ui.igHtmlEditor
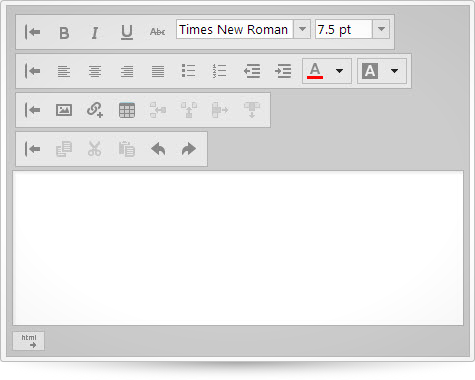
igHtmlEditor コントロールは標準 HTML 編集機能が特長の jQuery HTML エディター コントロールです。書式設定オプションには、フォント フェイス、フォント サイズ、テキストおよび画像の配置、リンク、およびテーブル サポートもあります。この API のクラス、オプション、イベント、メソッド、およびテーマの詳細は、上記の関連するタブの下に表示されます。
次のコード スニペットは igHtmlEditor コントロールの初期化方法を示しています。
igHtmlEditor コントロールに必要なスクリプトおよびテーマの参照方法についての詳細は、 Ignite UI での JavaScript リソースの使用および Ignite UI のスタイルとテーマの設定をお読みください。コード サンプル
<!doctype html> <html> <head> <!-- Modernizr --> <script src= "js/modernizr.js" ></script> <!-- jQuery Core --> <script src= "js/jquery.js" ></script> <!-- jQuery UI --> <script src= "js/jquery-ui.js" ></script> <!-- Infragistics loader --> <script src= "js/infragistics.loader.js" ></script> <script> $.ig.loader({ scriptPath: "js" , cssPath: "css" , resources: "igHtmlEditor" }); $.ig.loader( function () { $( "#htmlEditor" ).igHtmlEditor({ width: "100%" }); }); </script> </head> <body> <div id= "htmlEditor" ></div> </body> </html> |
関連サンプル
関連トピック
依存関係
-
customToolbars
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
HTML エディター カスタム ツールバー リスト。
コード サンプル
// Initialize
$(
".selector"
).igHtmlEditor({
customToolbars: [{
name:
"customToolbar"
,
collapseButtonIcon:
"ui-igbutton-collapse"
,
expandButtonIcon:
"ui-igbutton-expand"
,
items: [{
//Definition for custom button
name:
"customButton"
,
type:
"button"
,
handler:
function
() {
alert(
"Custom button clicked!"
);
},
scope:
this
,
props: {
isImage: {
value:
false
,
action:
'_isSelectedAction'
},
imageButtonTooltip: {
value:
"Custom button tooltip"
,
action:
'_tooltipAction'
},
imageButtonIcon: {
value:
"ui-igbutton-bold"
,
action:
'_buttonIconAction'
}
}
}, {
//Definition for custom combo
name:
"customCombo"
,
type:
"combo"
,
handler:
function
(el, obj) {
alert(
"Selected item is: "
+ obj.value);
},
scope:
this
,
props: {
customComboWidth: {
value: 115,
action:
"_comboWidthAction"
},
customComboHeight: {
value:
""
,
action:
"_comboHeightAction"
},
customComboItemsListWidth: {
value: 115,
action:
"_comboDropDownListWidth"
},
customComboSource: {
value: [{
text:
"Item 1"
,
value:
"Item 1"
}, {
text:
"Item 2"
,
value:
"Item 2"
}],
action:
"_comboDataSourceAction"
},
selectedCustomComboItem: {
value:
"Item 2"
,
action:
"_comboSelectedItem"
}
}
}]
}]
});
//Get
var
customToolbar = $(
".selector"
).igHtmlEditor(
"option"
,
"customToolbars"
);
-
height
- タイプ:
- enumeration
- デフォルト:
- 350
HTML エディターの高さ。ピクセル、文字列 (px)、またはパーセンテージ (%) を数字で設定できます。
メンバー
- string
- ウィジェットの高さはピクセル (px) およびパーセント (%) で設定できます (%)。
- number
- ウィジェットの高さは数値として設定できます。
コード サンプル
// Initialize
$(
".selector"
).igHtmlEditor({
height: 100
// or
// height: "100%"
});
//Get
var
height = $(
".selector"
).igHtmlEditor(
"option"
,
"height"
);
// Set
$(
".selector"
).igHtmlEditor(
"option"
,
"height"
, 300);
-
inputName
- タイプ:
- string
- デフォルト:
- "source"
HTML エディターのソース ビューの name 属性。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
inputName:
"blogContent"
});
//Get
var
inputName = $(
".selector"
).igHtmlEditor(
"option"
,
"inputName"
);
-
language
継承- タイプ:
- string
- デフォルト:
- "en"
ウィジェットのロケール言語設定を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
language:
"ja"
});
// Get
var
language = $(
".selector"
).igHtmlEditor(
"option"
,
"language"
);
// Set
$(
".selector"
).igHtmlEditor(
"option"
,
"language"
,
"ja"
);
-
locale
継承- タイプ:
- object
- デフォルト:
- null
ウィジェットのロケール設定を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
locale: {}
});
// Get
var
locale = $(
".selector"
).igHtmlEditor(
"option"
,
"locale"
);
// Set
$(
".selector"
).igHtmlEditor(
"option"
,
"locale"
, {});
-
regional
継承- タイプ:
- enumeration
- デフォルト:
- defaults
ウィジェットの領域設定を取得または設定します。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
regional:
"ja"
});
// Get
var
regional = $(
".selector"
).igHtmlEditor(
"option"
,
"regional"
);
// Set
$(
".selector"
).igHtmlEditor(
"option"
,
"regional"
,
"ja"
);
-
showCopyPasteToolbar
- タイプ:
- bool
- デフォルト:
- true
「コピー/貼り付け」ツールバーを表示/非表示にします。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
showCopyPasteToolbar:
false
});
//Get
var
showCopyPasteToolbar = $(
".selector"
).igHtmlEditor(
"option"
,
"showCopyPasteToolbar"
);
//Set
$(
".selector"
).igHtmlEditor(
"option"
,
"showCopyPasteToolbar"
,
true
);
-
showFormattingToolbar
- タイプ:
- bool
- デフォルト:
- true
"フォーマッティング" ツールバーを表示/非表示にします。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
showFormattingToolbar:
false
});
//Get
var
showFormattingToolbar = $(
".selector"
).igHtmlEditor(
"option"
,
"showFormattingToolbar"
);
//Set
$(
".selector"
).igHtmlEditor(
"option"
,
"showFormattingToolbar"
,
true
);
-
showInsertObjectToolbar
- タイプ:
- bool
- デフォルト:
- true
「オブジェクトの挿入」ツールバーを表示/非表示にします。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
showInsertObjectToolbar:
false
});
//Get
var
showInsertObjectToolbar = $(
".selector"
).igHtmlEditor(
"option"
,
"showInsertObjectToolbar"
);
//Set
$(
".selector"
).igHtmlEditor(
"option"
,
"showInsertObjectToolbar"
,
true
);
-
showTextToolbar
- タイプ:
- bool
- デフォルト:
- true
「テキスト」ツールバーを表示/非表示にします。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
showTextToolbar:
false
});
//Get
var
showTextToolbar = $(
".selector"
).igHtmlEditor(
"option"
,
"showTextToolbar"
);
//Set
$(
".selector"
).igHtmlEditor(
"option"
,
"showTextToolbar"
,
true
);
-
toolbarSettings
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
HTML エディター ツールバー リスト。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
toolbarSettings: [
{
name:
"textToolbar"
,
isExpanded:
false
}]
});
//Get
var
toolbarSettings = $(
".selector"
).igHtmlEditor(
"option"
,
"toolbarSettings"
);
-
value
- タイプ:
- string
- デフォルト:
- ""
HTML エディター内に最初コンテンツとして描画するために使用されます。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
value:
"Hello World!"
});
//Get
var
value = $(
".selector"
).igHtmlEditor(
"option"
,
"value"
);
//Set
$(
".selector"
).igHtmlEditor(
"option"
,
"value"
,
"Hello World!"
);
-
width
- タイプ:
- enumeration
- デフォルト:
- 725
HTML エディターの幅。ピクセル、文字列 (px)、またはパーセンテージ (%) を数字で設定できます。
メンバー
- string
- ウィジェットの幅をピクセル (px) またはパーセンテージ (%) に設定できます。
- number
- ウィジェット幅は数値として設定できます。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
width: 700
//or
//width: "100%"
});
//Get
var
width = $(
".selector"
).igHtmlEditor(
"option"
,
"width"
);
// Set
$(
".selector"
).igHtmlEditor(
"option"
,
"width"
, 300);
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
actionExecuted
- キャンセル可能:
- false
ツールバー項目がクリックされた後に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
actionExecuted:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditoractionexecuted"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
//return toolbar name
ui.toolbar;
//return action name
ui.actionName;
});
-
actionExecuting
- キャンセル可能:
- true
ツールバー項目がクリックされる前に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
actionExecuting:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditoractionexecuting"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
//return toolbar name
ui.toolbar;
//return action name
ui.actionName;
});
-
copy
- キャンセル可能:
- false
キーボードのコピー アクションで発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
copy:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditorcopy"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
});
-
cut
- キャンセル可能:
- false
キーボードの切り取りアクションで発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
cut:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditorcut"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
});
-
paste
- キャンセル可能:
- false
キーボードの貼り付けアクションで発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
paste:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditorpaste"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
});
-
redo
- キャンセル可能:
- false
キーボードのやり直しアクションで発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
redo:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditorredo"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
});
-
rendered
- キャンセル可能:
- false
HTML エディター ウィジェットが描画された後に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
rendered:
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
}
});
//Bind after initialization
$(document).on(
"ightmleditorrendered"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
});
-
rendering
- キャンセル可能:
- false
HTML エディター ウィジェットが描画される前に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
rendering:
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
}
});
//Bind after initialization
$(document).on(
"ightmleditorrendering"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
});
-
toolbarCollapsed
- キャンセル可能:
- false
ツールバーが縮小された後に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
toolbarCollapsed:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditortoolbarcollapsed"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
//return reference to toolbar object
ui.toolbar
//return reference to toolbar element object
ui.toolbarElement
});
-
toolbarCollapsing
- キャンセル可能:
- true
ツールバーが縮小される前に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
toolbarCollapsing:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditortoolbarcollapsing"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
//return reference to toolbar object
ui.toolbar
//return reference to toolbar element object
ui.toolbarElement
});
-
toolbarExpanded
- キャンセル可能:
- false
ツールバーが展開された後に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
toolbarExpanding:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditortoolbarexpanding"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
//return reference to toolbar object
ui.toolbar
//return reference to toolbar element object
ui.toolbarElement
});
-
toolbarExpanding
- キャンセル可能:
- true
ツールバーが展開される前に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
toolbarExpanded:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditortoolbarexpanded"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
//return reference to toolbar object
ui.toolbar
//return reference to toolbar element object
ui.toolbarElement
});
-
undo
- キャンセル可能:
- false
キーボードの取り消しアクションで発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
undo:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditorundo"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
});
-
workspaceResized
- キャンセル可能:
- false
ワークスペースのサイズ変更後に発生するイベント。
コード サンプル
//Initialize
$(
".selector"
).igHtmlEditor({
workspaceResized:
function
(evt, ui) {...}
});
//Bind after initialization
$(document).on(
"ightmleditorworkspaceresized"
,
".selector"
,
function
(evt, ui) {
//return reference to igHtmlEditor object
ui.owner
});
-
changeGlobalLanguage
継承- .igHtmlEditor( "changeGlobalLanguage" );
ウィジェットの言語をグローバルの言語に変更します。グローバルの言語は $.ig.util.language の値です。
コード サンプル
$(
".selector"
).igHtmlEditor(
"changeGlobalLanguage"
);
-
changeGlobalRegional
継承- .igHtmlEditor( "changeGlobalRegional" );
ウィジェットの地域設定をグローバルの地域設定に変更します。グローバルの地域設定は $.ig.util.regional にあります。
コード サンプル
$(
".selector"
).igHtmlEditor(
"changeGlobalRegional"
);
-
changeLocale
- .igHtmlEditor( "changeLocale" );
ウィジェット要素のすべてのロケールを options.language に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。コード サンプル
$(
".selector"
).igHtmlEditor(
"changeLocale"
);
-
contentDocument
- .igHtmlEditor( "contentDocument" );
- 返却型:
- object
- 返却型の説明:
- HTML エディターのコンテンツの編集可能な領域と関連されるドキュメント オブジェクト。
HTML エディターのコンテンツの編集可能な領域と関連されるドキュメント オブジェクトを返します。
コード サンプル
var
htmlEditorDocument = $(
".selector"
).igHtmlEditor(
"contentDocument"
);
-
contentEditable
- .igHtmlEditor( "contentEditable" );
- 返却型:
- object
- 返却型の説明:
- この HTML エディターに関連する編集可能なコンテンツ。
この HTML エディターに関連する編集可能なコンテンツを返します。
コード サンプル
var
htmlEditorContentEditable = $(
".selector"
).igHtmlEditor(
"contentEditable"
);
-
contentWindow
- .igHtmlEditor( "contentWindow" );
- 返却型:
- object
- 返却型の説明:
- HTML エディターのコンテンツの編集可能な領域と関連されるウィンドウ オブジェクト。
HTML エディターのコンテンツの編集可能な領域と関連されるウィンドウ オブジェクトを返します。
コード サンプル
var
htmlEditorContentWindow = $(
".selector"
).igHtmlEditor(
"contentWindow"
);
-
destroy
- .igHtmlEditor( "destroy" );
ウィジェットを破棄します。
コード サンプル
$(
".selector"
).igHtmlEditor(
"destroy"
);
-
executeAction
- .igHtmlEditor( "executeAction", actionName:string, [args:object] );
htmleditor コマンドを実行します。
- actionName
- タイプ:string
- コマンドの名前。
- args
- タイプ:object
- オプション
- コマンドの追加のパラメーター。
コード サンプル
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"increasefontsize"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"bold"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"italic"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"underline"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"strikethrough"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"decreasefontsize"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"fontname"
,
"Lucida Console"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"fontname"
,
"Verdana"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"fontsize"
,
"5"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"formatBlock"
,
"h6"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"forecolor"
,
"blue"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"backcolor"
,
"red"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"justifyright"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"justifycenter"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"justifyleft"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"justifyfull"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"insertunorderedlist"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"insertorderedlist"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"outdent"
);
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"indent"
);
//Works on Internet Explorer only
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"cut"
);
//Works on Internet Explorer only
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"copy"
);
//Works on Internet Explorer only
$(
".selector"
).igHtmlEditor(
"executeAction"
,
"paste"
);
-
getContent
- .igHtmlEditor( "getContent", format:string );
- 返却型:
- string
- 返却型の説明:
- エディターのコンテンツ。
HTML エディターのコンテンツを取得します。
- format
- タイプ:string
- コンテンツを HTML またはプレーン テキストとして返します。値は「text」または「html」が可能です。
コード サンプル
var
plainContent = $(
".selector"
).igHtmlEditor(
"getContent"
,
"text"
);
var
htmlContent = $(
".selector"
).igHtmlEditor(
"getContent"
,
"html"
);
-
insertAtCaret
- .igHtmlEditor( "insertAtCaret", element:object );
提供したコンテンツをカレットの位置に挿入します。
- element
- タイプ:object
- HTML 文字列、DOM 要素、または jQuery オブジェクトを受けます。
コード サンプル
$(
".selector"
).igHtmlEditor(
"insertAtCaret"
,
"<div />"
);
-
isDirty
- .igHtmlEditor( "isDirty" );
エディター コンテンツが変更した場合に True を返します。変更なしの場合、False を返します。
コード サンプル
var
isDirty = $(
".selector"
).igHtmlEditor(
"isDirty"
);
-
range
- .igHtmlEditor( "range" );
- 返却型:
- object
- 返却型の説明:
- 編集可能なコンテンツの現在範囲を表す Range オブジェクトを返します。
編集可能なコンテンツの現在範囲を表す Range オブジェクトを返します。
コード サンプル
var
range = $(
".selector"
).igHtmlEditor(
"range"
);
-
resizeWorkspace
- .igHtmlEditor( "resizeWorkspace" );
ワークスペースの高さをサイズ変更します。
コード サンプル
$(
".selector"
).igHtmlEditor(
"resizeWorkspace"
);
-
selection
- .igHtmlEditor( "selection" );
- 返却型:
- object
- 返却型の説明:
- 編集可能なコンテンツの現在選択を表す Selection オブジェクトを返します。
編集可能なコンテンツの現在選択を表す Selection オブジェクトを返します。
コード サンプル
var
selection = $(
".selector"
).igHtmlEditor(
"selection"
);
-
setContent
- .igHtmlEditor( "setContent", content:string, format:string );
HTML エディターのコンテンツを設定します。
- content
- タイプ:string
- 設定するコンテンツ。
- format
- タイプ:string
- コンテンツ タイプ: 「text」または「html」。
コード サンプル
$(
".selector"
).igHtmlEditor(
"setContent"
,
"Hello World!"
,
"text"
);
$(
".selector"
).igHtmlEditor(
"setContent"
,
"<h1>Hello World!</h1>"
,
"html"
);
-
widget
- .igHtmlEditor( "widget" );
ウィジェットがインスタンス化された要因を返します。
コード サンプル
var
widget = $(
".selector"
).igHtmlEditor(
"widget"
);
-
ui-widget ui-widget-content ui-corner-all ui-ightmleditor ui-helper-clearfix
- ウィジェット基本クラス css。
-
ui-ightmleditor-content
- HTML エディター コンテンツ スタイル。
-
ui-igpathfinder
- HTML エディター DOM ナビゲーション ツールバー css。
-
ui-igtoolbars-holder
- HTML エディター ツールバー スタイル。
-
ui-widget-content
- ワークスペース css。