ui.igDataChart
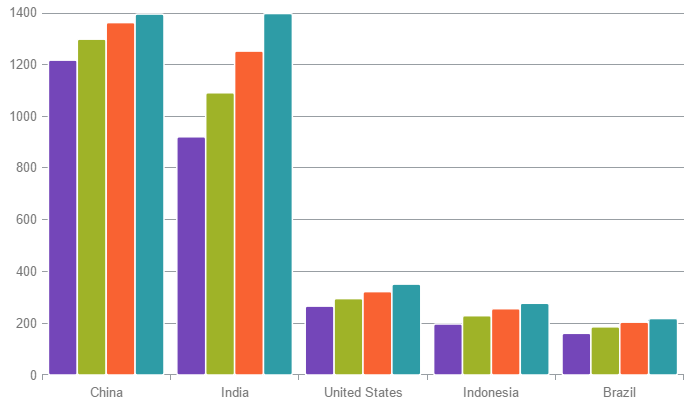
igDataChart コントロールは HTML 5 jQuery チャートです。この API のクラス、オプション、イベント、メソッド、およびテーマの詳細は、上記の関連するタブの下に表示されます。
次のコード スニペットは igDataChart コントロールの初期化方法を示しています。
igDataChart コントロールに必要なスクリプトおよびテーマの参照方法についての詳細は、 Ignite UI での JavaScript リソースの使用および Ignite UI のスタイルとテーマの設定をお読みください。コード サンプル
<!doctype html> <html> <head> <title>Ignite UI igDataChart</title> <!-- Infragistics Combined CSS --> <link href="css/themes/infragistics/infragistics.theme.css" rel="stylesheet" type="text/css" /> <link href="css/structure/infragistics.css" rel="stylesheet" type="text/css" /> <!-- jQuery Core --> <script src="js/jquery.js" type="text/javascript"></script> <!-- jQuery UI --> <script src="js/jquery-ui.js" type="text/javascript"></script> <!-- Infragistics Combined Scripts --> <script src="js/infragistics.core.js" type="text/javascript"></script> <script src="js/infragistics.dv.js" type="text/javascript"></script> <script type="text/javascript"> $(function () { var data = [ { "CountryName": "China", "Pop1995": 1216, "Pop2005": 1297, "Pop2015": 1361, "Pop2025": 1394 }, { "CountryName": "India", "Pop1995": 920, "Pop2005": 1090, "Pop2015": 1251, "Pop2025": 1396 }, { "CountryName": "United States", "Pop1995": 266, "Pop2005": 295, "Pop2015": 322, "Pop2025": 351 }, { "CountryName": "Indonesia", "Pop1995": 197, "Pop2005": 229, "Pop2015": 256, "Pop2025": 277 }, { "CountryName": "Brazil", "Pop1995": 161, "Pop2005": 186, "Pop2015": 204, "Pop2025": 218 } ]; $("#chart").igDataChart({ width: "700px", height: "400px", axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "CountryName", }, { name: "yAxis", type: "numericY", }], series: [{ name: "pop1995", dataSource: data, valueMemberPath: "Pop1995", type: "column", xAxis: "xAxis", yAxis: "yAxis" }, { name: "pop2005", dataSource: data, valueMemberPath: "Pop2005", type: "column", xAxis: "xAxis", yAxis: "yAxis", }, { name: "pop2015", dataSource: data, valueMemberPath: "Pop2015", type: "column", xAxis: "xAxis", yAxis: "yAxis", }, { name: "pop2025", dataSource: data, valueMemberPath: "Pop2025", type: "column", xAxis: "xAxis", yAxis: "yAxis", }] }); }); </script> </head> <body> <div id="chart"></div> </body> </html>
関連サンプル
関連トピック
依存関係
-
animateSeriesWhenAxisRangeChanges
- タイプ:
- bool
- デフォルト:
- false
True に設定すると、軸範囲が変更してもシリーズがアニメーションを再生しないデフォルト動作をオーバーライドできます。
コード サンプル
//Initialize $(".selector").igDataChart({ animateSeriesWhenAxisRangeChanges: true }); //Get var animate= $(".selector").igDataChart("option", "animateSeriesWhenAxisRangeChanges"); //Set $(".selector").igDataChart("option", "animateSeriesWhenAxisRangeChanges", true);
-
autoExpandMarginExtraPadding
- タイプ:
- number
- デフォルト:
- NaN
-
autoExpandMarginMaximumValue
- タイプ:
- number
- デフォルト:
- NaN
-
autoMarginHeight
- タイプ:
- number
- デフォルト:
- 0
余白をチャートに自動的に追加するときに自動的に追加する高さを取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ autoMarginHeight : 100 }); //Get var height = $(".selector").igDataChart("option", "autoMarginHeight"); //Set $(".selector").igDataChart("option", "autoMarginHeight", 100);
-
autoMarginWidth
- タイプ:
- number
- デフォルト:
- 20
余白をチャートに自動的に追加するときに自動的に追加する幅を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ autoMarginWidth : 200 }); //Get var width = $(".selector").igDataChart("option", "autoMarginWidth"); //Set $(".selector").igDataChart("option", "autoMarginWidth", 200);
-
axes
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
軸オブジェクトの配列。
コード サンプル
var data = [{ "Label": "2009", "Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, { "Label": "2010", "Item1": "Value2", "Item2": 1500, "Item3": 1180.25}, { "Label": "2011", "Item1": "Value3", "Item2": 1200, "Item3": 1205.55}, { "Label": "2012", "Item1": "Value4", "Item2": 1450, "Item3": 1144.65}]; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0]; // access 'xAxis' options axes[1]; // access 'yAxis' options // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label", labelVisibility: "collapsed" }, { name: "yAxis", type: "numericY", labelVisibility: "collapsed" }] );
-
coercionMethods
- タイプ:
- object
- デフォルト:
- null
データ ソースからデータを読み込むときに使用する強制メソッドを取得または設定します。
使用する場合、メンバー パスを設定する前に指定します。後に設定する場合、
チャートにデータを再インポートしません。コード サンプル
var data = [ { "Date": new Date(2011, 1, 1), "Value": 1000 }, { "Date": new Date(2011, 1, 2), "Value": 995 }, { "Date": new Date(2011, 1, 3), "Value": 925.5 }, { "Date": new Date(2011, 1, 4), "Value": 940.25 }, { "Date": new Date(2011, 1, 5), "Value": 1020.5 } ]; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryDateTimeX", dataSource: data, dateTimeMemberPath: "Date!parseDate", coercionMethods: { parseDate: function (val) { var newVal = new Date(val); newVal.setDate(newVal.getDate() + 4); return newVal; } } }] });
-
crossingAxis
- タイプ:
- string
- デフォルト:
- null
CrossingAxis プロパティを取得または設定します。
コード サンプル
var data = [{ "Label": "2009", "Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, { "Label": "2010", "Item1": "Value2", "Item2": 1500, "Item3": 1180.25}, { "Label": "2011", "Item1": "Value3", "Item2": 1200, "Item3": 1205.55}, { "Label": "2012", "Item1": "Value4", "Item2": 1450, "Item3": 1144.65}]; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY", crossingAxis: "xAxis", crossingValue: 700 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[1].crossingAxis; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY", crossingAxis: "xAxis", crossingValue: 700 }] );
-
crossingValue
- タイプ:
- object
- デフォルト:
- null
CrossingValue プロパティを取得または設定します。
コード サンプル
var data = [{ "Label": "2009", "Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, { "Label": "2010", "Item1": "Value2", "Item2": 1500, "Item3": 1180.25}, { "Label": "2011", "Item1": "Value3", "Item2": 1200, "Item3": 1205.55}, { "Label": "2012", "Item1": "Value4", "Item2": 1450, "Item3": 1144.65}]; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY", crossingAxis: "xAxis", crossingValue: 700 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[1].crossingValue; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY", crossingAxis: "xAxis", crossingValue: 700 }] );
-
dataSource
- タイプ:
- object
- デフォルト:
- null
$.ig.DataSource が受け入れる有効なデータ ソースまたは $.ig.DataSource 自体のインスタンスが可能です。
コード サンプル
var data = [{ "Label": "2009", "Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, { "Label": "2010", "Item1": "Value2", "Item2": 1500, "Item3": 1180.25}, { "Label": "2011", "Item1": "Value3", "Item2": 1200, "Item3": 1205.55}, { "Label": "2012", "Item1": "Value4", "Item2": 1450, "Item3": 1144.65}]; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].dataSource; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }] );
-
dataSourceType
- タイプ:
- string
- デフォルト:
- null
データ ソースのタイプ ("json" など) を明示的に設定します。$.ig.DataSource とそのタイプ プロパティのドキュメントを参照してください。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, dataSourceType: "json" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].dataSourceType; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSource: data, dataSourceType: "json" }] );
-
dataSourceUrl
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource からデータを要求するには、$.ig.DataSource により承諾されたリモート URL を指定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSourceUrl: "http://www.example.com/chart-data" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].dataSourceUrl; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSourceUrl: "http://www.example.com/chart-data" }] );
-
dateTimeMemberPath
- タイプ:
- string
- デフォルト:
- null
CategoryDateTimeXAxis の DateTime マッピング プロパティを取得または設定します。
コード サンプル
var data = [ { "Date": new Date(2011, 1, 1), "Value": 1000 }, { "Date": new Date(2011, 1, 2), "Value": 995 }, { "Date": new Date(2011, 1, 3), "Value": 925.5 }, { "Date": new Date(2011, 1, 4), "Value": 940.25 }, { "Date": new Date(2011, 1, 5), "Value": 1020.5 } ]; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryDateTimeX", dataSource: data, dateTimeMemberPath: "Date" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].dateTimeMemberPath; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryDateTimeX", dataSource: data, dateTimeMemberPath: "Date" }] );
-
displayType
- タイプ:
- enumeration
- デフォルト:
- null
軸の表示タイプを取得または設定します。Continuous 表示タイプでは軸を均等間隔に分割します。ラベルは必ずしもデータ ポイントに合わせる必要はありません。Discrete 表示タイプでは均等間隔を使用しませんが、各ラベルはデータ ポイントに合わせます。
メンバー
- continuous
- タイプ:string
- 特定の点にデータが存在しない場合でも、各点は均等な間隔で表示されます。
- discrete
- タイプ:string
- データが存在する場合、点は均等でない間隔で表示される可能性があります。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, displayType: "continuous" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].displayType; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSource: data, displayType: "continuous" }] );
-
enhancedIntervalMinimumCharacters
- タイプ:
- number
- デフォルト:
- 5
詳細なラベル ヒューリスティックを使用の場合、横軸に表示される最小文字数を取得または設定します。-1 を使用して、横軸にフィットするように間隔を調整します。
-
formatLabel
- タイプ:
- object
- デフォルト:
- null
オブジェクトを取得して、チャートの表示のために書式設定されるラベルを作成する関数を取得または設定します。
コード サンプル
var data = [ { "Date": new Date(2011, 1, 1), "Value": 1000 }, { "Date": new Date(2011, 1, 2), "Value": 995 }, { "Date": new Date(2011, 1, 3), "Value": 925.5 }, { "Date": new Date(2011, 1, 4), "Value": 940.25 }, { "Date": new Date(2011, 1, 5), "Value": 1020.5 } ]; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryDateTimeX", dataSource: data, label: "Date", formatLabel: function (item) { var ret = ''; ret += item.getFullYear(); ret += "/"; ret += item.getMonth() + 1; ret += "/"; ret += item.getDate(); return ret; } }] });
-
gap
- タイプ:
- number
- デフォルト:
- 0
現在の軸オブジェクトの隣接カテゴリ間のスペース量を取得または設定します。
使用される場合、スペースが範囲 [0, 1] に制限されます。コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", gap: 1 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].gap; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", gap: 1 }] );
-
innerRadiusExtentScale
- タイプ:
- number
- デフォルト:
- 0
チャートの中心を空白にするために最大半径範囲のパーセンテージを定義します。これは
0.0 ~ 1.0 の値である必要があります。コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", innerRadiusExtentScale: 0.15 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].innerRadiusExtentScale; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", innerRadiusExtentScale: 0.15 }] );
-
interval
- タイプ:
- number
- デフォルト:
- 0
表示するラベルの間隔を取得または設定します。
どのラベルを非表示にするかは、設定した値で決まります。たとえば、間隔 2 では 1 ラベルおきに表示します。コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", interval: 2 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].interval; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", interval: 2 }] );
-
isDataPreSorted
- タイプ:
- bool
- デフォルト:
- false
日時軸に割り当てられたデータが日時により並べ替えたかどうかを取得または設定します。
-
isInverted
- タイプ:
- bool
- デフォルト:
- false
IsInverted プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", isInverted: true }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].isInverted; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", isInverted: true }] );
-
isLogarithmic
- タイプ:
- bool
- デフォルト:
- false
IsLogarithmic プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", isLogarithmic: true }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].isLogarithmic; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", isLogarithmic: true }] );
-
label
- タイプ:
- object
- デフォルト:
- null
軸ラベルに使用するラベル マッピング プロパティを取得または設定します。
コード サンプル
var data = [{ "Label": "2009", "Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, { "Label": "2010", "Item1": "Value2", "Item2": 1500, "Item3": 1180.25}, { "Label": "2011", "Item1": "Value3", "Item2": 1200, "Item3": 1205.55}, { "Label": "2012", "Item1": "Value4", "Item2": 1450, "Item3": 1144.65}]; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].label; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }] );
-
labelAngle
- タイプ:
- number
- デフォルト:
- 0
軸のラベルが反転される角度を指定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelAngle: 30 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelAngle; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", labelAngle: 30 }] );
-
labelBottomMargin
- タイプ:
- number
- デフォルト:
- 0
ラベルに使用する下マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelBottomMargin: 10 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelBottomMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", labelBottomMargin: 10 }] );
-
labelExtent
- タイプ:
- number
- デフォルト:
- null
この軸のラベル領域の範囲を指定します。指定されない場合、この値が自動的に計算されます。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelExtent: 10 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelExtent; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", labelExtent: 10 }] );
-
labelHorizontalAlignment
- タイプ:
- enumeration
- デフォルト:
- right
ラベルに使用する水平方向。垂直方向の軸のみに適用できます。
メンバー
- left
- タイプ:string
- ラベルを左揃えにします。
- center
- タイプ:string
- ラベルを中央揃えにします。
- right
- タイプ:string
- ラベルを右揃えにします。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "yAxis", type: "numericY", labelHorizontalAlignment: "right" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelHorizontalAlignment; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", labelHorizontalAlignment: "right" }] );
-
labelLeftMargin
- タイプ:
- number
- デフォルト:
- 0
ラベルに使用する左マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelLeftMargin: 10 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelLeftMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", labelLeftMargin: 10 }] );
-
labelLocation
- タイプ:
- enumeration
- デフォルト:
- null
この軸の軸ラベルを表示する場所を指定します。
メンバー
- outsideTop
- タイプ:string
- ラベルには外側上部の位置がある必要があります。
- outsideBottom
- タイプ:string
- ラベルには外側下部の位置がある必要があります。
- outsideLeft
- タイプ:string
- ラベルには外側左の位置がある必要があります。
- outsideRight
- タイプ:string
- ラベルには外側右の位置がある必要があります。
- insideTop
- タイプ:string
- ラベルに内側上部の配置がある必要があります。
- insideBottom
- タイプ:string
- ラベルには内側下部の位置がある必要があります。
- insideLeft
- タイプ:string
- ラベルには内側左の位置がある必要があります。
- insideRight
- タイプ:string
- ラベルには内側右の位置がある必要があります。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelLocation: "outsideBottom" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelLocation; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", labelLocation: "outsideBottom" }] );
-
labelMargin
- タイプ:
- number
- デフォルト:
- 0
ラベルに使用するマージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelMargin: 5 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", labelMargin: 5 }] );
-
labelRightMargin
- タイプ:
- number
- デフォルト:
- 0
ラベルに使用する右マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelRightMargin: 10 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelRightMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", labelRightMargin: 10 }] );
-
labelTextColor
- タイプ:
- string
- デフォルト:
- null
軸ラベルのテキスト色をオーバーライドします。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelTextColor: "blue" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelTextColor; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", labelTextColor: "blue" }] );
-
labelTextStyle
- タイプ:
- string
- デフォルト:
- null
軸ラベルのテキスト スタイルをオーバーライドします。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelTextStyle: "8pt Helvetica, Arial, sans-serif" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelTextStyle; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", labelTextStyle: "8pt Helvetica, Arial, sans-serif" }] );
-
labelTopMargin
- タイプ:
- number
- デフォルト:
- 0
ラベルに使用する上マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelTopMargin: 10 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelTopMargin; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", labelTopMargin: 10 }] );
-
labelVerticalAlignment
- タイプ:
- enumeration
- デフォルト:
- top
ラベルに使用する垂直方向。水平方向の軸のみに適用できます。
メンバー
- top
- タイプ:string
- ラベルを上揃えにします。
- center
- タイプ:string
- ラベルを中央揃えにします。
- bottom
- タイプ:string
- ラベルを下揃えにします。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelVerticalAlignment: "center" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelVerticalAlignment; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", labelVerticalAlignment: "center" }] );
-
labelVisibility
- タイプ:
- enumeration
- デフォルト:
- visible
ラベルが可視かどうかを指定します。
メンバー
- visible
- タイプ:string
- この軸のラベルが可視である必要があります。
- collapsed
- タイプ:string
- ラベルを軸に表示しません。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", labelVisibility: "collapsed" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].labelVisibility; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", labelVisibility: "collapsed" }] );
-
logarithmBase
- タイプ:
- number
- デフォルト:
- 10
LogarithmBase プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", logarithmBase: 2 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].logarithmBase; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", logarithmBase: 2 }] );
-
majorStroke
- タイプ:
- string
- デフォルト:
- null
MajorStroke プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", majorStroke: "blue" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].majorStroke; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", majorStroke: "blue" }] );
-
majorStrokeThickness
- タイプ:
- number
- デフォルト:
- 1
MajorStrokeThickness プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", majorStrokeThickness: 5 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].majorStrokeThickness; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", majorStrokeThickness: 5 }] );
-
maximumValue
- タイプ:
- enumeration
- デフォルト:
- 0
軸 MaximumValue を取得または設定します。
メンバー
- number
- タイプ:number
- 軸が数値型の場合、最大値を数値に設定できます。
- date
- タイプ:date
- type オプションが "categoryDateTimeX" に設定される場合、最大値を日付オブジェクトに設定できます。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", maximumValue: 1500 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].maximumValue; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", maximumValue: 1500 }] );
-
minimumValue
- タイプ:
- enumeration
- デフォルト:
- 0
軸 MinimumValue を取得または設定します。
メンバー
- number
- タイプ:number
- 軸が数値型の場合、最小値を数値に設定できます。
- date
- タイプ:date
- type オプションが "categoryDateTimeX" に設定される場合、最小値を日付オブジェクトに設定できます。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", minimumValue: 500 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].minimumValue; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", minimumValue: 500 }] );
-
minorStroke
- タイプ:
- string
- デフォルト:
- null
MinorStroke プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", minorStroke: "grey" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].minorStroke; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", minorStroke: "grey" }] );
-
minorStrokeThickness
- タイプ:
- number
- デフォルト:
- 1
MinorStrokeThickness プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", minorStrokeThickness: 2 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].minorStrokeThickness; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", minorStrokeThickness: 2 }] );
-
name
- タイプ:
- string
- デフォルト:
- null
軸の一意の識別子。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].name; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX" }] );
-
overlap
- タイプ:
- number
- デフォルト:
- 0
現在の軸オブジェクトの隣接カテゴリ間の重複の量を取得または設定します。
重複は、使用時に範囲 [-1, 1] に通知なしで固定します。コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", overlap: 0.5 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].overlap; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", overlap: 0.5 }] );
-
radiusExtentScale
- タイプ:
- number
- デフォルト:
- 0.75
最大半径として使用する最大半径の範囲のパーセンテージを定義します。これは
0.0 ~ 1.0 の値である必要があります。コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", radiusExtentScale: 0.5 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].radiusExtentScale; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", radiusExtentScale: 0.5 }] );
-
referenceValue
- タイプ:
- number
- デフォルト:
- 0
ReferenceValue プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", referenceValue: 0.5 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].referenceValue; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", referenceValue: 0.5 }] );
-
remove
- タイプ:
- bool
- デフォルト:
- false
チャートから依存の軸を名前によって削除するには、true に設定します。
コード サンプル
//Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", remove: true }] );
-
responseDataKey
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource を参照してください。応答がラップされる場合に、データ レコードが保持されるプロパティの名前を指定します。
コード サンプル
var data = { "Records": [{ "Label": "2009", "Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, { "Label": "2010", "Item1": "Value2", "Item2": 1500, "Item3": 1180.25}, { "Label": "2011", "Item1": "Value3", "Item2": 1200, "Item3": 1205.55}, { "Label": "2012", "Item1": "Value4", "Item2": 1450, "Item3": 1144.65}] }; // Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, responseDataKey: "Records" }, { name: "yAxis", type: "numericY" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].responseDataKey; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", dataSource: data, responseDataKey: "Records" }] );
-
showFirstLabel
- タイプ:
- bool
- デフォルト:
- true
軸の最初のラベルを表示するかどうかを設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", showFirstLabel: false }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].showFirstLabel; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", showFirstLabel: false }] );
-
startAngleOffset
- タイプ:
- number
- デフォルト:
- 0
チャートの角度 0 度をオフセットする角度を示します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", startAngleOffset: 30 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].startAngleOffset; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", startAngleOffset: 30 }] );
-
strip
- タイプ:
- string
- デフォルト:
- null
Strip プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", strip: "grey" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].strip; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", strip: "grey" }] );
-
stroke
- タイプ:
- string
- デフォルト:
- null
Stroke プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", stroke: "blue" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].stroke; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX", stroke: "blue" }] );
-
strokeThickness
- タイプ:
- number
- デフォルト:
- 1
StrokeThickness プロパティを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", strokeThickness: 5 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].strokeThickness; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", strokeThickness: 5 }] );
-
tickLength
- タイプ:
- number
- デフォルト:
- 0
この軸に表示する目盛りの長さ。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", tickLength: 5 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].tickLength; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", tickLength: 5 }] );
-
tickStroke
- タイプ:
- object
- デフォルト:
- black
目盛りに使用する色。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", tickStroke: "blue" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].tickStroke; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", tickStroke: "blue" }] );
-
tickStrokeThickness
- タイプ:
- number
- デフォルト:
- 0.5
目盛りに使用するストロークの太さ。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", tickStrokeThickness: 2 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].tickStrokeThickness; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", tickStrokeThickness: 2 }] );
-
title
- タイプ:
- string
- デフォルト:
- null
コンポーネントに表示するタイトル。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].title; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years" }] );
-
titleAngle
- タイプ:
- number
- デフォルト:
- 0
軸タイトルに使用する角度。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleAngle: 90 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleAngle; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleAngle: 90 }] );
-
titleBottomMargin
- タイプ:
- number
- デフォルト:
- 0
タイトルに使用する下マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleBottomMargin:20 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleBottomMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleBottomMargin: 20 }] );
-
titleHorizontalAlignment
- タイプ:
- enumeration
- デフォルト:
- center
タイトルに使用する水平方向。
メンバー
- left
- タイプ:string
- タイトルを左揃えにします。
- center
- タイプ:string
- タイトルを中央揃えにします。
- right
- タイプ:string
- タイトルを右揃えにします。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleHorizontalAlignment: "left" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleHorizontalAlignment; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleHorizontalAlignment: "left" }] );
-
titleLeftMargin
- タイプ:
- number
- デフォルト:
- 0
タイトルに使用する左マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleLeftMargin: 20 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleLeftMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleLeftMargin: 20 }] );
-
titleMargin
- タイプ:
- number
- デフォルト:
- 0
軸タイトルの周りに表示するマージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleMargin: {top:20 , bottom:40, left:45, right:23} }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleMargin: {top:20 , bottom:40, left:45, right:23} }] );
-
titlePosition
- タイプ:
- enumeration
- デフォルト:
- auto
タイトルに使用する位置。
メンバー
- auto
- タイプ:string
- タイトルが自動的に配置されます。
- left
- タイプ:string
- タイトルがラベルの左側に配置されます。
- right
- タイプ:string
- タイトルがラベルの右側に配置されます。
- top
- タイプ:string
- タイトルがラベルの上側に配置されます。
- bottom
- タイプ:string
- タイトルがラベルの下側に配置されます。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titlePosition: "top" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titlePosition; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titlePosition: "top" }] );
-
titleRightMargin
- タイプ:
- number
- デフォルト:
- 0
タイトルに使用する右マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleRightMargin: 20 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleRightMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleRightMargin: 20 }] );
-
titleTextStyle
- タイプ:
- string
- デフォルト:
- null
タイトルに使用する css フォント プロパティ。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleTextStyle: "26pt Times New Roman|Georgia|Serif" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleTextStyle; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleTextStyle: "26pt Times New Roman|Georgia|Serif" }] );
-
titleTopMargin
- タイプ:
- number
- デフォルト:
- 0
タイトルに使用する上マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleTopMargin: 20 }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleTopMargin; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleTopMargin: 20 }] );
-
titleVerticalAlignment
- タイプ:
- enumeration
- デフォルト:
- center
タイトルに使用する垂直方向。
メンバー
- center
- タイプ:string
- タイトルを中央揃えにします。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX", title: "Years", titleVerticalAlignment: "center" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].titleVerticalAlignment; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "numericX", title: "Years", titleVerticalAlignment: "center" }] );
-
type
- タイプ:
- enumeration
- デフォルト:
- null
軸のタイプ。
メンバー
- numericX
- タイプ:string
- 数値 X 軸として軸を指定します。散布図、カテゴリ、および財務価格シリーズの表示に役立ちます。
- numericY
- タイプ:string
- 数値 Y 軸として軸を指定します。散布図、カテゴリ、および財務価格シリーズの表示に役立ちます。
- categoryX
- タイプ:string
- カテゴリ X 軸として軸を指定します。散布図、カテゴリ、および財務価格シリーズの表示に役立ちます。
- categoryDateTimeX
- タイプ:string
- カテゴリ datetime X 軸として軸を指定します。日付データのカテゴリ、および財務価格シリーズの表示に役立ちます。
- categoryY
- タイプ:string
- カテゴリ Y 軸として軸を指定します。散布図、カテゴリ、および財務価格シリーズの表示に役立ちます。
- categoryAngle
- タイプ:string
- カテゴリ角度軸として軸を指定します。ポーラおよびラジアル カテゴリの表示に便利です。
- numericAngle
- タイプ:string
- 数値角度軸として軸を指定します。ポーラおよびラジアル シリーズの表示に便利です。
- numericRadius
- タイプ:string
- 数値半径軸として軸を指定します。ポーラおよびラジアル シリーズの表示に便利です。
- time
- タイプ:string
- 時間 X 軸として軸を指定します。時間ブレークを持つデータに基づいて日付の表示で便利です。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].type; // Set $(".selector").igDataChart("option", "axes", blue [{ name: "xAxis", type: "numericX" }] );
-
useClusteringMode
- タイプ:
- bool
- デフォルト:
- false
クラスタリング モードを使用する必要な系列がない場合、カテゴリ軸がクラスタリング モードを使用するかどうかを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", useClusteringMode: true }] }); // Get var axes = $(".selector").igDataChart("option", "axes"); axes[0].useClusteringMode; // Set $(".selector").igDataChart("option", "axes", [{ name: "xAxis", type: "categoryX", useClusteringMode: true }] );
-
useEnhancedIntervalManagement
- タイプ:
- bool
- デフォルト:
- false
競合解決などの前に、初期状態で描画されるラベルの数を決定する際により詳細なヒューリスティックが使用されるかどうかを取得または設定します。
-
bottomMargin
- タイプ:
- number
- デフォルト:
- NaN
キャンバスでチャート コンテンツの周囲で使用する下余白を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ bottomMargin : 20 }); //Get var margin = $(".selector").igDataChart("option", "bottomMargin"); //Set $(".selector").igDataChart("option", "bottomMargin", 20);
-
brushes
- タイプ:
- object
- デフォルト:
- null
Brushes プロパティを取得または設定します。
自動的に割り当てられる系列ブラシを選択するパレットは、ブラシ プロパティで定義します。
提供された値は、css 色文字列の配列である必要があります。最初の要素は、コレクションの補間モードを指定する RGB または HSV の文字列に設定するオプションがあります。コード サンプル
// Initialization $(".selector").igDataChart({ brushes: [ "#ff0000", "#ffff00", "#00ffff" ] }); // Get var brushes = $(".selector").igDataChart("option", "brushes"); // Set $(".selector").igDataChart("option", "brushes", [ "#ff0000", "#ffff00", "#00ffff" ]);
-
circleMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャートに円マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
circle のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ circleMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
computedPlotAreaMarginMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- series
- タイプ:string
- none
- タイプ:string
-
contentHitTestMode
- タイプ:
- enumeration
- デフォルト:
- auto
シリーズが使用するヒット テストのタイプを取得または設定します。
メンバー
- auto
- タイプ:string
- シリーズの適切なヒット テスト モードを自動的に決定します。
- computational
- タイプ:string
- ヒットされたシリーズを決定するために計算に基づく方法を使用します。これはゆるやかな境界ボックスを使用し、ヒットを検索する時間計算量の範囲は O(1) から O(log n) までです。色エンコードと比較してフレームの描画時間を減少させます。
- colorEncoded
- タイプ:string
- 色エンコードされた画面に表示されていないバッファーを使用してヒット テストを実行します。色バッファーでアンチエイリアシングを無効にできないため、間違ったシリーズがヒットする誤検知の可能性はとても低く、ヒット シリーズの決定時間はいつも O(1) 時間です。ただし、フレームの描画時間が長くなります。ヒット テストでパフォーマンスの低下がある場合、この方法を使用できます。
- mixed
- タイプ:string
- 各シリーズが自分のヒット テスト モードに基づいて使用するヒット テスト モードを決定します。
- mixedFavoringComputational
- タイプ:string
- 各シリーズが自分のヒット テスト モードに基づいて使用するヒット テスト モードを決定しますが、すべての計算ヒットを色エンコード ヒットの前に評価します。
コード サンプル
// Initialization $(".selector").igDataChart({ contentHitTestMode: "auto" }); // Get var mode= $(".selector").igDataChart("option", "contentHitTestMode"); // Set $(".selector").igDataChart("option", "contentHitTestMode", "auto");
-
crosshairPoint
- タイプ:
- object
- デフォルト:
- {}
十字線の位置 (ワールド座標値) を取得または設定します
十字線の X または Y のいずれかまたは両方を double.NaN に設定することができます。
その場合、該当する十字線は非表示になります。コード サンプル
// Initialization $(".selector").igDataChart({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igDataChart("option", "crosshairPoint"); // Set $(".selector").igDataChart("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
x
- タイプ:
- number
- デフォルト:
- NaN
x 座標
コード サンプル
// Initialization $(".selector").igDataChart({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igDataChart("option", "crosshairPoint"); var x = crosshairPoint.x; // Set $(".selector").igDataChart("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
y
- タイプ:
- number
- デフォルト:
- NaN
y 座標
コード サンプル
// Initialization $(".selector").igDataChart({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igDataChart("option", "crosshairPoint"); var y = crosshairPoint.y; // Set $(".selector").igDataChart("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
crosshairVisibility
- タイプ:
- enumeration
- デフォルト:
- collapsed
現在の Chart の十字線の表示/非表示のオーバーライドを取得または設定します。注: このプロパティの設定はチャートのモバイル ブラウザー バージョンに影響しません。
メンバー
- visible
- タイプ:string
- 十字線を表示します。
- collapsed
- タイプ:string
- 十字線を表示しません。
コード サンプル
// Initialization $(".selector").igDataChart({ crosshairVisibility: "collapsed" }); // Get var opValue = $(".selector").igDataChart("option", "crosshairVisibility"); // Set $(".selector").igDataChart("option", "crosshairVisibility", "collapsed");
-
dataSource
- タイプ:
- object
- デフォルト:
- null
$.ig.DataSource が受け入れる有効なデータ ソースまたは $.ig.DataSource 自体のインスタンスが可能です。
コード サンプル
// Initialization var data1 = [{"ID": 1, "Cost": 12.5}, {"ID": 2, "Cost": 18.56}, {"ID": 3, "Cost": 22.18}]; $(".selector").igDataChart({ dataSource: data1 }); // Get var dataSource = $(".selector").igDataChart("option", "dataSource"); // Set var data1 = [{"ID": 1, "Cost": 12.5}, {"ID": 2, "Cost": 18.56}, {"ID": 3, "Cost": 22.18}]; $(".selector").igDataChart("option", "dataSource", data1);
-
dataSourceType
- タイプ:
- string
- デフォルト:
- null
データ ソースのタイプ ("json" など) を明示的に設定します。$.ig.DataSource とそのタイプ プロパティのドキュメントを参照してください。
コード サンプル
// Initialization $(".selector").igDataChart({ dataSourceType: "array" }); // Get var opValue = $(".selector").igDataChart("option", "dataSourceType"); // Set $(".selector").igDataChart("option", "dataSourceType", "array");
-
dataSourceUrl
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource からデータを要求するには、$.ig.DataSource により承諾されたリモート URL を指定します。
コード サンプル
// Initialization $(".selector").igDataChart({ dataSourceUrl: "http://www.example.com" }); // Get var opValue = $(".selector").igDataChart("option", "dataSourceUrl"); // Set $(".selector").igDataChart("option", "dataSourceUrl", "http://www.example.com");
-
defaultInteraction
- タイプ:
- enumeration
- デフォルト:
- dragZoom
DefaultInteraction プロパティを取得または設定します。デフォルトの操作状態により、マウス イベントに対するチャートの応答が定義されます。
メンバー
- none
- タイプ:string
- ユーザー動作はチャートの状態を変更しません。
- dragZoom
- タイプ:string
- ユーザー動作はチャートをズームするために矩形のドラッグを開始します。
- dragPan
- タイプ:string
- チャートのウインドウを移動するためにユーザー操作でパン操作を開始します。
コード サンプル
// Initialization $(".selector").igDataChart({ defaultInteraction: "dragZoom" }); // Get var opValue = $(".selector").igDataChart("option", "defaultInteraction"); // Set $(".selector").igDataChart("option", "defaultInteraction", "dragZoom");
-
diamondMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上のひし形マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
diamond のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ diamondMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
dragModifier
- タイプ:
- enumeration
- デフォルト:
- none
現在の Chart の DragModifier プロパティを取得または設定します。
メンバー
- none
- タイプ:string
- 修飾キーが設定されません。
- alt
- タイプ:string
- 修飾キーは alt キーに設定されます。
- control
- タイプ:string
- 修飾キーは control キーに設定されます。
- shift
- タイプ:string
- 修飾キーは shift キーに設定されます。
コード サンプル
// Initialization $(".selector").igDataChart({ dragModifier: "control" }); // Get var opValue = $(".selector").igDataChart("option", "dragModifier"); // Set $(".selector").igDataChart("option", "dragModifier", "control");
-
gridMode
- タイプ:
- enumeration
- デフォルト:
- behindSeries
GridMode プロパティを取得または設定します。
メンバー
- none
- タイプ:string
- チャート グリッドが見つかりません。
- beforeSeries
- タイプ:string
- チャート グリッドはデータ シリーズの前に描画する必要があります。
- behindSeries
- タイプ:string
- チャート グリッドはデータ シリーズの後ろに描画する必要があります。
コード サンプル
// Initialization $(".selector").igDataChart({ gridMode: "beforeSeries" }); // Get var opValue = $(".selector").igDataChart("option", "gridMode"); // Set $(".selector").igDataChart("option", "gridMode", "beforeSeries");
-
height
- タイプ:
- enumeration
- デフォルト:
- null
チャートの高さ。ピクセル、文字列 (px)、またはパーセンテージ (%) で数字として設定できます。
コード サンプル
// Initialization $(".selector").igDataChart({ height: 250 }); // Get var opValue = $(".selector").igDataChart("option", "height"); // Set $(".selector").igDataChart("option", "height", 250);
-
hexagonMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上の六角形マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
hexagon のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ hexagonMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
hexagramMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上のヘキサグラム マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
hexagram のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ hexagramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
highlightingBehavior
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- directlyOver
- タイプ:string
- nearestItems
- タイプ:string
- nearestItemsRetainMainShapes
- タイプ:string
- nearestItemsAndSeries
- タイプ:string
-
highlightingMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- none
- タイプ:string
- brightenSpecific
- タイプ:string
- brighten
- タイプ:string
- fadeOthersSpecific
- タイプ:string
- fadeOthers
- タイプ:string
-
highlightingTransitionDuration
- タイプ:
- number
- デフォルト:
- 300
強調表示トランジションの期間 (ミリ秒)。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ width: "100%", height: "400px", legend: { element: "lineLegend" }, title: "Population per Country", subtitle: "A comparision of population in 1995 and 2005", dataSource: data, axes: [ { name: "NameAxis", type: "categoryX", label: "CountryName" }, { name: "PopulationAxis", type: "numericY" } ], series: [ { name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isHighlightingEnabled: true, highlightingTransitionDuration: 500 } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].highlightingTransitionDuration; //Set $(".selector").igDataChart("option", "series", [{ name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", highlightingTransitionDuration: 500 }] );
-
horizontalZoomable
非推奨- タイプ:
- bool
- デフォルト:
- false
現在の Chart の水平ズーム可否を取得または設定します。このオプションは非推奨です。代わりに isHorizontalZoomEnabled を使用してください。
コード サンプル
// Initialization $(".selector").igDataChart({ horizontalZoomable: true }); // Get var opValue = $(".selector").igDataChart("option", "horizontalZoomable"); // Set $(".selector").igDataChart("option", "horizontalZoomable", true);
-
isHorizontalZoomEnabled
- タイプ:
- bool
- デフォルト:
- false
現在の Chart の水平ズーム可否を取得または設定します。
-
isPagePanningAllowed
- タイプ:
- bool
- デフォルト:
- true
要求された方向へコントロールをパンニングできない場合、シリーズ ビューアーがページのパンニングを許可するかどうかを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ isPagePanningAllowed: true }); // Get var opValue = $(".selector").igDataChart("option", "isPagePanningAllowed"); // Set $(".selector").igDataChart("option", "isPagePanningAllowed", true);
-
isSquare
- タイプ:
- bool
- デフォルト:
- false
チャートで四角の縦横比を使用するかどうかを取得または設定します。極座標およびラジアル チャートで true にロックされます。
コード サンプル
// Initialization $(".selector").igDataChart({ isSquare: true }); // Get var opValue = $(".selector").igDataChart("option", "isSquare"); // Set $(".selector").igDataChart("option", "isSquare", true);
-
isSurfaceInteractionDisabled
- タイプ:
- bool
- デフォルト:
- false
True に設定すると、プロット画面との操作を無効にします。
コード サンプル
// Initialization $(".selector").igDataChart({ isSurfaceInteractionDisabled: true }); // Get var opValue = $(".selector").igDataChart("option", "isSurfaceInteractionDisabled"); // Set $(".selector").igDataChart("option", "isSurfaceInteractionDisabled", true);
-
isVerticalZoomEnabled
- タイプ:
- bool
- デフォルト:
- false
現在の Chart の垂直ズーム可否を取得または設定します。
-
language
継承- タイプ:
- string
- デフォルト:
- "en"
ウィジェットのロケール言語設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ language: "ja" }); // Get var language = $(".selector").igDataChart("option", "language"); // Set $(".selector").igDataChart("option", "language", "ja");
-
leftMargin
- タイプ:
- number
- デフォルト:
- NaN
キャンバスでチャート コンテンツの周囲で使用する左余白を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ leftMargin : 20 }); //Get var margin = $(".selector").igDataChart("option", "leftMargin"); //Set $(".selector").igDataChart("option", "leftMargin", 20);
-
legend
- タイプ:
- object
- デフォルト:
- {}
$.ig.ChartLegend が受け入れる有効なオプションまたは $.ig.ChartLegend 自体のインスタンスが可能です。
コード サンプル
// Initialize $(".selector").igDataChart({ legend: { element: 'legend', type: 'legend' } }); // Get var legend = $(".selector").igDataChart("option", "legend"); // Set $(".selector").igDataChart("option", "legend", { element: 'legend', type: 'legend' });
-
element
- タイプ:
- string
- デフォルト:
- null
凡例に変更する要素の名前。
コード サンプル
// Initialize $(".selector").igDataChart({ legend: { element: "legend", type: "legend" } }); // Get var legend = $(".selector").igDataChart("option", "legend"); legend.element;
-
height
- タイプ:
- enumeration
- デフォルト:
- null
凡例の高さ。null の場合、他の高さが定義されていないときにデータにフィットするように垂直方向に伸びます。
メンバー
- null
- タイプ:object
- string
- ウィジェットの高さはピクセル (px) およびパーセント (%) で設定できます (%)。
- number
- 幅の高さは数値 (25) として設定できます。
コード サンプル
// Initialize $(".selector").igDataChart({ legend: { element: "legend", type: "legend", height: "100px" } }); // Get var legend = $(".selector").igDataChart("option", "legend"); legend.height;
-
type
- タイプ:
- enumeration
- デフォルト:
- legend
凡例のタイプ。
メンバー
- item
- タイプ:string
- 凡例を項目の凡例として指定します。igPieChart コントロール内の各パイに対して凡例の項目を表示します。
- legend
- タイプ:string
- 凡例を凡例として指定します。これは、igDataChart コントロールのすべてのタイプのシリーズによってサポートされます。
コード サンプル
// Initialize $(".selector").igDataChart({ legend: { element: "legend", type: "legend" } }); // Get var legend = $(".selector").igDataChart("option", "legend"); legend.type;
-
width
- タイプ:
- enumeration
- デフォルト:
- null
凡例の幅。
メンバー
- null
- タイプ:object
- 他の幅が定義されていない場合、データがフィットするよう伸縮します。
- string
- ウィジェットの幅はピクセル (px) およびパーセント (%) で設定できます (%)。
- number
- ウィジェット幅は数値として設定できます。
コード サンプル
// Initialize $(".selector").igDataChart({ legend: { element: "legend", type: "legend", width: "100px" } }); //Get var legend = $(".selector").igDataChart("option", "legend"); legend.width;
-
legendHighlightingMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- none
- タイプ:string
- matchSeries
- タイプ:string
-
legendItemBadgeMode
- タイプ:
- string
- デフォルト:
- "simplified"
-
legendItemBadgeShape
- タイプ:
- string
- デフォルト:
- "automatic"
-
locale
継承- タイプ:
- object
- デフォルト:
- null
ウィジェットのロケール設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ locale: {} }); // Get var locale = $(".selector").igDataChart("option", "locale"); // Set $(".selector").igDataChart("option", "locale", {});
-
markerBrushes
- タイプ:
- object
- デフォルト:
- null
MarkerBrushes プロパティを取得または設定します。
マーカー ブラシ プロパティは自動的に割り当てられるマーカー ブラシを選択するパレットを定義します。
提供された値は、css 色文字列の配列である必要があります。最初の要素は、コレクションの補間モードを指定する RGB または HSV の文字列に設定するオプションがあります。コード サンプル
// Initialization $(".selector").igDataChart({ markerBrushes: [ "#ff0000", "#ffff00", "#00ffff" ] }); // Get var opValue = $(".selector").igDataChart("option", "markerBrushes"); // Set $(".selector").igDataChart("option", "markerBrushes", [ "#ff0000", "#ffff00", "#00ffff" ]);
-
markerOutlines
- タイプ:
- object
- デフォルト:
- null
MarkerOutlines プロパティを取得または設定します。
マーカー アウトライン プロパティは自動的に割り当てられるマーカー アウトラインを選択するパレットを定義します。
提供された値は、css 色文字列の配列である必要があります。最初の要素は、コレクションの補間モードを指定する RGB または HSV の文字列に設定するオプションがあります。コード サンプル
// Initialization $(".selector").igDataChart({ markerOutlines: [ "#ff0000", "#ffff00", "#00ffff" ] }); // Get var markerOutlines = $(".selector").igDataChart("option", "markerOutlines"); // Set $(".selector").igDataChart("option", "markerOutlines", [ "#ff0000", "#ffff00", "#00ffff" ]);
-
outlines
- タイプ:
- object
- デフォルト:
- null
Outlines プロパティを取得または設定します。
自動的に割り当てられる categorySeries の輪郭線の選択元のパレットは、輪郭線プロパティで定義します。
提供された値は、css 色文字列の配列である必要があります。最初の要素は、コレクションの補間モードを指定する RGB または HSV の文字列に設定するオプションがあります。コード サンプル
// Initialization $(".selector").igDataChart({ outlines: [ "#ff0000", "#ffff00", "#00ffff" ] }); // Get var opValue = $(".selector").igDataChart("option", "outlines"); // Set $(".selector").igDataChart("option", "outlines", [ "#ff0000", "#ffff00", "#00ffff" ]);
-
overviewPlusDetailPaneVisibility
- タイプ:
- enumeration
- デフォルト:
- collapsed
OverviewPlusDetailPane の表示/非表示。
メンバー
- visible
- タイプ:string
- 概要ペインを表示します。
- collapsed
- タイプ:string
- 概要ペインを表示しません。
コード サンプル
// Initialization $(".selector").igDataChart({ overviewPlusDetailPaneVisibility: "visible" }); // Get var opValue = $(".selector").igDataChart("option", "overviewPlusDetailPaneVisibility"); // Set $(".selector").igDataChart("option", "overviewPlusDetailPaneVisibility", "visible");
-
panModifier
- タイプ:
- enumeration
- デフォルト:
- shift
現在の Chart の PanModifier プロパティを取得または設定します。
メンバー
- none
- タイプ:string
- 修飾キーが設定されません。
- alt
- タイプ:string
- 修飾キーは alt キーに設定されます。
- control
- タイプ:string
- 修飾キーは control キーに設定されます。
- shift
- タイプ:string
- 修飾キーは shift キーに設定されます。
コード サンプル
// Initialization $(".selector").igDataChart({ panModifier: "control" }); // Get var opValue = $(".selector").igDataChart("option", "panModifier"); // Set $(".selector").igDataChart("option", "panModifier", "control");
-
pentagonMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上の五角形マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
pentagon のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ pentagonMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
pentagramMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上のペンタグラム マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
pentagram のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ pentagramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
pixelScalingRatio
- タイプ:
- number
- デフォルト:
- 1
スケール変換を適用するためにメイン キャンバスの描画コンテキストにより使用されるスケール値を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ pixelScalingRatio: 2 }); //Get var pixelScalingRatio = $(".selector").igDataChart("option", "pixelScalingRatio"); //Set $(".selector").igDataChart("option", "pixelScalingRatio", 2);
-
plotAreaBackground
- タイプ:
- string
- デフォルト:
- null
現在の Chart オブジェクトのプロット領域の背景として使用するブラシを取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ plotAreaBackground: "grey" }); //Get var plotAreaBackground = $(".selector").igDataChart("option", "plotAreaBackground"); //Set var plotAreaBackground = $(".selector").igDataChart("option", "plotAreaBackground", "grey");
-
plotAreaMarginBottom
- タイプ:
- number
- デフォルト:
- NaN
-
plotAreaMarginLeft
- タイプ:
- number
- デフォルト:
- NaN
-
plotAreaMarginRight
- タイプ:
- number
- デフォルト:
- NaN
-
plotAreaMarginTop
- タイプ:
- number
- デフォルト:
- NaN
-
preferHigherResolutionTiles
- タイプ:
- bool
- デフォルト:
- false
タイル ズーム時にシリーズ ビューアーがより高い解像度のタイルに適しているかどうかを設定します。これを true に設定すると、パフォーマンスは低下しますが描画品質が高まります。
コード サンプル
//Initialize $(".selector").igDataChart({ preferHigherResolutionTiles: true }); //Get var preferHigherRes= $(".selector").igDataChart("option", "preferHigherResolutionTiles"); //Set $(".selector").igDataChart("option", "preferHigherResolutionTiles", true);
-
previewRect
- タイプ:
- object
- デフォルト:
- null
プレビュー矩形を設定または取得します。
プレビュー矩形は Rect.Empty に設定することができます。その場合、表示されているプレビューの
strokePath は非表示になります。
提供されたオブジェクトは、left、top、width および height と呼ばれる数値プロパティを持っている必要があります。コード サンプル
// Initialization $(".selector").igDataChart({ previewRect: { left: 0.3, top: 0.3, width: 0.5, height: 0.5 } }); // Get var previewRect = $(".selector").igDataChart("option", "previewRect"); // Set $(".selector").igDataChart("option", "previewRect", { left: 0.3, top: 0.3, width: 0.5, height: 0.5 });
-
pyramidMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上のピラミッド マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
pyramid のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ pyramidMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
regional
継承- タイプ:
- enumeration
- デフォルト:
- defaults
ウィジェットの領域設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ regional: "ja" }); // Get var regional = $(".selector").igDataChart("option", "regional"); // Set $(".selector").igDataChart("option", "regional", "ja");
-
responseDataKey
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource を参照してください。応答がラップされる場合に、データ レコードが保持されるプロパティの名前を指定します。
コード サンプル
// Initialize $(".selector").igDataChart({ responseDataKey: "Records" }); // Get var responseDataKey = $(".selector").igDataChart("option", "responseDataKey"); // Set var responseDataKey = $(".selector").igDataChart("option", "responseDataKey", "Records");
-
rightMargin
- タイプ:
- number
- デフォルト:
- NaN
キャンバスでチャート コンテンツの周囲で使用する右余白を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ rightMargin : 20 }); //Get var margin = $(".selector").igDataChart("option", "rightMargin"); //Set $(".selector").igDataChart("option", "rightMargin", 20);
-
series
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
シリーズ オブジェクトの配列。
コード サンプル
// Initialization var data = [{ "Label": "1/1/2012", "Item1": 980, "Item2": 1000 }, { "Label": "1/2/2012", "Item1": 1200, "Item2": 1500 }]; $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price Series 1", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0]; // Set $(".selector").igDataChart("series", [{ name: "series2", dataSource: data, title: "Price Series 2", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] );
-
angleAxis
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの有効な角度軸を取得します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "angleAxis", type: "categoryAngle", label: "Label" }, { name: "radiusAxis", type: "numericRadius" }], series: [{ name: "series1", dataSource: data, title: "Budget", type: "radialLine", angleAxis: "angleAxis", valueAxis: "radiusAxis", valueMemberPath: "Budget", }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].angleAxis; // Set var series = $(".selector").igDataChart("option", [{ name: "series1", dataSource: data, title: "Budget", type: "radialLine", angleAxis: "angleAxis", valueAxis: "radiusAxis", valueMemberPath: "Budget", }] );
-
angleMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
angleMemberPath
- タイプ:
- number
- デフォルト:
- null
列の角を丸めるために使用される楕円の x 半径を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "angleAxis", type: "numericAngle" }, { name: "radiusAxis", type: "numericRadius" }], series: [{ name: "series1", title: "Sales", type: "polarScatter", angleAxis: "angleAxis", radiusAxis: "radiusAxis", angleMemberPath: "Index", radiusMemberPath: "Value1" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].angleMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", title: "Sales", type: "polarScatter", angleAxis: "angleAxis", radiusAxis: "radiusAxis", angleMemberPath: "Index", radiusMemberPath: "Value1" }] );
-
areaFillOpacity
- タイプ:
- number
- デフォルト:
- 1.0
シリーズの領域塗りつぶし図形に適用する不透明度修飾子を取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "area", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", areaFillOpacity: 0.8 }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].areaFillOpacity; // Set $(".selector").igDataChart("series", [{ name: "series1", areaFillOpacity: 0.8 }] );
-
axisAnnoationFormatLabel
- タイプ:
- object
- デフォルト:
- null
-
axisAnnotationBackground
- タイプ:
- string
- デフォルト:
- null
-
axisAnnotationInterpolatedValuePrecision
- タイプ:
- number
- デフォルト:
- -1
-
axisAnnotationOutline
- タイプ:
- string
- デフォルト:
- null
-
axisAnnotationPaddingBottom
- タイプ:
- number
- デフォルト:
- NaN
-
axisAnnotationPaddingLeft
- タイプ:
- number
- デフォルト:
- NaN
-
axisAnnotationPaddingRight
- タイプ:
- number
- デフォルト:
- NaN
-
axisAnnotationPaddingTop
- タイプ:
- number
- デフォルト:
- NaN
-
axisAnnotationStrokeThickness
- タイプ:
- number
- デフォルト:
- NaN
-
axisAnnotationTextColor
- タイプ:
- string
- デフォルト:
- null
-
badgeShape
- タイプ:
- enumeration
- デフォルト:
- automatic
メンバー
- automatic
- タイプ:string
- circle
- タイプ:string
- line
- タイプ:string
- square
- タイプ:string
- marker
- タイプ:string
- bar
- タイプ:string
- column
- タイプ:string
- hidden
- タイプ:string
-
bandHighlightWidth
- タイプ:
- number
- デフォルト:
- 10.0
グリッド 系列(ライン、スプラインなど) の項目をバンド図形で強調表示する場合に、強調表示領域に使用する幅を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", bandHighlightWidth: 5.0 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].bandHighlightWidth; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", bandHighlightWidth: 5.0 }] );
-
brush
- タイプ:
- string
- デフォルト:
- null
シリーズに使用するブラシを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", brush: "blue" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].brush; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", brush: "blue" }] );
-
calloutBackground
- タイプ:
- string
- デフォルト:
- null
-
calloutCollisionMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- simulatedAnnealing
- タイプ:string
- greedy
- タイプ:string
- greedyCenterOfMass
- タイプ:string
-
calloutContentUpdating
- タイプ:
- object
- デフォルト:
- null
-
calloutLabelUpdating
- タイプ:
- object
- デフォルト:
- null
-
calloutLeaderBrush
- タイプ:
- string
- デフォルト:
- null
-
calloutOutline
- タイプ:
- string
- デフォルト:
- null
-
calloutPaddingBottom
- タイプ:
- number
- デフォルト:
- NaN
-
calloutPaddingLeft
- タイプ:
- number
- デフォルト:
- NaN
-
calloutPaddingRight
- タイプ:
- number
- デフォルト:
- NaN
-
calloutPaddingTop
- タイプ:
- number
- デフォルト:
- NaN
-
calloutPositionPadding
- タイプ:
- number
- デフォルト:
- NaN
-
calloutSeriesSelecting
- タイプ:
- object
- デフォルト:
- null
-
calloutStrokeThickness
- タイプ:
- number
- デフォルト:
- NaN
-
calloutTextColor
- タイプ:
- string
- デフォルト:
- null
-
clipSeriesToBounds
- タイプ:
- bool
- デフォルト:
- null
シリーズを境界線でクリップするかどうかを設定または取得します。
これを true に設定するとパフォーマンスに影響を及ぼします。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", clipSeriesToBounds: true }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].clipSeriesToBounds; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", clipSeriesToBounds: true }] );
-
closeMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
closeMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの終値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].closeMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] );
-
coercionMethods
- タイプ:
- object
- デフォルト:
- null
データ ソースからデータを読み込むときに使用する強制メソッドを取得または設定します。
使用する場合、メンバー パスを設定する前に指定します。後に設定する場合、
チャートにデータを再インポートしません。コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryDateTimeX", dataSource: data, label: "Date" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "line", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value!roundTo50", coercionMethods: { roundTo100: function (val) { var newVal = Math.round(val / 100) <dependency>100; return newVal; } } }] });
-
colorMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
consolidatedColumnVerticalPosition
- タイプ:
- enumeration
- デフォルト:
- median
単一の視覚要素に統合されたデータ項目の配置ロジックを決定するための値を取得または設定します。
メンバー
- maximum
- タイプ:string
- 統合された項目は最大値を使用して配置されます。
- minimum
- タイプ:string
- 統合された項目は最小値を使用して配置されます。
- median
- タイプ:string
- 連結された項目が範囲の中点に配置されます。
- relativeMinimum
- タイプ:string
- 統合された項目は相対する軸の参照値に一番近い値を使用して配置されます。
- relativeMaximum
- タイプ:string
- 統合された項目は相対する軸の参照値に一番遠い値を使用して配置されます。
コード サンプル
$(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "column", xAxis: "xAxis", yAxis: "yAxis", consolidatedColumnVerticalPosition: "maximum" }] });
-
contentMemberPath
- タイプ:
- string
- デフォルト:
- null
-
cursorPosition
- タイプ:
- object
- デフォルト:
- null
このレイヤーで注釈を表示するために、現在のマウス カーソルの位置の代わりに使用するカーソル位置を設定します。
0 ~ 1 のワールド座標で x および y プロパティを含むオブジェクトです。コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", cursorPosition: {x:0.5 , y:0.5} } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].cursorPosition; //Set $(".selector").igDataChart("option", "series", [{ name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", cursorPosition: {x:0.5 , y:0.5} }] );
-
dataSource
- タイプ:
- object
- デフォルト:
- null
$.ig.DataSource が受け入れる有効なデータ ソースまたは $.ig.DataSource 自体のインスタンスが可能です。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].dataSource; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] );
-
dataSourceType
- タイプ:
- string
- デフォルト:
- null
データ ソースのタイプ ("json" など) を明示的に設定します。$.ig.DataSource とそのタイプ プロパティのドキュメントを参照してください。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, dataSourceType: "json", title: "Price", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].dataSourceType; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, dataSourceType: "json", title: "Price", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] );
-
dataSourceUrl
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource からデータを要求するには、$.ig.DataSource により承諾されたリモート URL を指定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSourceUrl: "http://www.example.com/price-data", dataSourceType: "json", title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].dataSource; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSourceUrl: "http://www.example.com/price-data", dataSourceType: "json", title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] );
-
discreteLegendItemTemplate
- タイプ:
- enumeration
- デフォルト:
- null
DiscreteLegendItemTemplate プロパティを取得または設定します。
凡例項目コントロール コンテンツは、DiscreteLegendItemTemplate に従って、シリーズ オブジェクト自身によって
オンデマンドで作成されます。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", discreteLegendItemTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
displayType
- タイプ:
- enumeration
- デフォルト:
- candlestick
現在の FinancialIndicator オブジェクトの表示を取得または設定します。
メンバー
- candlestick
- タイプ:string
- 価格を日本のろうそくとして表示します。
- OHLC
- タイプ:string
- 価格を OHLC バーとして表示します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", type: "averageTrueRangeIndicator", displayType: "area" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].displayType; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", type: "averageTrueRangeIndicator", displayType: "area" }] );
-
excludedColumns
- タイプ:
- object
- デフォルト:
- null
-
excludedSeries
- タイプ:
- object
- デフォルト:
- null
-
expectFunctions
- タイプ:
- bool
- デフォルト:
- false
値を取得するために、シリーズのデータ ソース メンバーが関数として呼び出す必要があるかどうかを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "area", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", expectFunctions: false }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].expectFunctions; // Set $(".selector").igDataChart("series", [{ name: "series1", expectFunctions: false }] );
-
fillMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
fillMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの塗りつぶしマッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "bubble", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", radiusMemberPath: "Value2", fillMemberPath: "Value3", labelMemberPath: "Value2" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].fillMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "bubble", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", radiusMemberPath: "Value2", fillMemberPath: "Value3", labelMemberPath: "Value2" }] );
-
fillScale
- タイプ:
- object
- デフォルト:
- null
マーカー ブラシのブラシ スケールを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "bubble", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", radiusMemberPath: "Value2", fillMemberPath: "Value3", labelMemberPath: "Value2", fillScale: { type: "value", brushes: ["red", "blue"], minimumValue: 0, maximumValue: 20 } }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].fillScale; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "bubble", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", radiusMemberPath: "Value2", fillMemberPath: "Value3", labelMemberPath: "Value2", fillScale: { type: "value", brushes: ["red", "blue"], minimumValue: 0, maximumValue: 20 } }] );
-
finalValueSelectionMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- finalVisible
- タイプ:string
- finalVisibleInterpolated
- タイプ:string
- final
- タイプ:string
-
groupingMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- grouped
- タイプ:string
-
headerFormatCulture
- タイプ:
- string
- デフォルト:
- null
-
headerFormatDate
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- shortStyle
- タイプ:string
- longStyle
- タイプ:string
- mediumStyle
- タイプ:string
- fullStyle
- タイプ:string
- none
- タイプ:string
-
headerFormatSpecifiers
- タイプ:
- object
- デフォルト:
- null
-
headerFormatString
- タイプ:
- string
- デフォルト:
- null
-
headerFormatTime
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- shortStyle
- タイプ:string
- longStyle
- タイプ:string
- mediumStyle
- タイプ:string
- fullStyle
- タイプ:string
- none
- タイプ:string
-
headerRowVisible
- タイプ:
- bool
- デフォルト:
- false
-
headerText
- タイプ:
- string
- デフォルト:
- null
-
headerTextColor
- タイプ:
- string
- デフォルト:
- null
-
headerTextStyle
- タイプ:
- object
- デフォルト:
- null
-
heatMaximum
- タイプ:
- number
- デフォルト:
- 50
最高熱色にマップする値を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "highDensityScatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", heatMaximum: 100 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].heatMaximum; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "highDensityScatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" heatMaximum: 100 }]);
-
heatMaximumColor
- タイプ:
- object
- デフォルト:
- red
密度スケールに使用する最大ヒート色を取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "highDensityScatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", heatMaximumColor: "red" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].heatMaximumColor; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "highDensityScatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", heatMaximumColor: "red" }]);
-
heatMinimum
- タイプ:
- number
- デフォルト:
- 0
最低熱色にマップする密度値を取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "highDensityScatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", heatMinimum: 5 }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].heatMinimum; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "highDensityScatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", heatMinimum: 5 }]);
-
heatMinimumColor
- タイプ:
- object
- デフォルト:
- black
密度スケールに使用する最小ヒート色を取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "highDensityScatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", heatMinimumColor: "black" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].heatMinimumColor; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "highDensityScatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", heatMinimumColor: "black" }]);
-
highlightType
- タイプ:
- enumeration
- デフォルト:
- auto
項目の強調表示に使用する強調表示の図形タイプを取得または設定します。
メンバー
- auto
- タイプ:string
- この系列に自動強調表示タイプを使用します。
- marker
- タイプ:string
- この系列にマーカー強調表示タイプを使用します。
- shape
- タイプ:string
- この系列に図形強調表示タイプを使用します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ width: "100%", height: "400px", legend: { element: "lineLegend" }, title: "Population per Country", subtitle: "A comparision of population in 1995 and 2005", dataSource: data, axes: [ { name: "NameAxis", type: "categoryX", label: "CountryName" }, { name: "PopulationAxis", type: "numericY" } ], series: [ { name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isHighlightingEnabled: true, highlightType: "marker" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].highlightType; //Set $(".selector").igDataChart("option", "series", [{ name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isHighlightingEnabled: true, highlightType: "marker" }] );
-
highMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
highMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].highMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] );
-
hitTestMode
- タイプ:
- enumeration
- デフォルト:
- auto
シリーズが使用するヒット テストのタイプを取得または設定します。
メンバー
- auto
- タイプ:string
- シリーズの適切なヒット テスト モードを自動的に決定します。
- computational
- タイプ:string
- シリーズがヒットされたかどうかを決定するために計算に基づく方法を使用します。これはゆるやかな境界ボックスを使用し、ヒットを検索する時間計算量の範囲は O(1) から O(log n) までです。色エンコードと比較してフレームの描画時間を減少させます。
- colorEncoded
- タイプ:string
- 色エンコードされた画面に表示されていないバッファーを使用してヒット テストを実行します。色バッファーでアンチエイリアシングを無効にできないため、間違ったシリーズがヒットする誤検知の可能性はとても低く、ヒット シリーズの決定時間はいつも O(1) 時間です。ただし、フレームの描画時間が長くなります。ヒット テストでパフォーマンスの低下がある場合、この方法を使用できます。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "area", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", hitTestMode: auto }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].hitTestMode; // Set $(".selector").igDataChart("series", [{ name: "series1", hitTestMode: false }] );
-
horizontalLineStroke
- タイプ:
- string
- デフォルト:
- null
-
horizontalLineVisibility
- タイプ:
- enumeration
- デフォルト:
- visible
レイヤーの水平十字ポインター部分が表示されるかどうかを取得または設定します。
メンバー
- visible
- タイプ:string
- レイヤーの水平十字ポインター部分が表示されます。
- collapsed
- タイプ:string
- レイヤーの水平十字ポインター部分が非表示になります。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "column", name: "2005Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005" }, { type: "line", name: "1995Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop1995" }, { type: "crosshairLayer", name: "crosshairLayer", title: "crosshair", useInterpolation: false, transitionDuration: 500, targetSeries: "2005Population", horizontalLineVisibility: "visible" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[2].horizontalLineVisibilty; //Set $(".selector").igDataChart("option", "series", [{ name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", horizontalLineVisiblity: "visible" }] );
-
ignoreFirst
- タイプ:
- number
- デフォルト:
- 0
インジケータの開始を非表示にするための値の数を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", ignoreFirst: 2 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].ignoreFirst; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", ignoreFirst: 2 }] );
-
includedColumns
- タイプ:
- object
- デフォルト:
- null
-
includedSeries
- タイプ:
- object
- デフォルト:
- null
-
isAxisAnnotationEnabled
- タイプ:
- bool
- デフォルト:
- false
-
isCalloutOffsettingEnabled
- タイプ:
- bool
- デフォルト:
- true
-
isCustomCategoryMarkerStyleAllowed
- タイプ:
- bool
- デフォルト:
- false
カスタム カテゴリ マーカー スタイルが許可されるかどうかを取得または設定します。True に設定すると、提供されている場合に assigningCategoryMarkerStyle イベントを発生します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [{ type: "categoryHighlightLayer", name: "categoryHighlightLayer", title: "categoryHighlight", useInterpolation: false, transitionDuration: 500, targetSeries: "2005Population", isCustomCategoryMarkerStyleAllowed: "true" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].isCustomCategoryMarkerStyleAllowed; //Set $(".selector").igDataChart("option", "series", [{ type: "categoryHighlightLayer", name: "categoryHighlightLayer", title: "categoryHighlight", isCustomCategoryMarkerStyleAllowed: "true" }] );
-
isCustomCategoryStyleAllowed
- タイプ:
- bool
- デフォルト:
- false
カスタム カテゴリ スタイルが許可されるかどうかを取得または設定します。True に設定すると、提供されている場合に assigningCategoryStyle イベントを発生します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "categoryHighlightLayer", name: "categoryHighlightLayer", title: "categoryHighlight", useInterpolation: false, transitionDuration: 500, targetSeries: "2005Population", isCustomCategoryStyleAllowed: "true" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].isCustomCategoryStyleAllowed; //Set $(".selector").igDataChart("option", "series", [{ name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", isCustomCategoryStyleAllowed: "true" }] );
-
isDefaultCrosshairDisabled
- タイプ:
- bool
- デフォルト:
- true
このレイヤーがチャートのデフォルト十時線動作を無効にするかどうかを設定します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "categoryHighlightLayer", name: "categoryHighlightLayer", title: "categoryHighlight", isDefaultCrosshairDisabled: "true" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].isDefaultCrosshairDisabled; //Set $(".selector").igDataChart("option", "series", [{ type: "categoryHighlightLayer", name: "categoryHighlightLayer", title: "categoryHighlight", isDefaultCrosshairDisabled: "true" }] );
-
isDropShadowEnabled
- タイプ:
- bool
- デフォルト:
- false
ドロップ シャドウがこの系列で有効かどうかを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", isDropShadowEnabled: true }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].isDropShadowEnabled; // Set $(".selector").igDataChart("series", [{ name: "series1", isDropShadowEnabled: true }] );
-
isHighlightingEnabled
- タイプ:
- bool
- デフォルト:
- false
サポートされる場合、この系列の強調表示を有効にするかどうかを取得または設定します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [ { name: "NameAxis", type: "categoryX", label: "CountryName" }, { name: "PopulationAxis", type: "numericY", minimumValue: 0 } ], series: [ { name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isHighlightingEnabled: true }, { name: "1995Population", type: "line", title: "1995", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop1995", isHighlightingEnabled: true } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].isHighlightingEnabled; //Set $(".selector").igDataChart("option", "series", [{ name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isHighlightingEnabled: true }] );
-
isTransitionInEnabled
- タイプ:
- bool
- デフォルト:
- false
データ ソースが割り当てられたときに、系列がトランジションのアニメーションを再生するかどうかを取得または設定します。注: トランジションは積層型シリーズでサポートされていません。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [ { name: "NameAxis", type: "categoryX", label: "CountryName" }, { name: "PopulationAxis", type: "numericY", } ], series: [ { name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true }, { name: "1995Population", type: "line", title: "1995", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop1995", isTransitionInEnabled: true } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].isTransitionInEnabled; //Set $(".selector").igDataChart("option", "series", [{ name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true }] );
-
keyMemberPath
- タイプ:
- string
- デフォルト:
- null
-
labelDisplayMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- visible
- タイプ:string
- hidden
- タイプ:string
-
labelMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトのラベル マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "bubble", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", radiusMemberPath: "Value2", fillMemberPath: "Value3", labelMemberPath: "Value2" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].labelMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "bubble", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", radiusMemberPath: "Value2", fillMemberPath: "Value3", labelMemberPath: "Value2" }] );
-
labelTextColor
- タイプ:
- string
- デフォルト:
- null
-
labelTextMarginBottom
- タイプ:
- number
- デフォルト:
- 0
-
labelTextMarginLeft
- タイプ:
- number
- デフォルト:
- 12
-
labelTextMarginRight
- タイプ:
- number
- デフォルト:
- 0
-
labelTextMarginTop
- タイプ:
- number
- デフォルト:
- 0
-
labelTextStyle
- タイプ:
- object
- デフォルト:
- null
-
legend
- タイプ:
- object
- デフォルト:
- {}
$.ig.ChartLegend が受け入れる有効なオプションまたは $.ig.ChartLegend 自体のインスタンスが可能です。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" } }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].legend; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" } }] );
-
element
- タイプ:
- string
- デフォルト:
- null
凡例に変更する要素の名前。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" } }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].legend.element; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" } }] );
-
height
- タイプ:
- enumeration
- デフォルト:
- null
凡例の高さ。null の場合、他の高さが定義されていないときにデータにフィットするように垂直方向に伸びます。
メンバー
- null
- タイプ:object
- string
- ウィジェットの高さはピクセル (px) およびパーセント (%) で設定できます (%)。
- number
- 幅の高さは数値 (25) として設定できます。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend", height: "90px" } }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].legend.height; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend", height: "90px" } }] );
-
type
- タイプ:
- enumeration
- デフォルト:
- legend
凡例のタイプ。
メンバー
- item
- タイプ:string
- 凡例を項目の凡例として指定します。igPieChart コントロール内の各パイに対して凡例の項目を表示します。
- legend
- タイプ:string
- 凡例を凡例として指定します。これは、igDataChart コントロールのすべてのタイプのシリーズによってサポートされます。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" } }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].legend.type; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" } }] );
-
width
- タイプ:
- enumeration
- デフォルト:
- null
凡例の幅。
メンバー
- null
- タイプ:object
- 他の幅が定義されていない場合、データがフィットするよう伸縮します。
- string
- ウィジェットの幅はピクセル (px) およびパーセント (%) で設定できます (%)。
- number
- ウィジェット幅は数値として設定できます。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend", width: "90px" } }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].legend.width; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend", width: "90px" } }] );
-
legendItemBadgeMode
- タイプ:
- object
- デフォルト:
- simplified
-
legendItemBadgeShape
- タイプ:
- enumeration
- デフォルト:
- auto
-
legendItemBadgeTemplate
- タイプ:
- object
- デフォルト:
- null
LegendItemBadgeTemplate プロパティを取得または設定します。
凡例項目バッジは、LegendItemBadgeTemplate に従って、シリーズ オブジェクト自身によってオンデマンドで作成されます。
オンデマンドで作成されます。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。
これらはユーザー指定のカスタム描画を処理するために呼び出される関数です。
measure は以下のようなオブジェクトを渡されます:
{
context: [特定のテンプレートのシナリオに基づいて DOM 要素または CanvasContext2D のいずれか],
width: [値が存在する場合、使用可能な幅を指定します。ユーザーはコンテンツのために希望の幅を設定できます],
height: [値が存在する場合、使用可能な高さを指定します。ユーザーはコンテンツのために希望の高さを設定できます],
isConstant: [希望の幅と高さが常にこのテンプレートに対して同じである場合には、ユーザーは True に設定する必要があります],
data: [存在する場合、このテンプレートのコンテキストでを表します]
}
render は以下のようなオブジェクトを渡されます:
{
context: [特定のテンプレートのシナリオに基づいて DOM 要素または CanvasContext2D のいずれか],
xPosition: [存在する場合、コンテンツを描画する x 位置を指定します],
yPosition: [存在する場合、コンテンツを描画する y 位置を指定します],
availableWidth: [存在する場合、コンテンツを描画する使用可能な幅を指定します],
availableHeight: [存在する場合、コンテンツを描画する使用可能な高さを指定します],
data: [存在する場合、このコンテキストのためにコンテキストにあるデータを指定します],
isHitTestRender: [true の場合、これがヒットテストのために特別な描画パスであることを指定します。この場合データからのブラシを使用する必要があります]
}。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" }, legendItemBadgeTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
legendItemTemplate
- タイプ:
- object
- デフォルト:
- null
LegendItemTemplate プロパティを取得または設定します。
凡例項目コントロール コンテンツは、LegendItemTemplate に従って、シリーズ オブジェクト自身によってオンデマンドで作成されます。
オンデマンドで作成されます。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" }, legendItemTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
legendItemVisibility
- タイプ:
- enumeration
- デフォルト:
- visible
現在のシリーズ オブジェクトの凡例項目の表示状態を取得または設定します。
メンバー
- visible
- タイプ:string
- 凡例項目を表示します。
- collapsed
- タイプ:string
- 凡例項目を表示しません。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" }, legendItemVisibility: "collapsed" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].legendItemVisibility; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", legend: { element: "legend", type: "legend" }, legendItemVisibility: "collapsed" }] );
-
longPeriod
- タイプ:
- number
- デフォルト:
- 0
現在の AbsoluteVolumeOscillatorIndicator オブジェクトの短い移動平均期間を取得または設定します。
長い AVO 期間の一般的な値かつ初期値は 30 です。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "absoluteVolumeOscillatorIndicator", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", longPeriod: 15 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].longPeriod; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "absoluteVolumeOscillatorIndicator", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", longPeriod: 15 }] );
-
lowMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
lowMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].lowMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] );
-
markerBrush
- タイプ:
- string
- デフォルト:
- null
現在の系列オブジェクトのマーカーの内部をペイントする方法を指定するブラシを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerBrush: "blue", markerType: "automatic" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].markerBrush; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerBrush: "blue", markerType: "automatic" }] );
-
markerCollisionAvoidance
- タイプ:
- enumeration
- デフォルト:
- none
MarkerCollisionAvoidance を取得または設定します。
メンバー
- none
- タイプ:string
- 競合回避がありません。
- omit
- タイプ:string
- 競合するマーカーを表示しません。
- fade
- タイプ:string
- 競合するマーカーの不透明度をフォードします。
- omitAndShift
- タイプ:string
- 競合するマーカーを移動するか、表示しません。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerCollisionAvoidance: "fade", markerType: "automatic" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].markerCollisionAvoidance; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerCollisionAvoidance: "fade", markerType: "automatic" }] );
-
markerFillMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- normal
- タイプ:string
- matchMarkerOutline
- タイプ:string
-
markerFillOpacity
- タイプ:
- number
- デフォルト:
- NaN
-
markerOutline
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトのマーカーのアウトラインを塗りつぶす方法を指定するブラシを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerOutline: "black", markerType: "automatic" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].markerOutline; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerOutline: "black", markerType: "automatic" }] );
-
markerOutlineMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- normal
- タイプ:string
- matchMarkerBrush
- タイプ:string
-
markerTemplate
- タイプ:
- object
- デフォルト:
- null
現在のシリーズ オブジェクトの MarkerTemplate を取得または設定します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic", markerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
markerThickness
- タイプ:
- number
- デフォルト:
- 2.0
-
markerType
- タイプ:
- enumeration
- デフォルト:
- none
現在のシリーズ オブジェクトにマーカー タイプを取得または設定します。MarkerStyle プロパティが設定されると、MarkerStyle プロパティの設定は無視されます。
メンバー
- unset
- タイプ:string
- マーカーは設定されていません。
- none
- タイプ:string
- markerItems なし。
- automatic
- タイプ:string
- 自動マーカー形状。
- circle
- タイプ:string
- 円形マーカー形状。
- triangle
- タイプ:string
- 下向き三角形マーカー形状。
- pyramid
- タイプ:string
- 上向き三角形マーカー形状。
- square
- タイプ:string
- 四角形マーカー形状。
- diamond
- タイプ:string
- 菱形マーカー形状。
- pentagon
- タイプ:string
- 五角形マーカー形状。
- hexagon
- タイプ:string
- 六角形マーカー形状。
- tetragram
- タイプ:string
- 四角の星のマーカー形状。
- pentagram
- タイプ:string
- 五角の星のマーカー形状。
- hexagram
- タイプ:string
- 六角の星のマーカー形状。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].markerType; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic" }] );
-
maximumMarkers
- タイプ:
- number
- デフォルト:
- 400
現在の系列によって表示される markerItems の最大数を取得または設定します。
指定した数を超える markerItems が表示されている場合、シリーズは代表的なセットを
自動的に選択します。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic", maximumMarkers: 100 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].maximumMarkers; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic", maximumMarkers: 100 }] );
-
mouseOverEnabled
- タイプ:
- bool
- デフォルト:
- false
チャートがマウス移動イベントに反応するかどうかを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic", mouseOverEnabled: true }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].mouseOverEnabled; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic", mouseOverEnabled: true }] );
-
name
- タイプ:
- string
- デフォルト:
- null
シリーズの一意の識別子。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].name; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] );
-
negativeBrush
- タイプ:
- string
- デフォルト:
- null
系列の負の領域を描画するブラシを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", negativeBrush: "red" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].negativeBrush; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", negativeBrush: "red" }] );
-
openMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
openMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの始値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].openMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] );
-
outline
- タイプ:
- string
- デフォルト:
- null
シリーズのアウトラインを描画するブラシを取得または設定します。
LineSeries など、一部の Series タイプでは、輪郭が表示されません。そのため、このプロパティは一部のチャートに影響を与えません。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", outline: "black" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].outline; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", outline: "black" }] );
-
outlineMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- visible
- タイプ:string
- collapsed
- タイプ:string
-
period
- タイプ:
- number
- デフォルト:
- 0
現在の AverageDirectionalIndexIndicator オブジェクトの移動平均期間を取得または設定します。
AverageDirectionalIndexIndicator 期間の一般的な値かつ初期値は 14 です。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "averageDirectionalIndexIndicator", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", period: 7 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].period; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "averageDirectionalIndexIndicator", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", period: 7 }] );
-
progressiveLoad
- タイプ:
- bool
- デフォルト:
- true
データをチャートに徐々に読み込むかどうかを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic", progressiveLoad: false }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].progressiveLoad; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", markerType: "automatic", progressiveLoad: false }] );
-
radius
- タイプ:
- number
- デフォルト:
- 2
系列に使用する角の半径を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "angleAxis", type: "numericAngle" }, { name: "radius", type: "numericRadius" }], series: [{ name: "series1", title: "Sales", type: "polarScatter", angleAxis: "angleAxis", radius: "radius", angleMemberPath: "Index", radiusMemberPath: "Value1" }], }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].radius; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", title: "Sales", type: "polarScatter", angleAxis: "angleAxis", radius: "radius", angleMemberPath: "Index", radiusMemberPath: "Value1" }] );
-
radiusAxis
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの有効な半径軸を取得します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "angleAxis", type: "numericAngle" }, { name: "radiusAxis", type: "numericRadius" }], series: [{ name: "series1", title: "Sales", type: "polarScatter", angleAxis: "angleAxis", radiusAxis: "radiusAxis", angleMemberPath: "Index", radiusMemberPath: "Value1" }], }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].radiusAxis; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", title: "Sales", type: "polarScatter", angleAxis: "angleAxis", radiusAxis: "radiusAxis", angleMemberPath: "Index", radiusMemberPath: "Value1" }] );
-
radiusMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
radiusMemberPath
- タイプ:
- string
- デフォルト:
- null
現在の系列オブジェクトの半径マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "angleAxis", type: "numericAngle" }, { name: "radiusAxis", type: "numericRadius" }], series: [{ name: "series1", title: "Sales", type: "polarScatter", angleAxis: "angleAxis", radiusAxis: "radiusAxis", angleMemberPath: "Index", radiusMemberPath: "Value1" }], }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].radiusMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", title: "Sales", type: "polarScatter", angleAxis: "angleAxis", radiusAxis: "radiusAxis", angleMemberPath: "Index", radiusMemberPath: "Value1" }] );
-
radiusScale
- タイプ:
- object
- デフォルト:
- null
バブルの半径サイズ スケールを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "bubble", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", radiusMemberPath: "Value2", fillMemberPath: "Value3", labelMemberPath: "Value2", radiusScale: { minimumValue: 0, maximumValue: 20, isLogarithmic: "true", logarithmicBase: 2 } }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].radiusScale; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "bubble", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", radiusMemberPath: "Value2", fillMemberPath: "Value3", labelMemberPath: "Value2", radiusScale: { type: "value", brushes: ["red", "blue"], minimumValue: 0, maximumValue: 20 } }] );
-
radiusX
- タイプ:
- number
- デフォルト:
- 0.0
列の角を丸めるために使用される楕円の x 半径を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "column", radiusX: 5.5 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].radiusX; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "column", radiusX: 5.5 }] );
-
radiusY
- タイプ:
- number
- デフォルト:
- 0.0
列の角を丸めるために使用する楕円の y 半径を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "column", radiusY: 5.5 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].radiusY; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "column", radiusY: 5.5 }] );
-
remove
- タイプ:
- bool
- デフォルト:
- false
名前で、チャートから既存のシリーズを削除するために True に設定します。
コード サンプル
//Set $(".selector").igDataChart("option", "series", [{ name: "series1", remove: true }] );
-
resolution
- タイプ:
- number
- デフォルト:
- NaN
現在のシリーズ オブジェクトのレンダリング解像度を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", resolution: 3 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].resolution; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", resolution: 3 }] );
-
responseDataKey
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource を参照してください。応答がラップされる場合に、データ レコードが保持されるプロパティの名前を指定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, responseDataKey: "Records", title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].responseDataKey; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, responseDataKey: "Records", title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close" }] );
-
reverseLegendOrder
- タイプ:
- bool
- デフォルト:
- false
フラグメント シリーズの順序を凡例で逆にするかどうかを設定します。注: 凡例の順序の反転は集合形式シリーズのみでサポートされます。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "stackedColumn", xAxis: "xAxis", yAxis: "yAxis", reverseLegendOrder: true, series: [{ name: "fragment1", title: "fragment1", }, { name: "fragment2", title: "fragment2", }] }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].reverseLegendOrder; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", reverseLegendOrder: true }] );
-
series
- タイプ:
- array
- デフォルト:
- null
- 要素タイプ:
積層型チャートの系列を取得または設定します。系列オブジェクトの配列を含みます。配列の各項目はシリーズを表し、xAxis、yAxis、valueMemberPath などの最上位の系列オブジェクトによってサポートされるオプションがあります。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label", }, { name: "yAxis", type: "numericY", }], series: [{ name: "series1", type: "stackedColumn", xAxis: "xAxis", yAxis: "yAxis", series: [{ name: "China", type: "stackedFragment", valueMemberPath: "China" }, { name: "United States", type: "stackedFragment", valueMemberPath: "UnitedStates" }, { name: "Russia", type: "stackedFragment", valueMemberPath: "Russia" }, { name: "Saudi Arabia", type: "stackedFragment", valueMemberPath: "SaudiArabia" }, { name: "Canada", type: "stackedFragment", valueMemberPath: "Canada" }] }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].series; // Set $(".selector").igDataChart("series", [{ name: "series1", series: [{ name: "United States", type: "stackedFragment", valueMemberPath: "UnitedStates" }] }]);
-
shadowBlur
- タイプ:
- number
- デフォルト:
- 10
影付きで使用するぼかし量を取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", shadowBlur: 7 }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].shadowBlur; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", shadowBlur: 7 }] );
-
shadowColor
- タイプ:
- object
- デフォルト:
- rgba(95,95,95,0.5)
影付きで使用する色を取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", shadowColor: "blue" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].shadowColor; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", shadowColor: "blue" }] );
-
shadowOffsetX
- タイプ:
- number
- デフォルト:
- 5
影付きで使用する x オフセットを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", shadowOffsetX: 10 }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].shadowOffsetX; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", shadowOffsetX: 10 }] );
-
shadowOffsetY
- タイプ:
- number
- デフォルト:
- 5
影付きで使用する y オフセットを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", shadowOffsetY: 10 }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].shadowOffsetY; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", shadowOffsetY: 10 }] );
-
shortPeriod
- タイプ:
- number
- デフォルト:
- 0
現在の AbsoluteVolumeOscillatorIndicator オブジェクトの短い移動平均期間を取得または設定します。
短い AVO 期間の一般的な値かつ初期値は 10 です。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "absoluteVolumeOscillatorIndicator", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", shortPeriod: 7 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].shortPeriod; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "absoluteVolumeOscillatorIndicator", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", shortPeriod: 7 }] );
-
shouldUpdateWhenSeriesDataChanges
- タイプ:
- bool
- デフォルト:
- true
-
showTooltip
- タイプ:
- bool
- デフォルト:
- false
チャートがツールチップを描画すべきかどうか。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", showTooltip: true, tooltipTemplate: "template" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].showTooltip; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", showTooltip: true, tooltipTemplate: "template" }] );
-
skipUnknownValues
- タイプ:
- bool
- デフォルト:
- false
ホバー レイヤーが一番近い値を検索するときに不明な値をスキップするかどうかを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "toolTipLayer1", type: "itemToolTipLayer", skipUnknownValues: true }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].skipUnknownValues; //Set $(".selector").igDataChart("option", "series", [{ name: "toolTipLayer1", type: "itemToolTipLayer", skipUnknownValues: true }] );
-
splineType
- タイプ:
- enumeration
- デフォルト:
- natural
描画されるスプラインのタイプを取得または設定します。
メンバー
- natural
- タイプ:string
- 自然スプライン計算数式を使用することによってスプラインを計算します。
- clamped
- タイプ:string
- 固定スプライン計算数式を使用することによってスプラインを計算します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price Series 1", type: "spline", splineType: "clamped", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].splineType; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price Series 1", type: "spline", splineType: "clamped", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] );
-
summaryLabelText
- タイプ:
- string
- デフォルト:
- null
-
summaryLabelTextColor
- タイプ:
- string
- デフォルト:
- null
-
summaryLabelTextStyle
- タイプ:
- object
- デフォルト:
- null
-
summaryTitleText
- タイプ:
- string
- デフォルト:
- null
-
summaryTitleTextColor
- タイプ:
- string
- デフォルト:
- null
-
summaryTitleTextStyle
- タイプ:
- object
- デフォルト:
- null
-
summaryType
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- total
- タイプ:string
- average
- タイプ:string
- min
- タイプ:string
- max
- タイプ:string
- none
- タイプ:string
-
summaryUnitsText
- タイプ:
- string
- デフォルト:
- null
-
summaryUnitsTextColor
- タイプ:
- string
- デフォルト:
- null
-
summaryUnitsTextStyle
- タイプ:
- object
- デフォルト:
- null
-
summaryValueTextColor
- タイプ:
- string
- デフォルト:
- null
-
summaryValueTextStyle
- タイプ:
- object
- デフォルト:
- null
-
targetAxis
- タイプ:
- string
- デフォルト:
- null
レイヤーのターゲット軸の名前を取得または設定します。ターゲット軸を設定すると、レイヤーをその軸のみに対象するためにスコープを設定します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "column", name: "2005Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005" }, { type: "line", name: "1995Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop1995" }, { type: "categoryHighlightLayer", name: "catHighlightLayer", title: "categoryHighlight", useInterpolation: false, transitionDuration: 500, bandHighlightWidth: 50, targetAxis: "PopulationAxis" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[2].targetAxis; //Set $(".selector").igDataChart("option", "series", [{ type: "categoryHighlightLayer", name: "catHighlightLayer", title: "categoryHighlight", targetAxis: "PopulationAxis" }] );
-
targetCursorPositionX
- タイプ:
- number
- デフォルト:
- NaN
-
targetCursorPositionY
- タイプ:
- number
- デフォルト:
- NaN
-
targetSeries
- タイプ:
- string
- デフォルト:
- null
レイヤーのターゲット系列の名前を取得または設定します。ターゲット系列を設定すると、レイヤーをその系列のみに対象するためにスコープを設定します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "column", name: "2005Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005" }, { type: "line", name: "1995Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop1995" }, { type: "crosshairLayer", name: "crosshairLayer", title: "crosshair", useInterpolation: false, transitionDuration: 500, targetSeries: "2005Population" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[2].targetSeries; //Set $(".selector").igDataChart("option", "series", [{ name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", targetSeries: "2005Population" }] );
-
thickness
- タイプ:
- number
- デフォルト:
- 0
現在のシリーズ オブジェクトの線の太さの幅を取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price Series 1", type: "line", thickness: 5, xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].thickness; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price Series 1", type: "line", thickness: 5, xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] );
-
title
- タイプ:
- string
- デフォルト:
- null
Title プロパティを取得または設定します。
凡例項目コントロールは、Title に従って、シリーズ オブジェクト自身によってオンデマンドで作成されます。
オンデマンドで作成されます。コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price Series 1", type: "line", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].title; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price Series 1", type: "line", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1" }] );
-
titleTextColor
- タイプ:
- string
- デフォルト:
- null
-
titleTextMarginBottom
- タイプ:
- number
- デフォルト:
- 0
-
titleTextMarginLeft
- タイプ:
- number
- デフォルト:
- 0
-
titleTextMarginRight
- タイプ:
- number
- デフォルト:
- 4
-
titleTextMarginTop
- タイプ:
- number
- デフォルト:
- 0
-
titleTextStyle
- タイプ:
- object
- デフォルト:
- null
-
tooltipPosition
- タイプ:
- enumeration
- デフォルト:
- auto
ツールチップ コンテナーに適用する配置を取得または設定します。
メンバー
- auto
- タイプ:string
- カテゴリ ツールチップのために自動位置を使用します。
- outsideStart
- タイプ:string
- カテゴリ ツールチップを値軸の外部開始に配置します。
- insideStart
- タイプ:string
- カテゴリ ツールチップを値軸の内部開始に配置します。
- insideEnd
- タイプ:string
- カテゴリ ツールチップを値軸の内部終了に配置します。
- outsideEnd
- タイプ:string
- カテゴリ ツールチップを値軸の外部終了に配置します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "categoryToolTipLayer", name: "catToolTipLayer", title: "categoryToolTip", toolTipPosition: "insideStart" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].toolTipPosition; //Set $(".selector").igDataChart("option", "series", [{ type: "categoryToolTipLayer", name: "catToolTipLayer", title: "categoryToolTip", toolTipPosition: "insideStart" }] );
-
tooltipTemplate
- タイプ:
- string
- デフォルト:
- null
チャート ツールチップが描画するために使用するテンプレートの名前またはテンプレート。
コード サンプル
<!-- There are a number of properties available to the template related to the item or series it is used for. ${element} -- The reference to tooltip DOM element. ${item} -- The reference to current series item object. ${chart} -- The reference to chart object. ${series} -- The reference to current series object. ${actualItemBrush} -- The item brush. ${actualSeriesBrush} -- The series brush. --> <!-- Custom Tooltip Template --> <script id="usEnergyProductionTemplate" type="text/x-jquery-tmpl"> <div> <span style="color: ${actualSeriesBrush}">US </span> <span>${item.Year}</span><br /> Total Energy: <span style="font-weight: bold">${item.UnitedStates}</span> </div> </script> //Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "column", showTooltip: true, tooltipTemplate: "usEnergyProductionTemplate" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].tooltipTemplate; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "column", showTooltip: true, tooltipTemplate: "usEnergyProductionTemplate" }] );
-
transitionDuration
- タイプ:
- number
- デフォルト:
- 0
現在の系列のモーフィングの期間を取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data1, title: "Price Series 1", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1", transitionDuration: 500 }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].transitionDuration; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data1, title: "Price Series 1", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Item1", transitionDuration: 500 }] );
-
transitionEasingFunction
- タイプ:
- object
- デフォルト:
- null
トランジションで使用するためのイージング関数を提供します。イージング関数の名前 (「cubic」のみがサポートされている) であるか、数値を取得して関数が適用した出力を返す関数に設定します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [ { name: "NameAxis", type: "categoryX", label: "CountryName" }, { name: "PopulationAxis", type: "numericY", minimumValue: 0, } ], series: [ { name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true, transitionEasingFunction: "cubic" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].transitionEasingFunction; //Set $(".selector").igDataChart("option", "series", [{ name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true, transitionEasingFunction: "cubic" }] );
-
transitionInDuration
- タイプ:
- number
- デフォルト:
- 500
モーフィングで現在の系列トランジションの期間をミリ秒で取得または設定します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [ { name: "NameAxis", type: "categoryX", label: "CountryName" }, { name: "PopulationAxis", type: "numericY", minimumValue: 0, } ], series: [ { name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true, transitionInDuration: 500 } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].transitionInDuration; //Set $(".selector").igDataChart("option", "series", [{ name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true, transitionInDuration: 500 }] );
-
transitionInMode
- タイプ:
- enumeration
- デフォルト:
- auto
系列の入るトランジションのメソッドを取得または設定します。注: トランジションは積層型シリーズでサポートされていません。
メンバー
- auto
- タイプ:string
- 系列が自動的に選択された方法でトランジションします。
- fromZero
- タイプ:string
- 系列が数値軸の参照値からトランジションします。
- sweepFromLeft
- タイプ:string
- 系列が左側からスイープします。
- sweepFromRight
- タイプ:string
- 系列が右側からスイープします。
- sweepFromTop
- タイプ:string
- 系列が上側からスイープします。
- sweepFromBottom
- タイプ:string
- 系列が下側からスイープします。
- sweepFromCenter
- タイプ:string
- 系列が中央からスイープします。
- accordionFromLeft
- タイプ:string
- 系列が左側からアコーディオンします。
- accordionFromRight
- タイプ:string
- 系列が右側からアコーディオンします。
- accordionFromTop
- タイプ:string
- 系列が上側からアコーディオンします。
- accordionFromBottom
- タイプ:string
- 系列が下側からアコーディオンします。
- expand
- タイプ:string
- 系列が値中点から展開します。
- sweepFromCategoryAxisMinimum
- タイプ:string
- 系列がカテゴリ軸の最小値からスイープします。
- sweepFromCategoryAxisMaximum
- タイプ:string
- 系列がカテゴリ軸の最大値からスイープします。
- sweepFromValueAxisMinimum
- タイプ:string
- 系列が数値軸の最小値からスイープします。
- sweepFromValueAxisMaximum
- タイプ:string
- 系列が数値軸の最大値からスイープします。
- accordionFromCategoryAxisMinimum
- タイプ:string
- 系列がカテゴリ軸の最小値からアコーディオンします。
- accordionFromCategoryAxisMaximum
- タイプ:string
- 系列がカテゴリ軸の最大値からアコーディオンします。
- accordionFromValueAxisMinimum
- タイプ:string
- 系列が数値軸の最小値からアコーディオンします。
- accordionFromValueAxisMaximum
- タイプ:string
- 系列が数値軸の最大値からアコーディオンします。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [ { name: "NameAxis", type: "categoryX", label: "CountryName" }, { name: "PopulationAxis", type: "numericY", minimumValue: 0, } ], series: [ { name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true, transitionInMode: "sweepFromRight" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].isTransitionInMode; //Set $(".selector").igDataChart("option", "series", [{ name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true, transitionInMode: "sweepFromRight" }] );
-
transitionInSpeedType
- タイプ:
- enumeration
- デフォルト:
- auto
系列のデータ ポイントの入るトランジションのスピードを取得または設定します。
メンバー
- auto
- タイプ:string
- スピード タイプが自動的に選択されます。
- normal
- タイプ:string
- すべてのスピードは標準です。データ ポイントが同じ時間で入ります。
- valueScaled
- タイプ:string
- データ ポイントの値は開始ポイントからの距離が高い場合、後から入ります。
- indexScaled
- タイプ:string
- データ ポイントのインデックスは軸の基点からの距離が高い場合、後に入ります。
- random
- タイプ:string
- データ ポイントはランダム時間に入ります。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [ { name: "NameAxis", type: "categoryX", label: "CountryName" }, { name: "PopulationAxis", type: "numericY" } ], series: [ { name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true, transitionInSpeedType: "indexScaled" }, { name: "1995Population", type: "line", title: "1995", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop1995", isTransitionInEnabled: true, transitionInSpeedType: "indexScaled" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].transitionInSpeedType; //Set $(".selector").igDataChart("option", "series", [{ name: "2005Population", type: "line", title: "2005", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005", isTransitionInEnabled: true, transitionInSpeedType: "indexScaled" }] );
-
trendLineBrush
- タイプ:
- string
- デフォルト:
- null
トレンドラインを描画するブラシを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "exponentialAverage", trendLineBrush: "grey" }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].trendLineBrush; // Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "exponentialAverage", trendLineBrush: "grey" }] );
-
trendLinePeriod
- タイプ:
- number
- デフォルト:
- 7
現在の散布シリーズ オブジェクトの移動平均期間を取得または設定します。
トレンド折れ線の範囲の標準/初期値は 7 です。コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "simpleAverage", trendLinePeriod: 5 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].trendLinePeriod; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "simpleAverage", trendLinePeriod: 5 }] );
-
trendLineThickness
- タイプ:
- number
- デフォルト:
- 1.5
現在の散布シリーズ オブジェクトのトレンド ラインの太さを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "simpleAverage", trendLineThickness: 3 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].trendLineThickness; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "simpleAverage", trendLineThickness: 3 }] );
-
trendLineType
- タイプ:
- enumeration
- デフォルト:
- none
現在の散布シリーズのトレンド タイプを取得または設定します。
メンバー
- none
- タイプ:string
- トレンド折れ線を表示しません。
- linearFit
- タイプ:string
- リニア フィット。
- quadraticFit
- タイプ:string
- 二次多項式フィット。
- cubicFit
- タイプ:string
- 三次多項式フィット。
- quarticFit
- タイプ:string
- 四次多項式フィット。
- quinticFit
- タイプ:string
- 五次多項式フィット。
- logarithmicFit
- タイプ:string
- 対数フィット。
- exponentialFit
- タイプ:string
- 指数フィット。
- powerLawFit
- タイプ:string
- べき乗フィット。
- simpleAverage
- タイプ:string
- 単純移動平均。
- exponentialAverage
- タイプ:string
- 指数移動平均。
- modifiedAverage
- タイプ:string
- 変更された移動平均。
- cumulativeAverage
- タイプ:string
- 累積移動平均。
- weightedAverage
- タイプ:string
- 加重移動平均。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "simpleAverage" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].trendLineType; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "simpleAverage" }] );
-
trendLineZIndex
- タイプ:
- number
- デフォルト:
- 1001
近似曲線の z インデックスを取得または設定します。1000 より大きい値を指定すると、トレンド折れ線が系列データの前に描画されます。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "simpleAverage", trendLineZIndex: 1200 }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].trendLineZIndex; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", trendLineType: "simpleAverage", trendLineZIndex: 1200 }] );
-
type
- タイプ:
- enumeration
- デフォルト:
- null
シリーズのタイプ。
メンバー
- area
- タイプ:string
- エリア シリーズとしてシリーズを指定します。
- bar
- タイプ:string
- 棒シリーズとしてシリーズを指定します。
- column
- タイプ:string
- 柱状シリーズとしてシリーズを指定します。
- line
- タイプ:string
- 折れ線シリーズとしてシリーズを指定します。
- rangeArea
- タイプ:string
- 範囲エリア シリーズとしてシリーズを指定します。
- rangeColumn
- タイプ:string
- 範囲柱状シリーズとしてシリーズを指定します。
- splineArea
- タイプ:string
- スプライン エリア シリーズとしてシリーズを指定します。
- spline
- タイプ:string
- スプライン シリーズとしてシリーズを指定します。
- stepArea
- タイプ:string
- ステップ エリア シリーズとしてシリーズを指定します。
- stepLine
- タイプ:string
- ステップ折れ線シリーズとしてシリーズを指定します。
- waterfall
- タイプ:string
- ウォーターフォール シリーズとしてシリーズを指定します。
- financial
- タイプ:string
- 財務シリーズとしてシリーズを指定します。
- typicalPriceIndicator
- タイプ:string
- 一般的な価格インジケーター シリーズとしてシリーズを指定します。
- point
- タイプ:string
- ポイント シリーズとしてシリーズを指定します。
- polarSplineArea
- タイプ:string
- 極座標スプライン エリア シリーズとしてシリーズを指定します。
- polarSpline
- タイプ:string
- 極座標スプライン シリーズとしてシリーズを指定します。
- polarArea
- タイプ:string
- ポーラ エリア シリーズとしてシリーズを指定します。
- polarLine
- タイプ:string
- ポーラ折れ線シリーズとしてシリーズを指定します。
- polarScatter
- タイプ:string
- ポーラ散布図シリーズとしてシリーズを指定します。
- radialColumn
- タイプ:string
- ラジアル柱状シリーズとしてシリーズを指定します。
- radialLine
- タイプ:string
- ラジアル折れ線シリーズとしてシリーズを指定します。
- radialPie
- タイプ:string
- ラジアル パイ シリーズとしてシリーズを指定します。
- radialArea
- タイプ:string
- ラジアル エリア シリーズとしてシリーズを指定します。
- scatter
- タイプ:string
- 散布図シリーズとしてシリーズを指定します。
- scatterLine
- タイプ:string
- 散布図 - 折れ線シリーズとしてシリーズを指定します。
- scatterSpline
- タイプ:string
- 散布図スプライン シリーズとしてシリーズを指定します。
- scatterArea
- タイプ:string
- 散布エリア シリーズとしてシリーズを指定します。
- scatterContour
- タイプ:string
- 散布等高線シリーズとしてシリーズを指定します。
- scatterPolygon
- タイプ:string
- 散布多角形シリーズとしてシリーズを指定します。
- scatterPolyline
- タイプ:string
- 散布ポリライン シリーズとしてシリーズを指定します。
- bubble
- タイプ:string
- バブル シリーズとしてシリーズを指定します。
- absoluteVolumeOscillatorIndicator
- タイプ:string
- 絶対容積オシレーター インジケーター シリーズとしてシリーズを指定します。
- averageTrueRangeIndicator
- タイプ:string
- 平均 True 範囲インジケーター シリーズとしてシリーズを指定します。
- accumulationDistributionIndicator
- タイプ:string
- 累積配布インジケーター シリーズとしてシリーズを指定します。
- averageDirectionalIndexIndicator
- タイプ:string
- 平均指向指数インジケーター シリーズとしてシリーズを指定します。
- bollingerBandWidthIndicator
- タイプ:string
- ボリンジャー バンド幅インジケーター シリーズとしてシリーズを指定します。
- chaikinOscillatorIndicator
- タイプ:string
- チャイキン オシレーター インジケーター シリーズとしてシリーズを指定します。
- chaikinVolatilityIndicator
- タイプ:string
- チャイキン ボラティリティ インジケーター シリーズとしてシリーズを指定します。
- commodityChannelIndexIndicator
- タイプ:string
- コモディティ チャネル インデックス (CCI) インジケーター シリーズとしてシリーズを指定します。
- detrendedPriceOscillatorIndicator
- タイプ:string
- トレンド除去価格オシレーター インジケーター シリーズとしてシリーズを指定します。
- easeOfMovementIndicator
- タイプ:string
- イーズ オブ ムーブメント インジケーター シリーズとしてシリーズを指定します。
- fastStochasticOscillatorIndicator
- タイプ:string
- ファスト ストキャスティクス オシレーター インジケーター シリーズとしてシリーズを指定します。
- forceIndexIndicator
- タイプ:string
- 勢力指数インジケーター シリーズとしてシリーズを指定します。
- fullStochasticOscillatorIndicator
- タイプ:string
- フル ストキャスティクス オシレーター インジケーター シリーズとしてシリーズを指定します。
- marketFacilitationIndexIndicator
- タイプ:string
- マーケット ファシリテーション インデックス インジケーター シリーズとしてシリーズを指定します。
- massIndexIndicator
- タイプ:string
- マス インデックス インジケーター シリーズとしてシリーズを指定します。
- medianPriceIndicator
- タイプ:string
- 中間価格インジケーター シリーズとしてシリーズを指定します。
- moneyFlowIndexIndicator
- タイプ:string
- マネー フロー インデックス (MFI) インジケーター シリーズとしてシリーズを指定します。
- movingAverageConvergenceDivergenceIndicator
- タイプ:string
- 移動平均収束拡散手法インジケーター シリーズとしてシリーズを指定します。
- negativeVolumeIndexIndicator
- タイプ:string
- 負出来高指数 (NVI) インジケーター シリーズとしてシリーズを指定します。
- onBalanceVolumeIndicator
- タイプ:string
- バランスボリューム インジケーター シリーズとしてシリーズを指定します。
- percentagePriceOscillatorIndicator
- タイプ:string
- パーセンテージ価格オシレーター インジケーター シリーズとしてシリーズを指定します。
- percentageVolumeOscillatorIndicator
- タイプ:string
- パーセンテージ ボリューム オシレーター インジケーター シリーズとしてシリーズを指定します。
- positiveVolumeIndexIndicator
- タイプ:string
- ポジティブ ボリューム インデックス (PVI) インジケーター シリーズとしてシリーズを指定します。
- priceVolumeTrendIndictor
- タイプ:string
- 価格出来高トレンド インジケーター シリーズとしてシリーズを指定します。
- rateOfChangeAndMomentumIndicator
- タイプ:string
- 変化率とモメンタム インジケーター シリーズを表します。
- relativeStrengthIndexIndicator
- タイプ:string
- 相対力指数インジケーター シリーズとしてシリーズを指定します。
- slowStochasticOscillatorIndicator
- タイプ:string
- スロー ストキャスティクス オシレーター インジケーター シリーズとしてシリーズを指定します。
- standardDeviationIndicator
- タイプ:string
- 標準偏差インジケーター シリーズとしてシリーズを指定します。
- stochRSIIndicator
- タイプ:string
- ストキャスティクス RSI インジケーター シリーズとしてシリーズを指定します。
- trixIndicator
- タイプ:string
- TRIX インジケーター シリーズとしてシリーズを指定します。
- ultimateOscillatorIndicator
- タイプ:string
- アルティメット オシレーター インジケーター シリーズとしてシリーズを指定します。
- weightedCloseIndicator
- タイプ:string
- 重み付きクローズ インジケーター シリーズとしてシリーズを指定します。
- williamsPercentRIndicator
- タイプ:string
- ウィリアム パーセント レンジ インジケーター シリーズとしてシリーズを指定します。
- bollingerBandsOverlay
- タイプ:string
- ボリンジャー バンド オーバーレイ シリーズとしてシリーズを指定します。
- priceChannelOverlay
- タイプ:string
- プライス チャネル オーバーレイ シリーズとしてシリーズを指定します。
- customIndicator
- タイプ:string
- カスタム インジケーター シリーズとしてシリーズを指定します。
- stackedBar
- タイプ:string
- 積層棒シリーズとしてシリーズを指定します。
- stacked100Bar
- タイプ:string
- 積層 100 棒シリーズとしてシリーズを指定します。
- stackedArea
- タイプ:string
- 積層エリア シリーズとしてシリーズを指定します。
- stacked100Area
- タイプ:string
- 積層 100 エリア シリーズとしてシリーズを指定します。
- stackedColumn
- タイプ:string
- 積層柱状シリーズとしてシリーズを指定します。
- stacked100Column
- タイプ:string
- 積層 100 柱状シリーズとしてシリーズを指定します。
- stackedLine
- タイプ:string
- 積層折れ線シリーズとしてシリーズを指定します。
- stacked100Line
- タイプ:string
- 積層 100 折れ線シリーズとしてシリーズを指定します。
- stackedSpline
- タイプ:string
- 積層スプライン シリーズとしてシリーズを指定します。
- stacked100Spline
- タイプ:string
- 積層 100 スプライン シリーズとしてシリーズを指定します。
- stackedSplineArea
- タイプ:string
- 積層スプライン エリア シリーズとしてシリーズを指定します。
- stacked100SplineArea
- タイプ:string
- 積層 100 スプライン エリア シリーズとしてシリーズを指定します。
- crosshairLayer
- タイプ:string
- 十字線レイヤーとしてシリーズを指定します。
- categoryHighlightLayer
- タイプ:string
- カテゴリ強調表示レイヤーとしてシリーズを指定します。
- categoryItemHighlightLayer
- タイプ:string
- カテゴリ項目強調表示レイヤーとしてシリーズを指定します。
- itemToolTipLayer
- タイプ:string
- 項目ツールチップ レイヤーとしてシリーズを指定します。
- categoryToolTipLayer
- タイプ:string
- カテゴリ ツールチップ レイヤーとしてシリーズを指定します。
- calloutLayer
- タイプ:string
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].type;
-
unitsDisplayMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- visible
- タイプ:string
- hidden
- タイプ:string
-
unitsText
- タイプ:
- string
- デフォルト:
- null
-
unitsTextColor
- タイプ:
- string
- デフォルト:
- null
-
unitsTextMarginBottom
- タイプ:
- number
- デフォルト:
- 0
-
unitsTextMarginLeft
- タイプ:
- number
- デフォルト:
- 0
-
unitsTextMarginRight
- タイプ:
- number
- デフォルト:
- 0
-
unitsTextMarginTop
- タイプ:
- number
- デフォルト:
- 0
-
unitsTextStyle
- タイプ:
- object
- デフォルト:
- null
-
unknownValuePlotting
- タイプ:
- enumeration
- デフォルト:
- dontPlot
不明な値をチャートでプロットする方法を決定します。null および Double.NaN は不明な値の例です。
メンバー
- linearInterpolate
- タイプ:string
- 線形補間を使用すると、不明な値を囲む定義した値の中にプロットします。
- dontPlot
- タイプ:string
- 不明な値はチャートにプロットしません。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", unknownValuePlotting: "linearInterpolate" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].unknownValuePlotting; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", unknownValuePlotting: "linearInterpolate" }] );
-
useBruteForce
- タイプ:
- bool
- デフォルト:
- false
ブルート フォース モードを使用するかどうかを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "column", useBruteForce: true }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].useBruteForce; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "column", useBruteForce: true }] );
-
useCartesianInterpolation
- タイプ:
- bool
- デフォルト:
- true
Archimedian の代わりにデカルト補間を使用するかどうかを取得または設定します
スパイラル ベース補間。コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", unknownValuePlotting: "linearInterpolate", useCartesianInterpolation: false }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].useCartesianInterpolation; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", unknownValuePlotting: "linearInterpolate", useCartesianInterpolation: false }] );
-
useHighMarkerFidelity
- タイプ:
- bool
- デフォルト:
- false
短い X 間隔で Y 変動を多く持つ extreme データ図形のマーカーの忠実度を増やすかどうかを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", useHighMarkerFidelity: true }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].useHighMarkerFidelity; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1", useHighMarkerFidelity: true }] );
-
useIndex
- タイプ:
- bool
- デフォルト:
- false
自動的に色を決定するために、現在のレイヤーが系列コレクションのブラシ順序インデックスを使用するかどうかを設定します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "categoryHighlightLayer", name: "categoryHighlightLayer", title: "categoryHighlight", useIndex: "true" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].useIndex; //Set $(".selector").igDataChart("option", "series", [{ type: "categoryHighlightLayer", name: "categoryHighlightLayer", title: "categoryHighlight", useIndex: "true" }] );
-
useInterpolation
- タイプ:
- bool
- デフォルト:
- false
ホバー レイヤーが一番近い値に相対して配置するときに補間を使用するかどうかを取得または設定します。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "column", name: "2005Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005" }, { type: "line", name: "1995Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop1995" }, { type: "crosshairLayer", name: "crosshairLayer", title: "crosshair", useInterpolation: false, transitionDuration: 500, targetSeries: "2005Population", horizontalLineVisibility: "visible" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[2].useInterpolation; //Set $(".selector").igDataChart("option", "series", [{ name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", useInterpolation: false }] );
-
useLegend
- タイプ:
- bool
- デフォルト:
- false
現在のレイヤーがチャートの凡例にエントリを追加するかどうかを設定します。デフォルトで、注釈レイヤーが凡例に表示されていません。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", useLegend: "true" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[0].useLegend; //Set $(".selector").igDataChart("option", "series", [{ name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", useLegend: "true" }] );
-
useSingleShadow
- タイプ:
- bool
- デフォルト:
- true
影付きが完全の系列ビジュアルに適用されるか、系列の各図形に適用されるかどうかを取得または設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", title: "Sales", type: "column", xAxis: "xAxis", yAxis: "yAxis", valueMemberPath: "Value1", useSingleShadow: false }] }); // Get var series = $(".selector").igDataChart("option", "series"); series[0].useSingleShadow; // Set $(".selector").igDataChart("series", [{ name: "series1", useSingleShadow: false }] );
-
useSquareCutoffStyle
- タイプ:
- bool
- デフォルト:
- false
描画トラバーサルを中止する際に、結合領域の図形の代わりに四角を使用するかどうかを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ series: [{ name: "series1", type: "column", useSquareCutoffStyle: true }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].useSquareCutoffStyle; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "column", useSquareCutoffStyle: true }] );
-
valueAxis
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの有効な値軸を取得します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "angleAxis", type: "categoryAngle", label: "Label" }, { name: "radiusAxis", type: "numericRadius" }], series: [{ name: "series1", title: 'Budget', type: "radialLine", angleAxis: "angleAxis", valueAxis: "radiusAxis", valueMemberPath: "Budget" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].valueAxis; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", title: 'Budget', type: "radialLine", angleAxis: "angleAxis", valueAxis: "radiusAxis", valueMemberPath: "Budget" }] );
-
valueFormatAbbreviation
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- independent
- タイプ:string
- shared
- タイプ:string
- kilo
- タイプ:string
- million
- タイプ:string
- billion
- タイプ:string
- trillion
- タイプ:string
- quadrillion
- タイプ:string
- unset
- タイプ:string
- none
- タイプ:string
-
valueFormatCulture
- タイプ:
- string
- デフォルト:
- null
-
valueFormatCurrencyCode
- タイプ:
- string
- デフォルト:
- null
-
valueFormatMaxFractions
- タイプ:
- number
- デフォルト:
- -1
-
valueFormatMinFractions
- タイプ:
- number
- デフォルト:
- -1
-
valueFormatMode
- タイプ:
- enumeration
- デフォルト:
- auto
メンバー
- auto
- タイプ:string
- decimal
- タイプ:string
- currency
- タイプ:string
-
valueFormatSpecifiers
- タイプ:
- object
- デフォルト:
- null
-
valueFormatString
- タイプ:
- string
- デフォルト:
- null
-
valueFormatUseGrouping
- タイプ:
- bool
- デフォルト:
- true
-
valueMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
valueMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズの値を提供する項目パスを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "angleAxis", type: "categoryAngle", label: "Label" }, { name: "radiusAxis", type: "numericRadius" }], series: [{ name: "series1", title: 'Budget', type: "radialLine", angleAxis: "angleAxis", valueAxis: "radiusAxis", valueMemberPath: "Budget" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].valueMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", title: 'Budget', type: "radialLine", angleAxis: "angleAxis", valueAxis: "radiusAxis", valueMemberPath: "Budget" }] );
-
valueRowVisible
- タイプ:
- bool
- デフォルト:
- false
-
valueTextColor
- タイプ:
- string
- デフォルト:
- null
-
valueTextMarginBottom
- タイプ:
- number
- デフォルト:
- 0
-
valueTextMarginLeft
- タイプ:
- number
- デフォルト:
- 2
-
valueTextMarginRight
- タイプ:
- number
- デフォルト:
- 2
-
valueTextMarginTop
- タイプ:
- number
- デフォルト:
- 0
-
valueTextStyle
- タイプ:
- object
- デフォルト:
- null
-
valueTextUseSeriesColors
- タイプ:
- bool
- デフォルト:
- false
-
valueTextWhenMissingData
- タイプ:
- string
- デフォルト:
- "N/A"
-
verticalLineStroke
- タイプ:
- string
- デフォルト:
- null
-
verticalLineVisibility
- タイプ:
- enumeration
- デフォルト:
- visible
レイヤーの垂直十字線の部分が表示されるかどうかを取得または設定します。
メンバー
- visible
- タイプ:string
- レイヤーの垂直十字ポインター部分が表示されます。
- collapsed
- タイプ:string
- レイヤーの垂直十字ポインター部分が非表示になります。
コード サンプル
//Initialization <script type="text/javascript"> $(function () { $("#chart").igDataChart({ dataSource: data, axes: [{ type: "categoryX", name: "NameAxis", label: "CountryName", }, { type: "numericY", name: "PopulationAxis", }], series: [ { type: "column", name: "2005Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop2005" }, { type: "line", name: "1995Population", xAxis: "NameAxis", yAxis: "PopulationAxis", valueMemberPath: "Pop1995" }, { type: "crosshairLayer", name: "crosshairLayer", title: "crosshair", useInterpolation: false, transitionDuration: 500, targetSeries: "2005Population", verticalLineVisibility: "visible" } ] }); }); </script> //Get var series = $(".selector").igDataChart("option", "series"); series[2].verticalLineVisibilty; //Set $(".selector").igDataChart("option", "series", [{ name: "crosshairLayer", title: "crosshair", type: "crosshairLayer", verticalLineVisiblity: "visible" }] );
-
volumeMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの出来高マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "categoryX", dataSource: data, label: "Label" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", volumeMemberPath: "Volume" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].volumeMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", dataSource: data, title: "Price", type: "financial", xAxis: "xAxis", yAxis: "yAxis", openMemberPath: "Open", highMemberPath: "High", lowMemberPath: "Low", closeMemberPath: "Close", volumeMemberPath: "Volume" }] );
-
xAxis
- タイプ:
- string
- デフォルト:
- null
現在のオブジェクトの有効な X 軸を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].xAxis; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] );
-
xAxisAnnoationFormatLabel
- タイプ:
- object
- デフォルト:
- null
-
xAxisAnnotationBackground
- タイプ:
- string
- デフォルト:
- null
-
xAxisAnnotationInterpolatedValuePrecision
- タイプ:
- number
- デフォルト:
- -1
-
xAxisAnnotationOutline
- タイプ:
- string
- デフォルト:
- null
-
xAxisAnnotationPaddingBottom
- タイプ:
- number
- デフォルト:
- NaN
-
xAxisAnnotationPaddingLeft
- タイプ:
- number
- デフォルト:
- NaN
-
xAxisAnnotationPaddingRight
- タイプ:
- number
- デフォルト:
- NaN
-
xAxisAnnotationPaddingTop
- タイプ:
- number
- デフォルト:
- NaN
-
xAxisAnnotationStrokeThickness
- タイプ:
- number
- デフォルト:
- NaN
-
xAxisAnnotationTextColor
- タイプ:
- string
- デフォルト:
- null
-
xMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
xMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].xMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] );
-
yAxis
- タイプ:
- string
- デフォルト:
- null
現在のオブジェクトの有効な Y 軸を取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].yAxis; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] );
-
yAxisAnnoationFormatLabel
- タイプ:
- object
- デフォルト:
- null
-
yAxisAnnotationBackground
- タイプ:
- string
- デフォルト:
- null
-
yAxisAnnotationInterpolatedValuePrecision
- タイプ:
- number
- デフォルト:
- -1
-
yAxisAnnotationOutline
- タイプ:
- string
- デフォルト:
- null
-
yAxisAnnotationPaddingBottom
- タイプ:
- number
- デフォルト:
- NaN
-
yAxisAnnotationPaddingLeft
- タイプ:
- number
- デフォルト:
- NaN
-
yAxisAnnotationPaddingRight
- タイプ:
- number
- デフォルト:
- NaN
-
yAxisAnnotationPaddingTop
- タイプ:
- number
- デフォルト:
- NaN
-
yAxisAnnotationStrokeThickness
- タイプ:
- number
- デフォルト:
- NaN
-
yAxisAnnotationTextColor
- タイプ:
- string
- デフォルト:
- null
-
yMemberAsLegendUnit
- タイプ:
- string
- デフォルト:
- null
-
yMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $(".selector").igDataChart({ axes: [{ name: "xAxis", type: "numericX" }, { name: "yAxis", type: "numericY" }], series: [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] }); //Get var series = $(".selector").igDataChart("option", "series"); series[0].yMemberPath; //Set $(".selector").igDataChart("option", "series", [{ name: "series1", type: "scatter", xAxis: "xAxis", yAxis: "yAxis", xMemberPath: "Index", yMemberPath: "Value1" }] );
-
shouldAutoExpandMarginForInitialLabels
- タイプ:
- bool
- デフォルト:
- false
-
size
- タイプ:
- object
- デフォルト:
- null
チャートの両方のディメンションを一度に設定するには、width および height プロパティを持つオブジェクトを提供できます。
コード サンプル
//Initialize $(".selector").igDataChart({ size: { width: 450, height: 450 } }); //Get var size = $(".selector").igDataChart("option", "size"); //Set var size = $(".selector").igDataChart("option", "size", { width: 450, height: 450 });
-
squareMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上の四角マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
square のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ squareMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
subtitle
- タイプ:
- string
- デフォルト:
- null
コンポーネントに表示するサブタイトル。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", subtitle:"Results over a two year period", subtitleTextColor: "blue", subtitleTextStyle: "16pt Verdona" }); //Get var subtitleValue = $(".selector").igDataChart("option", "subtitle"); //Set $(".selector").igDataChart("option", "subtitle", "Results over a two year period");
-
subtitleBottomMargin
- タイプ:
- number
- デフォルト:
- 0
サブタイトルに使用する下マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", subtitle:"Results over a two year period", subtitleTextColor: "blue", subtitleTextStyle: "16pt Verdona", subtitleBottomMargin: 10 }); //Get var subtitleBottomMarginValue = $(".selector").igDataChart("option", "subtitleBottomMargin"); //Set $(".selector").igDataChart("option", "subtitleBottomMargin", 10);
-
subtitleHorizontalAlignment
- タイプ:
- enumeration
- デフォルト:
- center
サブタイトルに使用する水平方向。
メンバー
- left
- タイプ:string
- サブタイトルを左揃えにします。
- center
- タイプ:string
- サブタイトルを中央揃えにします。
- right
- タイプ:string
- サブタイトルを右揃えにします。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", subtitle:"Results over a two year period", subtitleTextColor: "blue", subtitleTextStyle: "16pt Verdona", subtitleHorizontalAlignment: "center" }); //Get var subtitleHorizontalAlignmentValue = $(".selector").igDataChart("option", "subtitleHorizontalAlignment"); //Set $(".selector").igDataChart("option", "subtitleHorizontalAlignment", "center");
-
subtitleLeftMargin
- タイプ:
- number
- デフォルト:
- 0
サブタイトルに使用する左マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", subtitle:"Results over a two year period", subtitleTextColor: "blue", subtitleTextStyle: "16pt Verdona", subtitleLeftMargin: 10 }); //Get var subtitleLeftMarginValue = $(".selector").igDataChart("option", "subtitleLeftMargin"); //Set $(".selector").igDataChart("option", "subtitleLeftMargin", 10);
-
subtitleRightMargin
- タイプ:
- number
- デフォルト:
- 0
サブタイトルに使用する右マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", subtitle:"Results over a two year period", subtitleTextColor: "blue", subtitleTextStyle: "16pt Verdona", subtitleRightMargin: 10 }); //Get var subtitleRightMarginValue = $(".selector").igDataChart("option", "subtitleRightMargin"); //Set $(".selector").igDataChart("option", "subtitleRightMargin", 10);
-
subtitleTextColor
- タイプ:
- object
- デフォルト:
- black
サブタイトルに使用する色。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", subtitle:"Results over a two year period", subtitleTextColor: "blue", subtitleTextStyle: "16pt Verdona", subtitleLeftMargin: 10 }); //Get var subtitleTextColorValue = $(".selector").igDataChart("option", "subtitleTextColor"); //Set $(".selector").igDataChart("option", "subtitleTextColor", "blue");
-
subtitleTextStyle
- タイプ:
- string
- デフォルト:
- null
タイトルに使用する css フォント プロパティ。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", subtitle:"Results over a two year period", subtitleTextColor: "blue", subtitleTextStyle: "16pt Verdona", subtitleLeftMargin: 10 }); //Get var subtitleTextStyleValue = $(".selector").igDataChart("option", "subtitleTextStyle"); //Set $(".selector").igDataChart("option", "subtitleTextStyle", "16pt Verdona");
-
subtitleTopMargin
- タイプ:
- number
- デフォルト:
- 0
サブタイトルに使用する上マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", subtitle:"Results over a two year period", subtitleTextColor: "blue", subtitleTextStyle: "16pt Verdona", subtitleTopMargin: 10 }); //Get var subtitleTopMarginValue = $(".selector").igDataChart("option", "subtitleTopMargin"); //Set $(".selector").igDataChart("option", "subtitleTopMargin", 10);
-
syncChannel
- タイプ:
- string
- デフォルト:
- null
このチャートを他のチャートと同期化するために使用するチャネル名。
コード サンプル
//Initialize $(".selector").igDataChart({ syncChannel: "syncCharts" }); //Get var syncChannel = $(".selector").igDataChart("option", "syncChannel"); //Set var syncChannel = $(".selector").igDataChart("option", "syncChannel", "syncCharts");
-
synchronizeHorizontally
- タイプ:
- bool
- デフォルト:
- false
チャートが水平に同期されるかどうか。
コード サンプル
// Initialization $(".selector").igDataChart({ synchronizeHorizontally: false }); // Get var opValue = $(".selector").igDataChart("option", "synchronizeHorizontally"); // Set $(".selector").igDataChart("option", "synchronizeHorizontally", false);
-
synchronizeVertically
- タイプ:
- bool
- デフォルト:
- true
チャートが垂直に同期されるかどうか。
コード サンプル
// Initialization $(".selector").igDataChart({ synchronizeVertically: false }); // Get var opValue = $(".selector").igDataChart("option", "synchronizeVertically"); // Set $(".selector").igDataChart("option", "synchronizeVertically", false);
-
tetragramMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上のテトラグラム マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
tetragram のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ tetragramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
theme
- タイプ:
- string
- デフォルト:
- "c"
このウィジェットをスタイルするために使用される見本。
コード サンプル
//Initialize $(".selector").igDataChart({ theme: "metro" }); //Get var theme = $(".selector").igDataChart("option", "theme");
-
title
- タイプ:
- string
- デフォルト:
- null
コンポーネントに表示するタイトル。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona" }); //Get var titleValue = $(".selector").igDataChart("option", "title"); //Set $(".selector").igDataChart("option", "title", "Energy Use Per Country");
-
titleBottomMargin
- タイプ:
- number
- デフォルト:
- 0
タイトルに使用する下マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", titleBottomMargin: 10 }); //Get var titleBottomMarginValue = $(".selector").igDataChart("option", "titleBottomMargin"); //Set $(".selector").igDataChart("option", "titleBottomMargin", 10);
-
titleHorizontalAlignment
- タイプ:
- enumeration
- デフォルト:
- center
タイトルに使用する水平方向。
メンバー
- left
- タイプ:string
- タイトルを左揃えにします。
- center
- タイプ:string
- タイトルを中央揃えにします。
- right
- タイプ:string
- タイトルを右揃えにします。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", titleHorizontalAlignment: "center" }); //Get var titleHorizontalAlignmentValue = $(".selector").igDataChart("option", "titleHorizontalAlignment"); //Set $(".selector").igDataChart("option", "titleHorizontalAlignment", "center");
-
titleLeftMargin
- タイプ:
- number
- デフォルト:
- 0
タイトルに使用する左マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", titleLeftMargin: 10 }); //Get var titleLeftMarginValue = $(".selector").igDataChart("option", "titleLeftMargin"); //Set $(".selector").igDataChart("option", "titleLeftMargin", 10);
-
titleRightMargin
- タイプ:
- number
- デフォルト:
- 0
タイトルに使用する右マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", titleRightMargin: 10 }); //Get var titleRightMarginValue = $(".selector").igDataChart("option", "titleRightMargin"); //Set $(".selector").igDataChart("option", "titleRightMargin", 10);
-
titleTextColor
- タイプ:
- object
- デフォルト:
- black
タイトルに使用する色。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", titleBottomMargin: 10 }); //Get var titleTextColorValue = $(".selector").igDataChart("option", "titleTextColor"); //Set $(".selector").igDataChart("option", "titleTextColor", "red");
-
titleTextStyle
- タイプ:
- string
- デフォルト:
- null
タイトルに使用する css フォント プロパティ。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", titleBottomMargin: 10 }); //Get var titleTextStyleValue = $(".selector").igDataChart("option", "titleTextStyle"); //Set $(".selector").igDataChart("option", "titleTextStyle", "24pt Verdona");
-
titleTopMargin
- タイプ:
- number
- デフォルト:
- 0
タイトルに使用する上マージン。
コード サンプル
// Initialization $(".selector").igDataChart({ title: "Energy Use Per Country", titleTextColor: "red", titleTextStyle: "24pt Verdona", titleTopMargin: 10 }); //Get var titleTopMarginValue = $(".selector").igDataChart("option", "titleTopMargin"); //Set $(".selector").igDataChart("option", "titleTopMargin", 10);
-
topMargin
- タイプ:
- number
- デフォルト:
- NaN
キャンバスでチャート コンテンツの周囲で使用する上余白を取得または設定します。
コード サンプル
//Initialize $(".selector").igDataChart({ topMargin : 20 }); //Get var margin = $(".selector").igDataChart("option", "topMargin"); //Set $(".selector").igDataChart("option", "topMargin", 20);
-
triangleMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
チャート上の三角マーカーに使用するためのテンプレートを取得または設定します。
マーカー タイプが
triangle のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igDataChart({ triangleMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
useTiledZooming
- タイプ:
- bool
- デフォルト:
- false
シリーズ ビューアーがデフォルト ライブ コンテンツではなくキャッシュ タイルを使用するどうかを設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ useTiledZooming: true }); // Get var opValue = $(".selector").igDataChart("option", "useTiledZooming"); // Set $(".selector").igDataChart("option", "useTiledZooming", true);
-
verticalZoomable
非推奨- タイプ:
- bool
- デフォルト:
- false
現在の Chart の垂直ズーム可否を取得または設定します。このオプションは非推奨です。代わりに isVerticalZoomEnabled を使用してください。
コード サンプル
// Initialization $(".selector").igDataChart({ verticalZoomable: true }); // Get var opValue = $(".selector").igDataChart("option", "verticalZoomable"); // Set $(".selector").igDataChart("option", "verticalZoomable", true);
-
width
- タイプ:
- enumeration
- デフォルト:
- null
チャートの幅。ピクセル、文字列 (px)、またはパーセンテージ (%) で数字として設定できます。
コード サンプル
// Initialization $(".selector").igDataChart({ width: "700px" }); // Get var width = $(".selector").igDataChart("option", "width"); // Set $(".selector").igDataChart("option", "width", "700px");
-
windowPositionHorizontal
- タイプ:
- number
- デフォルト:
- 0
水平スクロールの位置を決定する 0 ~ 1 の数。
このプロパティは WindowRect プロパティの X 位置へのショートカットと同じです。コード サンプル
// Initialization $(".selector").igDataChart({ windowPositionHorizontal: 0.25 }); // Get var opValue = $(".selector").igDataChart("option", "windowPositionHorizontal"); // Set $(".selector").igDataChart("option", "windowPositionHorizontal", 0.25);
-
windowPositionVertical
- タイプ:
- number
- デフォルト:
- 0
垂直スクロールの位置を決定する 0 ~ 1 の数。
このプロパティは WindowRect プロパティの Y 位置へのショートカットと同じです。コード サンプル
// Initialization $(".selector").igDataChart({ windowPositionVertical: 0.25 }); // Get var opValue = $(".selector").igDataChart("option", "windowPositionVertical"); // Set $(".selector").igDataChart("option", "windowPositionVertical", 0.25);
-
windowRect
- タイプ:
- object
- デフォルト:
- null
現在表示されているチャート部分を表す矩形。
X = 0、Y = 0、Height および Width が 1 に設定される矩形の場合、プロット可能な領域はすべて表示されていることを意味します。Height および Width は .5 に設定される場合、表示は半分ズームしています。
提供されたオブジェクトは、left、top、width および height と呼ばれる数値プロパティを持っている必要があります。コード サンプル
// Initialization $(".selector").igDataChart({ windowRect: { left: 0, top: 0, width: 0.5, height: 0.5 } }); // Get var opValue = $(".selector").igDataChart("option", "windowRect"); // Set $(".selector").igDataChart("option", "windowRect", { left: 0, top: 0, width: 0.5, height: 0.5 });
-
windowRectMinWidth
- タイプ:
- number
- デフォルト:
- 0
ウィンドウ矩形が固定される前に到達できる最低幅を設定または取得します。
ビューアーにさらにズームできるようにする場合はこの値を減らします。
この値が低すぎると、浮動小数点演算が正確でなくなるため、グラフィックが破損する場合があります。コード サンプル
// Initialization $(".selector").igDataChart({ windowRectMinWidth: 0.5 }); // Get var windowRectMinWidth = $(".selector").igDataChart("option", "windowRectMinWidth"); // Set $(".selector").igDataChart("option", "windowRectMinWidth", 0.5);
-
windowResponse
- タイプ:
- enumeration
- デフォルト:
- null
ユーザー パンニングおよびズーミングに応答します。ユーザー操作が発生しているときにビューを直ちに更新、あるいはユーザー操作が完了した後まで遅延するかどうか。ユーザー操作は、パンニングやズームを発生させるマウスドラッグなどの操作です。
メンバー
- deferred
- タイプ:string
- ユーザー アクションが完了してからビューの更新を開始します。
- immediate
- タイプ:string
- ユーザー アクションの発生と同時にビューを更新します。
コード サンプル
// Initialization $(".selector").igDataChart({ windowResponse: "immediate" }); // Get var opValue = $(".selector").igDataChart("option", "windowResponse"); // Set $(".selector").igDataChart("option", "windowResponse", "immediate");
-
windowScaleHorizontal
- タイプ:
- number
- デフォルト:
- 1
水平ズームのスケールを決定する 0 ~ 1 の数。
このプロパティは WindowRect プロパティの Width へのショートカットと同じです。コード サンプル
// Initialization $(".selector").igDataChart({ windowScaleHorizontal: 0.5 }); // Get var opValue = $(".selector").igDataChart("option", "windowScaleHorizontal"); // Set $(".selector").igDataChart("option", "windowScaleHorizontal", 0.5);
-
windowScaleVertical
- タイプ:
- number
- デフォルト:
- 1
垂直ズームのスケールを決定する 0 ~ 1 の数。
このプロパティは WindowRect プロパティの Height へのショートカットと同じです。コード サンプル
// Initialization $(".selector").igDataChart({ windowScaleVertical: 0.5 }); // Get var opValue = $(".selector").igDataChart("option", "windowScaleVertical"); // Set $(".selector").igDataChart("option", "windowScaleVertical", 0.5);
-
zoomTileCacheSize
- タイプ:
- number
- デフォルト:
- 30
シリーズ ビューアーがタイル ズーム モードでキャッシュするズーム タイルの最大数を設定します。
コード サンプル
// Initialization $(".selector").igDataChart({ zoomTileCacheSize: 30 }); // Get var opValue = $(".selector").igDataChart("option", "zoomTileCacheSize"); // Set $(".selector").igDataChart("option", "zoomTileCacheSize", 30);
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
assigningCategoryMarkerStyle
- キャンセル可能:
- false
カテゴリ系列または財務系列での項目のマーカーのスタイル設定をオーバーライドするために発生するイベント。系列の allowCustomCategoryMarkerStyle を true に設定する場合のみに発生します。
関数は引数 evt および ui を受け取ります。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
ui.startIndex を使用して、現在項目の開始インデックスを取得します。
ui.endIndex を使用して、現在項目の終了インデックスを取得します。
現在の項目のために、startIndex/endIndex の代わりに startDate および endDate を使用するかどうかを決定するには、ui.hasDateRange を使用します。
ui.hasDateRange が true の場合に ui.startDate を使用します。
ui.hasDateRange が true の場合に ui.endDate を使用します。
(必要に応じて) ui.getItems を使用して、イベントと関連するすべての項目を取得します。
ui.fill を使用して、現在項目で使用する塗りつぶしを取得または設定します。
ui.stroke を使用して、現在項目で使用するストロークを取得または設定します。
ui.opacity を使用して、現在項目で使用する不透明度を取得または設定します。
ui.highlightingHandled を使用して、このイベントで処理しているため、デフォルトの強調表示動作を実行しないことを設定します。
ui.maxAllSeriesHighlightingProgress を使用して、すべての系列で最大の強調表示の進行状況を取得します。
ui.sumAllSeriesHighlightingProgress を使用して、すべての系列で強調表示の進行状況の合計数を取得します。
ui.highlightingInfo.progress を使用して、highlightingInfo が null 以外の場合、現在項目の強調表示の進行状況を取得します。
ui.highlightingInfo.state を使用して、highlightingInfo が null 以外の場合、現在項目の強調表示が入っているか、出っているか、静的であるかどうかを取得します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartassigningcategorymarkerstyle", function (evt, ui) { // Overrides the default strokeThickness property of the marker. ui.strokeThickness; // Overrides the default strokeDashArray property of the marker. ui.strokeDashArray; // Overrides the default strokeDashCap property of the marker. ui.strokeDashCap; // Overrides the default radiusX property. ui.radiusX; // Overrides the default radiusY property. ui.radiusY; }); // Initialize $(".selector").igDataChart({ assigningCategoryMarkerStyle: function (evt, ui) { } });
-
assigningCategoryStyle
- キャンセル可能:
- false
カテゴリ系列または財務系列での項目のスタイル設定をオーバーライドするために発生するイベント。系列の allowCustomCategoryStyle を true に設定する場合のみに発生します。
関数は引数 evt および ui を受け取ります。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
ui.startIndex を使用して、現在項目の開始インデックスを取得します。
ui.endIndex を使用して、現在項目の終了インデックスを取得します。
現在の項目のために、startIndex/endIndex の代わりに startDate および endDate を使用するかどうかを決定するには、ui.hasDateRange を使用します。
ui.hasDateRange が true の場合に ui.startDate を使用します。
ui.hasDateRange が true の場合に ui.endDate を使用します。
(必要に応じて) ui.getItems を使用して、イベントと関連するすべての項目を取得します。
ui.fill を使用して、現在項目で使用する塗りつぶしを取得または設定します。
ui.stroke を使用して、現在項目で使用するストロークを取得または設定します。
ui.opacity を使用して、現在項目で使用する不透明度を取得または設定します。
ui.highlightingHandled を使用して、このイベントで処理しているため、デフォルトの強調表示動作を実行しないことを設定します。
ui.maxAllSeriesHighlightingProgress を使用して、すべての系列で最大の強調表示の進行状況を取得します。
ui.sumAllSeriesHighlightingProgress を使用して、すべての系列で強調表示の進行状況の合計数を取得します。
ui.highlightingInfo.progress を使用して、highlightingInfo が null 以外の場合、現在項目の強調表示の進行状況を取得します。
ui.highlightingInfo.state を使用して、highlightingInfo が null 以外の場合、現在項目の強調表示が入っているか、出っているか、静的であるかどうかを取得します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartassigningcategorystyle", function (evt, ui) { // Specifies the start index of the range of data that is currently being highlighted. ui.startIndex; // Specifies the end index of the range of the data that is currently being highlighted. ui.endIndex; // Specifies the start date of the range of data that is currently being highlighted. ui.startDate; // Specifies the end date of the range of data that is currently being highlighted. ui.endDate; // Specifies the actual items from the data source being highlighted. However if there was a lot of data and this was called every time the event was fired, it will negatively impact the performance ui.getItems; // Overrides the default Fill property of the series. However, this property only takes affect if the Fill property only affects that particular series. ui.fill; // Overrides the default Stroke property of the series. ui.stroke; // Overrides the default the Opacity property of the series. ui.opacity; // This is used to determine the styling of the highlighted series. ui.highlightingInfo; // Specifies the progress state of the highlighting of the series. Value from 0 to 1. ui.maxAllSeriesHighlightingProgress; // Specifies the progress state of the highlighting of the series. Value from 0 to 1. ui.sumAllSeriesHighlightingProgress; // When set to True the default highlighting doesn’t apply. ui.highlightingHandled; // Determines if you should use the startDate and endDate to know the current items instead of startIndex/endIndex. ui.hasDataRange; }); // Initialize $(".selector").igDataChart({ assigningCategoryStyle: function (evt, ui) { } });
-
axisRangeChanged
- キャンセル可能:
- false
チャートの範囲および軸が変更した場合に発生するイベント。
関数は引数 evt および ui を受け取ります。
現在のチャート軸 オブジェクトへの参照を取得するには ui.axis を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
新しい最大値を取得するには ui.newMaximumValue を使用します。
新しい最小値を取得するには ui.newMinimumValue を使用します。
以前の最大値を取得するには ui.oldMaximumValue を使用します。
以前の最小値を取得するには ui.oldMinimumValue を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartaxisrangechanged", function (evt, ui) { // Get reference to current chart axis object. ui.axis; // Get reference to chart object. ui.chart; // Get the new maximum value. ui.newMaximumValue; // Get the new minimum value. ui.newMinimumValue; // Get the old maximum value. ui.oldMaximumValue; // Get the old minimum value. ui.oldMinimumValue; }); // Initialize $(".selector").igDataChart({ axisRangeChanged: function (evt, ui) { } });
-
browserNotSupported
- キャンセル可能:
- false
HTML5 非互換のブラウザでコントロールを表示したときに発生するイベント。
コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartbrowsernotsupported", function (evt, ui) { }); // Initialize $(".selector").igDataChart({ browserNotSupported: function (evt, ui) { } });
-
gridAreaRectChanged
- キャンセル可能:
- false
現在の Chart のグリッド領域の矩形が変わった直後に発生します。
(おそらく別のチャート内で) サイズ変更されているチャート、または
追加またはサイズ変更されている 軸の結果に従って、グリッド領域が変更することがあります。
関数は引数 evt および ui を受け取ります。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
新しい高さ値を取得するには ui.newHeight を使用します。
新しい左値を取得するには ui.newLeft を使用します。
新しい上値を取得するには ui.newTop を使用します。
新しい幅値を取得するには ui.newWidth を使用します。
以前の高さ値を取得するには ui.oldHeight を使用します。
以前の左値を取得するには ui.oldLeft を使用します。
以前の上値を取得するには ui.oldTop を使用します。
以前の幅値を取得するには ui.oldWidth を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartgridarearectchanged", function (evt, ui) { // Get reference to chart object. ui.chart; // Get new height value. ui.newHeight; // Get new left value. ui.newLeft; // Get new top value. ui.newTop; // Get new top value. ui.newWidth; // Get old height value. ui.oldHeight; // Get old left value. ui.oldLeft; // Get old top value. ui.oldTop; // Get old top value. ui.oldWidth; }); // Initialize $(".selector").igDataChart({ gridAreaRectChanged: function (evt, ui) { } });
-
progressiveLoadStatusChanged
- キャンセル可能:
- false
シリーズの読み込んでいる状態が変更されたときに発生されるイベント。
関数は引数 evt および ui を受け取ります。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
現在の状態を取得するには ui.currentStatus を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartprogressiveloadstatuschanged", function (evt, ui) { // Get reference to chart object. ui.chart; // Get the series object. ui.series; // Get the current load status. ui.currentStatus; }); // Initialize $(".selector").igDataChart({ progressiveLoadStatusChanged: function (evt, ui) { } });
-
refreshCompleted
- キャンセル可能:
- false
チャートの更新処理が完了すると発生します。
関数は引数 evt および ui を受け取ります。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartrefreshcompleted", function (evt, ui) { // Get reference to chart object. ui.chart; }); // Initialize $(".selector").igDataChart({ refreshCompleted: function (evt, ui) { } });
-
seriesCursorMouseMove
- キャンセル可能:
- false
カーソルがこのチャートのシリーズの上に移動したときに発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartseriescursormousemove", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igDataChart({ seriesCursorMouseMove: function (evt, ui) { } });
-
seriesMouseEnter
- キャンセル可能:
- false
マウス ポインターがこのチャートの要素に入ったときに発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartseriesmouseenter", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igDataChart({ seriesMouseEnter: function (evt, ui) { } });
-
seriesMouseLeave
- キャンセル可能:
- false
マウス ポインターがこのチャートの要素から出たときに発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartseriesmouseleave", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igDataChart({ seriesMouseLeave: function (evt, ui) { } });
-
seriesMouseLeftButtonDown
- キャンセル可能:
- false
マウス ポインターがこのチャートの要素の上にある間に左のマウス ボタンが押されると発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartseriesmouseleftbuttondown", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igDataChart({ seriesMouseLeftButtonDown: function (evt, ui) { } });
-
seriesMouseLeftButtonUp
- キャンセル可能:
- false
マウス ポインターがこのチャートの要素の上にある間に左のマウス ボタンが離されると発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartseriesmouseleftbuttonup", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igDataChart({ seriesMouseLeftButtonUp: function (evt, ui) { } });
-
seriesMouseMove
- キャンセル可能:
- false
マウスのポインターがこのチャートの要素の上にある間にマウス ポインターが移動すると発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartseriesmousemove", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igDataChart({ seriesMouseMove: function (evt, ui) { } });
-
tooltipHidden
- キャンセル可能:
- false
ツールチップが非表示になった後に発生するイベント。
関数は引数 evt および ui を受け取ります。
ツールチップの DOM 要素への参照を取得するには ui.element を使用します。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatacharttooltiphidden", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igDataChart({ tooltipHidden: function (evt, ui) { } });
-
tooltipHiding
- キャンセル可能:
- true
マウスが系列から離れ、ツールチップが非表示になりそうなときに発生するイベント。
関数は引数 evt および ui を受け取ります。
ツールチップの DOM 要素への参照を取得するには ui.element を使用します。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatacharttooltiphiding", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igDataChart({ tooltipHiding: function (evt, ui) { } });
-
tooltipShowing
- キャンセル可能:
- true
マウスがシリーズにホバーされ、ツールチップが表示されるときに発生するイベント。
関数は引数 evt および ui を受け取ります。
ツールチップの DOM 要素への参照を取得するには ui.element を使用します。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatacharttooltipshowing", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igDataChart({ tooltipShowing: function (evt, ui) { } });
-
tooltipShown
- キャンセル可能:
- false
ツールチップが表示された後に発生するイベント。
関数は引数 evt および ui を受け取ります。
ツールチップの DOM 要素への参照を取得するには ui.element を使用します。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatacharttooltipshown", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to chart object. ui.chart; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igDataChart({ tooltipShown: function (evt, ui) { } });
-
typicalBasedOn
- キャンセル可能:
- false
このイベントは、カスタム一般価格の基となる列を指定するために処理します。
関数は引数 evt および ui を受け取ります。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
先頭から計算される位置の数を取得するには ui.count を使用します。
計算される開始位置を取得するには ui.position を使用します。
計算で使用する補助計算を取得するには ui.supportingCalculations を使用します。
計算で使用するデータを取得するには ui.dataSource を使用します。
どの列の変更でシリーズが無効になり再計算が発生するかを指定するために ui.basedOn を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatacharttypicalbasedon", function (evt, ui) { // Get reference to chart object. ui.chart; // Get the series object. ui.series; // Get the the number of positions that should be calculated from the start. ui.count; // Get the beginning position that should be calculated from. ui.position; // Get the supporting calculations to use in the calculation. ui.supportingCalculations; // Get the data to use for the calculation. ui.dataSource; // Specify which columns changing will invalidate the series and cause it to be recalculated. ui.basedOn; }); // Initialize $(".selector").igDataChart({ typicalBasedOn: function (evt, ui) { } });
-
windowRectChanged
- キャンセル可能:
- false
現在の Chart のウィンドウ矩形が変わった直後に発生します。
関数は引数 evt および ui を受け取ります。
チャート オブジェクトへの参照を取得するには ui.chart を使用します。
新しい高さ値を取得するには ui.newHeight を使用します。
新しい左値を取得するには ui.newLeft を使用します。
新しい上値を取得するには ui.newTop を使用します。
新しい幅値を取得するには ui.newWidth を使用します。
以前の高さ値を取得するには ui.oldHeight を使用します。
以前の左値を取得するには ui.oldLeft を使用します。
以前の上値を取得するには ui.oldTop を使用します。
以前の幅値を取得するには ui.oldWidth を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igdatachartwindowrectchanged", function (evt, ui) { // Get reference to chart object. ui.chart; // Get new height value. ui.newHeight; // Get new left value. ui.newLeft; // Get new top value. ui.newTop; // Get new top value. ui.newWidth; // Get old height value. ui.oldHeight; // Get old left value. ui.oldLeft; // Get old top value. ui.oldTop; // Get old top value. ui.oldWidth; }); // Initialize $(".selector").igDataChart({ windowRectChanged: function (evt, ui) { } });
-
addItem
- .igDataChart( "addItem", item:object, targetName:string );
新しい項目をデータ ソースに追加し、チャートに通知します。
- item
- タイプ:object
- データ ソースに追加する必要がある項目。
- targetName
- タイプ:string
- データ ソースにバインドされたシリーズまたは軸の名前。 データシリーズまたは軸にバインドされる場合のみ返します。データを igDataChart の dataSource にバインドする場合、2 つ目パラメーターは設定しないでください。
コード サンプル
$(".selector").igDataChart("addItem", {"Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, "series1" );
-
changeGlobalLanguage
継承- .igDataChart( "changeGlobalLanguage" );
ウィジェットの言語をグローバルの言語に変更します。グローバルの言語は $.ig.util.language の値です。
コード サンプル
$(".selector").igDataChart("changeGlobalLanguage");
-
changeGlobalRegional
継承- .igDataChart( "changeGlobalRegional" );
ウィジェットの地域設定をグローバルの地域設定に変更します。グローバルの地域設定は $.ig.util.regional にあります。
コード サンプル
$(".selector").igDataChart("changeGlobalRegional");
-
changeLocale
継承- .igDataChart( "changeLocale", $container:object );
指定したコンテナーに含まれるすべてのロケールを options.language で指定した言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。- $container
- タイプ:object
- オプションのパラメーター: 設定しない場合、ウィジェットの要素を $container として使用します。
コード サンプル
$(".selector").igDataChart("changeLocale");
-
clearTileZoomCache
- .igDataChart( "clearTileZoomCache" );
新しいタイルが生成されるためにタイル ズームのタイル キャッシュをクリアします。ビューアーがタイル ズームを使用している場合のみ影響します。
コード サンプル
$(".selector").igDataChart("clearTileZoomCache");
-
destroy
- .igDataChart( "destroy" );
ウィジェットを破棄します。
コード サンプル
$(".selector").igDataChart("destroy");
-
endTiledZoomingIfRunning
- .igDataChart( "endTiledZoomingIfRunning" );
実行されている場合、タイル ズームを手動的に終了します。
コード サンプル
$(".selector").igDataChart("endTiledZoomingIfRunning");
-
exportImage
- .igDataChart( "exportImage", [width:object], [height:object] );
- 返却型:
- object
- 返却型の説明:
- IMG DOM 要素を返します。
チャートを PNG 画像にエクスポートします。
- width
- タイプ:object
- オプション
- 画像の幅。
- height
- タイプ:object
- オプション
- 画像の高さ。
コード サンプル
var pngImage = $(".selector").igDataChart("exportImage", 200, 100);
-
exportVisualData
- .igDataChart( "exportVisualData" );
ユニット テストで支援するためにチャートからビジュアル データをエクスポートします。
コード サンプル
var data = $(".selector").igDataChart("exportVisualData");
-
flush
- .igDataChart( "flush" );
続行の前に保留中の作業をチャートで描画するように強制します。
コード サンプル
$(".selector").igDataChart("flush");
-
getActualInterval
- .igDataChart( "getActualInterval", targetName:string );
ターゲットの数値または日時軸の実際の間隔を取得します。
- targetName
- タイプ:string
- 間隔を取得する軸の名前。
-
getActualMaximumValue
- .igDataChart( "getActualMaximumValue", targetName:string );
ターゲットの数値または日時軸の実際の最大値を取得します。
- targetName
- タイプ:string
- 最大値を取得する軸の名前。
コード サンプル
var maxAxes = $(".selector").igDataChart("getActualMaximumValue", axe);
-
getActualMinimumValue
- .igDataChart( "getActualMinimumValue", targetName:string );
ターゲットの数値または日時軸の実際の最小値を取得します。
- targetName
- タイプ:string
- 最小値を取得する軸の名前。
コード サンプル
var minAxes = $(".selector").igDataChart("getActualMinimumValue", axis);
-
getItem
- .igDataChart( "getItem", targetName:string, worldPoint:object );
- 返却型:
- object
- 返却型の説明:
- 最近傍の項目です。
指定したワールド座標値に最も適合する項目を取得します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
コード サンプル
var item = $(".selector").igDataChart("getItem", series1, {x:0.5, y:0.5});
-
getItemFromSeriesPixel
- .igDataChart( "getItemFromSeriesPixel", targetName:string, seriesPoint:object );
- 返却型:
- object
- 返却型の説明:
- 最近傍の項目です。
指定したワールド座標値に最も適合する項目を取得します。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表す系列ピクセル位置 (書式: {x: [number], y: [number]} ) 。
コード サンプル
var item = $(".selector").igDataChart("getItemFromSeriesPixel", series1, {x:0.5, y:0.5});
-
getItemIndex
- .igDataChart( "getItemIndex", targetName:string, worldPoint:object );
- 返却型:
- number
- 返却型の説明:
- 項目インデックス。ワールド位置に項目が関連付けられていない場合は -1。
指定したワールド位置に関連付けられた項目インデックスを取得します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
コード サンプル
var item = $(".selector").igDataChart("getItemIndex", series1, {x:0.5, y:0.5});
-
getItemIndexFromSeriesPixel
- .igDataChart( "getItemIndexFromSeriesPixel", targetName:string, seriesPoint:object );
- 返却型:
- number
- 返却型の説明:
- 項目インデックス。ワールド位置に項目が関連付けられていない場合は -1。
指定した系列ピクセル座標に関連付けられた項目インデックスを取得します。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
コード サンプル
var item = $(".selector").igDataChart("getItemIndexFromSeriesPixel", series1, {x:0.5, y:0.5});
-
getItemSpan
- .igDataChart( "getItemSpan", targetName:string );
- 返却型:
- number
- 返却型の説明:
- シリーズ項目のカテゴリ内の幅。
カテゴリ プロット シリーズの場合、項目の現在の幅をカテゴリ内で使用します。項目に幅 (列、棒など) がある場合にのみ値を返します。そうでない場合は、0 を返します。
- targetName
- タイプ:string
- 対象するシリーズの名前。
コード サンプル
var span = $(".selector").igDataChart("getItemSpan", "series1");
-
getSeriesCategoryWidth
- .igDataChart( "getSeriesCategoryWidth", targetName:string );
- 返却型:
- number
- 返却型の説明:
- カテゴリのピクセル幅。
系列のカテゴリ幅を取得します。
- targetName
- タイプ:string
- 対象する系列の名前。
コード サンプル
var item = $(".selector").igDataChart("getSeriesCategoryWidth", series1);
-
getSeriesHighValue
- .igDataChart( "getSeriesHighValue", targetName:string, worldPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- number
- 返却型の説明:
- 指定したワールド座標にあるシリーズの値。利用可能ではない場合、double.NaN。
特定のワールド座標の場合、系列の最適な高値を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesHighValue", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesHighValueFromSeriesPixel
- .igDataChart( "getSeriesHighValueFromSeriesPixel", targetName:string, seriesPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- number
- 返却型の説明:
- 指定したワールド座標にあるシリーズの値。利用可能ではない場合、double.NaN。
特定のワールド座標の場合、系列の最適な高値を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表す系列ピクセル位置 (書式: {x: [number], y: [number]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesHighValueFromSeriesPixel", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesHighValuePosition
- .igDataChart( "getSeriesHighValuePosition", targetName:string, worldPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- object
- 返却型の説明:
- シリーズ ピクセル スペースでシリーズの最適な高値の位置。
特定のワールド座標の場合、系列の最適な高値位置を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesHighValuePosition", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesHighValuePositionFromSeriesPixel
- .igDataChart( "getSeriesHighValuePositionFromSeriesPixel", targetName:string, seriesPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- object
- 返却型の説明:
- シリーズ ピクセル スペースでシリーズの最適な高値の位置 (書式: {x: [number], y: [number]})。
特定のワールド座標の場合、系列の最適な高値位置を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表す系列ピクセル位置 (書式: {x: [number], y: [number]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesHighValuePositionFromSeriesPixel", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesLowValue
- .igDataChart( "getSeriesLowValue", targetName:string, worldPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- number
- 返却型の説明:
- 指定したワールド座標にあるシリーズの値。利用可能ではない場合、double.NaN。
特定のワールド座標の場合、系列の最適な安値を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesLowValue", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesLowValueFromSeriesPixel
- .igDataChart( "getSeriesLowValueFromSeriesPixel", targetName:string, seriesPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- number
- 返却型の説明:
- 指定したワールド座標にあるシリーズの値。利用可能ではない場合、double.NaN。
特定のワールド座標の場合、系列の最適な安値を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表す系列ピクセル位置 (書式: {x: [number], y: [number]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesLowValueFromSeriesPixel", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesLowValuePosition
- .igDataChart( "getSeriesLowValuePosition", targetName:string, worldPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- object
- 返却型の説明:
- シリーズ ピクセル スペースでシリーズの最適な安値の位置。
特定のワールド座標の場合、系列の最適な安値位置を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesLowValuePosition", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesLowValuePositionFromSeriesPixel
- .igDataChart( "getSeriesLowValuePositionFromSeriesPixel", targetName:string, seriesPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- object
- 返却型の説明:
- シリーズ ピクセル スペースでシリーズの最適な安値の位置 (書式: {x: [number], y: [number]})。
特定のワールド座標の場合、系列の最適な安値位置を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表す系列ピクセル位置 (書式: {x: [number], y: [number]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesLowValuePositionFromSeriesPixel", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesOffsetValue
- .igDataChart( "getSeriesOffsetValue", targetName:string );
- 返却型:
- number
- 返却型の説明:
- ピクセルのカテゴリ オフセット。
系列のカテゴリ オフセットを取得します。
- targetName
- タイプ:string
- 対象する系列の名前。
コード サンプル
var item = $(".selector").igDataChart("getSeriesOffsetValue", series1);
-
getSeriesValue
- .igDataChart( "getSeriesValue", targetName:string, worldPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- number
- 返却型の説明:
- 指定したワールド座標にあるシリーズの値。利用可能ではない場合、double.NaN。
特定のワールド座標の場合、系列の最適なメイン値を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesValue", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesValueBoundingBox
- .igDataChart( "getSeriesValueBoundingBox", targetName:string, worldPoint:object );
- 返却型:
- object
- 返却型の説明:
- 境界ボックス。有効な位置がない場合は空です。top、left、width、および height プロパティを持つオブジェクト リテラルです。
提供されたワールド座標と一致する最適な値を持つシリーズの利用可能な値の境界ボックスを返します。
- targetName
- タイプ:string
- 対象にするシリーズの名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
コード サンプル
var bbox = $(".selector").igDataChart("getSeriesValueBoundingBox", "series1", { x: 0.5, y: 0.5 });
-
getSeriesValueBoundingBoxFromSeriesPixel
- .igDataChart( "getSeriesValueBoundingBoxFromSeriesPixel", targetName:string, seriesPoint:object );
- 返却型:
- object
- 返却型の説明:
- 境界ボックス。有効な位置がない場合は空です。top、left、width、および height プロパティを持つオブジェクト リテラルです。
指定したシリーズ ピクセル座標と一致する最適な値を持つシリーズの利用可能な値の境界ボックスを返します。
- targetName
- タイプ:string
- 対象にするシリーズの名前。
- seriesPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表すシリーズ ピクセル位置 (書式: {x: [number], y: [number]} ) 。
コード サンプル
var bbox = $(".selector").igDataChart("getSeriesValueBoundingBoxFromSeriesPixel", "series1", { x: 0.5, y: 0.5 });
-
getSeriesValueFineGrainedBoundingBoxes
- .igDataChart( "getSeriesValueFineGrainedBoundingBoxes", targetName:string, worldPoint:object );
- 返却型:
- object
- 返却型の説明:
- 詳細に設定された値の境界ボックス。有効な位置がない場合は空です。top、left、width、および height プロパティを持つオブジェクト リテラルです。
提供されたワールド座標と一致する最適な値を持つシリーズの利用可能な詳細に設定された最適な値の境界ボックスを返します。
- targetName
- タイプ:string
- 対象にするシリーズの名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
コード サンプル
var bbox = $(".selector").igDataChart("getSeriesValueFineGrainedBoundingBoxes", "series1", { x: 0.5, y: 0.5 });
-
getSeriesValueFineGrainedBoundingBoxesFromSeriesPixel
- .igDataChart( "getSeriesValueFineGrainedBoundingBoxesFromSeriesPixel", targetName:string, worldPoint:object );
- 返却型:
- object
- 返却型の説明:
- 詳細に設定された値の境界ボックス。有効な位置がない場合は空です。top、left、width、および height プロパティを持つオブジェクト リテラルです。
提供されたシリーズ ピクセル座標と一致する最適な値を持つシリーズの利用可能な詳細に設定された最適な値の境界ボックスを返します。
- targetName
- タイプ:string
- 対象にするシリーズの名前。
- worldPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表すシリーズ ピクセル位置 (書式: {x: [number], y: [number]} ) 。
コード サンプル
var bbox = $(".selector").igDataChart("getSeriesValueFineGrainedBoundingBoxesFromSeriesPixel", "series1", { x: 0.5, y: 0.5 });
-
getSeriesValueFromSeriesPixel
- .igDataChart( "getSeriesValueFromSeriesPixel", targetName:string, seriesPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- number
- 返却型の説明:
- 指定したワールド座標にあるシリーズの値。利用可能ではない場合、double.NaN。
特定のワールド座標の場合、系列の最適なメイン値を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表す系列ピクセル位置 (書式: {x: [number], y: [number]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesValueFromSeriesPixel", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesValuePosition
- .igDataChart( "getSeriesValuePosition", targetName:string, worldPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- object
- 返却型の説明:
- シリーズ ピクセル スペースでシリーズの最適なメイン値の位置。
特定のワールド座標の場合、系列の最適なメイン値位置を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- 軸の間の位置を表すワールド位置 (書式: {x: [0 ~ 1 の数], y: [0 ~ 1 の数]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesValuePosition", series1, {x:0.5, y:0.5}, true, true);
-
getSeriesValuePositionFromSeriesPixel
- .igDataChart( "getSeriesValuePositionFromSeriesPixel", targetName:string, seriesPoint:object, useInterpolation:bool, skipUnknowns:bool );
- 返却型:
- object
- 返却型の説明:
- シリーズ ピクセル スペースでシリーズの最適なメイン値の位置 (書式: {x: [number], y: [number]})。
特定のワールド座標の場合、系列の最適なメイン値位置を返します。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- シリーズのピクセル スペースの位置を表す系列ピクセル位置 (書式: {x: [number], y: [number]} ) 。
- useInterpolation
- タイプ:bool
- true の場合、データ セットの実際値のみの代わりに、値の中にある値を取得するには補間を使用します。
- skipUnknowns
- タイプ:bool
- true の場合、不明な値をスキップします。
コード サンプル
var item = $(".selector").igDataChart("getSeriesValuePositionFromSeriesPixel", series1, {x:0.5, y:0.5}, true, true);
-
id
- .igDataChart( "id" );
- 返却型:
- string
チャートを含む親要素の ID を返します。
コード サンプル
var dataContainerElement = $(".selector").igDataChart("id");
-
insertItem
- .igDataChart( "insertItem", item:object, index:number, targetName:string );
新しい項目をデータ ソースに挿入し、チャートに通知します。
- item
- タイプ:object
- データ ソースに挿入する必要がある新規項目。
- index
- タイプ:number
- 新しい項目が挿入されるデータ ソースのインデックス。
- targetName
- タイプ:string
- データ ソースにバインドされたシリーズまたは軸の名前。
コード サンプル
$(".selector").igDataChart("insertItem", {"Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, 1, "series1" );
-
moveCursorPoint
- .igDataChart( "moveCursorPoint", targetName:string, worldPoint:object );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
対象の注釈レイヤーのカーソル ポイントを指定したワールド座標に移動します。
- targetName
- タイプ:string
- 対象する系列の名前。
- worldPoint
- タイプ:object
- カーソルへ移動するポイント。タイプ数を持つ x プロパティおよび y プロパティを持つ必要があります。
コード サンプル
$(".selector").igDataChart("moveCursorPoint", "series1", { x: 0, y: 0 });
-
notifyClearItems
- .igDataChart( "notifyClearItems", dataSource:object );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
項目が関連付けられたデータ ソースからクリアされたことをチャートに通知します。
同じ項目のソースを共有しているかどうか、変更の複数のターゲットに通知する必要はありません。- dataSource
- タイプ:object
- 変更が発生したデータ ソース。
コード サンプル
var response = $(".selector").igDataChart("notifyClearItems", series1DataSource);
-
notifyInsertItem
- .igDataChart( "notifyInsertItem", dataSource:object, index:number, newItem:object );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
項目がそのデータ ソースの指定されたインデックスに挿入されたことを対象の軸またはシリーズに通知します。
同じ項目のソースを共有しているかどうか、変更の複数のターゲットに通知する必要はありません。- dataSource
- タイプ:object
- 変更が発生したデータ ソース。
- index
- タイプ:number
- 新しい項目が挿入された項目ソースのインデックス。
- newItem
- タイプ:object
- コレクションに設定された新しい項目。
コード サンプル
var response = $(".selector").igDataChart("notifyInsertItem", series1DataSource, 1, {"Item1": "Value1", "Item2": 1000, "Item3": 1019.75});
-
notifyRemoveItem
- .igDataChart( "notifyRemoveItem", dataSource:object, index:number, oldItem:object );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
項目がそのデータ ソースの指定されたインデックスから削除されたことを対象の軸またはシリーズに通知します。
同じ項目のソースを共有しているかどうか、変更の複数のターゲットに通知する必要はありません。- dataSource
- タイプ:object
- 変更が発生したデータ ソース。
- index
- タイプ:number
- 旧項目の削除元となった項目ソースのインデックス。
- oldItem
- タイプ:object
- コレクションから削除された旧項目。
コード サンプル
var response = $(".selector").igDataChart("notifyRemoveItem", series1DataSource, 1, {"Item1": "Value1", "Item2": 1000, "Item3": 1019.75});
-
notifySetItem
- .igDataChart( "notifySetItem", dataSource:object, index:number, newItem:object, oldItem:object );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
項目が関連付けられたデータ ソースに設定されたことをチャートに通知します。
- dataSource
- タイプ:object
- 変更が発生したデータ ソース。
- index
- タイプ:number
- 変更された項目ソースのインデックス。
- newItem
- タイプ:object
- コレクションに設定された新しい項目。
- oldItem
- タイプ:object
- コレクションで上書きされた旧項目。
コード サンプル
var response = $(".selector").igDataChart("notifySetItem", series1DataSource, 1, {"Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, {"Item1": "Value1", "Item2": 999, "Item3": 1019.75});
-
notifyVisualPropertiesChanged
- .igDataChart( "notifyVisualPropertiesChanged", targetName:string );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
視覚プロパティに影響するものが変更して、視覚出力を再描画する必要があることをターゲット系列に通知します。
- targetName
- タイプ:string
- 通知する系列の名前。
コード サンプル
$(".selector").igDataChart("notifyVisualPropertiesChanged", "series1");
-
option
- .igDataChart( "option" );
-
print
- .igDataChart( "print" );
チャートを表示する印刷プレビュー ページを作成し、ページのすべての他の要素を非表示にします。
コード サンプル
$(".selector").igDataChart("print");
-
removeItem
- .igDataChart( "removeItem", index:number, targetName:string );
項目をデータ ソースから削除し、チャートに通知します。
- index
- タイプ:number
- 項目が削除されるデータ ソースのインデックス。
- targetName
- タイプ:string
- データ ソースにバインドされたシリーズまたは軸の名前。データシリーズまたは軸にバインドされる場合のみ返します。データを igDataChart の dataSource にバインドする場合、2 つ目パラメーターは設定しないでください。
コード サンプル
$(".selector").igDataChart("removeItem", 1, "series1");
-
renderSeries
- .igDataChart( "renderSeries", targetName:string, animate:bool );
更新を実行するオプションが変更されなくても、シリーズを描画することを示します。
- targetName
- タイプ:string
- 描画するシリーズの名前。
- animate
- タイプ:bool
- 可能な場合、変更をアニメーション化するかどうか。
コード サンプル
$(".selector").igDataChart("renderSeries", "xAxis", true);
-
replayTransitionIn
- .igDataChart( "replayTransitionIn", targetName:string );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
系列の入るトランジションのアニメーションを再度再生します。
- targetName
- タイプ:string
- 対象する系列の名前。
コード サンプル
$(".selector").igDataChart("replayTransitionIn", "series1");
-
resetCachedEnhancedInterval
- .igDataChart( "resetCachedEnhancedInterval", targetName:string );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
ターゲット軸で、間隔管理と詳細な間隔調整を使用する場合、キャッシュ済みの最大ラベル幅がリセットされ、現在のラベルで再計算されます。
- targetName
- タイプ:string
- 通知する軸の名前。
-
resetZoom
- .igDataChart( "resetZoom" );
- 返却型:
- object
- 返却型の説明:
- この igChart への参照を返します。
チャートのズーム レベルをデフォルト値にリセットします。
コード サンプル
var response = $(".selector").igDataChart("resetZoom");
-
scaleValue
- .igDataChart( "scaleValue", targetName:string, unscaledValue:number );
- 返却型:
- number
- 返却型の説明:
- スケールされた値を返します。
対象の軸に、要求された値を軸スペースからチャート スペースに拡大するよう通知します。
たとえば、チャートの幅に相対して x 軸の 50 値の位置を検索するために、このメソッドを使用できます。- targetName
- タイプ:string
- 通知する軸の名前。
- unscaledValue
- タイプ:number
- チャート スペースに変換する軸スペースの値。
コード サンプル
var scaled = $(".selector").igDataChart("scaleValue", "axis1", 250);
-
scrollIntoView
- .igDataChart( "scrollIntoView", targetName:string, item:object );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
対象の軸または系列に、要求されたデータ項目をビューにスクロールするよう通知します。
- targetName
- タイプ:string
- 通知する軸または系列の名前。
- item
- タイプ:object
- 可能な場合、ビューに入れるデータ項目。
コード サンプル
var response = $(".selector").igDataChart("scrollIntoView", 5);
-
setItem
- .igDataChart( "setItem", index:number, item:object, targetName:string );
データ ソース内の項目を更新し、チャートに通知します。
- index
- タイプ:number
- 変更する必要があるデータ ソース内の項目のインデックス。
- item
- タイプ:object
- データ ソース内で設定する新規項目オブジェクト。
- targetName
- タイプ:string
- データ ソースにバインドされたシリーズまたは軸の名前。
コード サンプル
$(".selector").igDataChart("setItem", 5, {"Item1": "Value1", "Item2": 1000, "Item3": 1019.75}, "series1");
-
simulateHover
- .igDataChart( "simulateHover", targetName:string, seriesPoint:object );
- 返却型:
- object
- 返却型の説明:
- このチャートへの参照を返します。
系列のビューポートの特定のポイントの上にホバー操作をシミュレートします。
- targetName
- タイプ:string
- 対象する系列の名前。
- seriesPoint
- タイプ:object
- ホバーするポイント。タイプ数を持つ x プロパティおよび y プロパティを持つ必要があります。
コード サンプル
$(".selector").igDataChart("simulateHover", "series1", { x: 100, y: 100 });
-
startTiledZoomingIfNecessary
- .igDataChart( "startTiledZoomingIfNecessary" );
実行されていない場合、タイル ズームを手動的に開始します。
コード サンプル
$(".selector").igDataChart("startTiledZoomingIfNecessary");
-
styleUpdated
- .igDataChart( "styleUpdated" );
- 返却型:
- object
- 返却型の説明:
- この igChart への参照を返します。
色の描画元になるスタイルが更新された可能性があることをチャートに通知します。
コード サンプル
var response = $(".selector").igDataChart("styleUpdated");
-
unscaleValue
- .igDataChart( "unscaleValue", targetName:string, scaledValue:number );
- 返却型:
- number
- 返却型の説明:
- スケールされていない値を返します。
対象の軸に、要求された値をチャート スペースから軸スペースに縮小するよう通知します。
たとえば、チャートの幅にスケールされないで x 軸の値を検索するために、このメソッドを使用できます。- targetName
- タイプ:string
- 通知する軸の名前。
- scaledValue
- タイプ:number
- 軸スペースに変換するチャート スペースの値。
コード サンプル
var unscaled = $(".selector").igDataChart("unscaleValue", "axis1", 50);
-
widget
- .igDataChart( "widget" );
チャートを含む要素を返します。
コード サンプル
var chartWidget = $(".selector").igDataChart("widget");
-
ui-chart-aligned-gridlines
- visibility CSS プロパティがこのクラスで visible に設定される場合、チャートのすべてのグリッド線がピクセルにスナップされます。
-
ui-angular-axis-labels
- 角度軸のラベルにフォント色を適用するクラス。
-
ui-chart-area-fill-opacity
- すべてのエリア型系列の塗りつぶし図形に不透明度値を適用するクラス。
-
ui-chart-axis
- すべてのチャート軸線に border-color を適用するクラス。
-
ui-chart-axis-major-line
- チャート軸の主線に border-color を適用するクラス。「Transparent」は「auto」として使用されます。つまり、チャートが系列タイプに基づいて表示される線を決定します。水平カテゴリ系列 (柱状、折れ線、エリア) の場合、水平線のみを表示します。垂直カテゴリ系列 (棒) の場合、垂直線のみを表示します。散布、極座標、およびラジアル系列の場合、すべてのグリッド線を表示します。
-
ui-chart-axis-stroke
- チャート軸のストローク線に border-color を適用するクラス。「Transparent」は「auto」として使用されます。つまり、チャートが系列タイプに基づいて表示される線を決定します。水平カテゴリ系列 (柱状、折れ線、エリア) の場合、水平線のみを表示します。垂直カテゴリ系列 (棒) の場合、垂直線のみを表示します。散布、極座標、およびラジアル系列の場合、すべてのグリッド線を表示します。
-
ui-chart-category-axis-tick
- カテゴリ軸の目盛りの幅/高さを指定するクラス。
-
ui-corner-all ui-widget-content ui-chart-container
- div 要素に適用されるクラス。
-
ui-horizontal-axis-labels
- 水平軸のラベルにフォント色、マージン、垂直方向の配置を適用するクラス。
-
ui-chart-horizontal-axis-title
- 水平チャート軸のタイトルにフォント、色、マージンを適用するクラス。
-
ui-chart-legend-item-badge
- 凡例項目のアイコンにすべてのスタイル設定オプションを設定するクラス。
-
ui-chart-legend-items-list
- チャート凡例にすべてのスタイル設定オプションを設定するクラス。
-
ui-chart-legend-item-text
- 凡例項目のテキストにすべてのスタイル設定オプションを設定するクラス。
-
ui-radial-axis-labels
- ラジアル軸のラベルにフォント色、マージン、垂直方向の配置を適用するクラス。
-
ui-chart-fill-palette-n
- チャートの n 番のシリーズの塗りつぶしに background-image を適用するクラス。グラデーション色のみを設定できます。複数のパレットを定義できます。
-
ui-chart-outline-palette-n
- チャートの n 番のシリーズのアウトラインに background-image を適用するクラス。グラデーション色のみを設定できます。複数のパレットを定義できます。
-
ui-chart-palette-n
- チャートの n 番のシリーズに background-color および border-color を適用するクラス。複数のパレットを定義できます。
-
ui-chart-subtitle
- チャートのサブタイトルにフォント、色、マージンを適用するクラス。
-
ui-chart-title
- チャートのタイトルにフォント、色、マージンを適用するクラス。
-
ui-chart-tooltip ui-widget-content ui-corner-all
- ツールチップ DIV 要素に適用されるクラス。
-
ui-html5-non-html5-supported-message ui-helper-clearfix ui-html5-non-html5
- DIV 要素に適用します。チャートが HTML5 非互換のブラウザーで開かれているときに表示します。
-
ui-vertical-axis-labels
- 垂直軸のラベルにフォント色、マージン、水平方向のテキスト配置を適用するクラス。
-
ui-chart-vertical-axis-title
- 垂直チャート軸のタイトルにフォント、色、マージンを適用するクラス。