ui.igScheduler
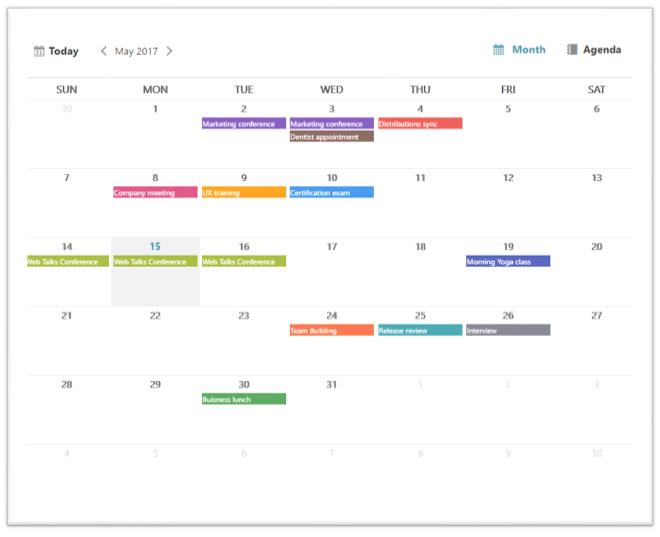
igScheduler コントロールは、時間範囲および関連アクティビティを表示し、管理するスケジュール ソリューションを提供します。
以下のコード スニペットは、igScheduler コントロールを初期化する方法を示します。
この API を使用して作業を開始するための情報はここをクリックしてください。igScheduler コントロールの必要なスクリプトおよびテーマを参照する方法については、「Ignite UI で JavaScript リソースを使用する」および「Ignite UI のスタイル設定とテーマ」を参照してください。コード サンプル
<!doctype html> <html> <head> <!-- Infragistics Combined CSS --> <link href="css/themes/infragistics/infragistics.theme.css" rel="stylesheet" type="text/css" /> <link href="css/structure/infragistics.css" rel="stylesheet" type="text/css" /> <!-- jQuery Core --> <script src="js/modernizr.min.js"></script> <script src="js/jquery.min.js"></script> <!-- jQuery UI --> <script src="js/jquery-ui.min.js" type="text/javascript"></script> <!-- Infragistics Scripts --> <script src="igniteui/js/infragistics.loader.js" type="text/javascript"></script> <script src="data-files/scheduler-data.js" type="text/javascript"></script> <script type="text/javascript"> $.ig.loader({ scriptPath: "../../igniteui/js/", cssPath: "../../igniteui/css/", resources: "igScheduler" }); $.ig.loader(function () { var scheduleListDataSource = new $.ig.scheduler.ScheduleListDataSource(), appointmentsDS = new $.ig.DataSource({ primaryKey: "id", dataSource: appointments }); appointmentsDS.dataBind(); scheduleListDataSource.resourceItemsSource(resources); scheduleListDataSource.appointmentItemsSource(appointmentsDS); $("#scheduler").igScheduler({ height: "550px", width: "100%", agendaViewSettings: { dateRangeInterval: 10 }, views: ["agendaView"], selectedDate: today, dataSource: scheduleListDataSource }); }); </script> </head> <body> <div id="scheduler"></div> </body> </html>
関連サンプル
関連トピック
依存関係
-
agendaViewSettings
- タイプ:
- object
- デフォルト:
- {}
AgendaView 設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ agendaViewSettings: { dateRangeInterval: 3 } }); //Get var setting = $(".selector").igScheduler("option", "agendaViewSettings"); //Set var setting = $(".selector").igScheduler("option", "agendaViewSettings"); setting.dateRangeInterval = 1; $(".selector").igScheduler("option", "agendaViewSettings", settings);
-
dateRangeInterval
- タイプ:
- number
- デフォルト:
- 7
AgendaView モードで表示される日数を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ agendaViewSettings: { dateRangeInterval: 3 } }); //Get var setting = $(".selector").igScheduler("option", "agendaViewSettings"); //Set var setting = $(".selector").igScheduler("option", "agendaViewSettings"); setting.dateRangeInterval = 1; $(".selector").igScheduler("option", "agendaViewSettings", settings);
-
appointmentDialogSuppress
- タイプ:
- bool
- デフォルト:
- false
予定ダイアログおよび関連する日および予定ポップアップを表示するかどうかを取得または設定します。
コード サンプル
// Initialize $(".selector").igScheduler({ appointmentDialogSuppress : true }); // Get var appointmentDialogSuppress = $(".selector").igScheduler("option", "appointmentDialogSuppress"); // Set $(".selector").igScheduler("option", "appointmentDialogSuppress", true);
-
dataSource
- タイプ:
- object
- デフォルト:
- null
$.ig.scheduler.ScheduleListDataSource 型の dataSource を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ dataSource : ds }); //Get var ds = $(".selector").igScheduler("option", "dataSource"); This code will return the appointments which are of type [$.ig.DataSource](ig.datasource) var appointmentItemsSource = ds.appointmentItemsSource(); This code will return the resources which are array of objects var appointmentItemsSource = ds.resourceItemsSource(); //Set $(".selector").igScheduler("option", "dataSource", ds);
-
dayViewSettings
- タイプ:
- object
- デフォルト:
- {}
DayView 設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ dayViewSettings: { timeSlotInterval: "tenMinutes", dayViewNumberOfDays: "3", workingHoursDisplayMode: "workingHoursOnly" } }); //Get var setting = $(".selector").igScheduler("option", "dayViewSettings"); //Set var setting = $(".selector").igScheduler("option", "dayViewSettings"); setting.timeSlotInterval = "tenMinutes"; $(".selector").igScheduler("option", "dayViewSettings", settings);
-
dayViewNumberOfDays
- タイプ:
- number
- デフォルト:
- 1
日表示で表示される日数を取得または設定します。1 日 ~ 7 日間がサポートされます。
コード サンプル
//Initialize $(".selector").igScheduler({ dayViewSettings: { dayViewNumberOfDays: 5 } }); //Get var setting = $(".selector").igScheduler("option", "dayViewSettings"); //Set var setting = $(".selector").igScheduler("option", "dayViewSettings"); setting.dayViewNumberOfDays = 5; $(".selector").igScheduler("option", "dayViewSettings", settings);
-
timeSlotInterval
- タイプ:
- number
- デフォルト:
- fiveMinutes
時間帯の期間を取得または設定します。5 分、6 分、10 分、15 分、30 分、および 60 分がサポートされます。
コード サンプル
//Initialize $(".selector").igScheduler({ dayViewSettings: { timeSlotInterval: "tenMinutes" } }); //Get var setting = $(".selector").igScheduler("option", "dayViewSettings"); //Set var setting = $(".selector").igScheduler("option", "dayViewSettings"); setting.timeSlotInterval = "tenMinutes"; $(".selector").igScheduler("option", "dayViewSettings", settings);
-
workingHoursDisplayMode
- タイプ:
- number
- デフォルト:
- workingHoursOnly
すべての時または稼動時間のみを表示するかどうかを取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ dayViewSettings: { workingHoursDisplayMode: "workingHoursAndNonWorkingHours" } }); //Get var setting = $(".selector").igScheduler("option", "dayViewSettings"); //Set var setting = $(".selector").igScheduler("option", "dayViewSettings"); setting.workingHoursDisplayMode = "workingHoursAndNonWorkingHours"; $(".selector").igScheduler("option", "dayViewSettings", settings);
-
enableTodayButton
- タイプ:
- bool
- デフォルト:
- true
[今日] ボタンを有効/無効にします。
コード サンプル
//Initialize $(".selector").igScheduler({ enableTodayButton: false }); //Get var isEnabled = $(".selector").igScheduler("option", "enableTodayButton");
-
height
- タイプ:
- enumeration
- デフォルト:
- 100%
コントロールの高さを取得または設定します。
メンバー
- null
- タイプ:object
- 他の高さが定義されていない場合、エディターは親コンテナーに収まります。
- string
- 高さをピクセル (px) またはパーセンテージ (%) に設定できます。
- number
- 高さをピクセル単位の数値で設定できます。
コード サンプル
//Initialize $(".selector").igScheduler({ height : 250 }); //Get var height = $(".selector").igScheduler("option", "height");
-
language
継承- タイプ:
- string
- デフォルト:
- "en"
ウィジェットのロケール言語設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ language: "ja" }); // Get var language = $(".selector").igScheduler("option", "language"); // Set $(".selector").igScheduler("option", "language", "ja");
-
locale
継承- タイプ:
- object
- デフォルト:
- null
ウィジェットのロケール設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ locale: {} }); // Get var locale = $(".selector").igScheduler("option", "locale"); // Set $(".selector").igScheduler("option", "locale", {});
-
monthViewSettings
- タイプ:
- object
- デフォルト:
- {}
MonthView 設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { isAgendaVisible: true, agendaVisibilityType: "onlyAppointmentsForSelectedMonthViewDay", viewSplitOrientation: "vertical", isHorizontalSeparatorVisibile: true, isVerticalSeparatorVisibile: true, isWeekdayVisible: true, isWeekNumberVisible: true, isPreviousMonthShown: true, isNextMonthShown: true } }); //Get var setting = $(".selector").igScheduler("option", "monthViewSettings"); //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.isHorizontalSeparatorVisibile = false; settings.isVerticalSeparatorVisibile = false; settings.isWeekdayVisible = false; settings.isWeekNumberVisible = false; settings.isPreviousMonthShown = false; settings.isNextMonthShown = false; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
agendaVisibilityType
- タイプ:
- string
- デフォルト:
- "allAppointments"
MonthView の AgendaView で表示される予定のスコープを取得または設定します。
allAppointments - MonthView で AgendaView がセカンダリ ビューの場合、すべての日の予定が表示されることを示します。
onlyAppointmentsForSelectedMonthViewDay - セカンダリ ビューの MonthView と関連付けられた AgendaView で MonthView の現在選択されている日のみの予定が表示されることを示します。コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { agendaVisibilityType: "onlyAppointmentsForSelectedMonthViewDay" } }); //Get var type = $(".selector").igScheduler("option", "monthViewSettings").agendaVisibilityType;
-
appointmentMode
- タイプ:
- string
- デフォルト:
- "auto"
MonthView 日で表示されるコンテンツのタイプを取得または設定します。
auto - スケジューラのサイズが 768px より大きい場合、画面サイズに基づいて、月ビューで予定の四角インジケーター モードを示します。それ以外の場合、水平のモードを識別します。
indicator - 四角インジケーターが表示されることを示します。
detailed - 件名が表示されることを示します。コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { appointmentMode: "indicator" } }); //Get var mode = $(".selector").igScheduler("option", "monthViewSettings").appointmentMode; //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.viewSplitOrientation = "indicator"; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
isAgendaVisible
- タイプ:
- bool
- デフォルト:
- false
MonthView で AgendaView の表示状態を取得または設定します。true の場合、MonthView は、Appointments のリストの上にある選択されている日の Appointments を表示する AgendaView を表示します。
コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { isAgendaVisible: true } }); //Get var isVisible = $(".selector").igScheduler("option", "monthViewSettings").isAgendaVisible;
-
isHorizontalSeparatorVisibile
- タイプ:
- bool
- デフォルト:
- true
MonthView の週の間の水平セパレーターの表示状態を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { isHorizontalSeparatorVisibile: true } }); //Get var isVisible = $(".selector").igScheduler("option", "monthViewSettings").isHorizontalSeparatorVisibile; //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.isHorizontalSeparatorVisibile = false; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
isNextMonthShown
- タイプ:
- bool
- デフォルト:
- true
特定の月の最後の週にある以後の月の日の表示状態を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { isNextMonthShown: true } }); //Get var isVisible = $(".selector").igScheduler("option", "monthViewSettings").isNextMonthShown; //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.isNextMonthShown = false; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
isPreviousMonthShown
- タイプ:
- bool
- デフォルト:
- true
特定の月の最初の週にある以前の月の日の表示状態を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { isPreviousMonthShown: true } }); //Get var isVisible = $(".selector").igScheduler("option", "monthViewSettings").isPreviousMonthShown; //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.isPreviousMonthShown = false; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
isVerticalSeparatorVisibile
- タイプ:
- bool
- デフォルト:
- false
MonthView の曜日の間の垂直セパレーターの表示状態を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { isVerticalSeparatorVisibile: true } }); //Get var isVisible = $(".selector").igScheduler("option", "monthViewSettings").isVerticalSeparatorVisibile; //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.isVerticalSeparatorVisibile = false; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
isWeekdayVisible
- タイプ:
- bool
- デフォルト:
- true
MonthView で曜日名の表示状態を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { isWeekdayVisible: true } }); //Get var isVisible = $(".selector").igScheduler("option", "monthViewSettings").isWeekdayVisible; //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.isWeekdayVisible = false; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
isWeekNumberVisible
- タイプ:
- bool
- デフォルト:
- false
MonthView で週番号の表示状態を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { isWeekNumberVisible: true } }); //Get var isVisible = $(".selector").igScheduler("option", "monthViewSettings").isWeekNumberVisible; //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.isWeekNumberVisible = false; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
viewSplitOrientation
- タイプ:
- string
- デフォルト:
- "auto"
MonthView および AgendaView が垂直方向または水平方向に分割されるかどうかを決定する方向を取得または設定します。このオプションは、AgendaView が MonthView で表示される場合に使用可能です。
auto - スケジューラのサイズが 768px より大きい場合、画面サイズに基づいて、垂直分割を示します。それ以外の場合、水平のモードを識別します。
vertical - Scheduler のビューの間の垂直分割を識別します。
horizontal - Scheduler のビューの間の水平分割を識別します。コード サンプル
//Initialize $(".selector").igScheduler({ monthViewSettings: { viewSplitOrientation: "horizontal" } }); //Get var orientation = $(".selector").igScheduler("option", "monthViewSettings").viewSplitOrientation; //Set var setting = $(".selector").igScheduler("option", "monthViewSettings"); settings.viewSplitOrientation = "horizontal"; $(".selector").igScheduler("option", "monthViewSettings", settings);
-
regional
継承- タイプ:
- enumeration
- デフォルト:
- en-US
ウィジェットの領域設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ regional: "ja" }); // Get var regional = $(".selector").igScheduler("option", "regional"); // Set $(".selector").igScheduler("option", "regional", "ja");
-
resources
- タイプ:
- object
- デフォルト:
- null
アクティビティのオーナーを含むリソース コレクションを取得します。
コード サンプル
//Initialize var resources = [ { id: 1, displayName: "Trina Friesen" }, { id: 2, displayName: "Mack Koch" }]; $(".selector").igScheduler({ resources: resources }); // Get var regional = $(".selector").igScheduler("option", "resources");
-
selectedDate
- タイプ:
- date
- デフォルト:
- new newDate()
スケジューラで選択された日付を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ selectedDate: new Date(2017, 3, 5) }); //Get var date = $(".selector").igScheduler("option", "selectedDate"); //Set $(".selector").igScheduler("option", "selectedDate", new Date(2017, 3, 5));
-
viewMode
- タイプ:
- enumeration
- デフォルト:
- null
Scheduler の現在ビュー モードを取得または設定します。この位置が定義されていない場合、views プロパティで最初に定義したビューが取得されます。
メンバー
- monthView
- タイプ:string
- Scheduler で MonthView を有効にします。
- agendaView
- タイプ:string
- Scheduler で AgendaView を有効にします。
コード サンプル
//Initialize $(".selector").igScheduler({ viewMode: "agendaView" }); //Get var mode = $(".selector").igScheduler("option", "viewMode"); //Set $(".selector").igScheduler("option", "width", "agendaView");
-
views
- タイプ:
- array
- デフォルト:
- []
- 要素タイプ:
- string
Scheduler で描画されるビューをすべてリストします。
コード サンプル
//Initialize $(".selector").igScheduler({ views: ["monthView", "agendaView"] }); //Get var views = $(".selector").igScheduler("option", "views");
-
weekViewSettings
- タイプ:
- object
- デフォルト:
- {}
WeekView 設定を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ weekViewSettings: { timeSlotInterval: "fiveMinutes", weekViewDisplayMode: "workingDaysOnly", workingHoursDisplayMode: "workingHoursOnly" } }); //Get var setting = $(".selector").igScheduler("option", "weekViewSettings"); //Set var setting = $(".selector").igScheduler("option", "weekViewSettings"); setting.timeSlotInterval = 30; $(".selector").igScheduler("option", "weekViewSettings", settings);
-
timeSlotInterval
- タイプ:
- number
- デフォルト:
- fiveMinutes
時間帯の期間を取得または設定します。5 分、6 分、10 分、15 分、30 分、および 60 分がサポートされます。
コード サンプル
//Initialize $(".selector").igScheduler({ weekViewSettings: { timeSlotInterval: "tenMinutes" } }); //Get var setting = $(".selector").igScheduler("option", "weekViewSettings"); //Set var setting = $(".selector").igScheduler("option", "weekViewSettings"); setting.timeSlotInterval = "tenMinutes"; $(".selector").igScheduler("option", "weekViewSettings", settings);
-
weekViewDisplayMode
- タイプ:
- number
- デフォルト:
- workingDaysOnly
週表示の表示モード (すべての日または稼動日のみ) を取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ weekViewSettings: { weekViewDisplayMode: "workingDaysOnly" } }); //Get var setting = $(".selector").igScheduler("option", "weekViewSettings"); //Set var setting = $(".selector").igScheduler("option", "weekViewSettings"); setting.weekViewDisplayMode = "all7Days"; $(".selector").igScheduler("option", "weekViewSettings", settings);
-
workingHoursDisplayMode
- タイプ:
- number
- デフォルト:
- workingHoursOnly
すべての時または稼動時間のみを表示するかどうかを取得または設定します。
コード サンプル
//Initialize $(".selector").igScheduler({ weekViewSettings: { workingHoursDisplayMode: "workingHoursOnly" } }); //Get var setting = $(".selector").igScheduler("option", "weekViewSettings"); //Set var setting = $(".selector").igScheduler("option", "weekViewSettings"); setting.workingHoursDisplayMode = "workingHoursOnly"; $(".selector").igScheduler("option", "weekViewSettings", settings);
-
width
- タイプ:
- enumeration
- デフォルト:
- 100%
コントロールの幅を取得または設定します。
メンバー
- null
- タイプ:object
- 他の幅が定義されていない場合、データに合わせて引き伸ばされます。
- string
- ウィジェットの幅をピクセル (px) またはパーセンテージ (%) に設定できます。
- number
- ウィジェットの幅は数値としてピクセルで設定できます。
コード サンプル
//Initialize $(".selector").igScheduler({ width : 700 }); //Get var width = $(".selector").igScheduler("option", "width");
Ignite UI コントロール イベントの詳細については、
Ignite UI でイベントを使用するを参照してください。
-
agendaRangeChanged
- キャンセル可能:
- false
[前へ] および [次へ] ボタンによって予定一覧ビューの範囲が変更した後に発生されます。予定一覧ビューのみで発生されます。
コード サンプル
//Bind after initialization $(document).on("igscheduleragendarangechanged", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the days to be shown in AgendaView mode. ui.dateRangeInterval; //return reference to the AgendaView start date. ui.newAgendaRangeStartDate; }); //Initialize $(".selector").igScheduler({ agendaRangeChanged: function(evt, ui) {...} });
-
agendaRangeChanging
- キャンセル可能:
- true
[前へ] および [次へ] ボタンによって予定一覧ビューの範囲が変更する前に発生されます。予定一覧ビューのみで発生されます。
コード サンプル
//Bind after initialization $(document).on("igscheduleragendarangechanging", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the days to be shown in AgendaView mode. ui.dateRangeInterval; //return reference to the current AgendaView start date. ui.currentAgendaRangeStartDate; //return reference to the new AgendaView start date. ui.newAgendaRangeStartDate; }); //Initialize $(".selector").igScheduler({ agendaRangeChanging: function(evt, ui) {...} });
-
appointmentCreated
- キャンセル可能:
- false
予定が作成された後に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentcreated", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the created appointment ui.appointment; }); // Initialize $(".selector").igScheduler({ appointmentCreated: function(evt, ui) {...} });
-
appointmentCreating
- キャンセル可能:
- true
予定が作成される前に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentcreating", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the appointment which is going to be created ui.appointment; }); // Initialize $(".selector").igScheduler({ appointmentCreating: function(evt, ui) {...} });
-
appointmentDeleted
- キャンセル可能:
- false
予定が削除された後に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentdeleted", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns the id of the deleted appointment ui.appointmentId; }); // Initialize $(".selector").igScheduler({ appointmentDeleted: function(evt, ui) {...} });
-
appointmentDeleting
- キャンセル可能:
- true
予定が削除される前に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentdeleting", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the appointment which is going to be deleted ui.appointment; }); // Initialize $(".selector").igScheduler({ appointmentDeleting: function(evt, ui) {...} });
-
appointmentDialogClosed
- キャンセル可能:
- false
予定の追加/編集のダイアログ ウィンドウが閉じた後に発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentdialogclosed", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the appointment dialog ui.element; // returns whether we are creating or updating an appointment ui.isAppointmentNew; }); // Initialize $(".selector").igScheduler({ appointmentDialogClosed: function(evt, ui) {...} });
-
appointmentDialogClosing
- キャンセル可能:
- true
予定の追加/編集のダイアログが閉じる前に発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentdialogclosing", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the appointment dialog ui.element; // returns whether we are creating or updating an appointment ui.isAppointmentNew; }); // Initialize $(".selector").igScheduler({ appointmentDialogClosing: function(evt, ui) {...} });
-
appointmentDialogOpened
- キャンセル可能:
- false
予定の作成/編集のダイアログが開いた後に発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentdialogopened", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the appointment dialog ui.element; // returns whether we are creating or updating an appointment ui.isAppointmentNew; }); // Initialize $(".selector").igScheduler({ appointmentDialogOpened: function(evt, ui) {...} });
-
appointmentDialogOpening
- キャンセル可能:
- true
予定の追加/編集のダイアログが開く前に発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentdialogopening", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the appointment dialog ui.element; // returns whether we are creating or updating an appointment ui.isAppointmentNew; }); // Initialize $(".selector").igScheduler({ appointmentDialogOpening: function(evt, ui) {...} });
-
appointmentEdited
- キャンセル可能:
- false
予定が編集された後に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentedited", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the edited appointment ui.appointment; }); // Initialize $(".selector").igScheduler({ appointmentEdited: function(evt, ui) {...} });
-
appointmentEditing
- キャンセル可能:
- true
予定が編集される前に発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerappointmentediting", ".selector", function (evt, ui) { // returns a reference to the scheduler ui.owner; // returns a reference to the original appointment ui.appointment; // returns a reference to the appointment with the edited values ui.newAppointment; }); // Initialize $(".selector").igScheduler({ appointmentEditing: function(evt, ui) {...} });
-
dayChanged
- キャンセル可能:
- false
[前へ] および [次へ] ボタンによって日が変更された後に発生されます。日ビューのみで発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerdaychanged", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the selected date. ui.newSelectedDate; }); //Initialize $(".selector").igScheduler({ dayChanged: function(evt, ui) {...} });
-
dayChanging
- キャンセル可能:
- true
[前へ] および [次へ] ボタンによって日が変更される前に発生されます。日ビューのみで発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerdaychanging", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the new selected date. ui.newSelectedDate; //return reference to the currently selected date. ui.currentSelectedDate; }); //Initialize $(".selector").igScheduler({ dayChanging: function(evt, ui) {...} });
-
daySelected
- キャンセル可能:
- false
日が datepicker カレンダーから選択されたときに発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerdayselected", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the selected date. ui.date; }); //Initialize $(".selector").igScheduler({ daySelected: function(evt, ui) {...} });
-
monthChanged
- キャンセル可能:
- false
[前へ] および [次へ] ボタンによって月が変更した後に発生されます。月ビューのみで発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulermonthchanged", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the selected date. ui.newSelectedDate; }); //Initialize $(".selector").igScheduler({ monthChanged: function(evt, ui) {...} });
-
monthChanging
- キャンセル可能:
- true
[前へ] および [次へ] ボタンによって月が変更される前に発生されます。月ビューのみで発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulermonthchanging", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the new selected date. ui.newSelectedDate; //return reference to the currently selected date. ui.currentSelectedDate; }); //Initialize $(".selector").igScheduler({ monthChanging: function(evt, ui) {...} });
-
rendered
- キャンセル可能:
- false
スケジューラのレンダリングが完了した後に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerrendered", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; }); //Initialize $(".selector").igScheduler({ rendered: function(evt, ui) {...} });
-
rendering
- キャンセル可能:
- false
スケジューラのレンダリングが開始する前に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerrendering", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; }); //Initialize $(".selector").igScheduler({ rendering: function(evt, ui) {...} });
-
viewChanged
- キャンセル可能:
- false
メニュー ボタンを使用することによりビューが変更された後に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerviewchanged", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the newly selected view. ui.newSelectedView; }); //Initialize $(".selector").igScheduler({ viewChanged: function(evt, ui) {...} });
-
viewChanging
- キャンセル可能:
- true
メニュー ボタンを使用することによりビューが変更される前に発生します。
コード サンプル
//Bind after initialization $(document).on("igschedulerviewchanging", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the currently selected view. ui.currentSelectedView //return reference to the newly selected view. ui.newSelectedView; }); //Initialize $(".selector").igScheduler({ viewChanging: function(evt, ui) {...} });
-
weekChanged
- キャンセル可能:
- false
[前へ] および [次へ] ボタンによって週が変更された後に発生されます。週ビューのみで発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerweekchanged", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the selected date. ui.newSelectedDate; }); //Initialize $(".selector").igScheduler({ weekChanged: function(evt, ui) {...} });
-
weekChanging
- キャンセル可能:
- true
[前へ] および [次へ] ボタンによって週が変更される前に発生されます。週ビューのみで発生されます。
コード サンプル
//Bind after initialization $(document).on("igschedulerweekchanging", ".selector", function (evt, ui) { //return reference to the scheduler. ui.owner; //return reference to the new selected date. ui.newSelectedDate; //return reference to the currently selected date. ui.currentSelectedDate; }); //Initialize $(".selector").igScheduler({ weekChanging: function(evt, ui) {...} });
-
changeGlobalLanguage
継承- .igScheduler( "changeGlobalLanguage" );
ウィジェットの言語をグローバルの言語に変更します。グローバルの言語は $.ig.util.language の値です。
コード サンプル
$(".selector").igScheduler("changeGlobalLanguage");
-
changeGlobalRegional
継承- .igScheduler( "changeGlobalRegional" );
ウィジェットの地域設定をグローバルの地域設定に変更します。グローバルの地域設定は $.ig.util.regional にあります。
コード サンプル
$(".selector").igScheduler("changeGlobalRegional");
-
changeLocale
- .igScheduler( "changeLocale" );
ウィジェット要素のすべてのロケールを options.language に指定される言語に変更します。
注: このメソッドは珍しいシナリオのみで使用されます。language または locale オプションのセッターを参照してください。コード サンプル
$(".selector").igScheduler("changeLocale");
-
createAppointment
- .igScheduler( "createAppointment", appointment:object );
予定を作成して appointment コレクションに追加します。
- appointment
- タイプ:object
- 予定。
コード サンプル
$(".selector").igScheduler("createAppointment", appointment);
-
dateRangeButton
- .igScheduler( "dateRangeButton" );
- 返却型:
- jquery
- 返却型の説明:
- 視覚エディター要素。
日付範囲の UI ボタンへの参照を取得します。
コード サンプル
$(".selector").igScheduler("dateRangeButton");
-
deleteAppointment
- .igScheduler( "deleteAppointment", appointment:object );
予定を予定コレクションから削除します。
- appointment
- タイプ:object
- 予定。
コード サンプル
$(".selector").igScheduler("deleteAppointment", appointment);
-
destroy
- .igScheduler( "destroy" );
ウィジェットを破棄します。
コード サンプル
$(".selector").igScheduler("destroy");
-
editAppointment
- .igScheduler( "editAppointment", appointment:object, updateAppoinment:object );
予定を編集します。
- appointment
- タイプ:object
- 予定。
- updateAppoinment
- タイプ:object
コード サンプル
$(".selector").igScheduler("editAppointment", appointment, { subject: "Some subject", location: "Somewhere", start: new Date(2017, 04, 05, 12, 30), end: new Date(2017, 04, 05, 12, 30), resourceId: 4, description: "Lorem ipsum dolor sit amet, consectetur adipisicing elit." });
-
getAppointmentsInRange
- .igScheduler( "getAppointmentsInRange", start:date, end:date );
指定した時間範囲ですべての予定のコレクションへの参照を取得します。
- start
- タイプ:date
- 開始日付。
- end
- タイプ:date
- 終了日付。
コード サンプル
$(".selector").igScheduler("getAppointmentsInRange", startDate, endDate);
-
getCalendar
- .igScheduler( "getCalendar" );
- 返却型:
- jquery
- 返却型の説明:
- 視覚エディター要素。
jQuery カレンダー UI コントロールへの参照を取得します。
コード サンプル
$(".selector").igScheduler("getCalendar");
-
nextButton
- .igScheduler( "nextButton" );
- 返却型:
- jquery
- 返却型の説明:
- 視覚エディター要素。
[次へ] の UI ボタンへの参照を取得します。
コード サンプル
$(".selector").igScheduler("nextButton");
-
previousButton
- .igScheduler( "previousButton" );
- 返却型:
- jquery
- 返却型の説明:
- 視覚エディター要素。
[前へ] の UI ボタンへの参照を取得します。
コード サンプル
$(".selector").igScheduler("previousButton");
-
todayButton
- .igScheduler( "todayButton" );
- 返却型:
- jquery
- 返却型の説明:
- 視覚エディター要素。
[今日] の UI ボタンへの参照を取得します。
コード サンプル
$(".selector").igScheduler("todayButton");
-
ui-icon ui-icon-note
- 予定一覧ビューのタブ アイコンに適用されるクラス。デフォルト値は 'ui-icon ui-icon-note' です。
-
ui-igscheduler-appointment-dialog
- 予定ダイアログに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog' です。
-
ui-igscheduler-appointment-dialog-cancel-button
- 予定ダイアログの [キャンセル] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-cancel-button' です。
-
ui-igscheduler-appointment-dialog-create-button
- 予定ダイアログの [作成] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-create-button' です。
-
ui-igscheduler-appointment-dialog-description
- 予定ダイアログの説明エディターに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-description' です。
-
ui-igscheduler-appointment-dialog-form
- 予定ダイアログのフォームに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-form' です。
-
ui-igscheduler-appointment-form-group
- 予定ダイアログのフォーム グループに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-form-group' です。
-
ui-igscheduler-appointment-dialog-from-date
- 予定ダイアログの開始日付 datepicker に適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-from-date' です。
-
ui-igscheduler-appointment-dialog-from-date-time-label
- 予定ダイアログの開始日付 datetime ラベルに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-from-date-time-label' です。
-
ui-igscheduler-appointment-dialog-from-time
- 予定ダイアログの開始日付 timepicker に適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-from-time' です。
-
ui-igscheduler-appointment-dialog-location
- 予定ダイアログの場所エディターに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-location' です。
-
ui-igscheduler-appointment-dialog-save-button
- 予定ダイアログの [保存] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-save-button' です。
-
ui-igscheduler-appointment-dialog-subject
- 予定ダイアログの件名エディターに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-subject' です。
-
ui-igscheduler-appointment-dialog-to-date
- 予定ダイアログの終了日付 datepicker に適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-to-date' です。
-
ui-igscheduler-appointment-dialog-to-date-time-label
- 予定ダイアログの終了日付 datetime ラベルに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-to-date-time-label' です。
-
ui-igscheduler-appointment-dialog-to-time
- 予定ダイアログの終了日付 timepicker に適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-dialog-to-time' です。
-
ui-igscheduler-appointment-actions
- 予定ポップオーバーの「開く」と「削除」操作に適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-actions' です。
-
ui-igscheduler-appointment-popover-content
- 予定ポップオーバーのコンテンツに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-popover-content' です。
-
ui-igscheduler-appointment-popover-delete-button ui-igdanger-btn
- 予定ポップオーバーの [削除] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-popover-delete-button ui-igdanger-btn' です。
-
ui-igscheduler-appointment-popover-from-to
- 予定ポップオーバーの開始時間および終了時間に適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-popover-from-to' です。
-
ui-igscheduler-appointment-popover-open-button
- 予定ポップオーバーの [開く] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-popover-open-button' です。
-
ui-igscheduler-appointment-popover-subject
- 予定ポップオーバーの件名に適用されるクラス。デフォルト値は 'ui-igscheduler-appointment-popover-subject' です。
-
ui-igscheduler-body
- スケジュール ビューを含む body 要素に適用されるクラス。デフォルト値は 'ui-igscheduler-body' です。
-
ui-widget ui-corner-all ui-state-default
- エディターがコンテナーに描画される場合に最上位の要素に適用されるクラス。デフォルト値は 'ui-widget ui-corner-all ui-state-default' です。
-
ui-igscheduler-navigator-date-range-button
- AgendaView モードでドロップダウン ボタンとして操作する日付表示要素に適用されるクラス。デフォルト値は 'ui-igscheduler-navigator-date-range-button' です。
-
date-range-button-text
- 日付表示 span 要素に適用されるクラス。デフォルト値は 'date-range-button-text' です。
-
ui-igscheduler-day-popover-content
- 日ポップオーバーのコンテンツに適用されるクラス。デフォルト値は 'ui-igscheduler-day-popover-content' です。
-
ui-igscheduler-day-popover-create-button
- 日ポップオーバーの [作成] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-day-popover-create-button' です。
-
ui-icon ui-icon-day
- 日ビューのタブ アイコンに適用されるクラス。デフォルト値は 'ui-icon ui-icon-day' です。
-
ui-igscheduler-delete-appointment-dialog-actions
- 予定の削除ダイアログの操作に適用されるクラス。デフォルト値は 'ui-igscheduler-delete-appointment-dialog-actions' です。
-
ui-igscheduler-delete-appointment-dialog-cancel-button
- 予定の削除ダイアログの [キャンセル] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-delete-appointment-dialog-cancel-button' です。
-
ui-igscheduler-delete-appointment-dialog-confirmation
- 予定の削除確認ダイアログに適用されるクラス。デフォルト値は 'ui-igscheduler-delete-appointment-dialog-confirmation' です。
-
ui-igscheduler-delete-appointment-dialog-delete-button ui-igdanger-btn
- 予定の削除ダイアログの [削除] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-delete-appointment-dialog-delete-button ui-igdanger-btn' です。
-
ui-igscheduler-delete-occurrence-dialog-actions
- インスタンスの削除ダイアログの操作に適用されるクラス。デフォルト値は 'ui-igscheduler-delete-occurrence-dialog-actions' です。
-
ui-igscheduler-delete-occurrence-dialog-cancel-button
- インスタンスの削除ダイアログの [キャンセル] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-delete-occurrence-dialog-cancel-button' です。
-
ui-igscheduler-delete-occurrence-dialog-delete-button ui-igdanger-btn
- インスタンスの削除ダイアログの [削除] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-delete-occurrence-dialog-delete-button ui-igdanger-btn' です。
-
ui-igscheduler-delete-occurrence-dialog-delete-series-button ui-igdanger-btn
- 予定の削除ダイアログの [シリーズを削除] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-delete-occurrence-dialog-delete-series-button ui-igdanger-btn' です。
-
ui-igscheduler-delete-occurrence-dialog-text
- インスタンスの削除ダイアログのテキストに適用されるクラス。デフォルト値は 'ui-igscheduler-delete-occurence-text' です。
-
ui-igscheduler-navigator-disabled-date-range-button
- MonthView の日付表示要素に適用されるクラス。デフォルト値は 'ui-igscheduler-navigator-disabled-date-range-button' です。
-
ui-igscheduler-edit-appointment-dialog-actions
- 予定の編集ダイアログの操作に適用されるクラス。デフォルト値は 'ui-igscheduler-edit-appointment-dialog-actions' です。
-
ui-igscheduler-edit-appointment-dialog-confirmation
- 予定の編集確認ダイアログに適用されるクラス。デフォルト値は 'ui-igscheduler-edit-appointment-dialog-confirmation' です。
-
ui-igscheduler-edit-appointment-dialog-occurrence-button
- 予定の編集ダイアログの [今回のみ] の編集ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-edit-appointment-dialog-occurrence-button' です。
-
ui-igscheduler-delete-appointment-dialog-series-button
- 予定の編集ダイアログの [削除] ボタンに適用されるクラス。デフォルト値は 'ui-igscheduler-edit-appointment-dialog-series-button' です。
-
ui-igscheduler-header
- 日付およびビューのナビゲーションを含むヘッダー要素に適用されるクラス。デフォルト値は 'ui-igscheduler-header' です。
-
ui-icon ui-icon-calendar
- 月ビューのタブ アイコンに適用されるクラス。デフォルト値は 'ui-icon ui-icon-calendar' です。
-
ui-igscheduler-navigator
- 日付ナビゲーションのコンテナー要素に適用されるクラス。デフォルト値は 'ui-igscheduler-navigator' です。
-
ui-igscheduler-navigator-next-button
- [次へ] ボタン要素に適用されるクラス。デフォルト値は 'ui-igscheduler-navigator-next-button' です。
-
ui-igscheduler-navigator-previous-button
- [前へ] ボタン要素に適用されるクラス。デフォルト値は 'ui-igscheduler-navigator-previous-button' です。
-
ui-igscheduler-recurrence-checkbox
- コンボのリソース要素に適用されるクラス。
-
ui-igscheduler-resources-combo
- コンボのリソース要素に適用されるクラス。
-
ui-igscheduler-resources-combo-item-color
- コンボの項目リソース色要素に適用されるクラス。
-
ui-igscheduler-resources-combo-item-text
- コンボの項目リソース テキスト要素に適用されるクラス。
-
ui-igscheduler
- メイン/最上位の要素に適用されるクラス。デフォルト値は 'ui-igscheduler' です。
-
ui-igscheduler-tabs-selected
- 選択したビューのタブに適用されるクラス。デフォルト値は 'ui-igscheduler-tabs-selected' です。
-
ui-igscheduler-side-by-side-group
- 予定ダイアログで入力フィールドのグループに適用されるクラス。デフォルト値は 'ui-igscheduler-side-by-side-group' です。
-
ui-igscheduler-tabs
- ビュー モードのタブに適用されるクラス。デフォルト値は 'ui-igscheduler-tabs' です。
-
ui-igscheduler-tabs-container
- タブ コンテナーに適用されるクラス。デフォルト値は 'ui-igscheduler-tabs-container' です。
-
ui-igscheduler-today-button
- [今日] ボタン要素に適用されるクラス。デフォルト値は 'ui-igscheduler-today-button' です。
-
ui-icon ui-icon-calendar-day
- [今日] ボタン アイコンに適用されるクラス。デフォルト値は 'ui-icon ui-icon-calendar-day' です。
-
ui-icon ui-icon-week
- 週ビューのタブ アイコンに適用されるクラス。デフォルト値は 'ui-icon ui-icon-week' です。