ui.igMap
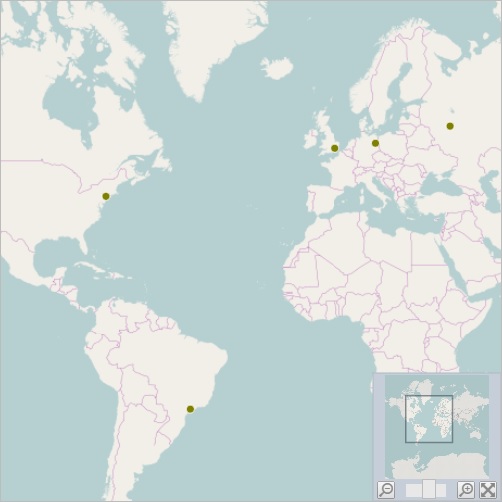
igMap コントロールは、Infragistics Silverlight xamMap™ コントロールに基づいた HTML5 jQuery マップ コントロールです。このコントロールは、マップ プロバイダー (OpenStreetMap®、Bing® Maps および CloudMade® Maps など) が提供する汎用のマップ データをプロットして、追加のマップ レイヤーを描画する機能を公開します。たとえば、特定のマップの位置をマークして、マップのポイントに情報を表示することができます。シェイプ ファイルを使用して地理的領域の周囲に図形を描画し、マップ領域に添付されたデータ パラメーターに応じて異なる色を使用できます。この API のクラス、オプション、イベント、メソッド、およびテーマの詳細は、上記の関連するタブの下に表示されます。
次のコード スニペットは igMap コントロールの初期化方法を示しています。
igMap コントロールに必要なスクリプトおよびテーマの参照方法についての詳細は、 Ignite UI での JavaScript リソースの使用および Ignite UI のスタイルとテーマの設定をお読みください。
コード サンプル
<!doctype html> <html> <head> <title>Ignite UI igMap</title> <!-- jQuery Core --> <script src="js/jquery.js" type="text/javascript"></script> <!-- jQuery UI --> <script src="js/jquery-ui.js" type="text/javascript"></script> <!-- Infragistics Loader --> <script type="text/javascript" src="http://localhost/ig_ui/js/infragistics.loader.js"></script> <script type="text/javascript"> var data = [ { Name: "London", Country: "England", Latitude: 51.50, Longitude: 0.12 }, { Name: "Berlin", Country: "Germany", Latitude: 52.50, Longitude: 13.33 }, { Name: "Moscow", Country: "Russia", Latitude: 55.75, Longitude: 37.51 }, { Name: "Sydney", Country: "Australia", Latitude: -33.83, Longitude: 151.2 }, { Name: "Tokyo", Country: "Japan", Latitude: 35.6895, Longitude: 139.6917 }, { Name: "Shanghai", Country: "China", Latitude: 31.2244, Longitude: 121.4759 }, { Name: "New York", Country: "United States", Latitude: 40.7561, Longitude: -73.9870 }, { Name: "Sao Paulo", Country: "Brasil", Latitude: -23.5489, Longitude: -46.6388 }, { Name: "Los Angeles", Country: "United States", Latitude: 34.0522, Longitude: -118.2434 } ]; </script> <script id="cityTemplate" type="text/x-jquery-tmpl"> <table> <tr><td>${item.Name}, ${item.Country}</td></tr> <tr><td>Latitude: ${item.Latitude}</td></tr> <tr><td>Longitude: ${item.Longitude}</td></tr> </table> </script> <script type="text/javascript"> $.ig.loader({ scriptPath: "http://localhost/ig_ui/js/", cssPath: "http://localhost/ig_ui/css/", resources: "igMap,igOverviewPlusDetailPane" }); $(function () { $("#map").igMap({ overviewPlusDetailPaneVisibility: "visible", overviewPlusDetailPaneBackgroundImageUri: "http://www.igniteui.com/images/samples/maps/world.png", backgroundContent: { type: "openStreet" }, series: [{ type: "geographicSymbol", name: "worldCities", dataSource: data, latitudeMemberPath: "Latitude", longitudeMemberPath: "Longitude", markerType: "automatic", markerBrush: "olive", showTooltip: true, tooltipTemplate: "cityTemplate" }], windowResponse: "immediate", windowRect: { left: 0.20, top: 0.20, height: 0.45, width: 0.45 } }); }); </script> </head> <body> <div id="map"></div> </body> </html>
関連サンプル
- Bing Maps
- CloudMade Maps
- OpenStreet Maps
- JSON のバインド
- コレクションにバインド
- 地理高密度散布シリーズ
- 地理シンボル シリーズ
- 地理図形シリーズ
- 地理的ポリライン シリーズ
- 地理散布シリーズ
- 地理等高線シリーズ
- マップのツールチップ
- マーカー テンプレート
関連トピック
依存関係
-
autoMarginHeight
- タイプ:
- number
- デフォルト:
- 0
自動的にマップにマージンを追加するときに追加する自動高さを取得または設定します。
コード サンプル
//Initialize $(".selector").igMap({ autoMarginHeight : 100 }); //Get var height = $(".selector").igMap("option", "autoMarginHeight"); //Set $(".selector").igMap("option", "autoMarginHeight", 100);
-
autoMarginWidth
- タイプ:
- number
- デフォルト:
- 20
自動的にマップにマージンを追加するときに追加する自動幅を取得または設定します。
コード サンプル
//Initialize $(".selector").igMap({ autoMarginWidth : 200 }); //Get var width = $(".selector").igMap("option", "autoMarginWidth"); //Set $(".selector").igMap("option", "autoMarginWidth", 200);
-
backgroundContent
- タイプ:
- object
- デフォルト:
- {}
背景コンテンツ オブジェクト。
コード サンプル
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456" });
-
bingUrl
- タイプ:
- string
- デフォルト:
- "http://dev.virtualearth.net/REST/v1/Imagery/Metadata/"
bing maps url を取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456", bingUrl: "http://dev.virtualearth.net/rest/v1/imagery/metadata/" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.bingUrl; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456", bingUrl: "http://dev.virtualearth.net/rest/v1/imagery/metadata/" });
-
imagerySet
- タイプ:
- string
- デフォルト:
- "AerialWithLabels"
画像のタイプを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456", imagerySet: "aerial" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.imagerySet; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456", imagerySet: "aerial" });
-
key
- タイプ:
- string
- デフォルト:
- null
キーを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.key; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456" });
-
parameter
- タイプ:
- string
- デフォルト:
- null
パラメーターを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456", parameter: 2 } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.parameter; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456", parameter: 2 });
-
tilePath
- タイプ:
- string
- デフォルト:
- null
マップ タイル イメージの URI を取得または設定します。
Bing Maps では、bing イメージで生成されます。
Open Street Map では、このオプションはタイルにカスタム URL を使用できます。デフォルトは、'tile.openstreetmap.org/{Z}/{X}/{Y}.png'。プロトコル設定なしの場合は、'http://' または 'https://' がホスティング サイトのプロトコルに基づいて自動的に追加されます。{Z} - タイル ズームを表します。{X} - タイルの水平位置を表します。{Y} - タイルの垂直位置を表します。コード サンプル
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456", tilePath: "http://www.example.com/tiles/" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.tilePath; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456", tilePath: "http://www.example.com/tiles/" });
-
type
- タイプ:
- enumeration
- デフォルト:
- openStreet
マップの背景コンテンツのタイプ。
メンバー
- openStreet
- タイプ:string
- OpenStreetMap 地理的データを表示するために背景コンテンツを指定します。デフォルトとして設定します。
- cloudMade
- タイプ:string
- CloudMade 地理的データを表示するために背景コンテンツを指定します。
- bing
- タイプ:string
- BingMaps 地理的データを表示するために背景コンテンツを指定します。
コード サンプル
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.type; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456" });
-
circleMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップに円マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
circle のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ circleMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
crosshairPoint
- タイプ:
- object
- デフォルト:
- {}
十字線の位置 (ワールド座標値) を取得または設定します
十字線の X または Y のいずれかまたは両方を double.NaN に設定することができます。
その場合、該当する十字線は非表示になります。コード サンプル
// Initialization $(".selector").igMap({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igMap("option", "crosshairPoint"); // Set $(".selector").igMap("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
x
- タイプ:
- number
- デフォルト:
- NaN
x 座標
コード サンプル
// Initialization $(".selector").igMap({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igMap("option", "crosshairPoint"); var x = crosshairPoint.x; // Set $(".selector").igMap("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
y
- タイプ:
- number
- デフォルト:
- NaN
y 座標
コード サンプル
// Initialization $(".selector").igMap({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igMap("option", "crosshairPoint"); var y = crosshairPoint.y; // Set $(".selector").igMap("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
crosshairVisibility
- タイプ:
- enumeration
- デフォルト:
- collapsed
現在の Map の十字線の表示/非表示のオーバーライドを取得または設定します。
メンバー
- visible
- タイプ:string
- 十字線を表示します。
- collapsed
- タイプ:string
- 十字線を表示しません。
コード サンプル
// Initialization $(".selector").igMap({ crosshairVisibility: "collapsed" }); // Get var crosshairVisibility = $(".selector").igMap("option", "crosshairVisibility"); // Set $(".selector").igMap("option", "crosshairVisibility", "collapsed");
-
dataSource
- タイプ:
- object
- デフォルト:
- null
$.ig.DataSource が受け入れる有効なデータ ソースまたは $.ig.DataSource 自体のインスタンスが可能です。
コード サンプル
// Initialization var data = [ { Name: "London", Country: "England", Latitude: 51.50, Longitude: 0.12 }, { Name: "Berlin", Country: "Germany", Latitude: 52.50, Longitude: 13.33 }, { Name: "Moscow", Country: "Russia", Latitude: 55.75, Longitude: 37.51 }, { Name: "Sydney", Country: "Australia", Latitude: -33.83, Longitude: 151.2 } ]; $(".selector").igMap({ dataSource: data }); // Get var dataSource = $(".selector").igMap("option", "dataSource"); // Set $(".selector").igMap("option", "dataSource", data);
-
dataSourceType
- タイプ:
- string
- デフォルト:
- null
データ ソースのタイプ ("json" など) を明示的に設定します。$.ig.DataSource とそのタイプ プロパティのドキュメントを参照してください。
コード サンプル
// Initialization $(".selector").igMap({ dataSourceType: "array" }); // Get var dataSourceType = $(".selector").igMap("option", "dataSourceType"); // Set $(".selector").igMap("option", "dataSourceType", "array");
-
dataSourceUrl
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource からデータを要求するには、$.ig.DataSource により承諾されたリモート URL を指定します。
コード サンプル
// Initialization $(".selector").igMap({ dataSourceUrl: "http://www.example.com" }); // Get var dataSourceUrl = $(".selector").igMap("option", "dataSourceUrl"); // Set $(".selector").igMap("option", "dataSourceUrl", "http://www.example.com");
-
defaultInteraction
- タイプ:
- enumeration
- デフォルト:
- dragPan
DefaultInteraction プロパティを取得または設定します。デフォルトの操作状態により、マウス イベントに対するマップの応答が定義されます。
メンバー
- none
- タイプ:string
- ユーザー動作はマップの状態を変更しません。
- dragZoom
- タイプ:string
- ユーザー動作はマップをズームするために矩形のドラッグを開始します。
- dragPan
- タイプ:string
- マップのウインドウを移動するためにユーザー操作でパン操作を開始します。
コード サンプル
// Initialization $(".selector").igMap({ defaultInteraction: "dragZoom" }); // Get var defaultInteraction = $(".selector").igMap("option", "defaultInteraction"); // Set $(".selector").igMap("option", "defaultInteraction", "dragZoom");
-
diamondMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップにひし形マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
diamond のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ diamondMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
dragModifier
- タイプ:
- enumeration
- デフォルト:
- none
現在の Map の DragModifier プロパティを取得または設定します。
メンバー
- none
- タイプ:string
- 修飾キーが設定されません。
- alt
- タイプ:string
- 修飾キーは alt キーに設定されます。
- control
- タイプ:string
- 修飾キーは control キーに設定されます。
- shift
- タイプ:string
- 修飾キーは shift キーに設定されます。
コード サンプル
// Initialization $(".selector").igMap({ dragModifier: "control" }); // Get var dragModifier = $(".selector").igMap("option", "dragModifier"); // Set $(".selector").igMap("option", "dragModifier", "control");
-
height
- タイプ:
- enumeration
- デフォルト:
- null
マップの高さ。ピクセル、文字列 (px)、またはパーセンテージ (%) で数字として設定できます。
メンバー
- string
- ウィジェットの高さはピクセル (px) およびパーセント (%) で設定できます (%)。
- number
- ウィジェットの高さは数値として設定できます。
コード サンプル
// Initialization $(".selector").igMap({ height: 250 }); // Get var height = $(".selector").igMap("option", "height"); // Set $(".selector").igMap("option", "height", 250);
-
hexagonMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップに六角形マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
hexagon のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ hexagonMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
hexagramMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップに六線星形マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
hexagram のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ hexagramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
language
継承- タイプ:
- string
- デフォルト:
- "en"
ウィジェットのロケール言語設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igMap({ language: "ja" }); // Get var language = $(".selector").igMap("option", "language"); // Set $(".selector").igMap("option", "language", "ja");
-
locale
継承- タイプ:
- object
- デフォルト:
- null
ウィジェットのロケール設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igMap({ locale: {} }); // Get var locale = $(".selector").igMap("option", "locale"); // Set $(".selector").igMap("option", "locale", {});
-
overviewPlusDetailPaneBackgroundImageUri
- タイプ:
- string
- デフォルト:
- null
概要詳細ペインで使用する背景画像 uri。
コード サンプル
// Initialization $(".selector").igMap({ overviewPlusDetailPaneBackgroundImageUri: "Content/img/World.png" }); // Get var OPDImageUri = $(".selector").igMap("option", "overviewPlusDetailPaneBackgroundImageUri"); // Set $(".selector").igMap("option", "overviewPlusDetailPaneBackgroundImageUri", "Content/img/World.png");
-
panModifier
- タイプ:
- enumeration
- デフォルト:
- shift
現在の Map の PanModifier プロパティを取得または設定します。
メンバー
- none
- タイプ:string
- 修飾キーが設定されません。
- alt
- タイプ:string
- 修飾キーは alt キーに設定されます。
- control
- タイプ:string
- 修飾キーは control キーに設定されます。
- shift
- タイプ:string
- 修飾キーは shift キーに設定されます。
コード サンプル
// Initialization $(".selector").igMap({ panModifier: "control" }); // Get var panModifier = $(".selector").igMap("option", "panModifier"); // Set $(".selector").igMap("option", "panModifier", "control");
-
pentagonMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップに五角形マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
pentagon のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ pentagonMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
pentagramMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップに星形五角形マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
pentagram のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ pentagramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
plotAreaBackground
- タイプ:
- string
- デフォルト:
- null
現在の Map オブジェクトのプロット領域の背景として使用するブラシを取得または設定します。
コード サンプル
//Initialize $(".selector").igMap({ plotAreaBackground: "grey" }); //Get var plotAreaBackground = $(".selector").igMap("option", "plotAreaBackground"); //Set var plotAreaBackground = $(".selector").igMap("option", "plotAreaBackground", "grey");
-
preferHigherResolutionTiles
- タイプ:
- bool
- デフォルト:
- false
タイル ズーム時にシリーズ ビューアーがより高い解像度のタイルに適しているかどうかを設定します。これを true に設定すると、パフォーマンスは低下しますが描画品質が高まります。
コード サンプル
//Initialize $(".selector").igMap({ preferHigherResolutionTiles: true }); //Get var preferHighResTiles = $(".selector").igMap("option", "preferHigherResolutionTiles"); //Set $(".selector").igMap("option", "preferHigherResolutionTiles", true);
-
previewRect
- タイプ:
- object
- デフォルト:
- null
プレビュー矩形を設定または取得します。
プレビュー矩形は Rect.Empty に設定することができます。その場合、表示されているプレビューの
strokePath は非表示になります。
提供されたオブジェクトは、left、top、width および height と呼ばれる数値プロパティを持っている必要があります。コード サンプル
// Initialization $(".selector").igMap({ previewRect: { left: 0.3, top: 0.3, width: 0.5, height: 0.5 } }); // Get var previewRect = $(".selector").igMap("option", "previewRect"); // Set $(".selector").igMap("option", "previewRect", { left: 0.3, top: 0.3, width: 0.5, height: 0.5 });
-
pyramidMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップにピラミッド マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
pyramid のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ pyramidMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
regional
継承- タイプ:
- enumeration
- デフォルト:
- en-US
ウィジェットの領域設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igMap({ regional: "ja" }); // Get var regional = $(".selector").igMap("option", "regional"); // Set $(".selector").igMap("option", "regional", "ja");
-
responseDataKey
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource を参照してください。応答がラップされる場合に、データ レコードが保持されるプロパティの名前を指定します。
コード サンプル
//Initialize $(".selector").igMap({ responseDataKey: "Records" }); //Get var responseDataKey = $(".selector").igMap("option", "responseDataKey"); //Set var responseDataKey = $(".selector").igMap("option", "responseDataKey", "Records");
-
series
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- object
シリーズ オブジェクトの配列。
コード サンプル
//Initialization var data = [ { Name: "London", Country: "England", Latitude: 51.50, Longitude: 0.12 }, { Name: "Berlin", Country: "Germany", Latitude: 52.50, Longitude: 13.33 } ]; $("#map").igMap({ series: [{ type: "geographicSymbol", name: "worldCities", dataSource: data, latitudeMemberPath: "Latitude", longitudeMemberPath: "Longitude" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0]; //Set $(".selector").igMap("option", "series", [{ type: "geographicSymbol", name: "worldCities", latitudeMemberPath: "Latitude", longitudeMemberPath: "Longitude" }] );
-
angleMemberPath
- タイプ:
- number
- デフォルト:
- null
列の角を丸めるために使用される楕円の x 半径を取得または設定します。
コード サンプル
//Initialization $(".selector").igMap({ series: [{ angleMemberPath: "Index" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].angleMemberPath; //Set $(".selector").igMap("option", "series", [{ angleMemberPath: "Index" }]);
-
brush
- タイプ:
- string
- デフォルト:
- null
シリーズに使用するブラシを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ brush: "blue" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].brush; //Set $(".selector").igMap("option", "series", [{ brush: "blue" }]);
-
clipSeriesToBounds
- タイプ:
- bool
- デフォルト:
- null
シリーズを境界線でクリップするかどうかを設定または取得します。
これを true に設定するとパフォーマンスに影響を及ぼします。コード サンプル
//Initialization $("#map").igMap({ series: [{ clipSeriesToBounds: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].clipSeriesToBounds; //Set $(".selector").igMap("option", "series", [{ clipSeriesToBounds: true }]);
-
closeMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの終値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ closeMemberPath: "Close" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].closeMemberPath; //Set $(".selector").igMap("option", "series", [{ closeMemberPath: "Close" }]);
-
colorMemberPath
- タイプ:
- string
- デフォルト:
- null
ColorScale によって色に変換できる数値を含むを各データ項目のプロパティの名前。
コード サンプル
//Initialization $("#map").igMap({ series: [{ colorMemberPath: "Temperature" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].colorMemberPath; //Set $(".selector").igMap("option", "series", [{ colorMemberPath: "Temperature" }]);
-
colorScale
- タイプ:
- object
- デフォルト:
- null
シリーズ内のポイントの色の値を解決するために使用する ColorScale。
コード サンプル
//Initialization $("#map").igMap({ series: [{ name: "sampleSeries", type: "geographicScatterArea", colorScale: { type: "customPalette", interpolationMode: "interpolateRGB", palette: [ "#3300CC", "#4775FF" ] } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].colorScale; //Set $(".selector").igMap("option", "series", [{ name: "sampleSeries", colorScale: { type: "customPalette", interpolationMode: "interpolateRGB", palette: [ "#3300CC", "#4775FF" ] } }]);
-
databaseSource
- タイプ:
- string
- デフォルト:
- null
文字列、データベース ソース URI
コード サンプル
//Initialization $("#map").igMap({ series: [{ name: "sampleSeries", type: "geographicShape", databaseSource: "ShapeData.dbf" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].databaseSource; //Set $(".selector").igMap("option", "series", [{ name: "sampleSeries", databaseSource: "ShapeData.dbf" }]);
-
dataSource
- タイプ:
- object
- デフォルト:
- null
$.ig.DataSource が受け入れる有効なデータ ソースまたは $.ig.DataSource 自体のインスタンスが可能です。
コード サンプル
//Initialization $("#map").igMap({ series: [{ dataSource: data }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].dataSource; //Set $(".selector").igMap("option", "series", [{ dataSource: data }]);
-
dataSourceType
- タイプ:
- string
- デフォルト:
- null
データ ソースのタイプ ("json" など) を明示的に設定します。$.ig.DataSource とそのタイプ プロパティのドキュメントを参照してください。
コード サンプル
//Initialization $("#map").igMap({ series: [{ dataSourceType: "array" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].dataSourceType; //Set $(".selector").igMap("option", "series", [{ dataSourceType: "array" }]);
-
dataSourceUrl
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource からデータを要求するには、$.ig.DataSource により承諾されたリモート URL を指定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ dataSourceUrl: "http://www.example.com/map-data" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].dataSourceUrl; //Set $(".selector").igMap("option", "series", [{ dataSourceUrl: "http://www.example.com/map-data" }]);
-
discreteLegendItemTemplate
- タイプ:
- object
- デフォルト:
- null
DiscreteLegendItemTemplate プロパティを取得または設定します。
凡例項目コントロール コンテンツは、DiscreteLegendItemTemplate に従って、シリーズ オブジェクト自身によって
オンデマンドで作成されます。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialization $("#map").igMap({ series: [{ discreteLegendItemTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
fillMemberPath
- タイプ:
- string
- デフォルト:
- null
FillScale を使用するブラシに変換される数値を含む、データ ソース項目のプロパティ名。
コード サンプル
//Initialization $(".selector").igMap({ series: [{ name: "sampleSeries", type: "geographicContourLine", fillMemberPath: "Temperature" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].fillMemberPath; //Set $(".selector").igMap("option", "series", [{ name: "series1", fillMemberPath: "Temperature" }]);
-
fillScale
- タイプ:
- object
- デフォルト:
- null
FillMemberPath にある値に基づいて各 Shape のブラシを決定するときに使用する ValueBrushScale。
コード サンプル
//Initialization $(".selector").igMap({ series: [{ name: "sampleSeries", type: "geographicContourLine", fillScale: { type: "value", brushes: ["#3300CC", "#4775FF", "#0099CC", "#00CC99", "#33CC00", "#99CC00", "#CC9900", "#FFC20A", "#CC3300"] } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].fillScale; //Set $(".selector").igMap("option", "series", [{ name: "series1", fillScale: { type: "value", brushes: ["#3300CC", "#4775FF", "#0099CC", "#00CC99", "#33CC00", "#99CC00", "#CC9900", "#FFC20A", "#CC3300"] } }]);
-
heatMaximum
- タイプ:
- number
- デフォルト:
- 50
最高熱色にマップする値を取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ heatMaximum: 100 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].heatMaximum; //Set $(".selector").igMap("option", "series", [{ heatMaximum: 100 }]);
-
heatMinimum
- タイプ:
- number
- デフォルト:
- 0
最低熱色にマップする密度値を取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ heatMinimum: 5 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].heatMinimum; //Set $(".selector").igMap("option", "series", [{ heatMinimum: 5 }]);
-
highMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ highMemberPath: "High" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].highMemberPath; //Set $(".selector").igMap("option", "series", [{ highMemberPath: "High" }]);
-
ignoreFirst
- タイプ:
- number
- デフォルト:
- 0
インジケータの開始を非表示にするための値の数を取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ ignoreFirst: 5 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].ignoreFirst; //Set $(".selector").igMap("option", "series", [{ ignoreFirst: 5 }]);
-
labelMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトのラベル マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ labelMemberPath: "Name" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].labelMemberPath; //Set $(".selector").igMap("option", "series", [{ labelMemberPath: "Name" }]);
-
latitudeMemberPath
- タイプ:
- string
- デフォルト:
- null
シンボルの緯度座標を含む、データ ソース項目のプロパティ名。
コード サンプル
//Initialization $("#map").igMap({ series: [{ latitudeMemberPath: "Latitude" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].latitudeMemberPath; //Set $(".selector").igMap("option", "series", [{ latitudeMemberPath: "Latitude" }]);
-
legendItemBadgeTemplate
- タイプ:
- object
- デフォルト:
- null
LegendItemBadgeTemplate プロパティを取得または設定します。
凡例項目バッジは、LegendItemBadgeTemplate に従って、シリーズ オブジェクト自身によってオンデマンドで作成されます。
オンデマンドで作成されます。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。
これらはユーザー指定のカスタム描画を処理するために呼び出される関数です。
measure は以下のようなオブジェクトを渡されます:
{
context: [特定のテンプレートのシナリオに基づいて DOM 要素または CanvasContext2D のいずれか],
width: [値が存在する場合、使用可能な幅を指定します。ユーザーはコンテンツのために希望の幅を設定できます],
height: [値が存在する場合、使用可能な高さを指定します。ユーザーはコンテンツのために希望の高さを設定できます],
isConstant: [希望の幅と高さが常にこのテンプレートに対して同じである場合には、ユーザーは True に設定する必要があります],
data: [存在する場合、このテンプレートのコンテキストでを表します]
}
render は以下のようなオブジェクトを渡されます:
{
context: [特定のテンプレートのシナリオに基づいて DOM 要素または CanvasContext2D のいずれか],
xPosition: [存在する場合、コンテンツを描画する x 位置を指定します],
yPosition: [存在する場合、コンテンツを描画する y 位置を指定します],
availableWidth: [存在する場合、コンテンツを描画する使用可能な幅を指定します],
availableHeight: [存在する場合、コンテンツを描画する使用可能な高さを指定します],
data: [存在する場合、このコンテキストのためにコンテキストにあるデータを指定します],
isHitTestRender: [true の場合、これがヒットテストのために特別な描画パスであることを指定します。この場合データからのブラシを使用する必要があります]
}。コード サンプル
//Initialization $("#map").igMap({ series: [{ legendItemBadgeTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
legendItemTemplate
- タイプ:
- object
- デフォルト:
- null
LegendItemTemplate プロパティを取得または設定します。
凡例項目コントロール コンテンツは、LegendItemTemplate に従って、シリーズ オブジェクト自身によってオンデマンドで作成されます。
オンデマンドで作成されます。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialization $("#map").igMap({ series: [{ legendItemTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
longitudeMemberPath
- タイプ:
- string
- デフォルト:
- null
シンボルの経度座標を含む、データ ソース項目のプロパティ名。
コード サンプル
//Initialization $("#map").igMap({ series: [{ longitudeMemberPath: "Longitude" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].longitudeMemberPath; //Set $(".selector").igMap("option", "series", [{ longitudeMemberPath: "Longitude" }]);
-
longPeriod
- タイプ:
- number
- デフォルト:
- 0
現在の AbsoluteVolumeOscillatorIndicator オブジェクトの短い移動平均期間を取得または設定します。
長い AVO 期間の一般的な値かつ初期値は 30 です。コード サンプル
//Initialization $("#map").igMap({ series: [{ longPeriod: 50 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].longPeriod; //Set $(".selector").igMap("option", "series", [{ longPeriod: 50 }]);
-
lowMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ lowMemberPath: "Low" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].lowMemberPath; //Set $(".selector").igMap("option", "series", [{ lowMemberPath: "Low" }]);
-
markerBrush
- タイプ:
- string
- デフォルト:
- null
現在の系列オブジェクトのマーカーの内部をペイントする方法を指定するブラシを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ markerBrush: "blue" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].markerBrush; //Set $(".selector").igMap("option", "series", [{ markerBrush: "blue" }]);
-
markerCollisionAvoidance
- タイプ:
- enumeration
- デフォルト:
- none
MarkerCollisionAvoidance を取得または設定します。
メンバー
- none
- タイプ:string
- 競合回避がありません。
- omit
- タイプ:string
- 競合するマーカーを表示しません。
- fade
- タイプ:string
- 競合するマーカーの不透明度をフォードします。
- omitAndShift
- タイプ:string
- 競合するマーカーを移動するか、表示しません。
コード サンプル
//Initialization $("#map").igMap({ series: [{ markerCollisionAvoidance: "fadeAndShift" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].markerCollisionAvoidance; //Set $(".selector").igMap("option", "series", [{ markerCollisionAvoidance: "fadeAndShift" }]);
-
markerOutline
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトのマーカーのアウトラインを塗りつぶす方法を指定するブラシを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ markerOutline: "black" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].markerOutline; //Set $(".selector").igMap("option", "series", [{ markerOutline: "black" }]);
-
markerTemplate
- タイプ:
- object
- デフォルト:
- null
現在のシリーズ オブジェクトの MarkerTemplate を取得または設定します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。
これらはユーザー指定のカスタム描画を処理するために呼び出される関数です。
measure は以下のようなオブジェクトを渡されます:
{
context: [特定のテンプレートのシナリオに基づいて DOM 要素または CanvasContext2D のいずれか],
width: [値が存在する場合、使用可能な幅を指定します。ユーザーはコンテンツのために希望の幅を設定できます],
height: [値が存在する場合、使用可能な高さを指定します。ユーザーはコンテンツのために希望の高さを設定できます],
isConstant: [希望の幅と高さが常にこのテンプレートに対して同じである場合には、ユーザーは True に設定する必要があります],
data: [存在する場合、このテンプレートのコンテキストでを表します]
}
render は以下のようなオブジェクトを渡されます:
{
context: [特定のテンプレートのシナリオに基づいて DOM 要素または CanvasContext2D のいずれか],
xPosition: [存在する場合、コンテンツを描画する x 位置を指定します],
yPosition: [存在する場合、コンテンツを描画する y 位置を指定します],
availableWidth: [存在する場合、コンテンツを描画する使用可能な幅を指定します],
availableHeight: [存在する場合、コンテンツを描画する使用可能な高さを指定します],
data: [存在する場合、このコンテキストのためにコンテキストにあるデータを指定します],
isHitTestRender: [true の場合、これがヒットテストのために特別な描画パスであることを指定します。この場合データからのブラシを使用する必要があります]
}。コード サンプル
//Initialization $("#map").igMap({ series: [{ markerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
markerType
- タイプ:
- enumeration
- デフォルト:
- none
現在のシリーズ オブジェクトにマーカー タイプを取得または設定します。MarkerTemplate プロパティが設定された場合、MarkerType プロパティの設定は無視されます。
メンバー
- unset
- タイプ:string
- none
- タイプ:string
- automatic
- タイプ:string
- circle
- タイプ:string
- triangle
- タイプ:string
- pyramid
- タイプ:string
- square
- タイプ:string
- diamond
- タイプ:string
- pentagon
- タイプ:string
- hexagon
- タイプ:string
- tetragram
- タイプ:string
- pentagram
- タイプ:string
- hexagram
- タイプ:string
コード サンプル
//Initialization $("#map").igMap({ series: [{ markerType: "square" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].markerType; //Set $(".selector").igMap("option", "series", [{ markerType: "square" }]);
-
maximumMarkers
- タイプ:
- number
- デフォルト:
- 400
現在の系列によって表示される markerItems の最大数を取得または設定します。
指定した数を超える markerItems が表示されている場合、シリーズは代表的なセットを
自動的に選択します。コード サンプル
//Initialization $("#map").igMap({ series: [{ maximumMarkers: 150 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].maximumMarkers; //Set $(".selector").igMap("option", "series", [{ maximumMarkers: 150 }]);
-
mouseOverEnabled
- タイプ:
- bool
- デフォルト:
- false
マップがマウス移動イベントに反応するかどうかを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ mouseOverEnabled: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].mouseOverEnabled; //Set $(".selector").igMap("option", "series", [{ mouseOverEnabled: true }]);
-
name
- タイプ:
- string
- デフォルト:
- null
シリーズの一意の識別子。
コード サンプル
//Initialization $("#map").igMap({ series: [{ name: "seriesName" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].name; //Set $(".selector").igMap("option", "series", [{ name: "seriesName" }]);
-
negativeBrush
- タイプ:
- string
- デフォルト:
- null
系列の負の領域を描画するブラシを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ negativeBrush: "red" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].negativeBrush; //Set $(".selector").igMap("option", "series", [{ negativeBrush: "red" }]);
-
openMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの値マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ openMemberPath: "Open" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].openMemberPath; //Set $(".selector").igMap("option", "series", [{ openMemberPath: "Open" }]);
-
outline
- タイプ:
- string
- デフォルト:
- null
シリーズのアウトラインを描画するブラシを取得または設定します。
LineSeries など、一部の Series タイプでは、輪郭が表示されません。コード サンプル
//Initialization $("#map").igMap({ series: [{ outline: "black" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].outline; //Set $(".selector").igMap("option", "series", [{ outline: "black" }]);
-
period
- タイプ:
- number
- デフォルト:
- 0
現在の AverageDirectionalIndexIndicator オブジェクトの移動平均期間を取得または設定します。
AverageDirectionalIndexIndicator 期間の一般的な値かつ初期値は 14 です。コード サンプル
//Initialization $("#map").igMap({ series: [{ period: 45 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].period; //Set $(".selector").igMap("option", "series", [{ period: 45 }]);
-
progressiveLoad
- タイプ:
- bool
- デフォルト:
- true
マップへデータを徐々に読み込むかどうかを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ progressiveLoad: false }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].progressiveLoad; //Set $(".selector").igMap("option", "series", [{ progressiveLoad: false }]);
-
radiusMemberPath
- タイプ:
- string
- デフォルト:
- null
現在の系列オブジェクトの半径マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ radiusMemberPath: "Sales" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].radiusMemberPath; //Set $(".selector").igMap("option", "series", [{ radiusMemberPath: "Sales" }]);
-
radiusScale
- タイプ:
- object
- デフォルト:
- null
バブルの半径サイズ スケールを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ radiusScale: { minimumValue: 5, maximumValue: 25 } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].radiusScale; //Set $(".selector").igMap("option", "series", [{ radiusScale: { minimumValue: 5, maximumValue: 25 } }]);
-
remove
- タイプ:
- bool
- デフォルト:
- false
マップから依存のシリーズを名前によって削除するには、true に設定します。
コード サンプル
//Set $(".selector").igMap("option", "series", [{ name: "series1", remove: true }]);
-
resolution
- タイプ:
- number
- デフォルト:
- 1
現在のシリーズ オブジェクトのレンダリング解像度を取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ resolution: 300 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].resolution; //Set $(".selector").igMap("option", "series", [{ resolution: true }]);
-
responseDataKey
- タイプ:
- string
- デフォルト:
- null
$.ig.DataSource を参照してください。応答がラップされる場合に、データ レコードが保持されるプロパティの名前を指定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ responseDataKey: "Results" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].responseDataKey; //Set $(".selector").igMap("option", "series", [{ responseDataKey: "Results" }]);
-
shapeDataSource
- タイプ:
- string
- デフォルト:
- null
三通貨換算されたファイル ソース URI または $.ig.ShapeDataSource のインスタンス。
コード サンプル
//Initialization $("#map").igMap({ series: [{ shapeDataSource: "ShapeData.shp" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeDataSource; //Set $(".selector").igMap("option", "series", [{ shapeDataSource: "ShapeData.shp" }]);
-
shapeFilterResolution
- タイプ:
- number
- デフォルト:
- 2
シリーズの図形をフィルターする解像度を取得または設定します。
たとえば、shapeFilterResolution を 3 に設定すると、 3 X 3 ピクセルより小さい境界四角形の要素がフィルターされます。コード サンプル
//Initialization $("#map").igMap({ series: [{ shapeFilterResolution: 50 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeFilterResolution; //Set $(".selector").igMap("option", "series", [{ shapeFilterResolution: 50 }]);
-
shapeMemberPath
- タイプ:
- string
- デフォルト:
- null
各形状に対して、多角形に変換されるポイントのリストを含むデータ ソース項目のプロパティ名。
シェイプ ファイルに関する詳細説明に一致するように、ポイントの各リストを Point の IEnumerable の IEnumerable、言い換えれば、ポイント リストのリストとして定義します。コード サンプル
//Initialization $("#map").igMap({ series: [{ shapeMemberPath: "points" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeMemberPath; //Set $(".selector").igMap("option", "series", [{ shapeMemberPath: "points" }]);
-
shapeStyle
- タイプ:
- object
- デフォルト:
- null
系列のすべての図形に適用するデフォルト スタイル。
コード サンプル
//Initialization $("#map").igMap({ series: [{ shapeStyle: { fill: "blue", stroke: "black" } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeStyle; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", shapeStyle: { fill: "blue", stroke: "black" } }]);
-
shapeStyleSelector
- タイプ:
- object
- デフォルト:
- null
各図形のスタイルを選択するために使用される StyleSelector。
コード サンプル
//Initialization $("#map").igMap({ series: [{ shapeStyleSelector: { selectStyle = function (shapeData, o) { var shapeID = shapeData.fields.item("ID"); return { fill: "blue", stroke: "black" }; } } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeStyleSelector; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", shapeStyleSelector: { selectStyle = function (shapeData, o) { var shapeID = shapeData.fields.item("ID"); return { fill: "blue", stroke: "black" }; } } }]);
-
shortPeriod
- タイプ:
- number
- デフォルト:
- 0
現在の AbsoluteVolumeOscillatorIndicator オブジェクトの短い移動平均期間を取得または設定します。
短い AVO 期間の一般的な値かつ初期値は 10 です。コード サンプル
//Initialization $("#map").igMap({ series: [{ shortPeriod: 15 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shortPeriod; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", shortPeriod: 15 }]);
-
showTooltip
- タイプ:
- bool
- デフォルト:
- false
マップがツールチップを描画するかどうか。
コード サンプル
//Initialization $("#map").igMap({ series: [{ showTooltip: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].showTooltip; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", showTooltip: true }]);
-
splineType
- タイプ:
- enumeration
- デフォルト:
- natural
描画されるスプラインのタイプを取得または設定します。
メンバー
- natural
- タイプ:string
- 自然スプライン計算数式を使用することによってスプラインを計算します。
- clamped
- タイプ:string
- 固定スプライン計算数式を使用することによってスプラインを計算します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ splineType: "clamped" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].splineType; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", splineType: "clamped" }]);
-
stiffness
- タイプ:
- number
- デフォルト:
- 0.5
Stiffness プロパティを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ stiffness: 0.8 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].stiffness; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", stiffness: 0.8 }]);
-
thickness
- タイプ:
- number
- デフォルト:
- 0
現在のシリーズ オブジェクトの線の太さの幅を取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ thickness: 2 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].thickness; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", thickness: 2 }]);
-
title
- タイプ:
- string
- デフォルト:
- null
Title プロパティを取得または設定します。
凡例項目コントロールは、Title に従って、シリーズ オブジェクト自身によってオンデマンドで作成されます。
オンデマンドで作成されます。コード サンプル
//Initialization $("#map").igMap({ series: [{ title: "Sales" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].title; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", title: "Sales" }]);
-
transitionDuration
- タイプ:
- number
- デフォルト:
- 0
現在の系列のモーフィングの期間を取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ transitionDuration: 500 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].transitionDuration; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", transitionDuration: 500 }]);
-
trendLineBrush
- タイプ:
- string
- デフォルト:
- null
トレンドラインを描画するブラシを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ trendLineBrush: "green" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLineBrush; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLineBrush: "green" }]);
-
trendLinePeriod
- タイプ:
- number
- デフォルト:
- 7
現在の散布シリーズ オブジェクトの移動平均期間を取得または設定します。
トレンド折れ線の範囲の標準/初期値は 7 です。コード サンプル
//Initialization $("#map").igMap({ series: [{ trendLinePeriod: 20 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLinePeriod; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLinePeriod: 20 }]);
-
trendLineThickness
- タイプ:
- number
- デフォルト:
- 1.5
現在の散布シリーズ オブジェクトのトレンド ラインの太さを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ trendLineThickness: 3 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLineThickness; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLineThickness: 3 }]);
-
trendLineType
- タイプ:
- enumeration
- デフォルト:
- none
現在の散布シリーズのトレンド タイプを取得または設定します。
メンバー
- none
- タイプ:string
- トレンド折れ線を表示しません。
- linearFit
- タイプ:string
- リニア フィット。
- quadraticFit
- タイプ:string
- 二次多項式フィット。
- cubicFit
- タイプ:string
- 三次多項式フィット。
- quarticFit
- タイプ:string
- 四次多項式フィット。
- quinticFit
- タイプ:string
- 五次多項式フィット。
- logarithmicFit
- タイプ:string
- 対数フィット。
- exponentialFit
- タイプ:string
- 指数フィット。
- powerLawFit
- タイプ:string
- べき乗フィット。
- simpleAverage
- タイプ:string
- 単純移動平均。
- exponentialAverage
- タイプ:string
- 指数移動平均。
- modifiedAverage
- タイプ:string
- 変更された移動平均。
- cumulativeAverage
- タイプ:string
- 累積移動平均。
- weightedAverage
- タイプ:string
- 加重移動平均。
コード サンプル
//Initialization $("#map").igMap({ series: [{ trendLineType: "logarithmicFit" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLineType; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLineType: "logarithmicFit" }]);
-
trendLineZIndex
- タイプ:
- number
- デフォルト:
- 1001
近似曲線の z インデックスを取得または設定します。1000 より大きい値を指定すると、トレンド折れ線が系列データの前に描画されます。
コード サンプル
//Initialization $("#map").igMap({ series: [{ trendLineZIndex: 500 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLineZIndex; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLineZIndex: 500 }]);
-
trianglesSource
- タイプ:
- object
- デフォルト:
- null
三角形分割データのソース。
このプロパティはオプションです。これが null として残された場合、三角形分割はデータ ソースの項目に基づいて作成されます。三角形分割は要求の厳しい操作であるため、特に多数のデータ項目が存在する場合に TriangulationSource を指定するとランタイムの実行が改善されます。コード サンプル
var data = [ { longitude: 0, latitude: 0, value: 1 }, { longitude: 50, latitude: 0, value: 2 }, { longitude: 50, latitude: 50, value: 3 }, { longitude: 0, latitude: 50, value: 1 } ]; // Initialization $("#map").igMap({ series: [{ name: "series1", type: "geographicScatterArea", latitudeMemberPath: "latitude", longitudeMemberPath: "longitude", colorMemberPath: "value", colorScale: { minimumValue: 1, interpolationMode: "select", palette: ["darkgreen", "green", 'limegreen', 'lightgreen'] }, trianglesSource: data }] }); // Get var series = $(".selector").igMap("option", "series"); series[0].trianglesSource; // Set $(".selector").igMap("option", "series", [{ name: "series1", type: "geographicScatterArea", latitudeMemberPath: "latitude", longitudeMemberPath: "longitude", colorMemberPath: "value", colorScale: { minimumValue: 1, interpolationMode: "select", palette: ["darkgreen", "green", 'limegreen', 'lightgreen'] }, trianglesSource: data }]);
-
triangleVertexMemberPath1
- タイプ:
- string
- デフォルト:
- null
各三角形に対してデータ ソースの最初の頂点のインデックスを含む、TrianglesSource 項目のプロパティ名。
コード サンプル
//Initialization $("#map").igMap({ series: [{ triangleVertexMemberPath1: "v1" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].triangleVertexMemberPath1; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", triangleVertexMemberPath1: "v1" }]);
-
triangleVertexMemberPath2
- タイプ:
- string
- デフォルト:
- null
各三角形に対してデータ ソースの 2 番目の頂点のインデックスを含む、TrianglesSource 項目のプロパティ名。
コード サンプル
//Initialization $("#map").igMap({ series: [{ triangleVertexMemberPath2: "v2" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].triangleVertexMemberPath2; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", triangleVertexMemberPath2: "v2" }]);
-
triangleVertexMemberPath3
- タイプ:
- string
- デフォルト:
- null
各三角形に対してデータ ソースの 3 番目の頂点のインデックスを含む、TrianglesSource 項目のプロパティ名。
コード サンプル
//Initialization $("#map").igMap({ series: [{ triangleVertexMemberPath3: "v3" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].triangleVertexMemberPath3; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", triangleVertexMemberPath3: "v3" }]);
-
triangulationDataSource
- タイプ:
- string
- デフォルト:
- null
三通貨換算されたファイル ソース URI または $.ig.TriangulationDataSource のインスタンス。
コード サンプル
//Initialization $("#map").igMap({ series: [{ triangulationDataSource: "Triangulation.itf" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].triangulationDataSource; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", triangulationDataSource: "Triangulation.itf" }]);
-
type
- タイプ:
- enumeration
- デフォルト:
- null
シリーズのタイプ。
メンバー
- geographicSymbolSeries
- タイプ:string
- 地理的シンボル シリーズとしてシリーズを指定します。
- geographicPolyLine
- タイプ:string
- 地理的ポリライン シリーズとしてシリーズを指定します。
- geographicScatterArea
- タイプ:string
- 地理的スキャッター エリア シリーズとしてシリーズを指定します。
- geographicShape
- タイプ:string
- 地理的図形シリーズとしてシリーズを指定します。
- geographicContourLine
- タイプ:string
- 地理的等高線シリーズとしてシリーズを指定します。
- geographicHighDensityScatter
- タイプ:string
- 地理的高密度散布シリーズとしてシリーズを指定します。
- geographicProportionalSymbol
- タイプ:string
- 地理的比例記号シリーズとしてシリーズを指定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ type: "geographicPolyLine" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].type; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", type: "geographicPolyLine" }]);
-
unknownValuePlotting
- タイプ:
- enumeration
- デフォルト:
- dontPlot
不明な値をマップでプロットする方法を決定します。null および Double.NaN は不明な値の例です。
メンバー
- linearInterpolate
- タイプ:string
- 線形補間を使用すると、不明な値を囲む定義した値の中にプロットします。
- dontPlot
- タイプ:string
- 不明な値はマップにプロットしません。
コード サンプル
//Initialization $("#map").igMap({ series: [{ unknownValuePlotting: "linearInterpolate" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].unknownValuePlotting; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", unknownValuePlotting: "linearInterpolate" }]);
-
useBruteForce
- タイプ:
- bool
- デフォルト:
- false
ブルート フォース モードを使用するかどうかを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ useBruteForce: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].useBruteForce; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", useBruteForce: true }]);
-
useCartesianInterpolation
- タイプ:
- bool
- デフォルト:
- true
Archimedian の代わりにデカルト補間を使用するかどうかを取得または設定します
スパイラル ベース補間。コード サンプル
//Initialization $("#map").igMap({ series: [{ useCartesianInterpolation: false }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].useCartesianInterpolation; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", useCartesianInterpolation: false }]);
-
useSquareCutoffStyle
- タイプ:
- bool
- デフォルト:
- false
描画トラバーサルを中止する際に、結合領域の図形の代わりに四角を使用するかどうかを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ useSquareCutoffStyle: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].useSquareCutoffStyle; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", useSquareCutoffStyle: true }]);
-
valueMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズの値を提供する項目パスを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ valueMemberPath: "Population" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].valueMemberPath; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", valueMemberPath: "Population" }]);
-
valueResolver
- タイプ:
- object
- デフォルト:
- null
等高線の数値を決定するために使用される ContourValueResolver を取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: { valueResolver: { type: "linear", valueCount: 300 } } }); //Get var series = $(".selector").igMap("option", "series"); series.valueResolver; //Set $(".selector").igMap("option", "series", { valueResolver: { type: "linear", valueCount: 300 } });
-
visibleFromScale
- タイプ:
- number
- デフォルト:
- 0
このシリーズが表示される最小スケール。
このプロパティのデフォルト値は 1.0 で、シリーズが常に表示されることを示します。VisibleFromScale の設定が 0.0 の場合、シリーズは決して表示されません。VisibleFromScale の設定が 0.5 の場合、マップが少なくとも 200% まで拡大表示されている限りシリーズは表示されます。コード サンプル
//Initialization $("#map").igMap({ series: [{ visibleFromScale: 0.5 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].visibleFromScale; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", visibleFromScale: 0.5 }]);
-
volumeMemberPath
- タイプ:
- string
- デフォルト:
- null
現在のシリーズ オブジェクトの出来高マッピング プロパティを取得または設定します。
コード サンプル
//Initialization $("#map").igMap({ series: [{ volumeMemberPath: Sales }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].volumeMemberPath; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", volumeMemberPath: Sales }]);
-
squareMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップに四角マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
square のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ squareMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
tetragramMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップに星形四角形マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
tetragram のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ tetragramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
theme
- タイプ:
- string
- デフォルト:
- "c"
このウィジェットをスタイルするために使用される見本。
コード サンプル
//Initialize $(".selector").igMap({ theme: "metro" }); //Get var theme = $(".selector").igMap("option", "theme");
-
triangleMarkerTemplate
- タイプ:
- object
- デフォルト:
- null
マップに三角形マーカーを使用するためのテンプレートを取得または設定します。
マーカー タイプが
triangle のシリーズで使用されるマーカー テンプレートを定義します。
提供されるオブジェクトには、描画とオプションでメジャーと呼ばれるプロパティがあります。option: legendItemBadgeTemplate の定義を参照してください。コード サンプル
//Initialize $(".selector").igMap({ triangleMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
useTiledZooming
- タイプ:
- bool
- デフォルト:
- false
シリーズ ビューアーがデフォルト ライブ コンテンツではなくキャッシュ タイルを使用するどうかを設定します。
コード サンプル
//Initialize $(".selector").igMap({ useTiledZooming: true }); //Get var useTiledZooming = $(".selector").igMap("option", "useTiledZooming"); //Set $(".selector").igMap("option", "useTiledZooming", true);
-
width
- タイプ:
- enumeration
- デフォルト:
- null
マップの幅。ピクセル、文字列 (px)、またはパーセンテージ (%) で数字として設定できます。
メンバー
- string
- ウィジェットの幅をピクセル (px) またはパーセンテージ (%) に設定できます。
- number
- ウィジェット幅は数値として設定できます。
コード サンプル
// Initialization $(".selector").igMap({ width: 250 }); // Get var width = $(".selector").igMap("option", "width"); // Set $(".selector").igMap("option", "width", 250);
-
windowPositionHorizontal
- タイプ:
- number
- デフォルト:
- 0
水平スクロールの位置を決定する 0 ~ 1 の数。
このプロパティは WindowRect プロパティの X 位置へのショートカットと同じです。コード サンプル
// Initialization $(".selector").igMap({ windowPositionHorizontal: 0.25 }); // Get var windowPositionHorizontal = $(".selector").igMap("option", "windowPositionHorizontal"); // Set $(".selector").igMap("option", "windowPositionHorizontal", 0.25);
-
windowPositionVertical
- タイプ:
- number
- デフォルト:
- 0
垂直スクロールの位置を決定する 0 ~ 1 の数。
このプロパティは WindowRect プロパティの Y 位置へのショートカットと同じです。コード サンプル
// Initialization $(".selector").igMap({ windowPositionVertical: 0.25 }); // Get var windowPositionVertical = $(".selector").igMap("option", "windowPositionVertical"); // Set $(".selector").igMap("option", "windowPositionVertical", 0.25);
-
windowRect
- タイプ:
- object
- デフォルト:
- null
現在表示されているマップ部分を表す矩形。
X = 0、Y = 0、Height および Width が 1 に設定される矩形の場合、プロット可能な領域はすべて表示されていることを意味します。Height および Width は .5 に設定される場合、表示は半分ズームしています。
提供されたオブジェクトは、left、top、width および height と呼ばれる数値プロパティを持っている必要があります。コード サンプル
// Initialization $(".selector").igMap({ windowRect: { left: 0, top: 0, width: 0.5, height: 0.5 } }); // Get var windowRect = $(".selector").igMap("option", "windowRect"); // Set $(".selector").igMap("option", "windowRect", { left: 0, top: 0, width: 0.5, height: 0.5 });
-
windowRectMinWidth
- タイプ:
- number
- デフォルト:
- 0
ウィンドウ矩形が固定される前に到達できる最低幅を設定または取得します。
ビューアーにさらにズームできるようにする場合はこの値を減らします。
この値が低すぎると、浮動小数点演算が正確でなくなるため、グラフィックが破損する場合があります。コード サンプル
// Initialization $(".selector").igMap({ windowRectMinWidth: 0.5 }); // Get var windowRectMinWidth = $(".selector").igMap("option", "windowRectMinWidth"); // Set $(".selector").igMap("option", "windowRectMinWidth", 0.5);
-
windowResponse
- タイプ:
- enumeration
- デフォルト:
- null
ユーザー パンニングおよびズーミングに応答します。ユーザー操作が発生しているときにビューを直ちに更新、あるいはユーザー操作が完了した後まで遅延するかどうか。ユーザー操作は、パンニングやズームを発生させるマウスドラッグなどの操作です。
メンバー
- deferred
- タイプ:string
- ユーザー アクションが完了してからビューの更新を開始します。
- immediate
- タイプ:string
- ユーザー アクションの発生と同時にビューを更新します。
コード サンプル
// Initialization $(".selector").igMap({ windowResponse: "immediate" }); // Get var windowResponse = $(".selector").igMap("option", "windowResponse"); // Set $(".selector").igMap("option", "windowResponse", "immediate");
-
windowScale
- タイプ:
- number
- デフォルト:
- 1
現在のマップのズーム スケールを取得または設定します。
コード サンプル
// Initialization $(".selector").igMap({ windowScale: "0.5" }); // Get var windowScale = $(".selector").igMap("option", "windowScale"); // Set $(".selector").igMap("option", "windowScale", "0.5");
-
zoomable
- タイプ:
- bool
- デフォルト:
- true
現在のマップのズーム可否を取得または設定します。
コード サンプル
// Initialization $(".selector").igMap({ zoomable: "0.5" }); // Get var zoomable = $(".selector").igMap("option", "zoomable"); // Set $(".selector").igMap("option", "zoomable", "0.5");
-
zoomTileCacheSize
- タイプ:
- number
- デフォルト:
- 30
シリーズ ビューアーがタイル ズーム モードでキャッシュするズーム タイルの最大数を設定します。
コード サンプル
// Initialization $(".selector").igMap({ zoomTileCacheSize: "15" }); // Get var zoomTileCacheSize = $(".selector").igMap("option", "zoomTileCacheSize"); // Set $(".selector").igMap("option", "zoomTileCacheSize", "15");
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
browserNotSupported
- キャンセル可能:
- false
HTML5 非互換のブラウザでコントロールを表示したときに発生するイベント。
コード サンプル
// Delegate $(document).delegate(".selector", "igmapbrowsernotsupported", function (evt, ui) { }); // Initialize $(".selector").igMap({ browserNotSupported: function (evt, ui) { } });
-
gridAreaRectChanged
- キャンセル可能:
- false
現在の Map のグリッド領域の矩形が変わった直後に発生します。
マップをサイズ変更すると、グリッドの領域を変更する可能性があります。
関数は引数 evt および ui を受け取ります。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
新しい高さ値を取得するには ui.newHeight を使用します。
新しい左値を取得するには ui.newLeft を使用します。
新しい上値を取得するには ui.newTop を使用します。
新しい幅値を取得するには ui.newWidth を使用します。
以前の高さ値を取得するには ui.oldHeight を使用します。
以前の左値を取得するには ui.oldLeft を使用します。
以前の上値を取得するには ui.oldTop を使用します。
以前の幅値を取得するには ui.oldWidth を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmapgridarearectchanged", function (evt, ui) { // Get reference to map object. ui.map; // Get new height value. ui.newHeight; // Get new left value. ui.newLeft; // Get new top value. ui.newTop; // Get new top value. ui.newWidth; // Get old height value. ui.oldHeight; // Get old left value. ui.oldLeft; // Get old top value. ui.oldTop; // Get old top value. ui.oldWidth; }); // Initialize $(".selector").igMap({ gridAreaRectChanged: function (evt, ui) { } });
-
refreshCompleted
- キャンセル可能:
- false
マップの更新処理が完了すると発生します。
関数は引数 evt および ui を受け取ります。
マップ オブジェクトへの参照を取得するには ui.map を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmaprefreshcompleted", function (evt, ui) { // Get reference to map object. ui.map; }); // Initialize $(".selector").igMap({ refreshCompleted: function (evt, ui) { } });
-
seriesCursorMouseMove
- キャンセル可能:
- false
カーソルがこのマップのシリーズの上に移動したときに発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmapseriescursormousemove", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to chart object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesCursorMouseMove: function (evt, ui) { } });
-
seriesMouseEnter
- キャンセル可能:
- false
マウス ポインターがこのマップの要素に入ったときに発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmapseriesmouseenter", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseEnter: function (evt, ui) { } });
-
seriesMouseLeave
- キャンセル可能:
- false
マウス ポインターがこのマップの要素から離れたときに発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmapseriesmouseleave", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseLeave: function (evt, ui) { } });
-
seriesMouseLeftButtonDown
- キャンセル可能:
- false
マウス ポインターがこのマップの要素の上にある間に左のマウス ボタンが押されると発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmapseriesmouseleftbuttondown", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseLeftButtonDown: function (evt, ui) { } });
-
seriesMouseLeftButtonUp
- キャンセル可能:
- false
マウス ポインターがこのマップの要素の上にある間に左のマウス ボタンが離されたと発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmapseriesmouseleftbuttonup", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseLeftButtonUp: function (evt, ui) { } });
-
seriesMouseMove
- キャンセル可能:
- false
マウスのポインターがこのマップの要素の上にある間にマウス ポインターが移動すると発生します。
関数は引数 evt および ui を受け取ります。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。
マウス X 位置を取得するには ui.positionX を使用します。
マウス Y 位置を取得するには ui.positionY を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmapseriesmousemove", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseMove: function (evt, ui) { } });
-
tooltipHidden
- キャンセル可能:
- false
ツールチップが非表示になった後に発生するイベント。
関数は引数 evt および ui を受け取ります。
ツールチップの DOM 要素への参照を取得するには ui.element を使用します。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmaptooltiphidden", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igMap({ tooltipHidden: function (evt, ui) { } });
-
tooltipHiding
- キャンセル可能:
- true
マウスが系列から離れ、ツールチップが非表示になりそうなときに発生するイベント。
関数は引数 evt および ui を受け取ります。
ツールチップの DOM 要素への参照を取得するには ui.element を使用します。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmaptooltiphiding", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igMap({ tooltipHiding: function (evt, ui) { } });
-
tooltipShowing
- キャンセル可能:
- true
マウスがシリーズにホバーされ、ツールチップが表示されるときに発生するイベント。
関数は引数 evt および ui を受け取ります。
ツールチップの DOM 要素への参照を取得するには ui.element を使用します。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmaptooltipshowing", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to chart object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igMap({ tooltipShowing: function (evt, ui) { } });
-
tooltipShown
- キャンセル可能:
- false
ツールチップが表示された後に発生するイベント。
関数は引数 evt および ui を受け取ります。
ツールチップの DOM 要素への参照を取得するには ui.element を使用します。
現在のシリーズ項目オブジェクトへの参照を取得するには ui.item を使用します。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
項目ブラシを取得するには ui.actualItemBrush を使用します。
シリーズ ブラシを取得するには ui.actualSeriesBrush を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmaptooltipshown", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igMap({ tooltipShown: function (evt, ui) { } });
-
triangulationStatusChanged
- キャンセル可能:
- false
実行している Triangulation の状態が変更されたときに発生されるイベント。
関数は引数 evt および ui を受け取ります。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
現在のシリーズ オブジェクトへの参照を取得するには ui.series を使用します。
現在の状態を取得するには ui.currentStatus を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmaptriangulationstatuschanged", function (evt, ui) { // Get reference to chart object. ui.map; // Get the current series object. ui.series; // Get the current triangulation status. ui.currentStatus; }); // Initialize $(".selector").igMap({ triangulationStatusChanged: function (evt, ui) { } });
-
windowRectChanged
- キャンセル可能:
- false
現在の Map のウィンドウ矩形が変わった直後に発生します。
関数は引数 evt および ui を受け取ります。
マップ オブジェクトへの参照を取得するには ui.map を使用します。
新しい高さ値を取得するには ui.newHeight を使用します。
新しい左値を取得するには ui.newLeft を使用します。
新しい上値を取得するには ui.newTop を使用します。
新しい幅値を取得するには ui.newWidth を使用します。
以前の高さ値を取得するには ui.oldHeight を使用します。
以前の左値を取得するには ui.oldLeft を使用します。
以前の上値を取得するには ui.oldTop を使用します。
以前の幅値を取得するには ui.oldWidth を使用します。コード サンプル
// Delegate $(document).delegate(".selector", "igmapwindowrectchanged", function (evt, ui) { // Get reference to chart object. ui.map; // Get new height value. ui.newHeight; // Get new left value. ui.newLeft; // Get new top value. ui.newTop; // Get new top value. ui.newWidth; // Get old height value. ui.oldHeight; // Get old left value. ui.oldLeft; // Get old top value. ui.oldTop; // Get old top value. ui.oldWidth; }); // Initialize $(".selector").igMap({ windowRectChanged: function (evt, ui) { } });
-
addItem
- .igMap( "addItem", item:object, targetName:string );
新しい項目をデータ ソースに追加し、マップに通知します。
- item
- タイプ:object
- データ ソースに追加する必要がある項目。
- targetName
- タイプ:string
- データ ソースにバインドされたシリーズの名前。
コード サンプル
$(".selector").igMap("addItem", {"Latitude": 42.2, "Longitude": 22.5 }, "series1" );
-
changeGlobalLanguage
継承- .igMap( "changeGlobalLanguage" );
ウィジェットの言語をグローバルの言語に変更します。グローバルの言語は $.ig.util.language の値です。
コード サンプル
$(".selector").igMap("changeGlobalLanguage");
-
changeGlobalRegional
継承- .igMap( "changeGlobalRegional" );
ウィジェットの地域設定をグローバルの地域設定に変更します。グローバルの地域設定は $.ig.util.regional にあります。
コード サンプル
$(".selector").igMap("changeGlobalRegional");
-
changeLocale
継承- .igMap( "changeLocale", $container:object );
指定したコンテナーに含まれるすべてのロケールを options.language で指定した言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。- $container
- タイプ:object
- オプションのパラメーター: 設定しない場合、ウィジェットの要素を $container として使用します。
コード サンプル
$(".selector").igMap("changeLocale");
-
clearTileZoomCache
- .igMap( "clearTileZoomCache" );
新しいタイルが生成されるためにタイル ズームのタイル キャッシュをクリアします。ビューアーがタイル ズームを使用している場合のみ影響します。
コード サンプル
$(".selector").igMap("clearTileZoomCache");
-
destroy
- .igMap( "destroy" );
ウィジェットを破棄します。
コード サンプル
$(".selector").igMap("destroy");
-
endTiledZoomingIfRunning
- .igMap( "endTiledZoomingIfRunning" );
実行されている場合、タイル ズームを手動的に終了します。
コード サンプル
$(".selector").igMap("endTiledZoomingIfRunning");
-
exportImage
- .igMap( "exportImage", [width:object], [height:object] );
- 返却型:
- object
- 返却型の説明:
- IMG DOM 要素を返します。
マップを PNG 画像にエクスポートします。
- width
- タイプ:object
- オプション
- 画像の幅。
- height
- タイプ:object
- オプション
- 画像の高さ。
コード サンプル
var pngImage = $(".selector").igMap("exportImage", 200, 100);
-
exportVisualData
- .igMap( "exportVisualData" );
ユニット テストのためにマップの視覚的なデータをエクスポートします。
コード サンプル
var data = $(".selector").igMap("exportVisualData");
-
flush
- .igMap( "flush" );
続行する前に、マップの保留中の操作を描画します。
コード サンプル
$(".selector").igMap("flush");
-
getActualMaximumValue
- .igMap( "getActualMaximumValue", targetName:object );
ターゲットの xAxis または yAxis の実際の最大値を取得します。
- targetName
- タイプ:object
コード サンプル
// Get maximum value for geographic latitude var maxLat = $(".selector").igMap("getActualMaximumValue", "yAxis"); // Get maximum value for geographic longitude var maxLon = $(".selector").igMap("getActualMaximumValue", "xAxis");
-
getActualMinimumValue
- .igMap( "getActualMinimumValue", targetName:object );
ターゲットの xAxis または yAxis の実際の最小値を取得します。
- targetName
- タイプ:object
コード サンプル
// Get minimum value for geographic latitude var maxLat = $(".selector").igMap("getActualMinimumValue", "yAxis"); // Get minimum value for geographic longitude var maxLon = $(".selector").igMap("getActualMinimumValue", "xAxis");
-
getGeographicFromZoom
- .igMap( "getGeographicFromZoom", rect:object );
- 返却型:
- object
- 返却型の説明:
- ウィンドウ ズーム領域。
コントロールの現在のプロット エリアと地理的領域を指定して、その地理的領域を含む WindowRect を取得します。
- rect
- タイプ:object
- 地理的領域の四角形。
コード サンプル
var geographic = $(".selector").igMap("getGeographicFromZoom", { left: 0, top: 0, width: 0.5, height: 0.5 });
-
getZoomFromGeographic
- .igMap( "getZoomFromGeographic", rect:object );
- 返却型:
- object
- 返却型の説明:
- ウィンドウ ズーム領域。
コントロールの現在のプロット エリアと地理的領域を指定して、その地理的領域を含む WindowRect を取得します。
- rect
- タイプ:object
- 地理的領域の四角形。
コード サンプル
var zoom = $(".selector").igMap("getZoomFromGeographic", { left: 10, top: 10, width: 3, height: 3 });
-
id
- .igMap( "id" );
- 返却型:
- string
マップを保持する親要素の ID を返します。
コード サンプル
var dataContainerElement = $(".selector").igMap("id");
-
insertItem
- .igMap( "insertItem", item:object, index:number, targetName:string );
新しい項目をデータ ソースに挿入し、マップに通知します。
- item
- タイプ:object
- データ ソースに挿入する必要がある新規項目。
- index
- タイプ:number
- 新しい項目が挿入されるデータ ソースのインデックス。
- targetName
- タイプ:string
- データ ソースにバインドされたシリーズの名前。
コード サンプル
$(".selector").igMap("insertItem", {"Latitude": 42.2, "Longitude": 22.5 }, 1, "series1" );
-
notifyClearItems
- .igMap( "notifyClearItems", dataSource:object );
- 返却型:
- object
- 返却型の説明:
- この igMap への参照を返します。
項目が関連付けられたデータ ソースからクリアされたことをマップに通知します。
同じ項目のソースを共有しているかどうか、変更の複数のターゲットに通知する必要はありません。- dataSource
- タイプ:object
- 変更が発生したデータ ソース。
コード サンプル
var response = $(".selector").igMap("notifyClearItems", series1DataSource);
-
notifyContainerResized
- .igMap( "notifyContainerResized" );
コンテナーのサイズが変更されたことをマップに通知します。
コード サンプル
$(".selector").igMap("notifyContainerResized");
-
notifyInsertItem
- .igMap( "notifyInsertItem", dataSource:object, index:number, newItem:object );
- 返却型:
- object
- 返却型の説明:
- この igMap への参照を返します。
項目がデータ ソースの指定されたインデックスに挿入されたことを対象シリーズに通知します。
同じ項目のソースを共有しているかどうか、変更の複数のターゲットに通知する必要はありません。- dataSource
- タイプ:object
- 変更が発生したデータ ソース。
- index
- タイプ:number
- 新しい項目が挿入された項目ソースのインデックス。
- newItem
- タイプ:object
- コレクションに設定された新しい項目。
コード サンプル
var response = $(".selector").igMap("notifyInsertItem", series1DataSource, 1, {"Latitude": 42.2, "Longitude": 22.5 });
-
notifyRemoveItem
- .igMap( "notifyRemoveItem", dataSource:object, index:number, oldItem:object );
- 返却型:
- object
- 返却型の説明:
- この igMap への参照を返します。
項目がデータ ソースの指定されたインデックスから削除されたことを対象シリーズに通知します。
同じ項目のソースを共有しているかどうか、変更の複数のターゲットに通知する必要はありません。- dataSource
- タイプ:object
- 変更が発生したデータ ソース。
- index
- タイプ:number
- 旧項目の削除元となった項目ソースのインデックス。
- oldItem
- タイプ:object
- コレクションから削除された旧項目。
コード サンプル
var response = $(".selector").igMap("notifyRemoveItem", series1DataSource, 1, {"Latitude": 42.2, "Longitude": 22.5 });
-
notifySetItem
- .igMap( "notifySetItem", dataSource:object, index:number, newItem:object, oldItem:object );
- 返却型:
- object
- 返却型の説明:
- この igMap への参照を返します。
項目が関連付けられたデータ ソースに設定されたことをマップに通知します。
- dataSource
- タイプ:object
- 変更が発生したデータ ソース。
- index
- タイプ:number
- 変更された項目ソースのインデックス。
- newItem
- タイプ:object
- コレクションに設定された新しい項目。
- oldItem
- タイプ:object
- コレクションで上書きされた旧項目。
コード サンプル
var response = $(".selector").igMap("notifySetItem", series1DataSource, 1, {"Latitude": 42.2, "Longitude": 22.5 });
-
option
- .igMap( "option" );
コード サンプル
$(".selector").igMap("option");
-
print
- .igMap( "print" );
ページのすべての他の要素を非表示してマップの印刷プレビュー ページを作成します。
コード サンプル
$(".selector").igMap("print");
-
removeItem
- .igMap( "removeItem", index:number, targetName:string );
項目をデータ ソースから削除し、マップに通知します。
- index
- タイプ:number
- 項目が削除されるデータ ソースのインデックス。
- targetName
- タイプ:string
- データ ソースにバインドされたシリーズの名前。
コード サンプル
$(".selector").igMap("removeItem", 1, "series1");
-
renderSeries
- .igMap( "renderSeries", targetName:string, animate:bool );
更新を実行するオプションが変更されなくても、シリーズを描画することを示します。
- targetName
- タイプ:string
- 描画するシリーズの名前。
- animate
- タイプ:bool
- 可能な場合、変更をアニメーション化するかどうか。
コード サンプル
$(".selector").igMap("renderSeries", "xAxis", true);
-
resetZoom
- .igMap( "resetZoom" );
- 返却型:
- object
- 返却型の説明:
- この igMap への参照を返します。
マップのズーム レベルをデフォルト値にリセットします。
コード サンプル
var response = $(".selector").igMap("resetZoom");
-
scaleValue
- .igMap( "scaleValue", targetName:string, unscaledValue:number );
- 返却型:
- number
- 返却型の説明:
- スケールされた値を返します。
軸スペースからマップ スペースに要求された値をスケールする xAxis または yAxis (経度または緯度)。
たとえば、マップの幅にスケールされた経度 50 の位置を表示するには、このメソッドを使用できます。- targetName
- タイプ:string
- 通知する xAxis または yAxis。
- unscaledValue
- タイプ:number
- マップ スペースに変換する軸スペースの値。
コード サンプル
var scaled = $(".selector").igMap("scaleValue", "series1", 250);
-
scrollIntoView
- .igMap( "scrollIntoView", targetName:string, item:object );
- 返却型:
- object
- 返却型の説明:
- この igMap への参照を返します。
対象のシリーズまたは軸に要求されたデータ項目をビューにスクロールすることを通知します。
- targetName
- タイプ:string
- 通知するシリーズまたは軸の名前。
- item
- タイプ:object
- 可能な場合、ビューに入れるデータ項目。
コード サンプル
var response = $(".selector").igMap("scrollIntoView", 5);
-
setItem
- .igMap( "setItem", index:number, item:object, targetName:string );
データ ソース内の項目を更新し、マップに通知します。
- index
- タイプ:number
- 変更する必要があるデータ ソース内の項目のインデックス。
- item
- タイプ:object
- データ ソース内で設定する新規項目オブジェクト。
- targetName
- タイプ:string
- データ ソースにバインドされたシリーズの名前。
コード サンプル
$(".selector").igMap("setItem", 5, {"Latitude": 42.2, "Longitude": 22.5 }, "series1");
-
startTiledZoomingIfNecessary
- .igMap( "startTiledZoomingIfNecessary" );
実行されていない場合、タイル ズームを手動的に開始します。
コード サンプル
$(".selector").igMap("startTiledZoomingIfNecessary");
-
styleUpdated
- .igMap( "styleUpdated" );
- 返却型:
- object
- 返却型の説明:
- この igMap への参照を返します。
色の描画元になるスタイルが更新された可能性があることをマップに通知します。
コード サンプル
var response = $(".selector").igMap("styleUpdated");
-
unscaleValue
- .igMap( "unscaleValue", targetName:string, scaledValue:number );
- 返却型:
- number
- 返却型の説明:
- スケールされていない値を返します。
マップ スペースから軸スペースに要求された値をスケール解除する xAxis または yAxis (経度または緯度)。
たとえば、マップの 0 幅にスケール解除された経度の値を決定するには、このメソッドを使用できます。- targetName
- タイプ:string
- 通知する xAxis または yAxis。
- scaledValue
- タイプ:number
- 軸スペースに変換するマップ スペースの値。
コード サンプル
var unscaled = $(".selector").igMap("unscaleValue", "series1", 50);
-
zoomToGeographic
- .igMap( "zoomToGeographic", rect:object );
- 返却型:
- object
- 返却型の説明:
- この igMap への参照を返します。
指定した地理領域にズームインします。マップの初期化を完了するまでに待つ必要があります。
- rect
- タイプ:object
- 地理的領域の四角形。
コード サンプル
$(".selector").igMap("zoomToGeographic", { left: 10, top: 10, width: 3, height: 3 });
-
ui-corner-all ui-widget-content
- div 要素に適用されるクラスを取得または設定します。
-
ui-chart-tooltip ui-widget-content ui-corner-all
- ツールチップ div 要素に適用されるクラスを取得または設定します。
-
ui-chart-non-html5-supported-message ui-helper-clearfix
- マップが HTML5 の互換性のないブラウザーで開いた場合に div 要素に適用されるクラスを取得または設定します。