ui.igTreeGridFiltering
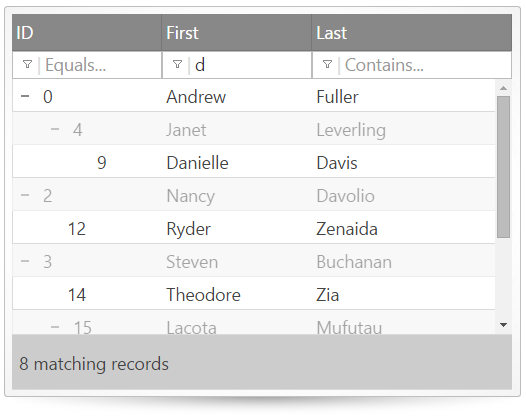
igTreeGrid は、一致する行のコンテキストを表示するフィルター機能があります。フィルター オプションは、数値、文字列、日付フィルターを含み、子行を含むか、含まないで一致を結果で表示できます。この API のクラス、オプション、イベント、メソッドおよびテーマに関する詳細は、上記の関連するタブを参照してください。
以下のコード スニペットは、igTreeGrid コントロールを初期化する方法を示します。
この API を使用して作業を開始するための情報はここをクリックしてください。igGrid コントロールの必要なスクリプトおよびテーマを参照する方法については、 「Ignite UI で JavaScript リソースを使用する」および「Ignite UI のスタイル設定とテーマ」を参照してください。
コード サンプル
<!DOCTYPE html> <html> <head> <!-- Infragistics Combined CSS --> <link href="css/themes/infragistics/infragistics.theme.css" rel="stylesheet" type="text/css" /> <link href="css/structure/infragistics.css" rel="stylesheet" type="text/css" /> <!-- jQuery Core --> <script src="js/jquery.js" type="text/javascript"></script> <!-- jQuery UI --> <script src="js/jquery-ui.js" type="text/javascript"></script> <!-- Infragistics Combined Scripts --> <script src="js/infragistics.core.js" type="text/javascript"></script> <script src="js/infragistics.lob.js" type="text/javascript"></script> <script type="text/javascript"> var employees = [ { "employeeId": 0, "supervisorId": -1, "firstName": "Andrew", "lastName": "Fuller" }, { "employeeId": 1, "supervisorId": -1, "firstName": "Jonathan", "lastName": "Smith" }, { "employeeId": 2, "supervisorId": -1, "firstName": "Nancy", "lastName": "Davolio" }, { "employeeId": 3, "supervisorId": -1, "firstName": "Steven", "lastName": "Buchanan" }, // Andrew Fuller's direct reports { "employeeId": 4, "supervisorId": 0, "firstName": "Janet", "lastName": "Leverling" }, { "employeeId": 5, "supervisorId": 0, "firstName": "Laura", "lastName": "Callahan" }, { "employeeId": 6, "supervisorId": 0, "firstName": "Margaret", "lastName": "Peacock" }, { "employeeId": 7, "supervisorId": 0, "firstName": "Michael", "lastName": "Suyama" }, // Janet Leverling's direct reports { "employeeId": 8, "supervisorId": 4, "firstName": "Anne", "lastName": "Dodsworth" }, { "employeeId": 9, "supervisorId": 4, "firstName": "Danielle", "lastName": "Davis" }, { "employeeId": 10, "supervisorId": 4, "firstName": "Robert", "lastName": "King" }, // Nancy Davolio's direct reports { "employeeId": 11, "supervisorId": 2, "firstName": "Peter", "lastName": "Lewis" }, { "employeeId": 12, "supervisorId": 2, "firstName": "Ryder", "lastName": "Zenaida" }, { "employeeId": 13, "supervisorId": 2, "firstName": "Wang", "lastName": "Mercedes" }, // Steve Buchanan's direct reports { "employeeId": 14, "supervisorId": 3, "firstName": "Theodore", "lastName": "Zia" }, { "employeeId": 15, "supervisorId": 3, "firstName": "Lacota", "lastName": "Mufutau" }, // Lacota Mufutau's direct reports { "employeeId": 16, "supervisorId": 15, "firstName": "Jin", "lastName": "Elliott" }, { "employeeId": 17, "supervisorId": 15, "firstName": "Armand", "lastName": "Ross" }, { "employeeId": 18, "supervisorId": 15, "firstName": "Dane", "lastName": "Rodriquez" }, // Dane Rodriquez's direct reports { "employeeId": 19, "supervisorId": 18, "firstName": "Declan", "lastName": "Lester" }, { "employeeId": 20, "supervisorId": 18, "firstName": "Bernard", "lastName": "Jarvis" }, // Bernard Jarvis' direct report { "employeeId": 21, "supervisorId": 20, "firstName": "Jeremy", "lastName": "Donaldson" } ]; $(function () { $("#treegrid").igTreeGrid({ dataSource: employees, primaryKey: "employeeId", foreignKey: "supervisorId", autoGenerateColumns: false, columns: [ { headerText: "ID", key: "employeeId", width: "150px", dataType: "number" }, { headerText: "First", key: "firstName", width: "150px", dataType: "string" }, { headerText: "Last", key: "lastName", width: "150px", dataType: "string" } ], features: [{ name: "Filtering", displayMode: "showWithAncestors" //displayMode: "showWithAncestorsAndDescendants" }] }); }); </script> </head> <body> <table id="treegrid"></table> </body> </html>
関連サンプル
関連トピック
依存関係
-
advancedModeEditorsVisible
継承- タイプ:
- bool
- デフォルト:
- false
詳細モードでエディターを表示/非表示にするかを定義します。false の場合、詳細モードではエディターは描画されません。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", advancedModeEditorsVisible : true } ] }); // Get var editorsVisible = $(".selector").igTreeGridFiltering("option", "advancedModeEditorsVisible"); // Set $(".selector").igTreeGridFiltering("option", "advancedModeEditorsVisible", true);
-
advancedModeHeaderButtonLocation
継承- タイプ:
- enumeration
- デフォルト:
- left
advancedModeEditorsVisible が False のとき (つまり、ボタンがヘッダーに描画されるとき) の詳細フィルタリング ボタンの場所。
メンバー
- left
- タイプ:string
- right
- タイプ:string
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", advancedModeHeaderButtonLocation : "right" } ] }); // Get var location = $(".selector").igTreeGridFiltering("option", "advancedModeHeaderButtonLocation"); // Set $(".selector").igTreeGridFiltering("option", "advancedModeHeaderButtonLocation", "right");
-
caseSensitive
継承- タイプ:
- bool
- デフォルト:
- false
フィルタリングで大文字小文字の区別を有効化または無効化します。ローカルのフィルタリングでのみ使用できます。True の場合、フィルタリングで大文字小文字の区別を有効にします。False の場合、フィルタリングで大文字小文字の区別を無効にします。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", caseSensitive : true } ] }); // Get var caseSensitive = $(".selector").igTreeGridFiltering("option", "caseSensitive"); // Set $(".selector").igTreeGridFiltering("option", "caseSensitive", true);
-
columnSettings
継承- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
カスタム フィルタリング オプションを列ごとに指定する列設定のリスト。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "ProductDescription", condition: "endsWith" } ] } ] }); // Get var colSettings = $(".selector").igTreeGridFiltering("option", "columnSettings"); // Set $(".selector").igTreeGridFiltering("option", "columnSettings", [{columnKey: "ProductDescription", condition: "endsWith" }] );
-
allowFiltering
- タイプ:
- bool
- デフォルト:
- true
列のフィルタリングを有効/無効にします。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "ProductDescription", allowFiltering: false } ] } ] }); //Get var colSettings = $(".selector").igTreeGridFiltering("option", "columnSettings"); var filterAllowed = colSettings[0].allowFiltering; // Set $(".selector").igTreeGridFiltering("option", "columnSettings", colSettings);
-
columnIndex
- タイプ:
- number
- デフォルト:
- null
列インデックスを指定します。すべての列設定にキーまたはインデックスのどちらかを設定する必要があります。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", columnSettings : [ { columnIndex: 0, allowFiltering: false } ] } ] }); // Get var colSettings = $(".selector").igTreeGridFiltering("option", "columnSettings"); var colIndex = colSettings[0].columnIndex;
-
columnKey
- タイプ:
- string
- デフォルト:
- null
列キーを指定します。すべての列設定にキーまたはインデックスのどちらかを設定する必要があります。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "ProductDescription", allowFiltering: false } ] } ] }); //Get var colSettings = $(".selector").igTreeGridFiltering("option", "columnSettings"); var colKey = colSettings[0].columnKey;
-
condition
- タイプ:
- enumeration
- デフォルト:
- null
列のデフォルト フィルタリング条件。
メンバー
- empty
- タイプ:string
- notEmpty
- タイプ:string
- null
- タイプ:string
- notNull
- タイプ:string
- equals
- タイプ:string
- doesNotEqual
- タイプ:string
- startsWith
- タイプ:string
- contains
- タイプ:string
- doesNotContain
- タイプ:string
- endsWith
- タイプ:string
- greaterThan
- タイプ:string
- lessThan
- タイプ:string
- greaterThanOrEqualTo
- タイプ:string
- lessThanOrEqualTo
- タイプ:string
- true
- タイプ:bool
- false
- タイプ:bool
- on
- タイプ:string
- notOn
- タイプ:string
- before
- タイプ:string
- after
- タイプ:string
- today
- タイプ:string
- yesterday
- タイプ:string
- thisMonth
- タイプ:string
- lastMonth
- タイプ:string
- nextMonth
- タイプ:string
- thisYear
- タイプ:string
- nextYear
- タイプ:string
- lastYear
- タイプ:string
- at
- タイプ:string
- notAt
- タイプ:string
- atBefore
- タイプ:string
- atAfter
- タイプ:string
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "ProductDescription", condition: "startsWith" } ] } ] }); // Get var colSettings = $(".selector").igTreeGridFiltering("option", "columnSettings"); var condition = colSettings[0].condition; // Set $(".selector").igTreeGridFiltering("option", "columnSettings", colSettings);
-
conditionList
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
この列に表示する conditions を決定する文字列の配列。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", columnSettings : [{ columnKey: "ProductNumber", conditionList: ["startsWith", "contains"] }] } ] }); //Get var conditionList = $(".selector").igTreeGridFiltering("option", "columnSettings")[2].conditionList;
-
customConditions
- タイプ:
- object
- デフォルト:
- null
カスタム フィルター条件をこの列のオブジェクトとして指定するためのオブジェクト。
labelText 列の条件ドロップダウンで表示するようなラベル。
expressionText requireExpr が false の場合にエディターに表示するテキスト。
requireExpr この条件にユーザーがフィルター式を入力する必要がある場合
filterImgIcon simple モードでドロップダウン項目に適用されるクラス。
filterFunc カスタム比較フィルター関数。定義: function (value, expression, dataType, ignoreCase, preciseDateFormat)。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", columnSettings : [{ columnKey: "ProductNumber", customConditions: { BE: { labelText: "BE", expressionText: "BE-####", requireExpr: false, filterFunc: filterProductNumber }, CA: { labelText: "CA", expressionText: "CA-####", requireExpr: false, filterFunc: filterProductNumber1 } } }] } ] }); function filterProductNumber(value, expression, dataType, ignoreCase, preciseDateFormat) { return value.startsWith("BE"); } function filterProductNumber1(value, expression, dataType, ignoreCase, preciseDateFormat) { return value.startsWith("CA"); } // Get var customConditions = $(".selector").igTreeGridFiltering("option", "columnSettings")[2].customConditions;
-
defaultExpressions
- タイプ:
- object
- デフォルト:
- []
最初のフィルタリング式。設定された場合、初期化で適用されます。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "Name", defaultExpressions: [ { expr: "B", cond: "startsWith" } ] } ] } ] }); // Get var defaultExpressions = $(".selector").igTreeGridFiltering("option", "columnSettings")[0].defaultExpressions;
-
editorOptions
- タイプ:
- object
- デフォルト:
- null
相対するエディターの初期化オプションを指定します。
コード サンプル
//Initialize $("#grid").igTreeGrid({ features: [ { name: "Filtering", columnSettings: [ { columnKey : "Name", editorType: "text", editorOptions: { buttonType: "dropdown", listItems: names, readOnly: true } } ] } ] }); //Get var columnSettings = $("#grid").igTreeGridFiltering("option", "columnSettings")[0].editorOptions; //Set $("#grid").igTreeGridFiltering("option", "columnSettings", columnSettings);
-
editorProvider
- タイプ:
- object
- デフォルト:
- null
カスタム エディター プロバイダー インスタンスを指定します。エディター プロバイダーの詳細については、ここおよびここを参照してください。
$.ig.EditorProvider を拡張機能するか、以下のメソッドの定義を含む必要があります:
$.ig.EditorProvider = $.ig.EditorProvider|| $.ig.EditorProvider.extend({
createEditor: function (callbacks, key, editorOptions, tabIndex, format, element) {},
attachErrorEvents: function (errorShowing, errorShown, errorHidden) {},
getEditor: function () {},
refreshValue: function () {},
getValue: function () {},
setValue: function (val) {},
setSize: function (width, height) {},
setFocus: function () {},
removeFromParent: function () {},
destroy: function () {},
validator: function () {},
validate: function (noLabel) {},
isValid: function () {}
});コード サンプル
// This editor provider demonstrates how to wrap HTML 5 number INPUT into editor provider for the igTreeGridFiltering $.ig.EditorProviderNumber = $.ig.EditorProviderNumber || $.ig.EditorProvider.extend({ // initialize the editor createEditor: function (callbacks, key, editorOptions, tabIndex, format, element) { element = element || $('<input />'); // call parent createEditor this._super(callbacks, key, editorOptions, tabIndex, format, element); element.on("keydown", $.proxy(this.keyDown, this)); element.on("change", $.proxy(this.change, this)); this.editor = {}; this.editor.element = element; return element; }, keyDown: function(evt) { var ui = {}; ui.owner = this.editor.element; ui.owner.element = this.editor.element; this.callbacks.keyDown(evt, ui, this.columnKey); // enable "Done" button only for numeric character if ((evt.keyCode >= 48 && evt.keyCode <= 57) || (evt.keyCode >= 96 && evt.keyCode <= 105)) { this.callbacks.textChanged(evt, ui, this.columnKey); } }, change: function (evt) { var ui = {}; ui.owner = this.editor.element; ui.owner.element = this.editor.element; this.callbacks.textChanged(evt, ui, this.columnKey); }, // get editor value getValue: function () { return parseFloat(this.editor.element.val()); }, // set editor value setValue: function (val) { return this.editor.element.val(val || 0); }, // size the editor into the TD cell setSize: function (width, height) { this.editor.element.css({ width: width - 2, height: height - 2, borderWidth: "1px", backgroundPositionY: "9px" }); }, // focus the editor setFocus: function () { this.editor.element.select(); }, // validate the editor validator: function () { // no validator return null; }, // destroy the editor destroy: function () { this.editor.remove(); } }); //Initialize $("#grid").igTreeGrid({ features: [ { name: "Filtering", columnSettings: [ { columnKey : "SafetyStockLevel", editorProvider: new $.ig.EditorProviderNumber() } ] } ] }); //Get var columnSettings = $("#grid").igTreeGridFiltering("option", "columnSettings")[0].editorProvider;
-
editorType
- タイプ:
- enumeration
- デフォルト:
- null
列に使用するエディターの型を指定します。
メンバー
- text
- タイプ:string
- igTextEditor は作成されます。
- mask
- タイプ:string
- igMaskEditor は作成されます。
- date
- タイプ:string
- igDateEditor は作成されます。
- datepicker
- タイプ:string
- igDatePicker は作成されます。
- timepicker
- タイプ:string
- igTimePicker は作成されます。
- numeric
- タイプ:string
- igNumericEditor は作成されます。
- checkbox
- タイプ:string
- igCheckboxEditor は作成されます。
- currency
- タイプ:string
- igCurrencyEditor は作成されます。
- percent
- タイプ:string
- igPercentEditor は作成されます。
- combo
- タイプ:string
- igCombo エディターは作成されます。ui.igCombo によって使用される css および js ファイルが利用可能です。
- rating
- タイプ:string
- igRating エディターは作成されます。ui.igRating によって使用される css および js ファイルが利用可能です。
コード サンプル
//Initialize $("#grid").igTreeGrid({ features: [ { name: "Filtering", columnSettings: [ { columnKey : "BirthDate", editorType: "datepicker" } ] } ] }); //Get var columnSettings = $("#grid").igTreeGridFiltering("option", "columnSettings")[0].editorType; //Set $("#grid").igTreeGridFiltering("option", "columnSettings", columnSettings);
-
dialogWidget
継承- タイプ:
- string
- デフォルト:
- "igGridModalDialog"
使用するダイアログ ウィジェットの名前。$.ui.igGridModalDialog から継承します。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", dialogWidget: "advancedModalDialog" } ] }); // Get var widgetName = $(".selector").igTreeGridFiltering("option", "dialogWidget");
-
displayMode
- タイプ:
- enumeration
- デフォルト:
- showWithAncestors
displayMode が showWithAncestorsAndDescendants の場合、子レコードがフィルター条件と一致しなくても子レコードを表示します。displayMode が showWithAncestors の場合、フィルター条件と一致するレコードのみを表示し、子レコードがフィルター条件と一致しない場合、子レコードを表示しません。
コード サンプル
$(".selector").igTreeGrid({ features: [ { name : "Filtering", displayMode : "showWithAncestorsAndDescendants" } ] }); //Get var displayMode = $(".selector").igTreeGridFiltering("option", "displayMode");
-
featureChooserText
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
フィルターが表示され、mode が simple である場合、フィルターの内容が表示されます。locale.featureChooserText オプションを使用します。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", featureChooserText : "Hide Filter" } ] }); // Get var text = $(".selector").igTreeGridFiltering("option", "featureChooserText"); // Set $(".selector").igTreeGridFiltering("option", "featureChooserText", "Hide Filter");
-
featureChooserTextAdvancedFilter
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
フィルター モードが詳細である場合、フィルターの内容が表示されます。locale.featureChooserTextAdvancedFilter オプションを使用します。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", featureChooserTextAdvancedFilter : "Advanced Filter" } ] }); // Get var text = $(".selector").igTreeGridFiltering("option", "featureChooserTextAdvancedFilter"); // Set $(".selector").igTreeGridFiltering("option", "featureChooserTextAdvancedFilter", "Advanced Filter");
-
featureChooserTextHide
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
フィルターが非表示で、mode が simple である場合の機能セレクターのテキスト。locale.featureChooserTextHide オプションを使用します。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", featureChooserTextHide : "Show Filter" } ] }); // Get var text = $(".selector").igTreeGridFiltering("option", "featureChooserTextHide"); // Set $(".selector").igTreeGridFiltering("option", "featureChooserTextHide", "Show Filter");
-
filterButtonLocation
継承- タイプ:
- enumeration
- デフォルト:
- left
フィルター ドロップダウンのフィルタリング ボタンは、フィルター エディターの左側または右側に描画できます。
メンバー
- left
- タイプ:string
- ボタンが左側に描画されます。
- right
- タイプ:string
- ボタンが右側に描画されます。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterButtonLocation : "right" } ] }); // Get var location = $(".selector").igTreeGridFiltering("option", "filterButtonLocation"); // Set $(".selector").igTreeGridFiltering("option", "filterButtonLocation", "right");
-
filterDelay
継承- タイプ:
- number
- デフォルト:
- 500
フィルタリング要求を送信する前に、キー入力までのウィジェットの待機時間 (ミリ秒)。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDelay : 1000 } ] }); // Get var delay = $(".selector").igTreeGridFiltering("option", "filterDelay"); // Set $(".selector").igTreeGridFiltering("option", "filterDelay", 1000);
-
filterDialogAddButtonWidth
継承- タイプ:
- enumeration
- デフォルト:
- 100
詳細フィルター ダイアログ内の [追加] ボタンの幅。
メンバー
- string
- タイプ:string
- ピクセルのダイアログ追加ボタン幅 (100px)
- number
- タイプ:number
- 数値のダイアログ追加ボタン幅 (100)
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogAddButtonWidth : 75 } ] }); // Get var width = $(".selector").igTreeGridFiltering("option", "filterDialogAddButtonWidth"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogAddButtonWidth", 75);
-
filterDialogAddConditionDropDownTemplate
継承- タイプ:
- string
- デフォルト:
- null
フィルター ダイアログの追加条件領域のドロップダウンのオプションのカスタム テンプレート。
デフォルト テンプレートは "<option value='${value}'>${text}</option>" です。filterDialogAddConditionTemplate が適用される場合に使用されます。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogAddConditionDropDownTemplate: "<option value='${text}'>${text}</option>" } ] }); // Get var dropDownTemplate = $(".selector").igTreeGridFiltering("option", "filterDialogAddConditionDropDownTemplate"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogAddConditionDropDownTemplate", "<option value='${text}'>${text}</option>");
-
filterDialogAddConditionTemplate
継承- タイプ:
- string
- デフォルト:
- null
フィルター ダイアログの追加条件領域のカスタム テンプレート。
デフォルトのテンプレートは "${label1}${label2}"。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogAddConditionTemplate: "<div><div><select></select></div><span>${label1}</span><span>${label2}</span></div>" } ] }); // Get var addConditionTemplate = $(".selector").igTreeGridFiltering("option", "filterDialogAddConditionTemplate"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogAddConditionTemplate", "<div><div><select></select></div><span>${label1}</span><span>${label2}</span></div>");
-
filterDialogColumnDropDownDefaultWidth
継承- タイプ:
- enumeration
- デフォルト:
- null
詳細フィルター ダイアログ内の列選択ドロップダウンの幅。
メンバー
- string
- タイプ:string
- ピクセルの列選択ドロップダウンの幅 (80px)。
- number
- タイプ:number
- 数値の列選択ドロップダウンの幅 (80)
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogColumnDropDownDefaultWidth : 100 } ] }); // Get var width = $(".selector").igTreeGridFiltering("option", "filterDialogColumnDropDownDefaultWidth"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogColumnDropDownDefaultWidth", 100);
-
filterDialogContainment
継承- タイプ:
- string
- デフォルト:
- "owner"
コンテインメント動作を制御します。
owner - フィルター ダイアログはグリッド領域でのみドラッグ可能です。
window - フィルター ダイアログはウィンドウ領域全体でドラッグ可能です。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogContainment : "window" } ] }); // Get var filterDialogContainment = $(".selector").igTreeGridFiltering("option", "filterDialogContainment");
-
filterDialogExprInputDefaultWidth
継承- タイプ:
- enumeration
- デフォルト:
- 100
詳細フィルター ダイアログ内のフィルタリングの式の入力ボックスの幅。
メンバー
- string
- タイプ:string
- ピクセルのフィルター式入力ボックスの幅 (80px)。
- number
- タイプ:number
- 数値のフィルター式入力ボックスの幅 (80)
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogExprInputDefaultWidth : 100 } ] }); // Get var width = $(".selector").igTreeGridFiltering("option", "filterDialogExprInputDefaultWidth"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogExprInputDefaultWidth", 100);
-
filterDialogFilterConditionTemplate
継承- タイプ:
- string
- デフォルト:
- null
フィルター ダイアログにある条件リストのオプションのカスタム テンプレート。
デフォルト テンプレートは "<option value='${condition}'>${text}</option>" です。"data-af-cond" 属性がある DOM 要素をカスタマイズ化するために使用されます。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogFilterConditionTemplate: "<option value='${conditionName}'>${conditionLabel}</option>" } ] }); // Get var filterConditionTemplate = $(".selector").igTreeGridFiltering("option", "filterDialogFilterConditionTemplate"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogFilterConditionTemplate", "<option value='${conditionName}'>${conditionLabel}</option>");
-
filterDialogFilterDropDownDefaultWidth
継承- タイプ:
- enumeration
- デフォルト:
- 150
詳細フィルター ダイアログ内のフィルタリング条件ドロップダウンの幅。
メンバー
- string
- タイプ:string
- ピクセルのフィルター条件ドロップダウンの幅 (80px)。
- number
- タイプ:number
- 数値のフィルター条件ドロップダウンの幅 (80)
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogFilterDropDownDefaultWidth : 100 } ] }); // Get var width = $(".selector").igTreeGridFiltering("option", "filterDialogFilterDropDownDefaultWidth"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogFilterDropDownDefaultWidth", 100);
-
filterDialogFilterTemplate
継承- タイプ:
- string
- デフォルト:
- null
フィルター ダイアログのカスタム テンプレート。
フィルター条件/列/フィルター式を選択するために使用される各 DOM 要素は "data-*" 属性があります。
例: 列を選択するための DOM 要素は "data-af-col" 属性があります。フィルター条件の選択で使用される要素は "data-af-cond" があります。フィルター式の要素は "data-af-expr" があります。
注: テンプレートは <tr /> のみでサポートされます。
デフォルト テンプレートは「<tr data-af-row><td><input data-af-col/></td><td><input data-af-cond/></td><td><input data-af-expr /> </td><td><span data-af-rmv></span></td></tr>」。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogFilterTemplate: "<tr><td>Choose column<input/></td><td>Condition<select></select></td><td>Search value<input /> </td><td><span></span></td></tr>" } ] }); // Get var dialogFilterTemplate = $(".selector").igTreeGridFiltering("option", "filterDialogFilterTemplate"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogFilterTemplate", "<tr><td>Choose column<input/></td><td>Condition<select></select></td><td>Search value<input /> </td><td><span></span></td></tr>");
-
filterDialogHeight
継承- タイプ:
- enumeration
- デフォルト:
- 340
デフォルト フィルター ダイアログの高さ (詳細フィルター mode で使用)。
メンバー
- string
- タイプ:string
- ピクセルでのダイアログ ウィンドウの高さ (350px)。
- number
- タイプ:number
- 数値のダイアログ ウィンドウの高さ (350)。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogHeight : 30 } ] }); // Get var height = $(".selector").igTreeGridFiltering("option", "filterDialogHeight"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogHeight", 30);
-
filterDialogMaxFilterCount
継承- タイプ:
- number
- デフォルト:
- 5
詳細フィルタリング ダイアログ内のフィルター行の最大数。この数を超えると、エラー メッセージが描画されます。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogMaxFilterCount : 3 } ] }); // Get var count = $(".selector").igTreeGridFiltering("option", "filterDialogMaxFilterCount"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogMaxFilterCount", 3);
-
filterDialogOkCancelButtonWidth
継承- タイプ:
- enumeration
- デフォルト:
- 120
詳細フィルタリング ダイアログ内の [OK] および [キャンセル] ボタンの幅。
メンバー
- string
- タイプ:string
- ピクセルの詳細フィルターダイアログ OK およびキャンセル ボタンの幅 (120 px)。
- number
- タイプ:number
- 数値の詳細フィルターダイアログ OK およびキャンセル ボタンの幅 (120)。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogOkCancelButtonWidth : 120 } ] }); // Get var width = $(".selector").igTreeGridFiltering("option", "filterDialogOkCancelButtonWidth"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogOkCancelButtonWidth", 120);
-
filterDialogWidth
継承- タイプ:
- enumeration
- デフォルト:
- 500
デフォルト フィルター ダイアログの幅 (詳細フィルター mode で使用)。
メンバー
- string
- タイプ:string
- ピクセルでのダイアログ ウィンドウ幅 (500 px)。
- number
- タイプ:number
- 数値のダイアログ ウィンドウの幅 (500)。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogWidth : 500 } ] }); // Get var width = $(".selector").igTreeGridFiltering("option", "filterDialogWidth"); // Set $(".selector").igTreeGridFiltering("option", "filterDialogWidth", 500);
-
filterDropDownAnimationDuration
継承- タイプ:
- number
- デフォルト:
- 500
フィルター ドロップダウンのアニメーションの期間 (ミリ秒単位)。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDropDownAnimationDuration : 300 } ] }); // Get var duration = $(".selector").igTreeGridFiltering("option", "filterDropDownAnimationDuration"); // Set $(".selector").igTreeGridFiltering("option", "filterDropDownAnimationDuration", 300);
-
filterDropDownAnimations
継承- タイプ:
- enumeration
- デフォルト:
- linear
列フィルター ドロップダウンのアニメーションのタイプ。
メンバー
- linear
- タイプ:string
- 列フィルター ドロップダウンがリニア アニメーションで表示されます。
- none
- タイプ:string
- フィルター ドロップダウンの表示にアニメーションはありません。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDropDownAnimations : "none" } ] }); // Get var animation = $(".selector").igTreeGridFiltering("option", "filterDropDownAnimations"); // Set $(".selector").igTreeGridFiltering("option", "filterDropDownAnimations", "none");
-
filterDropDownHeight
継承- タイプ:
- object
- デフォルト:
- 0
列フィルター ドロップダウンの高さ。
ピクセルの列フィルター ドロップダウンの高さ (0px)。
数値のフィルター ドロップダウンの高さ (0)。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDropDownHeight : 30 } ] }); // Get var height = $(".selector").igTreeGridFiltering("option", "filterDropDownHeight"); // Set $(".selector").igTreeGridFiltering("option", "filterDropDownHeight", 30);
-
filterDropDownItemIcons
継承- タイプ:
- enumeration
- デフォルト:
- true
フィルター アイコンの表示状態を有効/無効にします。
メンバー
- true
- タイプ:bool
- フィルター ドロップダウン内のすべての定義済みのフィルターで、テキストの前にアイコンが描画されます。
- false
- タイプ:bool
- アイコンは描画されません。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDropDownItemIcons : false } ] }); // Get var showIcons = $(".selector").igTreeGridFiltering("option", "filterDropDownItemIcons"); // Set $(".selector").igTreeGridFiltering("option", "filterDropDownItemIcons", false);
-
filterDropDownWidth
継承- タイプ:
- enumeration
- デフォルト:
- 0
列フィルター ドロップダウンの幅。
メンバー
- string
- タイプ:string
- ピクセルの幅 (0 px)
- number
- タイプ:number
- 数値の幅 (0)。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDropDownWidth : 100 } ] }); // Get var width = $(".selector").igTreeGridFiltering("option", "filterDropDownWidth"); // Set $(".selector").igTreeGridFiltering("option", "filterDropDownWidth", 100);
-
filterExprUrlKey
継承- タイプ:
- string
- デフォルト:
- null
リモート要求に対してフィルタリングの式をエンコードする方法を指定する URL キー名。たとえば、&filter('col') = startsWith。デフォルトは OData です。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterExprUrlKey : "filter" } ] }); // Get var key = $(".selector").igTreeGridFiltering("option", "filterExprUrlKey"); // Set $(".selector").igTreeGridFiltering("option", "filterExprUrlKey", "filter");
-
filterSummaryAlwaysVisible
継承- タイプ:
- bool
- デフォルト:
- true
フィルターの概要情報でフッターの表示を有効または無効にします。
False の場合、(フッター内の) フィルター集計行はページング (または、フッターを描画する他の機能) が有効なときのみ表示されます。
True の場合、フィルター集計行はフィルターが適用されるときのみ表示されます。デフォルトでは表示されません。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterSummaryAlwaysVisible : false } ] }); // Get var showSummary = $(".selector").igTreeGridFiltering("option", "filterSummaryAlwaysVisible"); // Set $(".selector").igTreeGridFiltering("option", "filterSummaryAlwaysVisible", false);
-
filterSummaryInPagerTemplate
削除- タイプ:
- string
- デフォルト:
- null
このオプションは 2017.2 バージョン以降サポートされません。
フィルタリングが適用され、ページングが有効な場合、ユーザーがその他のページに移動するときに使用されるテンプレート。igTreeGridPaging の pagerRecordsLabelTemplate より優先されます。null に設定される場合、igTreeGridPaging オプションを使用します。
サポートされるオプション:
$currentPageMatches (フィルター)
$totalMatches (フィルター)
$startRecord (ページング)
$endRecord (ページング)
$recordCount (ページング)。
locale.collapseTooltipText オプションを使用します。コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterSummaryInPagerTemplate : "${startRecord} - ${endRecord} of ${recordCount} records" } ] }); //Get var filterPagerSummary = $(".selector").igTreeGridFiltering("option", "filterSummaryInPagerTemplate");
-
filterSummaryTemplate
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
フッターの左下隅に表示されるサマリー テンプレート。「${matches} の一致レコード」の形式を持っています。locale.filterSummaryTemplate オプションを使用します。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterSummaryTemplate: "${matches} products found" } ] }); // Get var summaryTemplate = $(".selector").igTreeGridFiltering("option", "filterSummaryTemplate"); // Set $(".selector").igTreeGridFiltering("option", "filterSummaryTemplate", "${matches} products");
-
fromLevel
- タイプ:
- number
- デフォルト:
- 0
フィルターの適用を開始するデータ バインドされたレベルを指定します。0 は最初のレベルです。
コード サンプル
$(".selector").igTreeGrid({ features: [ { name : "Filtering", fromLevel: 1 } ] }); //Get var fromLevel = $(".selector").igTreeGridFiltering("option", "fromLevel");
-
labels
削除- タイプ:
- string
- デフォルト:
- "{}"
このオプションは 2017.2 バージョン以降サポートされません。
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する設定可能なローカライズ済みのラベルのリスト。locale オプションを使用します。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { advancedButtonLabel: "Advanced", after: "After", before: "Before", clear: "Clear Filter", contains: "Contains" //... } } ] }); // Get var filteringLabels = $(".selector").igTreeGridFiltering("option", "labels");
-
advancedButtonLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { advancedButtonLabel: "Advanced" } } ] });
-
after
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { after: "after" } } ] });
-
before
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { before: "before" } } ] });
-
clear
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { clear: "clear filter" } } ] });
-
contains
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { contains: "contains" } } ] });
-
doesNotContain
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { doesNotContain: "does Not contain" } } ] });
-
doesNotEqual
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { doesNotEqual: "does Not equal" } } ] });
-
empty
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { empty: "empty" } } ] });
-
endsWith
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { endsWith: "ends with" } } ] });
-
equals
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { equals: "equals" } } ] });
-
falseLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { falseLabel: "false" } } ] });
-
filterDialogAddLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogAddLabel: "add" } } ] });
-
filterDialogAllLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogAllLabel: "all" } } ] });
-
filterDialogAnyLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogAnyLabel: "any" } } ] });
-
filterDialogCancelLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogCancelLabel: "cancel" } } ] });
-
filterDialogCaptionLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogCaptionLabel: "Advanced Filtering" } } ] });
-
filterDialogClearAllLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogClearAllLabel: "clear all" } } ] });
-
filterDialogConditionLabel1
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogConditionLabel1: "Show" } } ] });
-
filterDialogConditionLabel2
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogConditionLabel2: "records matching the following criteria" } } ] });
-
filterDialogErrorLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogErrorLabel: "error" } } ] });
-
filterDialogOkLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterDialogOkLabel: "ok" } } ] });
-
filterSummaryTitleLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { filterSummaryTitleLabel: "filtering summary" } } ] });
-
greaterThan
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { greaterThan: "greater than" } } ] });
-
greaterThanOrEqualTo
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { greaterThanOrEqualTo: "greater than or equal to" } } ] });
-
lastMonth
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { lastMonth: "last month" } } ] });
-
lastYear
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { lastYear: "last year" } } ] });
-
lessThan
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { lessThan: "less than" } } ] });
-
lessThanOrEqualTo
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { lessThanOrEqualTo: "less than or equal to" } } ] });
-
nextMonth
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { nextMonth: "next month" } } ] });
-
nextYear
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { nextYear: "next year" } } ] });
-
noFilter
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { noFilter: "no filter" } } ] });
-
notEmpty
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { notEmpty: "not empty" } } ] });
-
notNull
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { notNull: "not null" } } ] });
-
notOn
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { notOn: "not on" } } ] });
-
nullLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { nullLabel: "null label" } } ] });
-
on
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { on: "on" } } ] });
-
startsWith
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { startsWith: "starts with" } } ] });
-
thisMonth
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { thisMonth: "this month" } } ] });
-
thisYear
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { thisYear: "this year" } } ] });
-
today
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { today: "today" } } ] });
-
true
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { true: "True" } } ] });
-
trueLabel
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { trueLabel: "true" } } ] });
-
yesterday
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", labels: { yesterday: "yesterday" } } ] });
-
locale
- タイプ:
- object
- デフォルト:
- {}
-
advancedButtonLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [詳細] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { advancedButtonLabel : "Advance Button"} } ] }); //Get var advancedButtonLabel = $(".selector").igTreeGridFiltering("option", "locale").advancedButtonLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {advancedButtonLabel : "Advance Button"});
-
afterLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~の後] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { afterLabel : "After"} } ] }); //Get var afterLabel = $(".selector").igTreeGridFiltering("option", "locale").afterLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {afterLabel : "After"});
-
afterNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~の後] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { afterNullText : "After"} } ] }); //Get var afterNullText = $(".selector").igTreeGridFiltering("option", "locale").afterNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {afterNullText : "After"});
-
atAfterLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [以後] ラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { atAfterLabel : "At or after"} } ] }); //Get var atAfterLabel = $(".selector").igTreeGridFiltering("option", "locale").atAfterLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {atAfterLabel : "At or after"});
-
atBeforeLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [以前] ラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { atBeforeLabel : "At or before"} } ] }); //Get var atBeforeLabel = $(".selector").igTreeGridFiltering("option", "locale").atBeforeLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {atBeforeLabel : "At or before"});
-
atLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [特定の時間] ラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { atLabel : "At"} } ] }); //Get var atLabel = $(".selector").igTreeGridFiltering("option", "locale").atLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {atLabel : "At"});
-
beforeLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~の前] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { beforeLabel : "Before"} } ] }); //Get var beforeLabel = $(".selector").igTreeGridFiltering("option", "locale").beforeLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {beforeLabel : "Before"});
-
beforeNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~の前] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { beforeNullText : "Before"} } ] }); //Get var beforeNullText = $(".selector").igTreeGridFiltering("option", "locale").beforeNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {beforeNullText : "Before"});
-
clearLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [クリア] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { clearLabel : "Clear"} } ] }); //Get var clearLabel = $(".selector").igTreeGridFiltering("option", "locale").clearLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {clearLabel : "Clear"});
-
containsLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~を含む] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { containsLabel : "Contains"} } ] }); //Get var containsLabel = $(".selector").igTreeGridFiltering("option", "locale").containsLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {containsLabel : "Contains"});
-
containsNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~を含む] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { containsNullText : "Contains"} } ] }); //Get var endsWithNullText = $(".selector").igTreeGridFiltering("option", "locale").containsNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {containsNullText : "Contains"});
-
doesNotContainLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~を含まない] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { doesNotContainLabel : "Does not contain"} } ] }); //Get var doesNotContainLabel = $(".selector").igTreeGridFiltering("option", "locale").doesNotContainLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {doesNotContainLabel : "Does not contain"});
-
doesNotContainNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~を含まない] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { doesNotContainNullText : "Doesn't contain"} } ] }); //Get var doesNotContainNullText = $(".selector").igTreeGridFiltering("option", "locale").doesNotContainNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {doesNotContainNullText : "Doesn't contain"});
-
doesNotEqualLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~に等しくない] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { doesNotEqualLabel : "Does not Equal"} } ] }); //Get var doesNotEqualLabel = $(".selector").igTreeGridFiltering("option", "locale").doesNotEqualLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {doesNotEqualLabel : "Does not Equal"});
-
doesNotEqualNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~に等しくない] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { doesNotEqualNullText : "Equals"} } ] }); //Get var doesNotEqualNullText = $(".selector").igTreeGridFiltering("option", "locale").doesNotEqualNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {doesNotEqualNullText : "Equals"});
-
emptyNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [空] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { beforeNullText : "Before"} } ] }); //Get var beforeNullText = $(".selector").igTreeGridFiltering("option", "locale").beforeNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {beforeNullText : "Before"});
-
endsWithLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~で始まる] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { endsWithLabel : "Starts With"} } ] }); //Get var endsWithLabel = $(".selector").igTreeGridFiltering("option", "locale").endsWithLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {endsWithLabel : "Ends With"});
-
endsWithNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~で終わる] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { endsWithNullText : "Ends With"} } ] }); //Get var endsWithNullText = $(".selector").igTreeGridFiltering("option", "locale").endsWithNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {endsWithNullText : "Ends With"});
-
equalsLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~に等しい] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { equalsLabel : "Equals"} } ] }); //Get var equalsLabel = $(".selector").igTreeGridFiltering("option", "locale").equalsLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {equalsLabel : "Equals"});
-
equalsNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~に等しい] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { equalsNullText : "Equals"} } ] }); //Get var equalsNullText = $(".selector").igTreeGridFiltering("option", "locale").equalsNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {equalsNullText : "Equals"});
-
falseLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [False] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { falseLabel : "False"} } ] }); //Get var falseLabel = $(".selector").igTreeGridFiltering("option", "locale").falseLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {falseLabel : "False"});
-
featureChooserText
継承- タイプ:
- string
- デフォルト:
- ""
フィルタが表示され、フィルター mode が簡易な場合の機能セレクター テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { featureChooserText : "Hide Filter"} } ] }); //Get var featureChooserText = $(".selector").igTreeGridFiltering("option", "locale").featureChooserText; //Set $(".selector").igTreeGridFiltering("option", "locale", {featureChooserText : "Hide Filter"});
-
featureChooserTextAdvancedFilter
継承- タイプ:
- string
- デフォルト:
- ""
フィルター mode が詳細である場合、フィルターの内容が表示されます。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { featureChooserTextAdvancedFilter : "Advanced Filter"} } ] }); //Get var featureChooserTextAdvancedFilter = $(".selector").igTreeGridFiltering("option", "locale").featureChooserTextAdvancedFilter; //Set $(".selector").igTreeGridFiltering("option", "locale", {featureChooserTextAdvancedFilter : "Advanced Filter"});
-
featureChooserTextHide
継承- タイプ:
- string
- デフォルト:
- ""
フィルターが非表示で、mode がシンプルである場合、フィルターの内容は表示されません。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { featureChooserTextHide : "Hide Filter"} } ] }); //Get var featureChooserTextHide = $(".selector").igTreeGridFiltering("option", "locale").featureChooserTextHide; //Set $(".selector").igTreeGridFiltering("option", "locale", {featureChooserTextHide : "Hide Filter"});
-
filterDialogAddLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルタリング ダイアログの [追加] ボタンのラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogAddLabel : "Add"} } ] }); //Get var filterDialogAddLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogAddLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogAddLabel : "Add"});
-
filterDialogAllLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルタリング ダイアログの [すべて] ボタンのラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogAllLabel : "All"} } ] }); //Get var filterDialogAllLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogAllLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogAllLabel : "All"});
-
filterDialogAnyLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルタリング ダイアログの [いずれか] ボタンのラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogAnyLabel : "Any"} } ] }); //Get var filterDialogAnyLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogAnyLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogAnyLabel : "Any"});
-
filterDialogCancelLabel
継承- タイプ:
- string
- デフォルト:
- ""
ダイアログの [キャンセル] ボタンのラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogCancelLabel : "Search"} } ] }); //Get var filterDialogCancelLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogCancelLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogCancelLabel : "Search"});
-
filterDialogCaptionLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ダイアログ キャプションのラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogCaptionLabel : "Label"} } ] }); //Get var filterDialogCaptionLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogCaptionLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogCaptionLabel : "Label"});
-
filterDialogClearAllLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ダイアログの [すべてクリア] ラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogClearAllLabel : "Clear ALL"} } ] }); //Get var filterDialogClearAllLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogClearAllLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogClearAllLabel : "Clear ALL"});
-
filterDialogCloseLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルタリング ダイアログの [閉じる] ラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogCloseLabel : "Close"} } ] }); //Get var filterDialogCloseLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogCloseLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogCloseLabel : "Close"});
-
filterDialogConditionDropDownLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター条件のドロップダウン ラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogConditionDropDownLabel : "Filtering condition"} } ] }); //Get var filterDialogConditionDropDownLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogConditionDropDownLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogConditionDropDownLabel : "Filtering condition"});
-
filterDialogConditionLabel1
継承- タイプ:
- string
- デフォルト:
- ""
フィルター条件のラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogConditionLabel1 : "Show records matching"} } ] }); //Get var filterDialogConditionLabel1 = $(".selector").igTreeGridFiltering("option", "locale").filterDialogConditionLabel1; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogConditionLabel1 : "Show records matching"});
-
filterDialogConditionLabel2
継承- タイプ:
- string
- デフォルト:
- ""
フィルター条件のラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogConditionLabel2 : " of the following criteria"} } ] }); //Get var filterDialogConditionLabel2 = $(".selector").igTreeGridFiltering("option", "locale").filterDialogConditionLabel2; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogConditionLabel2 : " of the following criteria"});
-
filterDialogErrorLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルタリング ダイアログの [エラー] ラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogErrorLabel : "You reached the maximum number of filters supported."} } ] }); //Get var filterDialogErrorLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogErrorLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogErrorLabel : "You reached the maximum number of filters supported."});
-
filterDialogOkLabel
継承- タイプ:
- string
- デフォルト:
- ""
ダイアログの [OK] ボタンのラベルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterDialogOkLabel : "Search"} } ] }); //Get var filterDialogOkLabel = $(".selector").igTreeGridFiltering("option", "locale").filterDialogOkLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterDialogOkLabel : "Search"});
-
filterSummaryInPagerTemplate
- タイプ:
- string
- デフォルト:
- ""
フィルタリングが適用され、ページングが有効な場合、ユーザーがその他のページに移動するときに使用されるテンプレート。igTreeGridPaging の pagerRecordsLabelTemplate より優先されます。null に設定される場合、igTreeGridPaging オプションを使用します。
サポートされるオプション:
${currentPageMatches} (フィルタリング)
${totalMatches} (フィルタリング)
${startRecord} (ページング)
${endRecord} (ページング)
${recordCount} (ページング)コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterSummaryInPagerTemplate : "${startRecord} - ${endRecord} of ${recordCount} records" } } ] }); //Get var filterPagerSummary = $(".selector").igTreeGridFiltering("option", "locale").filterSummaryInPagerTemplate;
-
filterSummaryTemplate
継承- タイプ:
- string
- デフォルト:
- ""
一致するレコードのサマリー テンプレートを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterSummaryTemplate : "${matches} matching records"} } ] }); //Get var filterSummaryTemplate = $(".selector").igTreeGridFiltering("option", "locale").filterSummaryTemplate; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterSummaryTemplate : "${matches} matching records"});
-
filterSummaryTitleLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルタリング サマリー タイトルを指定します。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { filterSummaryTitleLabel : "Search results"} } ] }); //Get var filterSummaryTitleLabel = $(".selector").igTreeGridFiltering("option", "locale").filterSummaryTitleLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {filterSummaryTitleLabel : "Search results"});
-
greaterThanLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~より大きい] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { greaterThanLabel : "Greater Than"} } ] }); //Get var greaterThanLabel = $(".selector").igTreeGridFiltering("option", "locale").greaterThanLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {greaterThanLabel : "Greater Than"});
-
greaterThanNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~より大きい] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { greaterThanNullText : "Greater than"} } ] }); //Get var greaterThanNullText = $(".selector").igTreeGridFiltering("option", "locale").greaterThanNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {greaterThanNullText : "Greater than"});
-
greaterThanOrEqualToLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [以上] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { greaterThanOrEqualToLabel : "Greater Than or Equal"} } ] }); //Get var greaterThanOrEqualToLabel = $(".selector").igTreeGridFiltering("option", "locale").greaterThanOrEqualToLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {greaterThanOrEqualToLabel : "Greater Than or Equal"});
-
greaterThanOrEqualToNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [以上] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { greaterThanOrEqualToNullText : "Greater Than or Equals to"} } ] }); //Get var greaterThanOrEqualToNullText = $(".selector").igTreeGridFiltering("option", "locale").greaterThanOrEqualToNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {greaterThanOrEqualToNullText : "Greater Than or Equals to"});
-
lastMonthLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [先月] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { lastMonthLabel : "Last Month"} } ] }); //Get var lastMonthLabel = $(".selector").igTreeGridFiltering("option", "locale").lastMonthLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {lastMonthLabel : "Last Month"});
-
lastYearLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [昨年] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { lastYearLabel : "Last Year"} } ] }); //Get var lastYearLabel = $(".selector").igTreeGridFiltering("option", "locale").lastYearLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {lastYearLabel : "Last Year"});
-
lessThanLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~より小さい] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { lessThanLabel : "Less Than"} } ] }); //Get var lessThanLabel = $(".selector").igTreeGridFiltering("option", "locale").lessThanLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {lessThanLabel : "Less Than"});
-
lessThanNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~より小さい] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { lessThanNullText : "Less Than Null"} } ] }); //Get var lessThanNullText = $(".selector").igTreeGridFiltering("option", "locale").lessThanNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {lessThanNullText : "Less Than Null"});
-
lessThanOrEqualToLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [以下] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { lessThanOrEqualToLabel : "Less Than or Equal"} } ] }); //Get var lessThanOrEqualToLabel = $(".selector").igTreeGridFiltering("option", "locale").lessThanOrEqualToLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {lessThanOrEqualToLabel : "Less Than or Equal"});
-
lessThanOrEqualToNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [以下] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { lessThanOrEqualToNullText : "Less Than or Equals to"} } ] }); //Get var lessThanOrEqualToNullText = $(".selector").igTreeGridFiltering("option", "locale").lessThanOrEqualToNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {lessThanOrEqualToNullText : "Less Than or Equals to"});
-
nextMonthLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [翌月] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { nextMonthLabel : "Next Month"} } ] }); //Get var nextMonthLabel = $(".selector").igTreeGridFiltering("option", "locale").nextMonthLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {nextMonthLabel : "Next Month"});
-
nextYearLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [来年] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { nextYearLabel : "Next Year"} } ] }); //Get var nextYearLabel = $(".selector").igTreeGridFiltering("option", "locale").nextYearLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {nextYearLabel : "Next Year"});
-
noFilterLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [なし] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { noFilterLabel : "No Filter"} } ] }); //Get var noFilterLabel = $(".selector").igTreeGridFiltering("option", "locale").noFilterLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {noFilterLabel : "No Filter"});
-
notAtLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [特定の時間以外] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { notAtLabel : "Not at"} } ] }); //Get var notAtLabel = $(".selector").igTreeGridFiltering("option", "locale").notAtLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {notAtLabel : "Not at"});
-
notEmptyNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [空以外] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { notEmptyNullText : "Empty"} } ] }); //Get var notEmptyNullText = $(".selector").igTreeGridFiltering("option", "locale").notEmptyNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {notEmptyNullText : "Empty"});
-
notNullNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [null 以外] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { notNullNullText : "Not Null"} } ] }); //Get var notNullNullText = $(".selector").igTreeGridFiltering("option", "locale").notNullNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {notNullNullText : "Not Null"});
-
notOnLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [指定日以外] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { notOnLabel : "Not On"} } ] }); //Get var notOnLabel = $(".selector").igTreeGridFiltering("option", "locale").notOnLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {notOnLabel : "Not On"});
-
notOnNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [指定日以外] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { notOnNullText : "Not On"} } ] }); //Get var notOnNullText = $(".selector").igTreeGridFiltering("option", "locale").notOnNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {notOnNullText : "Not On"});
-
nullNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [null 以外] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { nullNullText : "Null"} } ] }); //Get var nullNullText = $(".selector").igTreeGridFiltering("option", "locale").nullNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {nullNullText : "Null"});
-
onLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [指定日] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { onLabel : "On"} } ] }); //Get var onLabel = $(".selector").igTreeGridFiltering("option", "locale").onLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {onLabel : "On"});
-
onNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [指定日] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { onNullText : "On"} } ] }); //Get var onNullText = $(".selector").igTreeGridFiltering("option", "locale").onNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {onNullText : "On"});
-
startsWithLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [~で始まる] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { startsWithLabel : "Starts With"} } ] }); //Get var startsWithLabel = $(".selector").igTreeGridFiltering("option", "locale").startsWithLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {startsWithLabel : "Starts With"});
-
startsWithNullText
継承- タイプ:
- string
- デフォルト:
- ""
フィルター エディターで使用する [~で始まる] の null テキスト。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { startsWithNullText : "Starts With"} } ] }); //Get var startsWithNullText = $(".selector").igTreeGridFiltering("option", "locale").startsWithNullText; //Set $(".selector").igTreeGridFiltering("option", "locale", {startsWithNullText : "Starts With"});
-
thisMonthLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [今月] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { thisMonthLabel : "This Month"} } ] }); //Get var thisMonthLabel = $(".selector").igTreeGridFiltering("option", "locale").thisMonthLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {thisMonthLabel : "This Month"});
-
thisYearLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [今年] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { thisYearLabel : "This Year"} } ] }); //Get var thisYearLabel = $(".selector").igTreeGridFiltering("option", "locale").thisYearLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {thisYearLabel : "This Year"});
-
todayLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [今日] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { todayLabel : "Today"} } ] }); //Get var todayLabel = $(".selector").igTreeGridFiltering("option", "locale").todayLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {todayLabel : "Today"});
-
tooltipTemplate
継承- タイプ:
- string
- デフォルト:
- ""
フィルターが適用されるときのフィルター ボタンのカスタム ツールチップ テンプレート。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { tooltipTemplate : "${condition} filter applied"} } ] }); //Get var tooltipTemplate = $(".selector").igTreeGridFiltering("option", "locale").tooltipTemplate; //Set $(".selector").igTreeGridFiltering("option", "locale", {tooltipTemplate : "${condition} filter applied"});
-
trueLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [True] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { trueLabel : "True"} } ] }); //Get var trueLabel = $(".selector").igTreeGridFiltering("option", "locale").trueLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {trueLabel : "True"});
-
yesterdayLabel
継承- タイプ:
- string
- デフォルト:
- ""
フィルター ドロップダウン内の定義済みのフィルタリング条件で使用する [昨日] ボタンのラベル。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", locale: { yesterdayLabel : "Yesterday"} } ] }); //Get var yesterdayLabel = $(".selector").igTreeGridFiltering("option", "locale").yesterdayLabel; //Set $(".selector").igTreeGridFiltering("option", "locale", {yesterdayLabel : "Yesterday"});
-
matchFiltering
- タイプ:
- string
- デフォルト:
- "__matchFiltering"
dataRow がフィルター条件と一致するかどうかを示す dataRecord オブジェクトのブール値プロパティの名前を指定します。
フィルターの場合、指定した名前を持つブール値フラグが各のデータ レコード オブジェクトに追加されます。条件と一致する場合、フラグの値は True。それ以外の場合は False。
これは内部目的のために使用されます。コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", matchFiltering: "matchFiltering1" } ] }); //Get var matchFiltering = $(".selector").igTreeGridFiltering("option", "matchFiltering");
-
mode
継承- タイプ:
- enumeration
- デフォルト:
- null
デフォルトは、非仮想化グリッド用の「simple」で、virtualization が有効になると「advance」になります。
メンバー
- simple
- タイプ:string
- フィルター行のみを描画します。
- advanced
- タイプ:string
- ダイアログで複数のフィルターの設定を許可 - Excel スタイル。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", mode : "advanced" } ] }); // Get var filterMode = $(".selector").igTreeGridFiltering("option", "mode"); // Set $(".selector").igTreeGridFiltering("option", "mode", "advanced");
-
nullTexts
削除- タイプ:
- string
- デフォルト:
- "{}"
このオプションは 2017.2 バージョン以降サポートされません。
フィルター エディターで使用する設定可能なローカライズ済みの null テキストのリスト。locale オプションを使用します。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", nullTexts: { contains: "Contains...", doesNotContain: "Does not contain...", doesNotEqual: "Does not equal...", empty: "Empty", endsWith: "Ends with..." //... } } ] }); // Get var filteringNullTexts = $(".selector").igTreeGridFiltering("option", "nullTexts"); // Set $(".selector").igTreeGridFiltering("option", "nullTexts", { contains: "Contains...", doesNotContain: "Does not contain...", doesNotEqual: "Does not equal...", empty: "Empty", endsWith: "Ends with..." //... });
-
after
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
contains
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
empty
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
equals
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
greaterThan
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
greaterThanOrEqualTo
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
lastYear
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
nextMonth
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
null
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
on
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
startsWith
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
thisMonth
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
-
persist
継承- タイプ:
- bool
- デフォルト:
- true
状態間でフィルターの永続化を有効/無効にします。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features : [ { name: "Filtering", persist: false } ] }); // Get var persist = $(".selector").igTreeGridFiltering("option", "persist"); // Set $(".selector").igTreeGridFiltering("option", "persist", false);
-
recordCountKey
- タイプ:
- string
- デフォルト:
- null
データ ソース中のレコード総数を保持する応答内のプロパティです。
コード サンプル
//Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", recordCountKey: "count" } ] }); //Get var recordCountKey = $(".selector").igTreeGridFiltering("option", "recordCountKey");
-
renderFC
継承- タイプ:
- bool
- デフォルト:
- true
機能セレクター で描画します。機能セレクターは、igGrid ですべての有効な機能 (並べ替え、フィルタリング、非表示など) をリストするダイアログです。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", renderFC: false } ] }); // Get var filteringRenderFC = $(".selector").igTreeGridFiltering("option", "renderFC"); // Set $(".selector").igTreeGridFiltering("option", "renderFC", false);
-
renderFilterButton
継承- タイプ:
- bool
- デフォルト:
- true
フィルター ボタンの表示状態を有効/無効にします。False の場合、フィルター ドロップダウン ボタンを描画し、フィルターの定義済みリストは列に描画されません。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", renderFilterButton : false } ] }); // Get var showButton = $(".selector").igTreeGridFiltering("option", "renderFilterButton"); // Set $(".selector").igTreeGridFiltering("option", "renderFilterButton", false);
-
showEmptyConditions
継承- タイプ:
- bool
- デフォルト:
- false
フィルターで空条件の表示状態を有効/無効にします。True の場合、空と空以外のフィルター条件をドロップダウンで表示します。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", showEmptyConditions : true } ] }); // Get var showConditions = $(".selector").igTreeGridFiltering("option", "showEmptyConditions"); // Set $(".selector").igTreeGridFiltering("option", "showEmptyConditions", true);
-
showNullConditions
継承- タイプ:
- bool
- デフォルト:
- false
null 値 とnull 以外の値のフィルター条件をドロップダウンでの表示状態を有効/無効にします。True の場合、null 値と null 以外の値のフィルター条件をドロップダウンで表示します。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", showNullConditions : true } ] }); // Get var showConditions = $(".selector").igTreeGridFiltering("option", "showNullConditions"); // Set $(".selector").igTreeGridFiltering("option", "showNullConditions", true);
-
toLevel
- タイプ:
- number
- デフォルト:
- -1
フィルターの適用を開始するデータ バインドされたレベルを指定します。-1 の場合、フィルターは最後のデータ バインドされたレベルに適用されます。
コード サンプル
$(".selector").igTreeGrid({ features: [ { name : "Filtering", toLevel: 0 } ] }); //Get var toLevel = $(".selector").igTreeGridFiltering("option", "toLevel");
-
tooltipTemplate
削除- タイプ:
- string
- デフォルト:
- ""
このオプションは 2017.2 バージョン以降サポートされません。
フィルターが適用されるときのフィルター ボタンのカスタム ツールチップ テンプレート。locale.tooltipTemplate オプションを使用します。コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", tooltipTemplate: "${condition} filter applied" } ] }); // Get var template = $(".selector").igTreeGridFiltering("option", "tooltipTemplate"); // Set $(".selector").igTreeGridFiltering("option", "tooltipTemplate", "${condition} filter applied");
-
type
継承- タイプ:
- enumeration
- デフォルト:
- null
フィルタリングのタイプ。すべてのフィルタリング機能を $.ig.DataSource にデリゲートします。
メンバー
- remote
- タイプ:string
- フィルタリングがリモート エンドポイントにより実行されます。
- local
- タイプ:string
- フィルタリングは $.ig.DataSource によってローカルで実行されます。
コード サンプル
// Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", type : "local" } ] }); // Get var filterType = $(".selector").igTreeGridFiltering("option", "type");
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
dataFiltered
継承- キャンセル可能:
- false
フィルタリングが実行され結果が描画された後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringdatafiltered", ".selector", function (evt, ui) { //return column key ui.columnKey; //return column index ui.columnIndex; //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return filtering expressions from the data source ui.expressions; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", dataFiltered: function (evt, ui) {...} } ] });
-
dataFiltering
継承- キャンセル可能:
- true
フィルタリング操作 (リモートまたはローカル要求) が実行される前に発生するイベント。
フィルタリング操作をキャンセルするには、false を返します。-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
GridFiltering への参照を取得します。
-
owner.gridタイプ: Object
グリッドへの参照を取得します。
-
columnIndexタイプ: Number
列のインデックスを取得します。 フィルタリング モードが "simple" の場合のみ適用可能。
-
columnKeyタイプ: String
列のキーを取得します。 フィルタリング モードが "simple" の場合のみ適用可能。
-
newExpressionsタイプ: Array
フィルタリング式を取得します。このイベント ハンドラーでフィルタリング式を変更し、その後にデータ バインディングを適用します。このように、ユーザーがデータ バインディングの前にフィルターを制御できます。
-
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringdatafiltering", ".selector", function (evt, ui) { //return column key ui.columnKey; //return column index ui.columnIndex; //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return the new filtering expressions that are going to be applied to the data source ui.newExpressions; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", dataFiltering: function (evt, ui) {...} } ] });
-
dropDownClosed
継承- キャンセル可能:
- false
フィルター列ドロップダウンが完全に閉じた後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringdropdownclosed", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dropdown html element in the DOM ui.dropDown; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", dropDownClosed: function (evt, ui) {...} } ] });
-
dropDownClosing
継承- キャンセル可能:
- true
フィルター ドロップダウンが閉じる前に発生するイベント。
ドロップダウンのクローズをキャンセルするには、false を返します。コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringdropdownclosing", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dropdown html element in the DOM ui.dropDown; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", dropDownClosing: function (evt, ui) {...} } ] });
-
dropDownOpened
継承- キャンセル可能:
- false
特定の列のフィルター ドロップダウンが開いた後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringdropdownopened", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dropdown html element in the DOM ui.dropDown; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", dropDownOpened: function (evt, ui) {...} } ] });
-
dropDownOpening
継承- キャンセル可能:
- true
フィルター ドロップダウンが特定の列に開く前に発生するイベント。
ドロップダウンのオープンをキャンセルするには、false を返します。コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringdropdownopening", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dropdown html element in the DOM ui.dropDown; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", dropDownOpening: function (evt, ui) {...} } ] });
-
filterDialogClosed
継承- キャンセル可能:
- false
詳細フィルター ダイアログが閉じた後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogclosed", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogClosed: function (evt, ui) {...} } ] });
-
filterDialogClosing
継承- キャンセル可能:
- true
詳細フィルター ダイアログが閉じる前に発生するイベント。
フィルタリング ダイアログのクローズをキャンセルするには、false を返します。コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogclosing", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogClosing: function (evt, ui) {...} } ] });
-
filterDialogContentsRendered
継承- キャンセル可能:
- false
詳細フィルター ダイアログのコンテンツが描画された後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogcontentsrendered", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dialog html element in the DOM ui.dialogElement; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogContentsRendered: function (evt, ui) {...} } ] });
-
filterDialogContentsRendering
継承- キャンセル可能:
- true
詳細フィルター ダイアログのコンテンツが描画される前に発生するイベント。
フィルタリング ダイアログの描画をキャンセルするには、false を返します。コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogcontentsrendering", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dialog html element in the DOM ui.dialogElement; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogContentsRendering: function (evt, ui) {...} } ] });
-
filterDialogFilterAdded
継承- キャンセル可能:
- false
フィルター行が詳細フィルター ダイアログに追加された後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogfilteradded", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return filters table row element in the DOM ui.filter; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogFilterAdded: function (evt, ui) {...} } ] });
-
filterDialogFilterAdding
継承- キャンセル可能:
- true
フィルター行が詳細フィルター ダイアログに追加される前に発生するイベント。
フィルターが詳細フィルタリング ダイアログへ追加するのをキャンセルするには、false を返します。コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogfilteradding", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return filters table body element in the DOM ui.filtersTableBody; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogFilterAdding: function (evt, ui) {...} } ] });
-
filterDialogFiltering
継承- キャンセル可能:
- true
詳細フィルター ダイアログの [OK] ボタンをクリックしたときに発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogfiltering", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dialog html element in the DOM ui.dialog; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogFiltering: function (evt, ui) {...} } ] });
-
filterDialogMoving
継承- キャンセル可能:
- true
詳細フィルター ダイアログの位置が変わるたびに発生するイベント。
-
evtタイプ: Event
jQuery イベント オブジェクト。
-
uiタイプ: Object
-
ownerタイプ: Object
GridFiltering への参照を取得します。
-
owner.gridタイプ: Object
グリッドへの参照を取得します。
-
dialogタイプ: jQuery
フィルタリング ダイアログの DOM 要素への参照を取得します。
-
originalPositionタイプ: Object
グループ化ダイアログ div の元の位置をページに相対して { top, left } オブジェクトとして取得します。
-
positionタイプ: Object
グループ化ダイアログ div の現在の位置をページに相対して { top, left } オブジェクトとして取得します。
-
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogmoving", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dialog html element in the DOM ui.dialog; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogMoving: function (evt, ui) {...} } ] });
-
filterDialogOpened
継承- キャンセル可能:
- false
詳細フィルター ダイアログがすでに開いた後に発生するイベント。
コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogopened", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dialog html element in the DOM ui.dialog; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogOpened: function (evt, ui) {...} } ] });
-
filterDialogOpening
継承- キャンセル可能:
- true
詳細フィルタリング ダイアログが開く前に発生するイベント。
フィルター ダイアログの開くをキャンセルするには、false を返します。コード サンプル
//Bind after initialization $(document).on("igtreegridfilteringfilterdialogopening", ".selector", function (evt, ui) { //return reference to igTreeGridFiltering ui.owner; //return reference to igTreeGrid ui.owner.grid; //return dialog html element in the DOM ui.dialog; }); //Initialize $(".selector").igTreeGrid({ features: [ { name : "Filtering", filterDialogOpening: function (evt, ui) {...} } ] });
-
changeLocale
継承- .igTreeGridFiltering( "changeLocale" );
ウィジェット要素のすべてのロケールを options.language に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。コード サンプル
$(".selector").igTreeGridFiltering("changeLocale");
-
changeRegional
継承- .igTreeGridFiltering( "changeRegional" );
ウィジェット要素の地域設定を options.regional に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。regional オプションのセッターを使用してください。コード サンプル
$(".selector").igTreeGridFiltering("changeRegional");
-
destroy
- .igTreeGridFiltering( "destroy" );
フィルタリングウィジェットを破棄 - フィルター行の削除、イベントのバインドの解除、グリッドを前の状態に戻します。
コード サンプル
$(".selector").igTreeGridFiltering("destroy");
-
filter
継承- .igTreeGridFiltering( "filter", expressions:array, [updateUI:bool] );
フィルタリングをプログラム的に適用し、デフォルトで UI を更新します。
- expressions
- タイプ:array
- フィルタリングの式の配列。それぞれが {fieldName: , expr: , cond: , logic: } の形式です。ここで、fieldName は列のキー、expr はフィルターに使用する実際の式文字列、logic は「AND」または「OR」、cond は以下の文字列の 1 つです: equals、doesNotEqual、contains、doesNotContain、greaterThan、lessThan、greaterThanOrEqualTo、lessThanOrEqualTo、true、false、null、notNull、empty、notEmpty、startsWith、endsWith、today、yesterday、on、notOn、thisMonth、lastMonth、nextMonth、before、after、thisYear、lastYear、nextYear。空と null のフィルタリング条件の違いは、空は null、NaN、未定義、および空の文字列を含んでいる点です。
- updateUI
- タイプ:bool
- オプション
- グリッドがフィルタリングされたらフィルター行も更新するかどうかを指定します。
コード サンプル
/* Expressions is an array of individual filtering expressions that the grid uses when filtering. If you only want to execute one filter criteria, then you add a single filtering expression to the array, otherwise you can add additional constraints to the filter by adding more expressions into the array. For example: [{ fieldname: "title", expr: "introduction", cond: "contains", logic: "OR" }, { fieldname: "description", expr: "introduction", cond: "contains", logic: "OR" }] Using the expressions above the applied filter returns records in the grid where either the "title" or "description" fields have values that contain the string "introduction". Note: The available values for the filtering condition are: - equals - doesNotEqual - contains - doesNotContain - greaterThan - lessThan - greaterThanOrEqualTo - lessThanOrEqualTo - true - false - null - notNull - empty - notEmpty - startsWith - endsWith - today - yesterday - on - notOn - thisMonth - lastMonth - nextMonth - before - after - this year - last year - nextYear The difference between the "empty" and "null" filtering conditions is that "empty" includes "null", "NaN", "undefined" and empty strings. Note: Available values for "logic" are "OR" and "AND". The default logic is "AND". */ $(".selector").igTreeGridFiltering("filter", ([{fieldName: "Name", expr: "Adjustable Race", cond: "equals", logic: "OR"}]));
-
getFilteringMatchesCount
- .igTreeGridFiltering( "getFilteringMatchesCount" );
- 返却型:
- number
- 返却型の説明:
- フィルターされたレコードの数。
フィルター条件と一致するデータ レコードの数を返します。
コード サンプル
$(".selector").igTreeGridFiltering("getFilteringMatchesCount");
-
requiresFilteringExpression
継承- .igTreeGridFiltering( "requiresFilteringExpression", filterCondition:string );
- 返却型:
- bool
- 返却型の説明:
- false の場合、filterCondition にフィルタリング式は必要ありません。
filterCondition がフィルタリング式を必要とするかどうかを確認します。たとえば、filterCondition が "lastMonth"、"thisMonth"、"null"、"notNull"、"true"、"false" などの場合、フィルタリング式は必要ない、など。
- filterCondition
- タイプ:string
- フィルタリング状態 - "true"、"false"、"yesterday"、"empty"、"null" など。
コード サンプル
$(".selector").igTreeGridFiltering("requiresFilteringExpression", "yesterday");
-
toggleFilterRowByFeatureChooser
継承- .igTreeGridFiltering( "toggleFilterRowByFeatureChooser", event:string );
モードが簡易または advancedModeEditorsVisible が TRUE のとき、フィルター行を切り替えます。それ以外の場合、詳細ダイアログを表示/表示にします。
- event
- タイプ:string
- 列キー。
コード サンプル
$(".buttonSelector").igButton({ labelText: $(".buttonSelector").val(), click: function (event) { $(".gridSelector").igTreeGridFiltering("toggleFilterRowByFeatureChooser", event); } });
-
ui-widget-overlay ui-iggrid-blockarea
- 詳細フィルター ダイアログが開いていて、その後ろの領域 (ブロック領域) がグレー アウトしているときに、フィルタリング ブロック領域に適用されるクラス。
-
ig-igtreegrid-filter-matching-cell
- フィルター条件と一致するセルに適用されるクラス。
-
ui-igeditor-input-container ui-corner-all
- カスタム エディター プロバイダーから提供された入力コンテナーの親 div に適用されるクラス。
-
ui-igedit ui-igedit-container ui-widget ui-corner-all ui-state-default
-
ui-icon ui-iggrid-icon-advanced-filter
- フィルターが詳細ダイアログを表示する場合、機能選択アイコンに適用されるクラス。
-
ui-iggrid-filterbutton ui-corner-all ui-icon ui-icon-triangle-1-s
- すべてのフィルタリング ドロップダウン ボタンに適用されるクラス。
-
ui-iggrid-filterbuttonactive ui-state-active
- フィルター ボタンを選択するときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbutton ui-iggrid-filterbuttonadvanced ui-icon ui-icon-search
- モードが詳細のときにボタンに適用されるクラス。これは、ボタンがヘッダーに描画されるときにもそのボタンに適用されます (デフォルト動作)。
-
ui-iggrid-filterbuttonadvancedactive ui-state-active
- 詳細ボタンを選択するときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbuttonadvanceddisabled ui-state-disabled
- 詳細ボタンが無効のときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbuttonadvancedfocus ui-state-focus
- 詳細ボタンにフォーカスがあるときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbuttonadvancedhover ui-state-hover
- 詳細ボタンがホバー状態のときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbuttonright ui-iggrid-filterbuttonadvanced ui-icon ui-icon-search
- 詳細ボタンが右側に描画されるときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbuttonbool
- ブール値フィルターが列に適用されるときに、フィルター ボタンに適用されるクラス (デフォルト)。
-
ui-iggrid-filterbuttondate
- 日付フィルターが列に定義されるときに、フィルター ボタンに適用されるクラス。
-
ui-iggrid-filterbuttondisabled ui-state-disabled
- フィルタリング ボタンが無効のときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbuttonfocus ui-state-focus
- フィルター ボタンにフォーカスがあるが選択されていないときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbuttonhover ui-state-hover
- フィルター ボタンのホバー状態のときに、そのボタンに適用されるクラス。
-
ui-iggrid-filterbuttonnumber
- 数値フィルターが列に適用されるときに、フィルター ボタンに適用されるクラス (デフォルト)。
-
ui-iggrid-filterbuttonright
- ボタンが右側に描画されるときに、簡易フィルタリング エディター コンテナーに適用されるクラス。
-
ui-iggrid-filterbuttonstring
- 文字列フィルターが列に適用されるときに、フィルター ボタンに適用されるクラス (デフォルト)。
-
ui-iggrid-filtercell
- すべてのフィルター セル TH に適用されるクラス。
-
ui-iggrid-filtereditor
- すべてのフィルター エディター要素 (igEditor) に適用されるクラス。
-
ui-dialog ui-draggable ui-resizable ui-iggrid-dialog ui-widget ui-widget-content ui-corner-all
- フィルター ダイアログ要素に適用されるクラス。
-
ui-iggrid-filterdialogaddbuttoncontainer ui-helper-reset
- フィルター ダイアログの [追加] ボタンに適用されるクラス。
-
ui-iggrid-filterdialogaddcondition
- フィルター ダイアログの追加条件領域に適用されるクラス。
-
ui-iggrid-filterdialogaddconditionlist
- フィルター ダイアログの追加条件 SELECT ドロップダウンに適用されるクラス。
-
ui-iggrid-filterdialogclearall
- フィルター ダイアログの [すべてクリア] ボタンに適用されるクラス。
-
ui-icon ui-icon-closethick
- フィルター テーブルからフィルターを削除するために使用される [X] ボタンに適用されるクラス。
-
ui-iggrid-filtertable ui-helper-reset
- フィルター ダイアログのフィルター テーブルに適用されるクラス。
-
ui-dialog-titlebar ui-iggrid-filterdialogcaption ui-widget-header ui-corner-all ui-helper-reset ui-helper-clearfix
- フィルター ダイアログのヘッダー キャプション領域に適用されるクラス。
-
ui-dialog-title
- フィルター ダイアログのヘッダー キャプション タイトルに適用されるクラス。
-
ui-dialog-buttonpane ui-widget-content ui-helper-clearfix ui-iggrid-filterdialogokcancelbuttoncontainer
- フィルター ダイアログの [OK] ボタンと [キャンセル] ボタンに適用されるクラス。
-
ui-iggrid-filterdd
- ドロップダウン UL を折り返す DIV に適用されるクラス。
-
ui-menu ui-widget ui-widget-content ui-iggrid-filterddlist ui-corner-all
- UL フィルター ドロップダウン リストに適用されるクラス。
-
ui-iggrid-filterddlistitem
- 各フィルター ドロップダウン リスト項目 (LI) に適用されるクラス。
-
ui-iggrid-filterddlistitemactive ui-state-active
- リスト項目を選択するときに、その項目に適用されるクラス。
-
ui-iggrid-filterddlistitemadvanced
- mode = "advanced" のときにエディターを表示するようにオプションが設定されている場合に詳細ボタンを保持するリスト項目に適用されるクラス。
-
ui-iggrid-filterddlistitemclear
- "clear" フィルター リスト項目に適用されるクラス。
-
ui-iggrid-filterddlistitemhover ui-state-hover
- リスト項目がホバー状態のときに、その項目に適用されるクラス。
-
ui-iggrid-filterddlistitemcontainer
- すべてのフィルター リスト項目 (LI) にテキストを保持する要素に適用されるクラス。
-
ui-iggrid-filterddlistitemicons ui-state-default
- リスト項目のフィルタリング アイコンが表示されているときに、その項目に適用されるクラス。
-
ui-iggrid-filtericon
- すべてのフィルター ドロップダウン リスト項目の画像アイコン領域に適用されるクラス。
-
ui-iggrid-filtericonafter
- 項目が after 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonbefore
- 項目が before 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonclear
- 項目が clear 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericoncontainer
- 項目アイコンのコンテナー要素に適用されるクラス。
-
ui-iggrid-filtericoncontains
- 項目が contains 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericondoesnotcontain
- 項目が doesNotContain 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericondoesnotequal
- 項目が doesNotEqual 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonendswith
- 項目が endsWith 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonequals
- 項目が contains 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonfalse
- 項目が false 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericongreaterthan
- 項目が greaterThan 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericongreaterthanorequalto
- 項目が greaterThanOrEqualTo 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonlastmonth
- 項目が lastMonth 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonlastyear
- 項目が lastYear 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonlessthan
- 項目が lessThan 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonlessthanorequalto
- 項目が lessThanOrEqualTo 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonnextmonth
- 項目が nextMonth 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonnextyear
- 項目が nextYear 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonnoton
- 項目が notOn 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonon
- 項目が on 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonstartswith
- 項目が startsWith 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonthismonth
- 項目が thisMonth 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonthisyear
- 項目が thisYear 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericontoday
- 項目が today 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericontrue
- 項目が true 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filtericonyesterday
- 項目が yesterday 条件を保持するときに、項目アイコンのスパンに適用されるクラス。
-
ui-iggrid-filterrow ui-widget
- ヘッダー テーブル内のフィルター行 TR に適用されるクラス。
-
ig-igtreegrid-filter-matching-row
- フィルター条件と一致する行に適用されるクラス。
-
ui-igtreegrid-record-not-matchfiltering
- フィルター条件と一致しない行に適用されるクラス。