumeration
- デフォルト:
- okcancelbuttons
計算が行われる時を指定します。
メンバー
- onselect
- タイプ:string
- チェックボックスが選択または選択解除されると、集計は更新されます。
- okcancelbuttons
- タイプ:string
- [OK] ボタンをクリックしたときのみ集計は更新されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
calculateRenderMode:
"onselect"
}
]
});
//Get
var
calculateRenderMode = $(
".selector"
).igGridSummaries(
"option"
,
"calculateRenderMode"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"calculateRenderMode"
,
"onselect"
);
-
callee
- タイプ:
- function
- デフォルト:
- null
機能の参照 - データがデータ ソースから検索されるときに呼び出されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
callee:
function
() {...}
}
]
});
-
columnSettings
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
カスタム集計オプションを列ごとに指定する列設定のリスト。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
true
},
]
}
]
});
//Get
var
accessibility = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
-
allowSummaries
- タイプ:
- bool
- デフォルト:
- true
列の集計を有効または無効にします。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnIndex: 0, allowSummaries:
false
},
]
}
]
});
//Get
var
accessibility = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
var
allowSummaries = accessibility[0].allowSummaries;
//Set
//get the array of column settings
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
//set new value to the column with index 0
arrayOfColumnSettings[0].allowSummaries =
false
;
$(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
, arrayOfColumnSettings);
-
columnIndex
- タイプ:
- number
- デフォルト:
- -1
列インデックス。列キーの代わりに使用できます。列設定の生成には列を常に識別子として使用することを推奨します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnIndex: 0, allowSummaries:
false
},
]
}
]
});
-
columnKey
- タイプ:
- string
- デフォルト:
- null
列キー。これは、columnIndex が設定されていない場合に各列設定で必要なプロパティです。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
false
},
]
}
]
});
-
summaryOperands
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
defaultSummaryOperands をチェックします。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
false
, summaryOperands:
[{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"count"
,
"active"
: count,
"order"
: 0 }]
}
]
}
]
});
//Get
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
var
firstColumn = arrayOfColumnSettings[0].summaryOperands;
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
, [{ columnKey:
"ReorderPoint"
, allowSummaries:
true
, summaryOperands:
[{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"count"
,
"active"
: count,
"order"
: 0 }]
}]);
-
active
- タイプ:
- bool
- デフォルト:
- true
false の場合、集計オペランドがドロップダウンに表示されますが計算は実行されません。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
false
, summaryOperands:
[{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"count"
,
"active"
: count,
"order"
: 0 }]
}
]
}
]
});
//Get
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
var
firstColumn = arrayOfColumnSettings[0].summaryOperands;
var
active = firstColumn[0].active;
//Set
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
//Set new value
arrayOfColumnSettings[0].summaryOperands[0].active =
true
;
$(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
, arrayOfColumnSettings);
-
format
- タイプ:
- string
- デフォルト:
- ""
現在の集計オペランドに適用される書式設定を指定します。
このオプションが設定されていない場合、含まれている列の format が使用されます。
このオプションおよび列の format が設定されている場合、autoFormat オプションに基づいて領域設定が使用されます。
autoFormat オプションで列タイプを指定せず、列および集計オペランドで書式が設定されていない場合、書式設定は適用されません。コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
false
, summaryOperands:
[{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"count"
,
"active"
: count,
"order"
: 0, format:
"number"
}]
}
]
}
]
});
//Get
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
var
firstColumn = arrayOfColumnSettings[0].summaryOperands;
var
format = firstColumn[0].format;
//Set
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
//Set new value
arrayOfColumnSettings[0].summaryOperands[0].format =
"number"
;
$(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
, arrayOfColumnSettings);
-
order
- タイプ:
- number
- デフォルト:
- 5
ドロップダウン内の要素の順序を指定します。カスタム オペランドの順序を設定し、5 以上にすることを推奨します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
false
, summaryOperands:
[{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"count"
,
"active"
: count,
"order"
: 0, order: 6 }]
}
]
}
]
});
//Get
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
var
firstColumn = arrayOfColumnSettings[0].summaryOperands;
var
order = firstColumn[0].order;
//Set
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
//Set new value
arrayOfColumnSettings[0].summaryOperands[0].order = 6;
$(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
, arrayOfColumnSettings);
-
rowDisplayLabel
- タイプ:
- string
- デフォルト:
- ""
集計セルに表示される集計メソッドのテキスト。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
false
, summaryOperands:
[{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"count"
,
"active"
: count,
"order"
: 0
}]
}
]
}
]
});
//Get
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
var
firstColumn = arrayOfColumnSettings[0].summaryOperands;
var
rowDisplayLabel = firstColumn[0].rowDisplayLabel;
//Set
var
arrayOfColumnSettings = $(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
);
//Set new value
arrayOfColumnSettings[0].summaryOperands[0].rowDisplayLabel =
"New Label"
;
$(
".selector"
).igGridSummaries(
"option"
,
"columnSettings"
, arrayOfColumnSettings);
-
summaryCalculator
- タイプ:
- string
- デフォルト:
- null
タイプがカスタムのときに実行されるカスタム集計関数の名前。
コード サンプル
$(
"#grid1"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
, width: 230 },
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, width: 120 },
{ headerText:
"Reorder Point"
, key:
"ReorderPoint"
, dataType:
"number"
, width: 230 },
{ headerText:
"Product Number"
, key:
"ProductNumber"
, dataType:
"string"
, width: 120 }
],
dataSource: ds,
responseDataKey:
"responseKey"
,
features: [
{
name:
"Summaries"
,
showHeaderButton:
false
,
showDropDownButton:
true
,
compactRenderingMode:
false
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
true
,
summaryOperands:
[
{
"rowDisplayLabel"
:
"Avg"
,
"type"
:
"AVG"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"COUNT"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Min"
,
"type"
:
"MIN"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Sum of even numbers"
,
"type"
:
"custom1"
,
"active"
:
true
, summaryCalculator: $.proxy(CalculateEvenSum,
this
),
"order"
: 5},
{
"rowDisplayLabel"
:
"Sum of odd numbers"
,
"type"
:
"custom2"
,
"active"
:
true
, summaryCalculator: $.proxy(CalculateOddSum,
this
),
"order"
: 6}
]
},
{ columnKey:
"ReorderPoint"
, allowSummaries:
true
,
summaryOperands:
[
{
"rowDisplayLabel"
:
"Avg"
,
"type"
:
"AVG"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"COUNT"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Min"
,
"type"
:
"MIN"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Sum of even numbers"
,
"type"
:
"custom1"
,
"active"
:
true
, summaryCalculator: $.proxy(CalculateEvenSum,
this
),
"order"
: 5},
{
"rowDisplayLabel"
:
"Sum of odd numbers"
,
"type"
:
"custom2"
,
"active"
:
true
, summaryCalculator: $.proxy(CalculateOddSum,
this
),
"order"
: 6}
]
},
{ columnKey:
"ProductNumber"
, allowSummaries:
true
}
]
}
]
});
function
CalculateEvenSum (data) {
var
i, l = data.length, sum = 0;
for
(i = 0; i < l; i++) {
elem = data[i];
if
(elem % 2 === 0) {
sum += elem;
}
}
return
sum;
}
function
CalculateOddSum (data) {
var
i, l = data.length, sum = 0;
for
(i = 0; i < l; i++) {
elem = data[i];
if
(elem % 2 !== 0) {
sum += elem;
}
}
return
sum;
}
-
type
- タイプ:
- enumeration
- デフォルト:
- custom
集計オペランドを設定します。
メンバー
- count
- タイプ:string
- 指定された列の結果行の数を計算します。
- min
- タイプ:string
- 指定された列の結果行の最小値を計算します。
- max
- タイプ:string
- 指定された列の結果行の最大値を計算します。
- sum
- タイプ:string
- 指定された列の結果行の合計値を計算します。
- avg
- タイプ:string
- 指定された列の結果行の平均値を計算します。
- custom
- タイプ:string
- 指定された列の結果行の (summaryCalculator プロパティで指定された) カスタム関数を計算します。
コード サンプル
$(
"#grid1"
).igGrid({
autoGenerateColumns:
false
,
columns: [
{ headerText:
"Product ID"
, key:
"ProductID"
, dataType:
"number"
, width: 230 },
{ headerText:
"Product Name"
, key:
"Name"
, dataType:
"string"
, width: 120 },
{ headerText:
"Reorder Point"
, key:
"ReorderPoint"
, dataType:
"number"
, width: 230 },
{ headerText:
"Product Number"
, key:
"ProductNumber"
, dataType:
"string"
, width: 120 }
],
dataSource: ds,
responseDataKey:
"responseKey"
,
features: [
{
name:
"Summaries"
,
showHeaderButton:
false
,
showDropDownButton:
true
,
compactRenderingMode:
false
,
columnSettings: [
{ columnKey:
"ProductID"
, allowSummaries:
true
,
summaryOperands:
[
{
"rowDisplayLabel"
:
"Avg"
,
"type"
:
"AVG"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"COUNT"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Min"
,
"type"
:
"MIN"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Sum of even numbers"
,
"type"
:
"custom1"
,
"active"
:
true
, summaryCalculator: $.proxy(CalculateEvenSum,
this
),
"order"
: 5},
{
"rowDisplayLabel"
:
"Sum of odd numbers"
,
"type"
:
"custom2"
,
"active"
:
true
, summaryCalculator: $.proxy(CalculateOddSum,
this
),
"order"
: 6}
]
},
{ columnKey:
"ReorderPoint"
, allowSummaries:
true
,
summaryOperands:
[
{
"rowDisplayLabel"
:
"Avg"
,
"type"
:
"AVG"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Count"
,
"type"
:
"COUNT"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Min"
,
"type"
:
"MIN"
,
"active"
:
true
},
{
"rowDisplayLabel"
:
"Sum of even numbers"
,
"type"
:
"custom1"
,
"active"
:
true
, summaryCalculator: $.proxy(CalculateEvenSum,
this
),
"order"
: 5},
{
"rowDisplayLabel"
:
"Sum of odd numbers"
,
"type"
:
"custom2"
,
"active"
:
true
, summaryCalculator: $.proxy(CalculateOddSum,
this
),
"order"
: 6}
]
},
{ columnKey:
"ProductNumber"
, allowSummaries:
true
}
]
}
]
});
function
CalculateEvenSum (data) {
var
i, l = data.length, sum = 0;
for
(i = 0; i < l; i++) {
elem = data[i];
if
(elem % 2 === 0) {
sum += elem;
}
}
return
sum;
}
function
CalculateOddSum (data) {
var
i, l = data.length, sum = 0;
for
(i = 0; i < l; i++) {
elem = data[i];
if
(elem % 2 !== 0) {
sum += elem;
}
}
return
sum;
}
-
compactRenderingMode
- タイプ:
- enumeration
- デフォルト:
- true
集計を描画する際のサイズを指定します。true の場合、集計のコンパクトな表示が可能になり、異なる集計を同じ行に混在表示させることもできます。
false の場合、集計はタイプごとに行を変えて表示されます。
Auto に設定すると、表示可能な集計の最大数が 1 以下の場合 True を使用します。そうでない場合、False を使用します。コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
compactRenderingMode:
false
}
]
});
//Get
var
compactRenderingMode = $(
".selector"
).igGridSummaries(
"option"
,
"compactRenderingMode"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"compactRenderingMode"
,
"auto"
);
-
dialogButtonCancelText
削除- タイプ:
- string
- デフォルト:
- null
このオプションは 2017.2 バージョン以降サポートされません。
集計ドロップダウンの [キャンセル] ボタンのテキスト。
locale.dialogButtonCancelText オプションを使用します。コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
dialogButtonCancelText:
"New Value"
}
]
});
//Get
var
dialogButtonCancelText = $(
".selector"
).igGridSummaries(
"option"
,
"dialogButtonCancelText"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"dialogButtonCancelText"
,
"New Value"
);
-
dialogButtonOKText
削除- タイプ:
- string
- デフォルト:
- null
このオプションは 2017.2 バージョン以降サポートされません。
集計ドロップダウンの [OK] ボタンのテキスト。
locale.dialogButtonOKText オプションを使用します。コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
dialogButtonOKText:
"New Value"
}
]
});
//Get
var
dialogButtonOKText = $(
".selector"
).igGridSummaries(
"option"
,
"dialogButtonOKText"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"dialogButtonOKText"
,
"New Value"
);
-
dropDownDialogAnimationDuration
- タイプ:
- number
- デフォルト:
- 400
ドロップダウンのアニメーションの時間。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
dropDownDialogAnimationDuration: 1000
}
]
});
//Get
var
dropDownDialogAnimationDuration = $(
".selector"
).igGridSummaries(
"option"
,
"dropDownDialogAnimationDuration"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"dropDownDialogAnimationDuration"
, 1500);
-
dropDownHeight
- タイプ:
- number
- デフォルト:
- ""
ドロップダウンの高さ (ピクセル)。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
dropDownHeight: 250
}
]
});
//Get
var
dropDownHeight = $(
".selector"
).igGridSummaries(
"option"
,
"dropDownHeight"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"dropDownHeight"
, 350);
-
dropDownWidth
- タイプ:
- number
- デフォルト:
- ""
ドロップダウンの幅 (ピクセル)。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
dropDownWidth: 250
}
]
});
//Get
var
dropDownWidth = $(
".selector"
).igGridSummaries(
"option"
,
"dropDownWidth"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"dropDownWidth"
, 350);
-
emptyCellText
削除- タイプ:
- string
- デフォルト:
- null
このオプションは 2017.2 バージョン以降サポートされません。
空のセルに表示される空のテキスト テンプレート。
locale.emptyCellText オプションを使用します。コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
emptyCellText:
"empty cell"
}
]
});
//Get
var
emptyCellText = $(
".selector"
).igGridSummaries(
"option"
,
"emptyCellText"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"emptyCellText"
,
"empty cell"
);
-
featureChooserText
削除- タイプ:
- string
- デフォルト:
- null
このオプションは 2017.2 バージョン以降サポートされません。
集計が非表示される場合、機能セレクターのドロップダウンで表示されるテキストを取得または設定します。
locale.featureChooserText オプションを使用します。コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
featureChooserText:
"New Value"
}
]
});
//Get
var
featureChooserText = $(
".selector"
).igGridSummaries(
"option"
,
"featureChooserText"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"featureChooserText"
,
"New Value"
);
-
featureChooserTextHide
削除- タイプ:
- string
- デフォルト:
- null
このオプションは 2017.2 バージョン以降サポートされません。
集計が表示される場合、機能セレクターのドロップダウンで表示されるテキストを取得または設定します。
locale.featureChooserTextHide オプションを使用します。コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
featureChooserTextHide:
"Summaries"
}
]
});
//Get
var
text = $(
".selector"
).igGridSummaries(
"option"
,
"featureChooserTextHide"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"featureChooserTextHide"
,
"Summaries"
);
-
inherit
- タイプ:
- bool
- デフォルト:
- false
子レイアウトで機能継承を有効または無効にします。注: igHierarchicalGrid のみに適用します。
-
language
継承- タイプ:
- string
- デフォルト:
- "en"
ウィジェットのロケール言語設定を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGridSummaries({
language:
"ja"
});
// Get
var
language = $(
".selector"
).igGridSummaries(
"option"
,
"language"
);
// Set
$(
".selector"
).igGridSummaries(
"option"
,
"language"
,
"ja"
);
-
locale
- タイプ:
- object
- デフォルト:
- {}
-
dialogButtonCancelText
- タイプ:
- string
- デフォルト:
- ""
集計ドロップダウンの [キャンセル] ボタンのテキスト。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
locale: {
dialogButtonCancelText:
"New Value"
}
}
]
});
//Get
var
dialogButtonCancelText = $(
".selector"
).igGridSummaries(
"option"
,
"locale"
).dialogButtonCancelText;
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"locale"
, {
"dialogButtonCancelText"
:
"New Value"
});
-
dialogButtonOKText
- タイプ:
- string
- デフォルト:
- ""
集計ドロップダウンの [OK] ボタンのテキスト。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
locale: {
dialogButtonOKText:
"New Value"
}
}
]
});
//Get
var
dialogButtonOKText = $(
".selector"
).igGridSummaries(
"option"
,
"locale"
).dialogButtonOKText;
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"locale"
, {
"dialogButtonOKText"
:
"New Value"
});
-
emptyCellText
- タイプ:
- string
- デフォルト:
- ""
空のセルに表示される空のテキスト テンプレート。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
locale: {
emptyCellText:
"empty cell"
}
}
]
});
//Get
var
emptyCellText = $(
".selector"
).igGridSummaries(
"option"
,
"locale"
).emptyCellText;
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"locale"
, {
"emptyCellText"
:
"empty cell"
});
-
featureChooserText
- タイプ:
- string
- デフォルト:
- ""
集計が非表示される場合、機能セレクターのドロップダウンで表示されるテキストを取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
locale: {
featureChooserText:
"New Value"
}
}
]
});
//Get
var
featureChooserText = $(
".selector"
).igGridSummaries(
"option"
,
"locale"
).featureChooserText;
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"locale"
, {
"featureChooserText"
:
"New Value"
});
-
featureChooserTextHide
- タイプ:
- string
- デフォルト:
- ""
集計が表示される場合、機能セレクターのドロップダウンで表示されるテキストを取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
locale: {
featureChooserTextHide:
"Summaries"
}
}
]
});
//Get
var
text = $(
".selector"
).igGridSummaries(
"option"
,
"locale"
).featureChooserTextHide;
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"locale"
, {
"featureChooserTextHide"
:
"Summaries"
});
-
summariesHeaderButtonTooltip
- タイプ:
- string
- デフォルト:
- ""
ヘッダー セル ボタンのツールチップ テキスト。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
locale: {
summariesHeaderButtonTooltip:
"New ToolTip Text"
}
}
]
});
//Get
var
summariesHeaderButtonTooltip = $(
".selector"
).igGridSummaries(
"option"
,
"locale"
).summariesHeaderButtonTooltip;
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"locale"
, {
"summariesHeaderButtonTooltip"
:
"New ToolTip Text"
});
-
regional
継承- タイプ:
- enumeration
- デフォルト:
- defaults
ウィジェットの領域設定を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igGridSummaries({
regional:
"ja"
});
// Get
var
regional = $(
".selector"
).igGridSummaries(
"option"
,
"regional"
);
// Set
$(
".selector"
).igGridSummaries(
"option"
,
"regional"
,
"ja"
);
-
renderSummaryCellFunc
- タイプ:
- enumeration
- デフォルト:
- null
javascript 関数の参照または名前。集計セルをグリッド フッターに描画します。設定されない場合、デフォルトの描画関数を使用します。3 つのパラメーターを受信します - methodName(summary method)、columnKey、data(描画する集計データ)。
コード サンプル
<script type=
"text/javascript"
>
function
renderSummaryCell(methodName, columnKey, data) {
var
res = data.rowDisplayLabel +
" - "
+ data.result,
sContainerId =
"#"
+
this
.grid.element[0].id +
"_summaries_footer_row_text_container_"
;
this
.grid.container()
.find(sContainerId + methodName +
"_"
+ columnKey)
.html(res);
}
</script>
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
renderSummaryCellFunc: renderSummaryCell
}
}
]
});
//Get
var
showDropDownButton = $(
".selector"
).igGridSummaries(
"option"
,
"renderSummaryCellFunc"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"renderSummaryCellFunc"
, renderSummaryCell);
-
resultTemplate
- タイプ:
- string
- デフォルト:
- "{0} = {1}"
集計結果の結果テンプレート (テーブル セル内に表示される)。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
resultTemplate:
"{0} is {1}"
}
]
});
//Get
var
resultTemplate = $(
".selector"
).igGridSummaries(
"option"
,
"resultTemplate"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"resultTemplate"
,
"{0} is {1}"
);
-
showDropDownButton
- タイプ:
- bool
- デフォルト:
- true
フッター ボタンの表示/非表示 (表示/非表示ドロップダウンのクリック時)。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
showDropDownButton:
false
}
]
});
//Get
var
showDropDownButton = $(
".selector"
).igGridSummaries(
"option"
,
"showDropDownButton"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"showDropDownButton"
,
false
);
-
showSummariesButton
- タイプ:
- bool
- デフォルト:
- true
ヘッダーのセルにヘッダー ボタン アイコンを表示します。クリック時 - 集計を表示/非表示します。 false の場合、集計アイコンは機能セレクターに表示されません。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
showSummariesButton:
false
}
]
});
//Get
var
showSummariesButton = $(
".selector"
).igGridSummaries(
"option"
,
"showSummariesButton"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"showSummariesButton"
,
false
);
-
summariesHeaderButtonTooltip
削除- タイプ:
- string
- デフォルト:
- null
このオプションは 2017.2 バージョン以降サポートされません。
ヘッダー セル ボタンのツールチップ テキスト。
locale.summariesHeaderButtonTooltip オプションを使用します。コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
summariesHeaderButtonTooltip:
"New ToolTip Text"
}
]
});
//Get
var
summariesHeaderButtonTooltip = $(
".selector"
).igGridSummaries(
"option"
,
"summariesHeaderButtonTooltip"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"summariesHeaderButtonTooltip"
,
"New ToolTip Text"
);
-
summariesResponseKey
- タイプ:
- string
- デフォルト:
- "summaries"
リモート データ ソースによって返された結果からデータを取得する結果キー。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
summariesResponseKey:
"summaries"
}
]
});
//Get
var
summariesResponseKey = $(
".selector"
).igGridSummaries(
"option"
,
"summariesResponseKey"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"summariesResponseKey"
,
"summaries"
);
-
summaryExecution
- タイプ:
- enumeration
- デフォルト:
- afterfilteringbeforepaging
タイプがローカルのとき集計値をいつ計算するかを決定します。
メンバー
- priortofilteringandpaging
- タイプ:string
- 集計は、フィルタリングとページングの前に計算されます。
- afterfilteringbeforepaging
- タイプ:string
- 集計は、フィルタリングの後、ページングの前に計算されます。
- afterfilteringandpaging
- タイプ:string
- 集計は、フィルタリングとページングの後に計算されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
summaryExecution:
"priortofilteringandpaging"
}
]
});
//Get
var
summaryExecution = $(
".selector"
).igGridSummaries(
"option"
,
"summaryExecution"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"summaryExecution"
,
"priortofilteringandpaging"
);
-
summaryExprUrlKey
- タイプ:
- string
- デフォルト:
- "summaries"
集計の GET 要求にキーを設定します (タイプがリモートの場合にのみ使用)。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
summaryExprUrlKey:
"summaries"
}
]
});
//Get
var
summaryExprUrlKey = $(
".selector"
).igGridSummaries(
"option"
,
"summaryExprUrlKey"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"summaryExprUrlKey"
,
"summaries"
);
-
type
- タイプ:
- enumeration
- デフォルト:
- null
集計計算のタイプ。
メンバー
- remote
- タイプ:string
- リモートのときは、集計計算はサーバーで実行されます。
- local
- タイプ:string
- ローカルのときは、計算はクライアントで実行されます。
コード サンプル
//Initialize
$(
".selector"
).igGrid({
features: [
{
name:
"Summaries"
,
type:
"remote"
}
]
});
//Get
var
type = $(
".selector"
).igGridSummaries(
"option"
,
"type"
);
//Set
$(
".selector"
).igGridSummaries(
"option"
,
"type"
,
"remote"
);
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
dropDownCancelClicked
- キャンセル可能:
- false
ドロップダウンで [キャンセル] ボタンがクリックされたときに発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariesdropdowncancelclicked"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get the column key, for which Cancel button is clicked
ui.columnKey;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
dropDownCancelClicked:
function
(evt, ui){ ... }
}
]
});
-
dropDownClosed
- キャンセル可能:
- false
集計列のドロップダウンを閉じた後に発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariesdropdownclosed"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get the column key, for which Cancel button is clicked
ui.columnKey;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
dropDownClosed:
function
(evt, ui){ ... }
}
]
});
-
dropDownClosing
- キャンセル可能:
- true
集計列のドロップダウンが閉じ始める前に発生するイベント。
ドロップダウンを閉じるのをキャンセルするには、false を返します。コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariesdropdownclosing"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get the column key, for which Cancel button is clicked
ui.columnKey;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
dropDownClosing:
function
(evt, ui){ ... }
}
]
});
-
dropDownOKClicked
- キャンセル可能:
- false
ドロップダウンで [OK] ボタンがクリックされたときに発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariesdropdownokclicked"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get the column key, for which Cancel button is clicked
ui.columnKey;
// get data about which summary methods are(not) selected. Format of event data is {type: "min", active: false};
ui.eventData;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
dropDownOKClicked:
function
(evt, ui){ ... }
}
]
});
-
dropDownOpened
- キャンセル可能:
- false
特定の列の集計ドロップダウンが開いた後に発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariesdropdownopened"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get the column key, for which Cancel button is clicked
ui.columnKey;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
dropDownOpened:
function
(evt, ui){ ... }
}
]
});
-
dropDownOpening
- キャンセル可能:
- true
特定の列の集計に対してドロップダウンが開く前に発生するイベント。
ドロップダウンを開くのをキャンセルするには、false を返します。コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariesdropdownopening"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get the column key, for which Cancel button is clicked
ui.columnKey;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
dropDownOpening:
function
(evt, ui){ ... }
}
]
});
-
summariesCalculated
- キャンセル可能:
- false
集計の計算が完了した後に発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariessummariescalculated"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get data for calculated summaries
ui.data;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
summariesCalculated:
function
(evt, ui){ ... }
}
]
});
-
summariesCalculating
- キャンセル可能:
- true
集計の計算が行われる前に発生するイベント。
集計の計算をキャンセルするには、false を返します。コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariessummariescalculating"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
summariesCalculating:
function
(evt, ui){ ... }
}
]
});
-
summariesMethodSelectionChanged
- キャンセル可能:
- false
ユーザーがチェックボックスから集計メソッドを選択/選択解除するときに発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariessummariesmethodselectionchanged"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get the column key, for which Cancel button is clicked
ui.columnKey;
// get whether method is selected or not
ui.isSelected;
// get summary method name
ui.methodName;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
summariesMethodSelectionChanged:
function
(evt, ui){ ... }
}
]
});
-
summariesToggled
- キャンセル可能:
- false
集計行が切り替えられた後に発生するイベント。
コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariessummariestoggled"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get whether summaries are shown or not
ui.isToShow;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
summariesToggled:
function
(evt, ui){ ... }
}
]
});
-
summariesToggling
- キャンセル可能:
- true
集計行の切り替えが開始する前に発生するイベント。
集計の表示/非表示をキャンセルするには、false を返します。コード サンプル
//Bind after initialization
$(document).on(
"iggridsummariessummariestoggling"
,
".selector"
,
function
(evt, ui) {
//return the triggered event
evt;
// get whether summaries are shown or not
ui.isToShow;
// access the igGridSummaries widget object
ui.owner;
});
//Initialize
$(
".selector"
).igGrid({
features : [
{
name :
"Summaries"
,
summariesToggling:
function
(evt, ui){ ... }
}
]
});
-
calculateSummaries
- .igGridSummaries( "calculateSummaries" );
集計を計算します。
コード サンプル
$(
"#grid1"
).igGridSummaries(
"calculateSummaries"
);
-
calculateSummaryColumn
- .igGridSummaries( "calculateSummaryColumn", ck:string, columnMethods:array, data:object, dataType:object );
集計は、指定した列キー、columnMethods および dataType のデータ全体を計算します (データソースがリモートであり、dataType が日付または時間のときに使用される)。
- ck
- タイプ:string
- ColumnKey。
- columnMethods
- タイプ:array
- 列メソッド オブジェクトの配列。
- data
- タイプ:object
- 結果を表すオブジェクト。
現在の列の dataType を表します。 - dataType
- タイプ:object
コード サンプル
$(
"#grid1"
).igGridSummaries(
"calculateSummaryColumn"
,
"ProductID"
, [{type:
"min"
, active:
true
, rowDisplayLabel:
"Min"
, format:
"number"
}], [{
"ProductID"
: 1}, {
"ProductID"
: 2}],
"number"
);
-
changeLocale
- .igGridSummaries( "changeLocale" );
ウィジェット要素のすべてのロケールを options.language に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。コード サンプル
$(
".selector"
).igGridSummaries(
"changeLocale"
);
-
changeRegional
- .igGridSummaries( "changeRegional" );
ウィジェット要素の地域設定を options.regional に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。regional オプションのセッターを使用してください。コード サンプル
$(
".selector"
).igGridSummaries(
"changeRegional"
);
-
clearAllFooterIcons
- .igGridSummaries( "clearAllFooterIcons" );
すべての集計ドロップダウン ボタンを削除します。
コード サンプル
$(
"#grid1"
).igGridSummaries(
"clearAllFooterIcons"
);
-
destroy
- .igGridSummaries( "destroy" );
コード サンプル
$(
"#grid1"
).igGridSummaries(
"destroy"
);
-
isSummariesRowsHidden
- .igGridSummaries( "isSummariesRowsHidden" );
集計行が非表示かどうかを返します。
コード サンプル
$(
"#grid1"
).igGridSummaries(
"isSummariesRowsHidden"
);
-
selectCheckBox
- .igGridSummaries( "selectCheckBox", $checkbox:object, isToSelect:bool );
指定されたチェックボックスを選択/選択解除します。
- $checkbox
- タイプ:object
- チェックボックスの jQuery オブジェクトを指定します。
- isToSelect
- タイプ:bool
- チェックボックスを選択するかどうかを指定します。
コード サンプル
$(
"#grid1"
).igGridSummaries(
"selectCheckBox"
, $(
"#checkboxSelector"
),
true
);
-
showHideDialog
- .igGridSummaries( "showHideDialog", $dialog:object );
ダイアログを表示/非表示します。
- $dialog
- タイプ:object
- ドロップダウン DIV 要素の jQuery オブジェクト表現。
コード サンプル
$(
"#grid1"
).igGridSummaries(
"showHideDialog"
, $(
"#dialogSelector"
));
-
summariesFor
- .igGridSummaries( "summariesFor", columnKey:object );
指定された列キーを持つ列の全ての集計を保持する JQUERY オブジェクトを返します。
- columnKey
- タイプ:object
コード サンプル
var
summariesResiltByKey = $(
"#grid1"
).igGridSummaries(
"summariesFor"
,
"ProductID"
);
-
summaryCollection
- .igGridSummaries( "summaryCollection" );
すべての列の全集計を保持する JQUERY オブジェクトを返します。
コード サンプル
var
collection = $(
"#grid1"
).igGridSummaries(
"summaryCollection"
);
-
toggleCheckstate
- .igGridSummaries( "toggleCheckstate", $checkbox:object );
checkboxMode が off に設定されていない場合に、チェックボックスのチェック状態を切り替えます。それ以外の場合は何も行いません。
- $checkbox
- タイプ:object
- チェックボックスの jQuery オブジェクトを指定します。
コード サンプル
$(
"#grid1"
).igGridSummaries(
"toggleCheckstate"
, $(
"#checkboxSelector"
));
-
toggleDropDown
- .igGridSummaries( "toggleDropDown", columnKey:string, event:object );
ドロップダウンを切り替えます。
- columnKey
- タイプ:string
- 指定したキーのある列でドロップダウンを切り替えます。
- event
- タイプ:object
- イベント オブジェクト。そのデータは、現在の columnKey、isAnimating、および buttonId を含む必要があります。
コード サンプル
$(
"#grid1"
).igGridSummaries(
"toggleDropDown"
,
null
);
-
toggleSummariesRows
- .igGridSummaries( "toggleSummariesRows", isToShow:bool, isInternalCall:bool );
集計行を切り替えます。
- isToShow
- タイプ:bool
- 集計を表示するかどうかを指定します。
- isInternalCall
- タイプ:bool
- オプションのパラメーター。この関数がウィジェットで内部に呼び出されるかどうかを指定します。
コード サンプル
$(
"#grid1"
).igGridSummaries(
"toggleSummariesRows"
,
true
,
null
);
-
ui-ie7
- IE7 のクラス。
-
ui-state-default ui-corner-all ui-igcheckbox-normal
- チェックボックス コンテナーに適用されるクラス。
-
ui-state-hover
- チェックボックスのホバー状態のスタイルを定義するクラス。
-
ui-icon ui-icon-check ui-igcheckbox-normal-off
- チェックボックスのチェックされていない状態を定義するクラス。
-
ui-icon ui-icon-check ui-igcheckbox-normal-on
- チェックボックスのチェックされた状態を定義するクラス。
-
ui-iggrid-summaries-dialog
- ドロップダウンに適用されるクラス。
-
ui-iggrid-summaries-dropdown-listcontainer ui-widget
- ドロップダウンの全てのチェックボックスを保持するコンテナーに適用されるクラス。
-
ui-iggrid-summaries-dialog-listitem
- ドロップダウンのリスト項目に適用されるクラス。
-
ui-icon ui-iggrid-icon-summaries
- 機能チューザーに表示されるアイコンのクラス。
-
ui-icon ui-icon-calculator ui-iggrid-icon-summaries
- (ドロップダウンを表示/非表示にする) フッター ボタン アイコンに適用されるクラス。
-
ui-iggrid-filterbuttonactive ui-state-active
- 選択された (ドロップダウンが開いた) ときにフッター ボタン アイコンに適用されるクラス.。
-
ui-iggrid-filterbuttonhover ui-state-hover
- (ボタンの表示/非表示ドロップダウンで) ホバーされたときにフッター ボタン アイコンに適用されるクラス。
-
ui-iggrid-summaries-footer-dialog-buttons-container
- ドロップダウンの [OK]/[キャンセル] ボタンのコンテナーに適用されるクラス。
-
ui-widget-footer ui-state-default
- フッター行のセルに適用されるクラス。
-
ui-state-hover
- ホバー状態のときに、フッター行のセルに適用されるクラス。
-
ui-corner-all ui-iggrid-summaries-footer-icon-container
- 集計セル内のアイコンのテキストを含む DOM 要素に適用されるクラス。
-
ui-iggrid-summaries-footer-icon-container-empty
- showSummariesButton が false の場合に DOM 要素に適用されるクラス。
-
ui-iggrid-summaries-footer-text-container
- 集計結果のテキストを含む集計セル内の DOM 要素に適用されるクラス。
-
ui-iggrid-summaries-footer-text-container-empty
- showSummariesButton が false の場合、集計結果のテキストを含む集計セル内の DOM 要素に適用されるクラス。
-
ui-iggrid-summaries-headerbuttoncontainer ui-corner-all
- (集計を表示/非表示にする) ヘッダー ボタンのコンテナー div に適用されるクラス。
-
ui-iggrid-summaries-header-icon ui-corner-all ui-icon ui-icon-calculator ui-icon-summaries
- (集計を表示/非表示にする) ヘッダー ボタンに適用されるクラス。
-
ui-iggrid-summaries-header-icon-hover ui-state-hover
- ホバー状態のときに (集計を表示/非表示にする) ヘッダー ボタンに適用されるクラス。